一、 工厂方法(Factory Method)模式
工厂方法(FactoryMethod)模式是类的创建模式,其用意是定义一个创建产品对象的工厂接口,将实际创建工作推迟到子类中。在工厂方法模式中,核心的工厂类不再负责所有产品的创建,而是将具体创建工作交给子类去做。这个核心类仅仅负责给出具体工厂必须实现的接口,而不接触哪一个产品类被实例化这种细节。这使得工厂方法模式可以允许系统在不修改工厂角色的情况下引进新产品。在Factory Method模式中,工厂类与产品类往往具有平行的等级结构,它们之间一一对应。
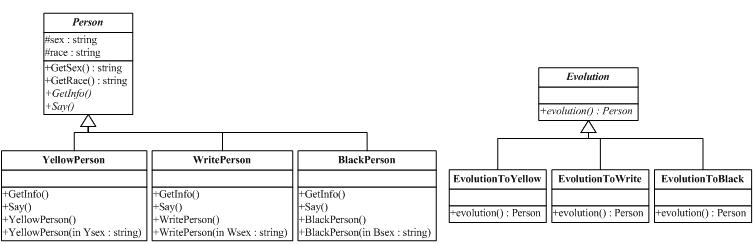
抽象工厂(Creator)角色:是工厂方法模式的核心,与应用程序无关。任何在模式中创建的对象的工厂类必须实现这个接口,这里为Evolution抽象类。
具体工厂(Concrete Creator)角色:这是实现抽象工厂接口的具体工厂类,包含与应用程序密切相关的逻辑,并且受到应用程序调用以创建产品对象。这里为EvolutionToYellow,EvolutionToWirte,EvolutionToBlack三个类。
抽象产品(Product)角色:工厂方法模式所创建的对象的超类型,也就是产品对象的共同父类或共同拥有的接口。这里为Person抽象类。
具体产品(Concrete Product)角色:这个角色实现了抽象产品角色所定义的接口。某具体产品有专门的具体工厂创建,它们之间往往一一对应,这里为YellowPerson,WritePerson,BlackPerson 三个类。
具体代码(C#):
1
using
System;
2

3
namespace
Factory_Method
4

{
5
/**//**//**//// <summary>
6
///============== Program Description==============
7
///Name:FactoryMethod.cs
8
///Objective:FactoryMethod
9
///Date:2006-02-09
10
///Written By coffee.liu
11
///================================================
12
/// </summary>
13
class Class1
14
{
15
/**//**//**//// <summary>
16
/// 应用程序的主入口点。
17
/// </summary>
18
[STAThread]
19
static void Main(string[] args)
20
{
21
Evolution yev=new EvolutionToYellow();
22
Evolution bev=new EvolutionToBlack();
23
Evolution wev=new EvolutionToWrite();
24
Person yp=yev.evolution();
25
yp.GetInfo();
26
yp.Say();
27
Console.WriteLine(".");
28
Person bp=bev.evolution();
29
bp.GetInfo();
30
bp.Say();
31
Console.WriteLine(".");
32
Person wp=wev.evolution();
33
wp.GetInfo();
34
wp.Say();
35
}
36
}
37
public abstract class Person
38
{
39
protected string sex,race;
40
public string GetSex()
41
{
42
return sex;
43
}
44
public string GetRace()
45
{
46
return race;
47
}
48
public abstract void GetInfo();
49
public abstract void Say();
50
}
51
52
public class YellowPerson:Person
53
{
54
public YellowPerson()
55
{
56
sex="Man";
57
race="Yellow";
58
}
59
public YellowPerson(string Ysex)
60
{
61
sex=Ysex;
62
race="Yellow";
63
}
64
public override void GetInfo()
65
{
66
Console.WriteLine("the "+race+" Person Info:"+sex);
67
}
68
public override void Say()
69
{
70
Console.WriteLine("I am a Yellow Baby");
71
}
72
73
}
74
75
public class BlackPerson:Person
76
{
77
public BlackPerson()
78
{
79
sex="Man";
80
race="Black";
81
}
82
public BlackPerson(string Bsex)
83
{
84
sex=Bsex;
85
race="Black";
86
}
87
public override void GetInfo()
88
{
89
Console.WriteLine("the "+race+" Person Info:"+sex);
90
}
91
public override void Say()
92
{
93
Console.WriteLine("I am a Black Baby");
94
}
95
}
96
97
public class WritePerson:Person
98
{
99
public WritePerson()
100
{
101
sex="Man";
102
race="Write";
103
}
104
public WritePerson(string Wsex)
105
{
106
sex=Wsex;
107
race="Write";
108
}
109
public override void GetInfo()
110
{
111
Console.WriteLine("the "+race+" Person Info:"+sex);
112
}
113
public override void Say()
114
{
115
Console.WriteLine("I am a Write Baby");
116
}
117
}
118
public abstract class Evolution
{
119
public abstract Person evolution();
120
}
121
public class EvolutionToYellow:Evolution
122
{
123
public override Person evolution()
124
{
125
return new YellowPerson();
126
}
127
128
}
129
public class EvolutionToBlack:Evolution
130
{
131
public override Person evolution()
132
{
133
return new BlackPerson();
134
}
135
}
136
public class EvolutionToWrite:Evolution
137
{
138
public override Person evolution()
139
{
140
return new WritePerson();
141
}
142
}
143
144
}
145
程序代码(Pascal)
1
program FactoryMethod;
2
//==============
Program Description
==============
3
//
Name:FactoryMethod.dpr
4
//
Objective:FactoryMethod
5
//
Date
:
2006
-
04
-
23
6
//
Written By coffee.liu
7
//================================================
8
{$APPTYPE CONSOLE}
9

10
uses
11
SysUtils;
12

type Person
=
Class protectedclass
Class protectedclass
13
protected
14
sex:string;
15
race:string;
16
public Function GetSex()Function GetSex()Function GetSex()function GetSex():string;
17
public Function GetRace()Function GetRace()Function GetRace()function GetRace():string;
18
public procedure GetInfo;virtual;abstract;
19
public procedure say;virtual;abstract;
20
21
end;
22
type YellowPerson=Class (Class (Class (class(Person)
23
constructor YellowPerson();
24
public procedure GetInfo;override;
25
public procedure Say;override;
26
end;
27
type BlackPerson=Class (Class (Class (class(Person)
28
constructor BlackPerson();
29
public procedure GetInfo;override;
30
public procedure Say;override;
31
end;
32
type WritePerson=Class (Class (Class (class(Person)
33
constructor WritePerson();
34
public procedure GetInfo;override;
35
public procedure Say;override;
36
end;
37
type Evolution=Class publicclassClass publicclass
38
public Function evolution()Function evolution()Function evolution()function evolution():Person;virtual;abstract;
39
end;
40
type EvolutionToYellow=Class (Class (Class (class(Evolution)
41
constructor EvolutionToYellow();
42
public Function evolution()Function evolution()Function evolution()function evolution():Person;override;
43
end;
44
type EvolutionToBlack=Class (Class (Class (class(Evolution)
45
constructor EvolutionToBlack();
46
public Function evolution()Function evolution()Function evolution()function evolution():Person;override;
47
end;
48
type EvolutionToWrite=Class (Class (Class (class(Evolution)
49
constructor EvolutionToWrite();
50
public Function evolution()Function evolution()Function evolution()function evolution():Person;override;
51
end;
52
{ Person }
53
54
Function Person()Function Person()Function Person()function Person.GetRace: string;
55
begin
56
result:=race;
57
end;
58
59
Function Person()Function Person()Function Person()function Person.GetSex: string;
60
begin
61
result:=sex;
62
end;
63
64
{ YellowPerson }
65
66
constructor YellowPerson.YellowPerson;
67
begin
68
sex:='Man';
69
race:='Yellow';
70
end;
71
procedure YellowPerson.GetInfo;
72
begin
73
inherited;
74
WriteLn('the '+race+' Person Info:'+sex);
75
end;
76
procedure YellowPerson.Say;
77
begin
78
inherited;
79
WriteLn('I am a Yellow Baby');
80
end;
81
82
{ WritePerson }
83
84
procedure WritePerson.GetInfo;
85
begin
86
inherited;
87
WriteLn('the '+race+' Person Info:'+sex);
88
end;
89
90
procedure WritePerson.Say;
91
begin
92
inherited;
93
WriteLn('I am a Write Baby');
94
end;
95
96
constructor WritePerson.WritePerson;
97
begin
98
sex:='Man';
99
race:='Write';
100
end;
101
102
{ BlackPerson }
103
104
constructor BlackPerson.BlackPerson;
105
begin
106
sex:='Man';
107
race:='Black';
108
end;
109
110
procedure BlackPerson.GetInfo;
111
begin
112
inherited;
113
WriteLn('the '+race+' Person Info:'+sex);
114
end;
115
116
procedure BlackPerson.Say;
117
begin
118
inherited;
119
WriteLn('I am a Black Baby');
120
end;
121
122
{ EvolutionToBlack }
123
124
Function EvolutionToBlack()Function EvolutionToBlack()Function EvolutionToBlack()function EvolutionToBlack.evolution: Person;
125
begin
126
result:=BlackPerson.BlackPerson;
127
end;
128
129
constructor EvolutionToBlack.EvolutionToBlack;
130
begin
131
132
end;
133
134
{ EvolutionToWrite }
135
136
Function EvolutionToWrite()Function EvolutionToWrite()Function EvolutionToWrite()function EvolutionToWrite.evolution: Person;
137
begin
138
result:=WritePerson.WritePerson;
139
end;
140
141
constructor EvolutionToWrite.EvolutionToWrite;
142
begin
143
144
end;
145
146
{ EvolutionToYellow }
147
148
Function EvolutionToYellow()Function EvolutionToYellow()Function EvolutionToYellow()function EvolutionToYellow.evolution: Person;
149
begin
150
result:=YellowPerson.YellowPerson;
151
end;
152
153
154
155
constructor EvolutionToYellow.EvolutionToYellow;
156
begin
157
158
end;
159
var
160
yev,wev,bev:Evolution;
161
yp,wp,bp:Person;
162
begin
163
yev:=EvolutionToYellow.EvolutionToYellow;
164
yp:=yev.evolution;
165
wev:=EvolutionToWrite.EvolutionToWrite;
166
wp:=wev.evolution;
167
bev:=EvolutionToBlack.EvolutionToBlack;
168
bp:=bev.evolution;
169
yp.GetInfo;
170
yp.say;
171
WriteLn('..');
172
wp.GetInfo;
173
wp.say;
174
WriteLn('..');
175
bp.GetInfo;
176
bp.say;
177
178
end.
179
何时使用工厂方法:
1.一个类无法预测它要创建的对象属于哪个类。
2.一个类用他的子类来指定所创建的对象。
3.把要创建哪个类的信息局部化的时候。
对于实现工厂模式需要注意的问题:
1.基类是一个抽象类或一个接口,模式必须返回一个完整的可工作的类。
2.基类包含默认方法,除非默认方法不能胜任,才会调用这些方法。
3.可以将参数传递给工厂,告诉工厂返回哪个类型的类。这种情况下,类可以共享相同的方法名,但完成的工作可以不同。
我们甚至可以将上面的代码变得更复杂一点:
代码如下:
1
using
System;
2
using
System.Collections;
3

4
namespace
FactoryMethodPlus2
5

{
6
/**//**//**//// <summary>
7
///============== Program Description==============
8
///Name:FactoryMethodPlus2.cs
9
///Objective:FactoryMethodPlus2
10
///Date:2006-04-23
11
///Written By coffee.liu
12
///================================================
13
/// </summary>
14
class Class1
15
{
16
/**//**//**//// <summary>
17
/// 应用程序的主入口点。
18
/// </summary>
19
[STAThread]
20
static void Main(string[] args)
21
{
22
Evolution[] evs=new Evolution[5];
23
evs[0]=new EvolutionToYellow();
24
evs[1]=new EvolutionToBlack();
25
evs[2]=new EvolutionToWrite();
26
evs[3]=new EolutionToAsia();
27
evs[4]=new EolutionToNorthAmerica();
28
foreach(Evolution ev in evs )
{
29
Console.WriteLine("..");
30
foreach(Person ps in ev.Persons)
{
31
//ps.GetInfo();
32
ps.Say();
33
}
34
}
35
36
}
37
}
38
public abstract class Person
39
{
40
protected string sex,race;
41
public string GetSex()
42
{
43
return sex;
44
}
45
public string GetRace()
46
{
47
return race;
48
}
49
public abstract void GetInfo();
50
public abstract void Say();
51
}
52
53
public class YellowPerson:Person
54
{
55
public YellowPerson()
56
{
57
sex="Man";
58
race="Yellow";
59
}
60
public YellowPerson(string Ysex)
61
{
62
sex=Ysex;
63
race="Yellow";
64
}
65
public override void GetInfo()
66
{
67
Console.WriteLine("the "+race+" Person Info:"+sex);
68
}
69
public override void Say()
70
{
71
Console.WriteLine("I am a Yellow Baby");
72
}
73
74
}
75
/**//**//**//// <summary>
76
/// 黄种人分布
77
/// </summary>
78
public class AsiaYellowPerson:YellowPerson
79
{
80
private string state="Asia";
81
public string State
{
82
get
{return state;}
83
}
84
public AsiaYellowPerson():base()
85
{}
86
public AsiaYellowPerson(string Ysex):base(Ysex)
87
{}
88
public override void Say()
89
{
90
Console.WriteLine("I am a Yellow Baby ,My state in Asia");
91
}
92
}
93
94
public class NorthAmericaYellowPerson:YellowPerson
95
{
96
private string state="NorthAmerica";
97
public string State
98
{
99
get
{return state;}
100
}
101
public NorthAmericaYellowPerson():base()
102
{}
103
public NorthAmericaYellowPerson(string Ysex):base(Ysex)
104
{}
105
public override void Say()
106
{
107
Console.WriteLine("I am a Yellow Baby ,My state in NorthAmerica");
108
}
109
}
110
public class SouthAmericaYellowPerson:YellowPerson
111
{
112
private string state="SouthAmerica";
113
public string State
114
{
115
get
{return state;}
116
}
117
public SouthAmericaYellowPerson():base()
118
{}
119
public SouthAmericaYellowPerson(string Ysex):base(Ysex)
120
{}
121
public override void Say()
122
{
123
Console.WriteLine("I am a Yellow Baby ,My state in SouthAmerica");
124
}
125
}
126
public class AustrialiaYellowPerson:YellowPerson
127
{
128
private string state="Austrialia";
129
public string State
130
{
131
get
{return state;}
132
}
133
public AustrialiaYellowPerson():base()
134
{}
135
public AustrialiaYellowPerson(string Ysex):base(Ysex)
136
{}
137
public override void Say()
138
{
139
Console.WriteLine("I am a Yellow Baby ,My state in Austrialia");
140
}
141
}
142
143
public class BlackPerson:Person
144
{
145
public BlackPerson()
146
{
147
sex="Man";
148
race="Black";
149
}
150
public BlackPerson(string Bsex)
151
{
152
sex=Bsex;
153
race="Black";
154
}
155
public override void GetInfo()
156
{
157
Console.WriteLine("the "+race+" Person Info:"+sex);
158
}
159
public override void Say()
160
{
161
Console.WriteLine("I am a Black Baby");
162
}
163
}
164
/**//**//**//// <summary>
165
/// 黑人分布
166
/// </summary>
167
public class EuropeBlackPerson:BlackPerson
168
{
169
private string state="Europe";
170
public string State
171
{
172
get
{return state;}
173
}
174
public EuropeBlackPerson():base()
175
{}
176
public EuropeBlackPerson(string Ysex):base(Ysex)
177
{}
178
public override void Say()
179
{
180
Console.WriteLine("I am a Black Baby ,My state in Europe");
181
}
182
}
183
public class AfricaBlackPerson:BlackPerson
184
{
185
private string state="Africa";
186
public string State
187
{
188
get
{return state;}
189
}
190
public AfricaBlackPerson():base()
191
{}
192
public AfricaBlackPerson(string Ysex):base(Ysex)
193
{}
194
public override void Say()
195
{
196
Console.WriteLine("I am a Black Baby ,My state in Africa");
197
}
198
}
199
200
201
public class WritePerson:Person
202
{
203
public WritePerson()
204
{
205
sex="Man";
206
race="Write";
207
}
208
public WritePerson(string Wsex)
209
{
210
sex=Wsex;
211
race="Write";
212
}
213
public override void GetInfo()
214
{
215
Console.WriteLine("the "+race+" Person Info:"+sex);
216
}
217
public override void Say()
218
{
219
Console.WriteLine("I am a Write Baby");
220
}
221
}
222
/**//**//**//// <summary>
223
///白人分布
224
/// </summary>
225
226
public class EuropeWritePerson:WritePerson
227
{
228
private string state="Europe";
229
public string State
230
{
231
get
{return state;}
232
}
233
public EuropeWritePerson():base()
234
{}
235
public EuropeWritePerson(string Ysex):base(Ysex)
236
{}
237
public override void Say()
238
{
239
Console.WriteLine("I am a Write Baby ,My state in Europe");
240
}
241
}
242
public class NorthAmericaWritePerson:WritePerson
243
{
244
private string state="NorthAmerica";
245
public string State
246
{
247
get
{return state;}
248
}
249
public NorthAmericaWritePerson():base()
250
{}
251
public NorthAmericaWritePerson(string Ysex):base(Ysex)
252
{}
253
public override void Say()
254
{
255
Console.WriteLine("I am a Write Baby ,My state in NorthAmerica");
256
}
257
}
258
public class SouthAmericaWritePerson:WritePerson
259
{
260
private string state="SouthAmerica";
261
public string State
262
{
263
get
{return state;}
264
}
265
public SouthAmericaWritePerson():base()
266
{}
267
public SouthAmericaWritePerson(string Ysex):base(Ysex)
268
{}
269
public override void Say()
270
{
271
Console.WriteLine("I am a Write Baby ,My state in SouthAmerica");
272
}
273
}
274
public class OrangutanWritePerson:WritePerson
275
{
276
private string state="Orangutan";
277
public string State
278
{
279
get
{return state;}
280
}
281
public OrangutanWritePerson():base()
282
{}
283
public OrangutanWritePerson(string Ysex):base(Ysex)
284
{}
285
public override void Say()
286
{
287
Console.WriteLine("I am a . Baby ,I am a Orangutan,I like banananananana..");
288
}
289
}
290
291
/**//**//**//// <summary>
292
/// 进化
293
/// </summary>
294
public abstract class Evolution
295
{
296
public Evolution()
{
297
this.evolution();
298
}
299
protected ArrayList persons=new ArrayList();
300
public abstract void evolution();
301
public ArrayList Persons
302
{
303
get
{return persons;}
304
}
305
}
306
public class EvolutionToYellow:Evolution
307
{
308
309
public override void evolution()
310
{
311
persons.Add(new AsiaYellowPerson());
312
persons.Add(new NorthAmericaYellowPerson());
313
persons.Add(new SouthAmericaYellowPerson());
314
persons.Add(new AustrialiaYellowPerson());
315
}
316
}
317
public class EvolutionToBlack:Evolution
318
{
319
320
public override void evolution()
321
{
322
persons.Add(new EuropeBlackPerson());
323
persons.Add(new AfricaBlackPerson());
324
}
325
}
326
public class EvolutionToWrite:Evolution
327
{
328
public override void evolution()
329
{
330
persons.Add(new EuropeWritePerson());
331
persons.Add(new NorthAmericaWritePerson());
332
persons.Add(new SouthAmericaWritePerson());
333
persons.Add(new OrangutanWritePerson());
334
}
335
}
336
public class EolutionToAsia:Evolution
{
337
public override void evolution()
{
338
persons.Add(new AsiaYellowPerson());
339
}
340
}
341
public class EolutionToNorthAmerica:Evolution
342
{
343
public override void evolution()
344
{
345
persons.Add(new NorthAmericaYellowPerson());
346
persons.Add(new NorthAmericaWritePerson());
347
}
348
}
349
}
350
程序代码(Pascal):
1
program FactoryMethodPlus1;
2
//==============
Program Description
==============
3
//
Name:FactoryMethodPlus1.dpr
4
//
Objective:FactoryMethodPlus1
5
//
Date
:
2006
-
04
-
23
6
//
Written By coffee.liu
7
//================================================
8
{$APPTYPE CONSOLE}
9

10
uses
11
SysUtils;
12

type Person
=
Class protectedclass
Class protectedclass
13
protected
14
sex:string;
15
race:string;
16
public Function GetSex()Function GetSex()Function GetSex()function GetSex():string;
17
public Function GetRace()Function GetRace()Function GetRace()function GetRace():string;
18
public procedure GetInfo;virtual;abstract;
19
public procedure say;virtual;abstract;
20
21
end;
22
/yellow///
23
type YellowPerson=Class (Class (Class (class(Person)
24
constructor YellowPerson();
25
public procedure GetInfo;override;
26
public procedure Say;override;
27
end;
28
type AsiaYellowPerson=Class (Class (Class (class(YellowPerson)
29
constructor create;
30
public procedure Say;override;
31
end;
32
type NorthAmericaYellowPerson=Class (Class (Class (class(YellowPerson)
33
constructor create;
34
public procedure Say;override;
35
end;
36
37
type SouthAmericaYellowPerson=Class (Class (Class (class(YellowPerson)
38
constructor create;
39
public procedure Say;override;
40
end;
41
42
type AustrialiaYellowPerson=Class (Class (Class (class(YellowPerson)
43
constructor create;
44
public procedure Say;override;
45
end;
46
//black//
47
type BlackPerson=Class (Class (Class (class(Person)
48
constructor BlackPerson();
49
public procedure GetInfo;override;
50
public procedure Say;override;
51
end;
52
type EuropeBlackPerson=Class (Class (Class (class(BlackPerson)
53
constructor create;
54
public procedure Say;override;
55
end;
56
type AfricaBlackPerson=Class (Class (Class (class(BlackPerson)
57
constructor create;
58
public procedure Say;override;
59
end;
60
write/
61
type WritePerson=Class (Class (Class (class(Person)
62
constructor WritePerson();
63
public procedure GetInfo;override;
64
public procedure Say;override;
65
end;
66
type EuropeWritePerson=Class (Class (Class (class(WritePerson)
67
constructor create;
68
public procedure Say;override;
69
end;
70
type NorthAmericaWritePerson=Class (Class (Class (class(WritePerson)
71
constructor create;
72
public procedure Say;override;
73
end;
74
type SouthAmericaWritePerson=Class (Class (Class (class(WritePerson)
75
constructor create;
76
public procedure Say;override;
77
end;
78
type OrangutanWritePerson=Class (Class (Class (class(WritePerson)
79
constructor create;
80
public procedure Say;override;
81
end;
82
Evolution
83
type ArrPersons=array of Person;
84
type Evolution=Class publicclassClass publicclass
85
public
86
Persons: ArrPersons;
87
public procedure evolution();virtual;abstract;
88
constructor create;
89
// published
90
// Property GetPerson()Property GetPerson()Property GetPerson()property GetPerson:ArrPersons read Persons;
91
end;
92
type EvolutionToYellow=Class (Class (Class (class(Evolution)
93
constructor EvolutionToYellow();
94
public procedure evolution();override;
95
end;
96
type EvolutionToBlack=Class (Class (Class (class(Evolution)
97
constructor EvolutionToBlack();
98
public procedure evolution();override;
99
end;
100
type EvolutionToWrite=Class (Class (Class (class(Evolution)
101
constructor EvolutionToWrite();
102
public procedure evolution();override;
103
end;
104
{ Person }
105
106
Function Person()Function Person()Function Person()function Person.GetRace: string;
107
begin
108
result:=race;
109
end;
110
111
Function Person()Function Person()Function Person()function Person.GetSex: string;
112
begin
113
result:=sex;
114
end;
115
116
{ YellowPerson }
117
118
constructor YellowPerson.YellowPerson;
119
begin
120
inherited ;
121
sex:='Man';
122
race:='Yellow';
123
end;
124
procedure YellowPerson.GetInfo;
125
begin
126
inherited;
127
WriteLn('the '+race+' Person Info:'+sex);
128
end;
129
procedure YellowPerson.Say;
130
begin
131
inherited;
132
WriteLn('I am a Yellow Baby');
133
end;
134
135
{ WritePerson }
136
137
procedure WritePerson.GetInfo;
138
begin
139
inherited;
140
WriteLn('the '+race+' Person Info:'+sex);
141
end;
142
143
procedure WritePerson.Say;
144
begin
145
inherited;
146
WriteLn('I am a Write Baby');
147
end;
148
149
constructor WritePerson.WritePerson;
150
begin
151
inherited;
152
sex:='Man';
153
race:='Write';
154
end;
155
156
{ BlackPerson }
157
158
constructor BlackPerson.BlackPerson;
159
begin
160
inherited;
161
sex:='Man';
162
race:='Black';
163
end;
164
165
procedure BlackPerson.GetInfo;
166
begin
167
inherited;
168
WriteLn('the '+race+' Person Info:'+sex);
169
end;
170
171
procedure BlackPerson.Say;
172
begin
173
inherited;
174
WriteLn('I am a Black Baby');
175
end;
176
177
{ EvolutionToBlack }
178
179
procedure EvolutionToBlack.evolution;
180
var
181
eb:EuropeBlackPerson;
182
ab:AfricaBlackPerson;
183
begin
184
setlength(Persons,2);
185
eb:=EuropeBlackPerson.create;
186
ab:=AfricaBlackPerson.create;
187
persons[0]:= person(eb);
188
persons[1]:= person(ab);
189
190
// result:=BlackPerson.BlackPerson;
191
end;
192
193
constructor EvolutionToBlack.EvolutionToBlack;
194
begin
195
inherited create;
196
end;
197
198
{ EvolutionToWrite }
199
200
procedure EvolutionToWrite.evolution;
201
var
202
ew:EuropeWritePerson;
203
nw:NorthAmericaWritePerson;
204
sw:SouthAmericaWritePerson;
205
ow:OrangutanWritePerson;
206
begin
207
setlength(Persons,4);
208
ew:=EuropeWritePerson.create;
209
nw:=NorthAmericaWritePerson.create;
210
sw:=SouthAmericaWritePerson.create;
211
ow:=OrangutanWritePerson.create;
212
persons[0]:= person(ew);
213
persons[1]:= person(nw);
214
persons[2]:= person(sw);
215
persons[3]:= person(ow);
216
end;
217
218
constructor EvolutionToWrite.EvolutionToWrite;
219
begin
220
inherited create;
221
end;
222
223
{ EvolutionToYellow }
224
225
procedure EvolutionToYellow.evolution;
226
var
227
ay:AsiaYellowPerson;
228
asy:AustrialiaYellowPerson;
229
ny:NorthAmericaYellowPerson;
230
sy:SouthAmericaYellowPerson;
231
begin
232
setlength(Persons,4);
233
ay:=AsiaYellowPerson.create;
234
asy:=AustrialiaYellowPerson.create;
235
ny:=NorthAmericaYellowPerson.create;
236
sy:=SouthAmericaYellowPerson.create;
237
persons[0]:= person(ay);
238
persons[1]:= person(asy);
239
persons[2]:= person(ny);
240
persons[3]:= person(sy);
241
end;
242
243
244
245
constructor EvolutionToYellow.EvolutionToYellow;
246
begin
247
inherited create;
248
end;
249
250
{ AsiaYellowPerson }
251
252
constructor AsiaYellowPerson.create;
253
begin
254
inherited YellowPerson;
255
end;
256
257
procedure AsiaYellowPerson.Say;
258
begin
259
// inherited;
260
WriteLn(' am a Yellow Baby ,My state in Asia');
261
end;
262
263
{ AustrialiaYellowPerson }
264
265
constructor AustrialiaYellowPerson.create;
266
begin
267
inherited YellowPerson;
268
end;
269
270
procedure AustrialiaYellowPerson.Say;
271
begin
272
//inherited;
273
WriteLn(' am a Yellow Baby ,My state in Austrialia');
274
end;
275
276
{ NorthAmericaYellowPerson }
277
278
constructor NorthAmericaYellowPerson.create;
279
begin
280
inherited YellowPerson;
281
end;
282
283
procedure NorthAmericaYellowPerson.Say;
284
begin
285
// inherited;
286
WriteLn(' am a Yellow Baby ,My state in NorthAmerica');
287
end;
288
289
{ SouthAmericaYellowPerson }
290
291
constructor SouthAmericaYellowPerson.create;
292
begin
293
inherited YellowPerson;
294
end;
295
296
procedure SouthAmericaYellowPerson.Say;
297
begin
298
// inherited;
299
WriteLn(' am a Yellow Baby ,My state in SouthAmerica');
300
end;
301
302
{ AfricaBlackPerson }
303
304
constructor AfricaBlackPerson.create;
305
begin
306
inherited BlackPerson;
307
end;
308
309
procedure AfricaBlackPerson.Say;
310
begin
311
// inherited;
312
WriteLn('I am a Black Baby ,My state in Africa');
313
314
end;
315
316
{ EuropeBlackPerson }
317
318
constructor EuropeBlackPerson.create;
319
begin
320
inherited BlackPerson;
321
end;
322
323
procedure EuropeBlackPerson.Say;
324
begin
325
// inherited;
326
WriteLn('I am a Black Baby ,My state in Europe');
327
end;
328
329
{ OrangutanWritePerson }
330
331
constructor OrangutanWritePerson.create;
332
begin
333
inherited WritePerson;
334
end;
335
336
procedure OrangutanWritePerson.Say;
337
begin
338
// inherited;
339
WriteLn('I am a Baby ,I am a Orangutan,I like banananananana..');
340
end;
341
342
{ EuropeWritePerson }
343
344
constructor EuropeWritePerson.create;
345
begin
346
inherited WritePerson;
347
end;
348
349
procedure EuropeWritePerson.Say;
350
begin
351
// inherited;
352
WriteLn('I am a Write Baby ,My state in Europe');
353
end;
354
355
{ NorthAmericaWritePerson }
356
357
constructor NorthAmericaWritePerson.create;
358
begin
359
inherited WritePerson;
360
end;
361
362
procedure NorthAmericaWritePerson.Say;
363
begin
364
//inherited;
365
WriteLn('I am a Write Baby ,My state in NorthAmerica');
366
end;
367
368
{ SouthAmericaWritePerson }
369
370
constructor SouthAmericaWritePerson.create;
371
begin
372
inherited WritePerson;
373
end;
374
375
procedure SouthAmericaWritePerson.Say;
376
begin
377
// inherited;
378
WriteLn('I am a Write Baby ,My state in SouthAmerica');
379
end;
380
381
{ Evolution }
382
383
constructor Evolution.create;
384
begin
385
386
evolution;
387
end;
388
389
390
var
391
//yev,wev,bev:Evolution;
392
//yp,wp,bp:Person;
393
evs:array [0..2] of Evolution;
394
i,j:integer;
395
begin
396
397
evs[0]:=EvolutionToYellow.EvolutionToYellow;
398
evs[1]:=EvolutionToBlack.EvolutionToBlack;
399
evs[2]:=EvolutionToWrite.EvolutionToWrite;
400
for j:=0 to 2 do
401
begin
402
for i:=0 to length(evs[j].Persons)-1 do
403
begin
404
evs[j].Persons[i].say;
405
end;
406
end;
407
408
end.