1、从一个数组中选择最大最小值
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ConsoleApp1
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine("请输入5个数字:");
int[] arr = new int[5];
int max = int.MinValue;
int min = int.MaxValue;
for (int i = 0; i < 5; ++i)
{
arr[i] = Convert.ToInt16(Console.ReadLine());
if (max < arr[i])
max = arr[i];
if (min > arr[i])
min = arr[i];
}
Console.WriteLine("最大的数是:" + max);
Console.WriteLine("最小的数是:" + min);
Console.ReadKey();
}
}
}
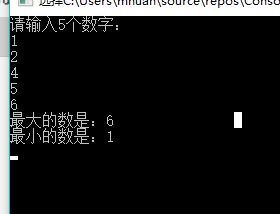
image.png
2、九九乘法表
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ConsoleApp2
{
class Program
{
static void Main(string[] args)
{
for (int i = 1; i < 10; ++i)
{
for (int j = 1; j <= i; ++j)
{
if (i * j > 9)
Console.Write("" + i + " * " + j + " = " + i * j + " ");
else
Console.Write("" + i + " * " + j + " = " + i * j + " ");
}
Console.WriteLine();
}
Console.ReadKey();
}
}
}
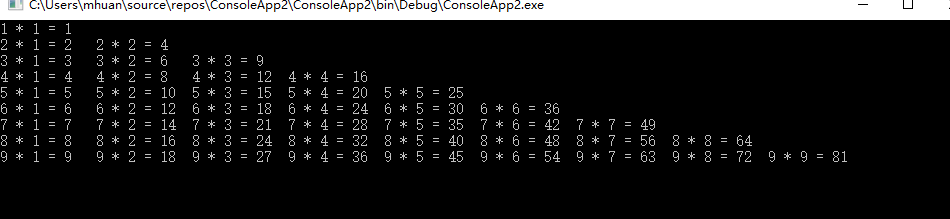
image.png
3、分别使用for循环和while循环设计一个程序,计算从1加到100的和
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ConsoleApp3
{
class Program
{
static void Main(string[] args)
{
int sum = 0;
for (int i = 1; i <= 100; ++i)
{
sum += i;
}
Console.WriteLine("for:1+..+100 = " + sum);
sum = 0;
int j = 1;
while (j <= 100)
{
sum += j;
j++;
}
Console.WriteLine("while:1+..+100 = " + sum);
Console.ReadKey();
}
}
}
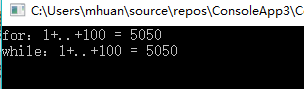
image.png
4、利用数学类提供的平方根方法计算并输出1.0,2.0,3.0,…,10.0的平方根。
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ConsoleApp4
{
class Program
{
static void Main(string[] args)
{
for (int i = 1; i <= 10; ++i)
{
Console.WriteLine(i + "的平方根" + " = " + Math.Sqrt(i));
}
Console.ReadKey();
}
}
}
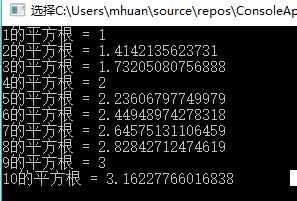
image.png
5、随机数方法产生5个1~10(包括1和10)之间的整数。
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ConsoleApp5
{
class Program
{
static void Main(string[] args)
{
Random ran = new Random();
for (int i = 1; i <= 5; ++i)
{
Console.WriteLine("第" + i + "个随机数是 " + ran.Next(1, 11));
}
Console.ReadKey();
}
}
}
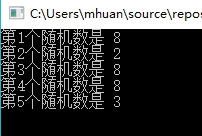
image.png
6、编程实现:随机产生1~20之间的整数,总共生成1000次,统计其中生成的整数0,1,2,3,... …,20的个数分别是多少,并输出统计结果(每5个数一行)。
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ConsoleApp6
{
class Program
{
static void Main(string[] args)
{
Random ran = new Random();
int[] arr = new int[1001];
for (int i = 1; i <= 1000; ++i)
{
arr[ran.Next(1, 21)]++;
}
for (int i = 1; i < 10; ++i)
{
Console.WriteLine(i + " 出现的次数是: " + arr[i]);
}
for (int i = 10; i <= 20; ++i)
{
Console.WriteLine(i + " 出现的次数是: " + arr[i]);
}
Console.ReadKey();
}
}
}
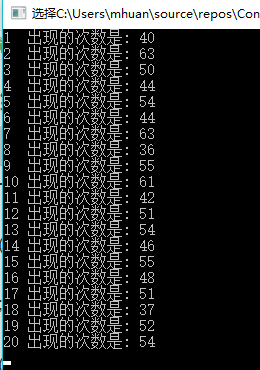
image.png
7、统计从键盘中输入字符串中分别有多少个数字和字母。
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ConsoleApp7
{
class Program
{
static void Main(string[] args)
{
int letter = 0, digit = 0;
string s1 = Console.ReadLine();
foreach (char c in s1)
{
if (char.IsLetter(c))
{
letter++;
continue;
}
if (char.IsDigit(c))
{
digit++;
continue;
}
}
Console.WriteLine("字母出现的次数是: " + letter);
Console.WriteLine("数字出现的次数是: " + digit);
Console.ReadKey();
}
}
}
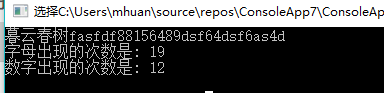
image.png
8、编写程序,要求用户输入月份号码,然后显示该月的英文名称。例如,如果用户输入2,程序应显示February。要求月的英文名称存于数组中。
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ConsoleApp8
{
class Program
{
static void Main(string[] args)
{
string[] s = new string[12];
s[0] = "January"; s[1] = "February"; s[2] = "March"; s[3] = "April";
s[4] = "May"; s[5] = "June"; s[6] = "July"; s[7] = "August";
s[8] = "September"; s[9] = "October"; s[10] = "November"; s[11] = "December";
int i = Convert.ToInt16(Console.ReadLine());
Console.WriteLine(i + "月份" + "是 " + s[i - 1]);
Console.ReadKey();
}
}
}
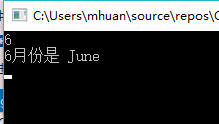
image.png
9、请输入一个代表项数的正整数N(N ≤ 100),然后输出1-3+5-7+9-11+……前N项的和。
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ConsoleApp9
{
class Program
{
static void Main(string[] args)
{
int i = Convert.ToInt16(Console.ReadLine());
int sum = 0;
int num = 1;
for (int j = 1; j <= i; ++j, num += 2)
{
if (j % 2 == 0)
sum -= num;
else
sum += num;
}
Console.WriteLine("前" + i + "项和是 " + sum);
Console.ReadKey();
}
}
}
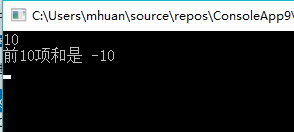
image.png
10、请编写两个程序,分别通过if语句和switch语句两种方式完成,输入一个成绩,将百分制成绩转换成等级制成绩(A为100~90 ,…, F为不及格)。
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ConsoleApp10
{
class Program
{
static void Main(string[] args)
{
int i = Convert.ToInt16(Console.ReadLine());
while (i < 0 || i > 100)
{
Console.WriteLine("数错了,再输一遍");
i = Convert.ToInt16(Console.ReadLine());
}
char a = 'F';
if (i >= 90)
a = 'A';
else if (i >= 80)
a = 'B';
else if (i >= 70)
a = 'C';
else if (i >= 60)
a = 'D';
Console.WriteLine(a);
Console.ReadKey();
}
}
}
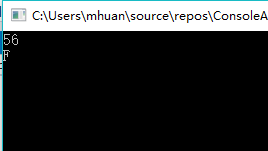
image.png
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ConsoleApp10_2
{
class Program
{
static void Main(string[] args)
{
int i = Convert.ToInt16(Console.ReadLine());
while (i < 0 || i > 100)
{
Console.WriteLine("数错了,再输一遍");
i = Convert.ToInt16(Console.ReadLine());
}
char a = 'F';
switch (i / 10)
{
case 10:
case 9: a = 'A'; break;
case 8: a = 'B'; break;
case 7: a = 'C'; break;
case 6: a = 'D'; break;
}
Console.WriteLine(a);
Console.ReadKey();
}
}
}
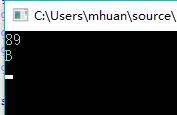
image.png
11、读入两个正整数m和n,输出m和n的最小公倍数。
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ConsoleApp11
{
class Program
{
static void Main(string[] args)
{
int n1 = Convert.ToInt16(Console.ReadLine());
while (n1 <= 0)
{
Console.WriteLine("数错了,再输一遍");
n1 = Convert.ToInt16(Console.ReadLine());
}
int n2 = Convert.ToInt16(Console.ReadLine());
while (n2 < 0)
{
Console.WriteLine("数错了,再输一遍");
n2 = Convert.ToInt16(Console.ReadLine());
}
int min = Math.Min(n1, n2);
for (int i = Math.Max(n1, n2); i < int.MaxValue; i++)
{
if (i % n1 == 0 && i % n2 == 0)
{
Console.WriteLine("最小公倍数是 " + i); Console.ReadKey();
return;
}
}
Console.WriteLine("没找到最小公倍数");
Console.ReadKey();
}
}
}
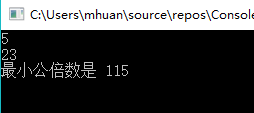
image.png
12、判断一个整数n是否为素数。若是则输出:n是素数;否则输出n不是素数(注:n是具体的值)。
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ConsoleApp12
{
class Program
{
static void Main(string[] args)
{
int n = Convert.ToInt16(Console.ReadLine());
while (n < 0)
{
Console.WriteLine("请输入正整数,再输一遍");
n = Convert.ToInt16(Console.ReadLine());
}
if (n == 0 || n == 1)
{
Console.WriteLine(n + "不是素数"); Console.ReadKey();
return;
}
int MAX = (int)Math.Sqrt(n);
for (int i = 2; i <= MAX; ++i)
{
if (n % i == 0)
{
Console.WriteLine(n + "不是素数"); Console.ReadKey();
return;
}
}
Console.WriteLine(n + "是素数!");
Console.ReadKey();
}
}
}
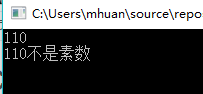
image.png
13、用for循环计算s=1!+2!+3!+…+n!
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ConsoleApp13
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine("请输入一个正整数:");
int n = Convert.ToInt16(Console.ReadLine());
while (n < 0)
{
Console.WriteLine("请输入正整数,再输一遍");
n = Convert.ToInt16(Console.ReadLine());
}
int sum = 0;
int j = 1;
for (int i = 1; i <= n; ++i)
{
j = j * i;
sum += j;
}
Console.WriteLine("s=1!+2!+3!+…+n!的结果为:"+sum);
Console.ReadKey();
}
}
}
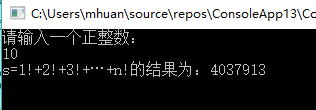
image.png
14、

image.png
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ConsoleApp14
{
class Program
{
static void Main(string[] args)
{
double d = 0;
for (int i = 2; i <= 20; ++i)
{
d += Math.Pow(i, i);
}
if (double.MaxValue - 0.01 < d)
Console.WriteLine("越界!!");
Console.WriteLine(d);
Console.ReadKey();
}
}
}
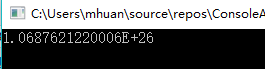
image.png
15、编写一个程序,打印出以下图形:
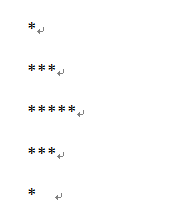
image.png