网络性能信息,乍一看,好像很复杂的样子,本来还想走《主机性能监控系统--6.获取磁盘性能信息》的简单路线,不过看到“”同学在《主机性能监控系统--6.获取磁盘性能信息》发表的评论,觉得也是,性能信息和基本信息是有差别的,所以,在网络性能信息这个小程序里多了很多跟网络性能有关的信息,OK,废话不多说,先看效果图
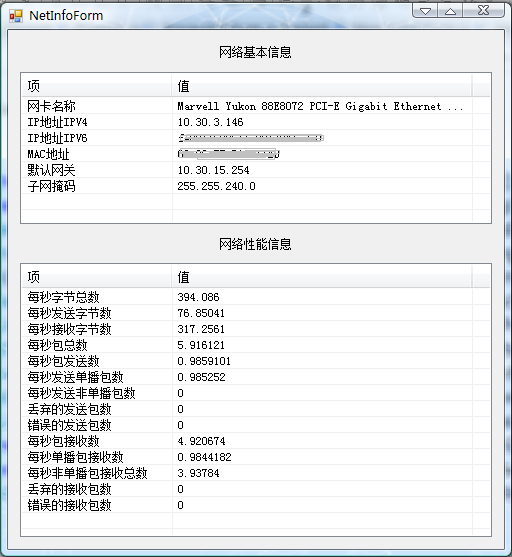
在这个小程序中获取网络性能信息的方法是利用PerformanceCounter 这个类,这个类所获取的数据跟Windows自带的性能监视器所展示的数据很相近,所以应该还算是准确吧,哈哈,不过找不到那些英文单词比较准确的中文资料,所以,就自己蛮翻译了一下了。
OK,开始上代码,同样的,新建一个类,命名为:NetInfo,类图和代码如下:
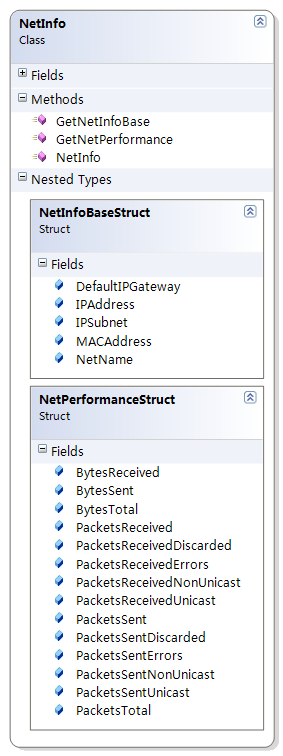
NetInfo
public class NetInfo

{
NetPerformanceStruct netPerformanceStruct;


定义PerformanceCounter#region 定义PerformanceCounter
PerformanceCounter pcBytesTotal = new PerformanceCounter();
PerformanceCounter pcBytesSent = new PerformanceCounter();
PerformanceCounter pcBytesReceived = new PerformanceCounter();
PerformanceCounter pcPacketsTotal = new PerformanceCounter();
PerformanceCounter pcPacketsSent = new PerformanceCounter();
PerformanceCounter pcPacketsSentUnicast = new PerformanceCounter();
PerformanceCounter pcPacketsSentNonUnicast = new PerformanceCounter();
PerformanceCounter pcPacketsSentDiscarded = new PerformanceCounter();
PerformanceCounter pcPacketsSentErrors = new PerformanceCounter();
PerformanceCounter pcPacketsReceived = new PerformanceCounter();
PerformanceCounter pcPacketsReceivedUnicast = new PerformanceCounter();
PerformanceCounter pcPacketsReceivedNonUnicast = new PerformanceCounter();
PerformanceCounter pcPacketsReceivedDiscarded = new PerformanceCounter();
PerformanceCounter pcPacketsReceivedErrors = new PerformanceCounter();
#endregion


构造函数#region 构造函数
public NetInfo()

{

}
#endregion


网络基础信息结构体#region 网络基础信息结构体
public struct NetInfoBaseStruct

{
public string NetName; //网络名称
public string[] IPAddress; //IP地址,包括IPv4和IPv6
public string MACAddress; //MAC地址
public string IPSubnet; //子网掩码
public string DefaultIPGateway; //默认网关
}
#endregion


网络性能结构体#region 网络性能结构体
public struct NetPerformanceStruct

{

字节#region 字节
public float BytesTotal; //每秒总字节数
public float BytesSent; //每秒发送字节数
public float BytesReceived; //每秒发送字节数
#endregion


包#region 包
public float PacketsTotal; //每秒总包数


包发送#region 包发送
public float PacketsSent; //每秒发送包数
public float PacketsSentUnicast; //每秒发送单播包数
public float PacketsSentNonUnicast; //每秒发送非单播包数
public float PacketsSentDiscarded; //被丢弃的发送包数
public float PacketsSentErrors; //错误的发送包数
#endregion


包接收#region 包接收
public float PacketsReceived; //每秒接收包数
public float PacketsReceivedUnicast; //每秒接收单播包数
public float PacketsReceivedNonUnicast; //每秒接收非单播包数
public float PacketsReceivedDiscarded; //被丢弃的接收包数
public float PacketsReceivedErrors; //错误的接收包数
#endregion
#endregion

}
#endregion


获取网络基本信息#region 获取网络基本信息

/**//// <summary>
/// 获取网络基本信息
/// </summary>
/// <returns>包含网络基本信息结构体的列表</returns>
public List<NetInfoBaseStruct> GetNetInfoBase()

{
List<NetInfoBaseStruct> lNetInfo = new List<NetInfoBaseStruct>();
NetInfoBaseStruct netInfoBaseStruct;


ManagementObjectSearcher query =
new ManagementObjectSearcher("SELECT * FROM Win32_NetworkAdapterConfiguration WHERE IPEnabled = 'TRUE'");
ManagementObjectCollection moCollection = query.Get();

//遍历
foreach (ManagementObject mObject in moCollection)

{
try

{
netInfoBaseStruct = new NetInfoBaseStruct();
netInfoBaseStruct.NetName = mObject["Description"].ToString();
netInfoBaseStruct.IPAddress = (string[])mObject["IPAddress"];
netInfoBaseStruct.MACAddress = mObject["MACAddress"].ToString();

DefaultIPGateway#region DefaultIPGateway
if (mObject["DefaultIPGateway"] == null) //如果默认网关只有一个则返回

{
netInfoBaseStruct.DefaultIPGateway = mObject["DefaultIPGateway"].ToString();
}
else //否则返回默认网关的数组(注:这里为了简单起见,只返回第一个网关)

{
netInfoBaseStruct.DefaultIPGateway = ((string[])mObject["DefaultIPGateway"])[0];
}
#endregion

IPSubnet#region IPSubnet
if (mObject["IPSubnet"] == null)

{
netInfoBaseStruct.IPSubnet = mObject["IPSubnet"].ToString();
}
else

{
netInfoBaseStruct.IPSubnet = ((string[])mObject["IPSubnet"])[0];
}
#endregion
lNetInfo.Add(netInfoBaseStruct);
}
catch

{
continue;
}

}

return lNetInfo;
}
#endregion


GetNetPerformance#region GetNetPerformance
public NetPerformanceStruct GetNetPerformance(string NetName)

{
netPerformanceStruct = new NetPerformanceStruct();


定义CategoryName#region 定义CategoryName
pcBytesTotal.CategoryName = "Network Interface";
pcBytesSent.CategoryName = "Network Interface";
pcBytesReceived.CategoryName = "Network Interface";
pcPacketsTotal.CategoryName = "Network Interface";
pcPacketsSent.CategoryName = "Network Interface";
pcPacketsSentUnicast.CategoryName = "Network Interface";
pcPacketsSentNonUnicast.CategoryName = "Network Interface";
pcPacketsSentDiscarded.CategoryName = "Network Interface";
pcPacketsSentErrors.CategoryName = "Network Interface";
pcPacketsReceived.CategoryName = "Network Interface";
pcPacketsReceivedUnicast.CategoryName = "Network Interface";
pcPacketsReceivedNonUnicast.CategoryName = "Network Interface";
pcPacketsReceivedDiscarded.CategoryName = "Network Interface";
pcPacketsReceivedErrors.CategoryName = "Network Interface";
#endregion


定义InstanceName#region 定义InstanceName
pcBytesTotal.InstanceName = NetName;
pcBytesSent.InstanceName = NetName;
pcBytesReceived.InstanceName = NetName;
pcPacketsTotal.InstanceName = NetName;
pcPacketsSent.InstanceName = NetName;
pcPacketsSentUnicast.InstanceName = NetName;
pcPacketsSentNonUnicast.InstanceName = NetName;
pcPacketsSentDiscarded.InstanceName = NetName;
pcPacketsSentErrors.InstanceName = NetName;
pcPacketsReceived.InstanceName = NetName;
pcPacketsReceivedUnicast.InstanceName = NetName;
pcPacketsReceivedNonUnicast.InstanceName = NetName;
pcPacketsReceivedDiscarded.InstanceName = NetName;
pcPacketsReceivedErrors.InstanceName = NetName;
#endregion


定义CounterName#region 定义CounterName
pcBytesTotal.CounterName = "Bytes Total/sec";
pcBytesSent.CounterName = "Bytes Sent/sec";
pcBytesReceived.CounterName = "Bytes Received/sec";
pcPacketsTotal.CounterName = "Packets/sec";
pcPacketsSent.CounterName = "Packets Sent/sec";
pcPacketsSentUnicast.CounterName = "Packets Sent Unicast/sec";
pcPacketsSentNonUnicast.CounterName = "Packets Sent Non-Unicast/sec";
pcPacketsSentDiscarded.CounterName = "Packets Outbound Discarded";
pcPacketsSentErrors.CounterName = "Packets Outbound Errors";
pcPacketsReceived.CounterName = "Packets Received/sec";
pcPacketsReceivedUnicast.CounterName = "Packets Received Unicast/sec";
pcPacketsReceivedNonUnicast.CounterName = "Packets Received Non-Unicast/sec";
pcPacketsReceivedDiscarded.CounterName = "Packets Received Discarded";
pcPacketsReceivedErrors.CounterName = "Packets Received Errors";
#endregion


为结构体赋值#region 为结构体赋值
netPerformanceStruct.BytesTotal = pcBytesTotal.NextValue();
netPerformanceStruct.BytesSent = pcBytesSent.NextValue();
netPerformanceStruct.BytesReceived = pcBytesReceived.NextValue();
netPerformanceStruct.PacketsTotal = pcPacketsTotal.NextValue();
netPerformanceStruct.PacketsSent = pcPacketsSent.NextValue();
netPerformanceStruct.PacketsSentUnicast = pcPacketsSentUnicast.NextValue();
netPerformanceStruct.PacketsSentNonUnicast = pcPacketsSentNonUnicast.NextValue();
netPerformanceStruct.PacketsSentDiscarded = pcPacketsSentDiscarded.NextValue();
netPerformanceStruct.PacketsSentErrors = pcPacketsSentErrors.NextValue();
netPerformanceStruct.PacketsReceived = pcPacketsReceived.NextValue();
netPerformanceStruct.PacketsReceivedUnicast = pcPacketsReceivedUnicast.NextValue();
netPerformanceStruct.PacketsReceivedNonUnicast = pcPacketsReceivedNonUnicast.NextValue();
netPerformanceStruct.PacketsReceivedDiscarded = pcPacketsReceivedDiscarded.NextValue();
netPerformanceStruct.PacketsReceivedErrors = pcPacketsReceivedErrors.NextValue();
#endregion

return netPerformanceStruct;
}
#endregion
}
新建一个窗体,命名为:NetInfoForm,窗体控件布局如效果图所示,代码如下:
NetInfo Form
public partial class NetInfoForm : Form

{
public NetInfoForm()

{
InitializeComponent();
}
ComputerInfo.NetInfo netinfo = new ComputerInfo.NetInfo();
string strNetName;
PerformanceCounter pc;
ComputerInfo.NetInfo.NetPerformanceStruct netInfoStruct;
private void NetInfoForm_Load(object sender, EventArgs e)

{

获取网络基本信息#region 获取网络基本信息

List<ComputerInfo.NetInfo.NetInfoBaseStruct> lNetInfoBase = netinfo.GetNetInfoBase();

设置ListView的样式以及列#region 设置ListView的样式以及列
lvNet.View = View.Details;
lvNet.Columns.Add("项", 150);
lvNet.Columns.Add("值", 300);
lvNet.FullRowSelect = true;
lvNet.GridLines = true;
#endregion

strNetName = lNetInfoBase[0].NetName;


定义一些需要用到的项#region 定义一些需要用到的项

ListViewItem lviNetDes = new ListViewItem(new string[]
{ "网卡名称", lNetInfoBase[0].NetName });

ListViewItem lviIPAddressV4 = new ListViewItem(new string[]
{ "IP地址IPV4", lNetInfoBase[0].IPAddress[0] });

ListViewItem lviIPAddressV6 = new ListViewItem(new string[]
{ "IP地址IPV6", lNetInfoBase[0].IPAddress[1] });

ListViewItem lviMACAddress = new ListViewItem(new string[]
{ "MAC地址", lNetInfoBase[0].MACAddress });

ListViewItem lviDefaultIPGateway = new ListViewItem(new string[]
{ "默认网关", lNetInfoBase[0].DefaultIPGateway });

ListViewItem lviIPSubnet = new ListViewItem(new string[]
{ "子网掩码", lNetInfoBase[0].IPSubnet });
#endregion


将项添加到ListView控件里面#region 将项添加到ListView控件里面
lvNet.Items.Add(lviNetDes);
lvNet.Items.Add(lviIPAddressV4);
lvNet.Items.Add(lviIPAddressV6);
lvNet.Items.Add(lviMACAddress);
lvNet.Items.Add(lviDefaultIPGateway);
lvNet.Items.Add(lviIPSubnet);
#endregion
#endregion


设置ListView的样式以及列#region 设置ListView的样式以及列
lvNetPerformance.View = View.Details;
lvNetPerformance.Columns.Add("项", 150);
lvNetPerformance.Columns.Add("值", 300);
lvNetPerformance.FullRowSelect = true;
lvNetPerformance.GridLines = true;
#endregion

}

private void NetInfotimer_Tick(object sender, EventArgs e)

{

netInfoStruct = netinfo.GetNetPerformance(strNetName);
lvNetPerformance.Items.Clear();

定义一些需要用到的项#region 定义一些需要用到的项

ListViewItem lviBytesTotal = new ListViewItem(new string[]
{ "每秒字节总数", netInfoStruct.BytesTotal.ToString() });

ListViewItem lviBytesSent = new ListViewItem(new string[]
{ "每秒发送字节数", netInfoStruct.BytesSent.ToString() });

ListViewItem lviBytesReceived = new ListViewItem(new string[]
{ "每秒接收字节数", netInfoStruct.BytesReceived.ToString() });

ListViewItem lviPacketsTotal = new ListViewItem(new string[]
{ "每秒包总数", netInfoStruct.PacketsTotal.ToString() });

ListViewItem lviPacketsSent = new ListViewItem(new string[]
{ "每秒包发送数", netInfoStruct.PacketsSent.ToString() });

ListViewItem lviPacketsSentUnicast = new ListViewItem(new string[]
{ "每秒发送单播包数", netInfoStruct.PacketsSentUnicast.ToString() });

ListViewItem lviPacketsSentNonUnicast = new ListViewItem(new string[]
{ "每秒发送非单播包数", netInfoStruct.PacketsSentNonUnicast.ToString() });

ListViewItem lviPacketsSentDiscarded = new ListViewItem(new string[]
{ "丢弃的发送包数", netInfoStruct.PacketsSentDiscarded.ToString() });

ListViewItem lviPacketsSentErrors = new ListViewItem(new string[]
{ "错误的发送包数", netInfoStruct.PacketsSentErrors.ToString() });

ListViewItem lviPacketsReceived = new ListViewItem(new string[]
{ "每秒包接收数", netInfoStruct.PacketsReceived.ToString() });

ListViewItem lviPacketsReceivedUnicast = new ListViewItem(new string[]
{ "每秒单播包接收数", netInfoStruct.PacketsReceivedUnicast.ToString() });

ListViewItem lviPacketsReceivedNonUnicast = new ListViewItem(new string[]
{ "每秒非单播包接收总数", netInfoStruct.PacketsReceivedNonUnicast.ToString() });

ListViewItem lviPacketsReceivedDiscarded = new ListViewItem(new string[]
{ "丢弃的接收包数", netInfoStruct.PacketsReceivedDiscarded.ToString() });

ListViewItem lviPacketsReceivedErrors = new ListViewItem(new string[]
{ "错误的接收包数", netInfoStruct.PacketsReceivedErrors.ToString() });
#endregion


将项添加到ListView控件里面#region 将项添加到ListView控件里面
lvNetPerformance.Items.Add(lviBytesTotal);
lvNetPerformance.Items.Add(lviBytesSent);
lvNetPerformance.Items.Add(lviBytesReceived);
lvNetPerformance.Items.Add(lviPacketsTotal);
lvNetPerformance.Items.Add(lviPacketsSent);
lvNetPerformance.Items.Add(lviPacketsSentUnicast);
lvNetPerformance.Items.Add(lviPacketsSentNonUnicast);
lvNetPerformance.Items.Add(lviPacketsSentDiscarded);
lvNetPerformance.Items.Add(lviPacketsSentErrors);
lvNetPerformance.Items.Add(lviPacketsReceived);
lvNetPerformance.Items.Add(lviPacketsReceivedUnicast);
lvNetPerformance.Items.Add(lviPacketsReceivedNonUnicast);
lvNetPerformance.Items.Add(lviPacketsReceivedDiscarded);
lvNetPerformance.Items.Add(lviPacketsReceivedErrors);
#endregion



}
}
OK,这样就把网络性能信息这个小程序做完了,由于在功能和技术方面上都没有什么大的难点,在此就不做过多的说明了,有不懂的同学,可以留言,至于“哎呀呀”同学在《主机性能监控系统--6.获取磁盘性能信息》提到获取的信息太简单,太少的问题,我会在后期整合的时候考虑把磁盘的一些性能信息加进去的,在此感谢“哎呀呀”以及其他提出建议或意见的同学,OK,收工~~
未完,待续~~