类的访问修饰符
修饰符有default Public Private Internal Protected Protected internal等几种
现在从(类内部、子类、程序集内、程序集外)对他们的可访问范围进行了解:
using
System;
namespace
testclassmodifier<?xml:namespace prefix = o ns = "urn:schemas-microsoft-com:office:office" />
{ class Mod
{ void defaultMethod()
{Console.WriteLine("
我是用default修饰的方法"
);}
public void publicMethod()
{Console.WriteLine("
我是用public修饰的方法"
);}
private void privateMethod()
{Console.WriteLine("
我是用private修饰的方法"
);}
internal void internalMethod()
{Console.WriteLine("
我是用internal修饰的方法"
);}
protected void protectedMethod()
{Console.WriteLine("
我是用protected修饰的方法"
);}
protected internal void protectedInternalMethod()
{Console.WriteLine("
我是用protected internal修饰的方法"
);}
static void Main(string[] args)
{ Mod mod = new Mod();
mod.defaultMethod();
mod.publicMethod();
mod.privateMethod();
mod.internalMethod();
mod.protectedMethod();
mod.protectedInternalMethod();
} }}
运行结果:
我是用default修饰的方法
我是用public修饰的方法
我是用private修饰的方法
我是用internal修饰的方法
我是用protected修饰的方法
我是用protected internal修饰的方法
请按任意键继续. . .
可见这六个方法再同一个类中都可以被访问
现在我们把Main方法放到外面看看在同一个程序集(
testclassmodifier
)内是否可被访问
namespace
testclassmodifier
{
class Mod{
个个方法同上
}
class test
{
static void Main(string[] args)
{
Mod mod = new Mod();
mod.defaultMethod();
mod.publicMethod();
mod.privateMethod();
mod.internalMethod();
mod.protectedMethod();
mod.protectedInternalMethod();
}
}
}
编译结果出现错误
<?xml:namespace prefix = v ns = "urn:schemas-microsoft-com:vml" />
可见被
default private protected
修饰的方法在同一程序集是不可以访问的:但我们把些方法注释掉后运行结果:
我是用public修饰的方法
我是用internal修饰的方法
我是用protected internal修饰的方法
请按任意键继续. . .
可见:被public internal protected interna修饰的方法在同一程序集是可以被访问的
现在看看在子类中代码做如下修改添加一个子类,在子类方法中调用父类方法
using
System;
namespace
testclassmodifier
{
class Mod{ }
class ModChild : Mod
{
public void RunMod()
{
defaultMethod();
publicMethod();
privateMethod();
internalMethod();
protectedMethod();
protectedInternalMethod();
}}
class test
{
static void Main(string[] args)
{
ModChild modc = new ModChild();
modc.RunMod();
}}}
编译出错结果:
可见:被
default
private
修饰的方法在子类中是不可以被访问的。当我们把些方法注释掉后运行结果:
我是用public修饰的方法
我是用internal修饰的方法
我是用protected修饰的方法
我是用protected internal修饰的方法
请按任意键继续. . .
可见:被
其他方法
修饰的方法在子类中是可以被访问的
现在看看在程序集外边 如何做:添加类库文件 将类class Mod生成dll文件注意命名空间要于相同 添加dll文件引用。代码如下:
编译结果:可见只有public修饰的方法可以被访问
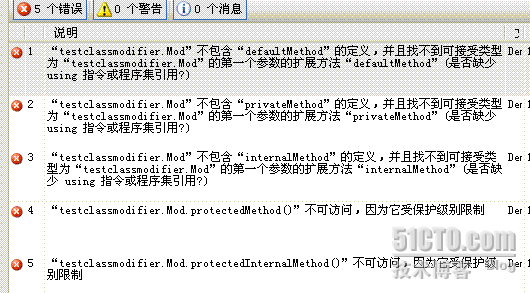
访问控制修饰符
|
类内部
|
子类
|
程序集内
|
程序集外
|
Default
|
可以
|
|
|
|
Public
|
可以
|
可以
|
可以
|
可以
|
Private
|
可以
|
|
|
|
Internal
|
可以
|
可以
|
可以
|
|
Protected
|
可以
|
可以
|
|
|
Protected internal
|
可以
|
可以
|
可以
|
|
我们会注意到Internal Protected internal 一样有何区别?
当父类和子类在一个程序集的时候internal成员为可见的,当父类和子类不在一个程序集的时候子类不能访问父类成员,子类可以访问父类Protected internal成员
namespace
testclassmodifier
{
class Demo
{
class ModChild : Mod
{
public void RunMod()
{
//internalMethod();
不可访问,不可见
protectedInternalMethod();
}
}
static void Main(string[] args)
{
ModChild modc = new ModChild();
modc.RunMod();
}}}
使用Sealed修饰符的类不能在被继承
Partial
修饰符可以把一个类放在不同的文件中
转载于:https://blog.51cto.com/baixl/358546