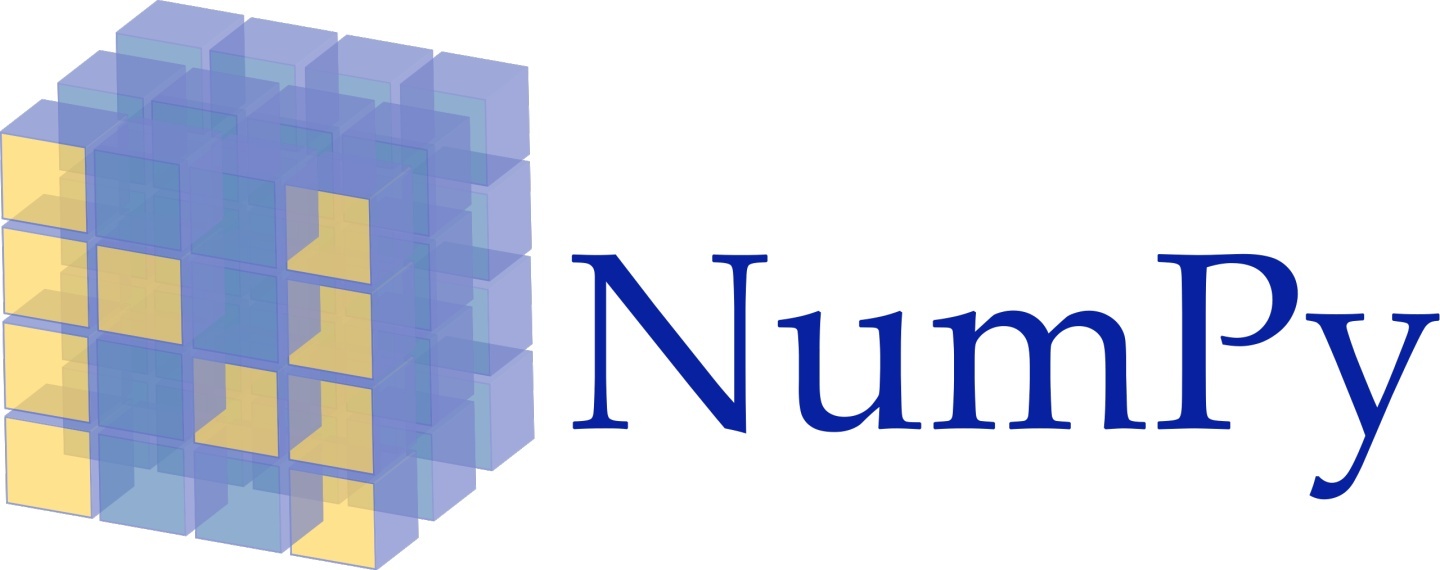
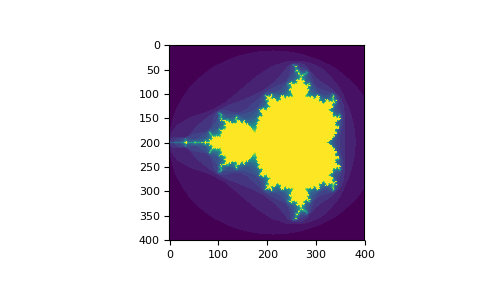
创建保存
np.genfromtxt(data, ...) 链接
np.genfromtxt(data, ...)
>>> data = BytesIO("So it goesn#a b cn1 2 3n 4 5 6")
>>> np.genfromtxt(data, skip_header=1, names=True)
array([(1.0, 2.0, 3.0), (4.0, 5.0, 6.0)],
dtype=[('a', '<f8'), ('b', '<f8'), ('c', '<f8')])
>>> data = "N/A, 2, 3n4, ,???"
>>> kwargs = dict(delimiter=",",
... dtype=int,
... names="a,b,c",
... missing_values={
0:"N/A", 'b':" ", 2:"???"},
... filling_values={
0:0, 'b':0, 2:-999})
>>> np.genfromtxt(BytesIO(data), **kwargs)
array([(0, 2, 3), (4, 0, -999)],
dtype=[('a', '<i8'), ('b', '<i8'), ('c', '<i8')])
load(file[, mmap_mode, allow_pickle, …])
Load arrays or pickled objects from .npy, .npz or pickled files.
save(file, arr[, allow_pickle, fix_imports])
Save an array to a binary file in NumPy .npy format.
savez(file, *args, **kwds)
Save several arrays into a single file in uncompressed .npz format.
savez_compressed(file, *args, **kwds)
Save several arrays into a single file in compressed .npz format.
ndarray.tofile(fid[, sep, format])
Write array to a file as text or binary (default).
ndarray.tolist()
Return the array as an a.ndim-levels deep nested list of Python scalars.
索引查找
k维的数组,索引时需要k个索引值,索引值可以是常数、切片、数组索引(整数、布尔)
a = array([[ 0, 1, 2, 3],
[ 4, 5, 6, 7],
[ 8, 9, 10, 11]])
# a.shape = (3, 4)
# 整数及切片索引
# Slice用的是view的方式,而index用的是copy方式
a[1,2] # 6
a[0, [1,2]] # [1, 2]
a[0:2, 2:4] # [[2, 3], [6, 7]]
a[:, 2:4] # [[2, 3], [6, 7], [10, 11]]
a[0:1, ..., 2:4, 0]
# 整数数组索引
i=array([[1, 2], [0, 1]])
j=array([[0, 2], [1, 2]])
a[i, j] == array([[ 2, 5],
[ 7, 11]])
# a[i, j].shape == a[0, j].shape == a[i, 0].shape == (2, 2)
a[:, j] == array([[[ 2, 1],
[ 3, 3]],
[[ 6, 5],
[ 7, 7]],
[[10, 9],
[11, 11]]])
# a[:, j].shape == (3, 2, 2)
a[i, :] == array([[[ 0, 1, 2, 3],
[ 4, 5, 6, 7]],
[[ 4, 5, 6, 7],
[ 8, 9, 10, 11]]])
# a[i, :].shape == (2, 2, 4)
# 布尔数组索引
b = a > 4
b == array([[False, False, False, False],
[False, True, True, True],
[ True, True, True, True]], dtype=bool)
a[b] == array([ 5, 6, 7, 8, 9, 10, 11])
a[b] = 0 # 只是视图,可以赋值
a == array([[0, 1, 2, 3],
[4, 0, 0, 0],
[0, 0, 0, 0]])
a[a[:,0]>2, a[0,:]>1] # a中第一列大于2的行且第一行大于1的列的元素
# ix_函数可以产生多元组合
a = array([2,3,4,5])
b = array([8,5,4])
c = array([5,4,6,8,3])
ax,bx,cx = ix_(a,b,c)
# ax.shape, bx.shape, cx.shape == ((4, 1, 1), (1, 3, 1), (1, 1, 5))
result = ax+bx*cx #利用广播机制,产生不同维度的所有组合
result[3,2,4] == a[3]+b[2]*c[4] == 17
# 笛卡尔积
numpy.transpose([numpy.tile(x, len(y)), numpy.repeat(y, len(x))])
array([[1, 4],
[2, 4],
[3, 4],
[1, 5],
[2, 5],
[3, 5]])
[[x0, y0] for x0 in x for y0 in y]
# 查找元素
np.where(condition, [x, y]) #[x,y]为可选项,满足条件返回x,不满足条件返回y
>>> np.where(a < 4, a, -1) # -1 is broadcast
array([[ 0, 1, 2],
[ 0, 2, -1],
[ 0, 3, -1]])
np.argwhere() #返回满足括号内条件的元素的索引,数组形式
>>> np.argwhere(a>5)
array([[0, 2],
[1, 0],
[1, 3],
[2, 3]], dtype=int64)
数组遍历
# 获取索引迭代器
>>> for index in np.ndindex(3, 2, 1):
| ... print(index)
| (0, 0, 0)
| (0, 1, 0)
| (1, 0, 0)
| (1, 1, 0