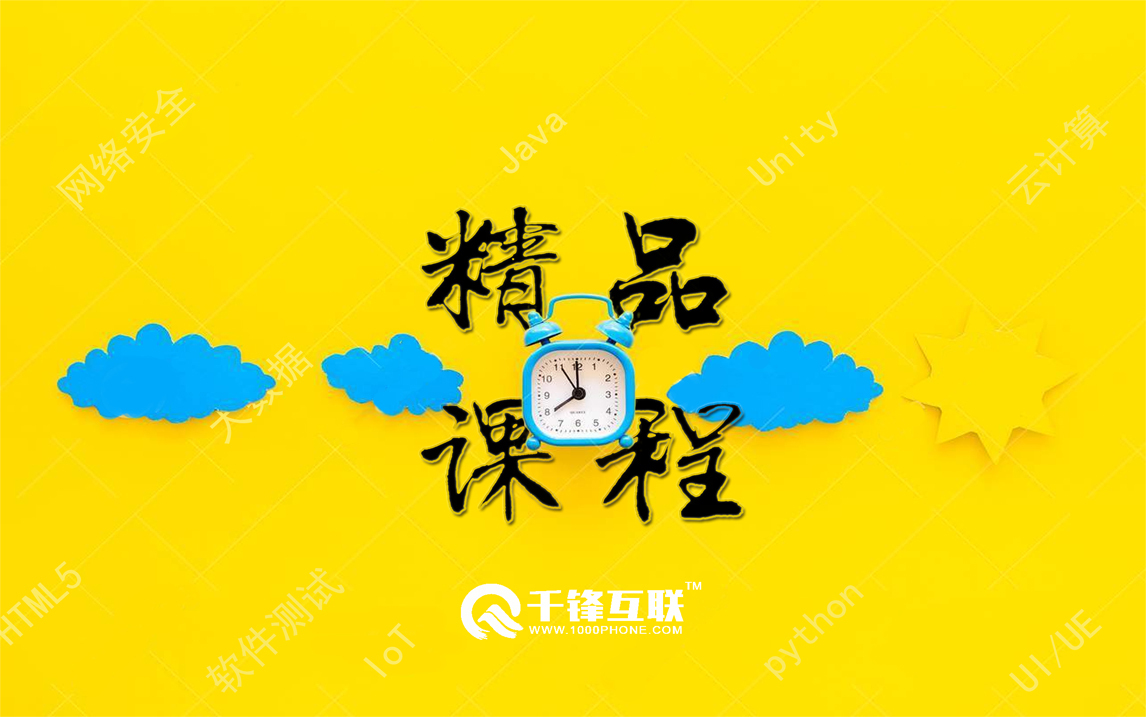
首先我们会发现我们网上看到的打字游戏都是上来就敲代码,小白看的一脸懵,所以鑫哥在这给大家送上一份超级详细的打字游戏编写流程及对应的结果展示,希望可以帮到小白进行入门学习。
话不多说,我们先看一下什么叫做打字游戏:
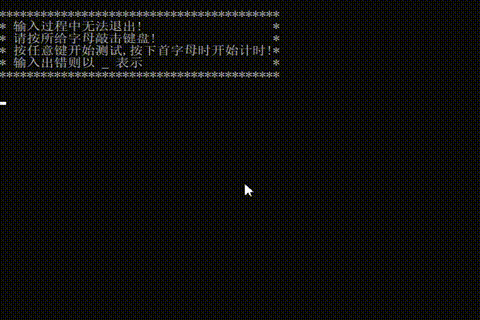
很简单的一个C语言的小游戏,接下来鑫哥将带领大家进行编写运行。
一、开发环境:
本次使用的环境为:Ubuntu系统云+sublime进行开发的。
二、框架分析:
最外层时一个大循环:----------while
打印游戏规则
按下任意键打印生成的随机字符串(20字母的字符串)
先生成一个随机的字符串----按下任意键----打印出来
按下任意键----生成一个随机的字符串----打印出来
按下任意键(需要做出一个没有回显的)----------获取键盘输入
生成一个随机的字符串(生成 随机数->字母->数组)(已知次数循环)--for-函数的调用
打印出来--------输出
按照上面的字符串进行敲键盘
同时进行判断,如果正确就原样输出,如果错误就_输出-------if ---else
在第0次按下时开始计时、最后结束计时--------调用时间函数获取当前系统时间
如果按下的按键和对应的数组中的字母相同---原样输出(同时k++)
如果不一样-----打印_
最后完成了,打印用时、正确个数(正确个数由k表示)
用时 = 结束时间-开始时间 正确个数 = k
按下esc退出、按下空格键继续
判断按下的是什么
ESC退出: retrun 0;//结束当前函数并返回一个0
空格继续:清屏继续玩
三、开始编程:
1.能玩一次的打字游戏
1.1打印游戏规则:
#include <stdio.h>
int main()
{
//1.打印游戏规则
printf("按下任意键开始游戏,按下首字母开始计时,正确原样输出,错误_表示n");
//2.按下任意键,生成一个随机的字符串,打印输出
}

1.2.按下任意键
提供给大家一个源码:mygetch 无回显的getchar(不需要回车)
//------------------封装好的mygetch------------------
#include <termios.h>
#include <unistd.h>
char mygetch()
{
struct termios oldt, newt;
char ch;
tcgetattr( STDIN_FILENO, &oldt );
newt = oldt;
newt.c_lflag &= ~( ICANON | ECHO );
tcsetattr( STDIN_FILENO, TCSANOW, &newt );
ch = getchar();
tcsetattr( STDIN_FILENO, TCSANOW, &oldt );
return ch;
}
//-----------------------------------------------------
复制粘贴在main函数上方进行使用
正常代码:(按下任意键不需要知道按了什么,所以不需要用变量去接返回值)
#include <stdio.h>
//------------------封装好的mygetch------------------
#include <termios.h>
#include <unistd.h>
char mygetch()
{
struct termios oldt, newt;
char ch;
tcgetattr( STDIN_FILENO, &oldt );
newt = oldt;
newt.c_lflag &= ~( ICANON | ECHO );
tcsetattr( STDIN_FILENO, TCSANOW, &newt );
ch = getchar();
tcsetattr( STDIN_FILENO, TCSANOW, &oldt );
return ch;
}
//-----------------------------------------------------
int main()
{
//1.打印游戏规则
printf("按下任意键开始游戏,按下首字母开始计时,正确原样输出,错误_表示n");
//2.按下任意键,生成一个随机的字符串,打印输出
mygetch();//函数名+( );就表示函数的调用 按下任意键
return 0;
}
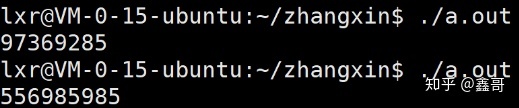
将随机数->字母
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
int main()
{
srand(time(NULL));//设置随机数种子,使用的是时间(当前的系统时间)
int a =rand();
printf("%dn",a);
char b = a%26+'a';
//一个很大的数%26取余就变成了0-25
//一个0-25的数+‘a’就变成了‘a’-‘z’随机字母(ASCII码)
printf("%cn",b);
return 0;
}
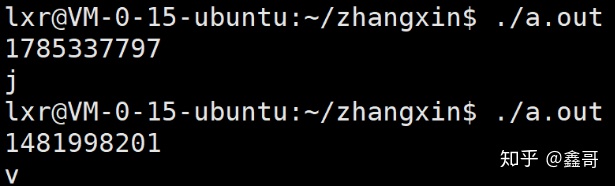
创建数组,将生成好的字母(字符)放入数组的对应位置中
#include <stdio.h>
//------------------封装好的mygetch------------------
#include <termios.h>
#include <unistd.h>
#include <stdlib.h>
#include <time.h>
char mygetch()
{
struct termios oldt, newt;
char ch;
tcgetattr( STDIN_FILENO, &oldt );
newt = oldt;
newt.c_lflag &= ~( ICANON | ECHO );
tcsetattr( STDIN_FILENO, TCSANOW, &newt );
ch = getchar();
tcsetattr( STDIN_FILENO, TCSANOW, &oldt );
return ch;
}
// //-----------------------------------------------------
int main()
{
char haha[20] = "";//20 表示的是数组的长度
srand(time(NULL));//设置随机数种子,使用的是时间(当前的系统时间)
//循环的生成、放入、生成、放入
//因为一共20次,因此我们使用已知循环次数的循环for
int i;
mygetch();//按下任意键
for (i = 0; i < 20; ++i)//要将int i= 0;的int去掉
{
// char b = rand()%26+'a';//生成一个随机的字母
// haha[i] = b;//给数组中的第i个赋值
haha[i] = rand()%26+'a';
}
printf("%sn", haha);//打印生成好的随机字符串
// int a =rand();
// printf("%dn",a);
// char b = a%26+'a';
// //一个很大的数%26取余就变成了0-25
// //一个0-25的数+‘a’就变成了‘a’-‘z’随机字母
// printf("%cn",b);
return 0;
}
按照你上面有的字符进行敲击键盘
#include <stdio.h>
//------------------封装好的mygetch------------------
#include <termios.h>
#include <unistd.h>
#include <stdlib.h>
#include <time.h>
char mygetch()
{
struct termios oldt, newt;
char ch;
tcgetattr( STDIN_FILENO, &oldt );
newt = oldt;
newt.c_lflag &= ~( ICANON | ECHO );
tcsetattr( STDIN_FILENO, TCSANOW, &newt );
ch = getchar();
tcsetattr( STDIN_FILENO, TCSANOW, &oldt );
return ch;
}
// //-----------------------------------------------------
int main()
{
char haha[20] = "";//20 表示的是数组的长度
srand(time(NULL));//设置随机数种子,使用的是时间(当前的系统时间)
int i;
mygetch();//按下任意键
for (i = 0; i < 20; ++i)//要将int i= 0;的int去掉
{
haha[i] = rand()%26+'a';
}
printf("%sn", haha);//打印生成好的随机字符串
int time_s = 0,time_e = 0,k = 0;
for (i = 0; i < 20; ++i)
{
char a = mygetch();//获取键盘输入的一个字符
if (i == 0)
{
time_s = time(NULL);//如果第0次按下就获取下当前的时间
}
if (a == haha[i])
{
printf("%c", a);
k++;
}
else
printf("_");
}
time_e = time(NULL);
printf("n");
printf("用时:%ds,正确个数%d个n",time_e - time_s ,k);
return 0;
}
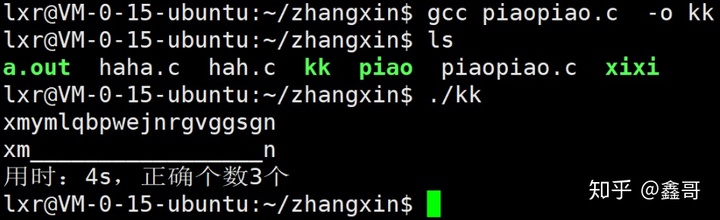
3.如何做成可以玩多次的打字游戏:
按下空格就打印haha,按下esc就退出
#include <stdio.h>
//------------------封装好的mygetch------------------
#include <termios.h>
#include <unistd.h>
#include <stdlib.h>
#include <time.h>
char mygetch()
{
struct termios oldt, newt;
char ch;
tcgetattr( STDIN_FILENO, &oldt );
newt = oldt;
newt.c_lflag &= ~( ICANON | ECHO );
tcsetattr( STDIN_FILENO, TCSANOW, &newt );
ch = getchar();
tcsetattr( STDIN_FILENO, TCSANOW, &oldt );
return ch;
}
int main(int argc, char const *argv[])
{
int r = 1;
while(1)//死循环
{
printf("hahahahahan");
r = 1;
while(r)
{
printf("esc退出,空格继续,其他不好使n");
char ch = mygetch();
if (ch == 27)
{
return 0;
}
else if (ch ==32)
{
r = 0;
}
else
r = 1;
}
}
return 0;
}
将上面进行合并最后就是整个小项目:
#include <stdio.h>
//------------------封装好的mygetch------------------
#include <termios.h>
#include <unistd.h>
#include <stdlib.h>
#include <time.h>
char mygetch()
{
struct termios oldt, newt;
char ch;
tcgetattr( STDIN_FILENO, &oldt );
newt = oldt;
newt.c_lflag &= ~( ICANON | ECHO );
tcsetattr( STDIN_FILENO, TCSANOW, &newt );
ch = getchar();
tcsetattr( STDIN_FILENO, TCSANOW, &oldt );
return ch;
}
int main(int argc, char const *argv[])
{
int r = 1;
while(1)//死循环
{
system("clear");//清空屏幕
printf("按下任意键开始游戏,按下首字母开始计时,正确原样输出,错误_表示n");
char haha[21] = "";//20 表示的是数组的长度
srand(time(NULL));//设置随机数种子,使用的是时间(当前的系统时间)
int i;
mygetch();//按下任意键
for (i = 0; i < 20; ++i)//要将int i= 0;的int去掉
{
haha[i] = rand()%26+'a';
}
printf("%sn", haha);//打印生成好的随机字符串
int time_s = 0,time_e = 0,k = 0;
for (i = 0; i < 20; ++i)
{
char a = mygetch();//获取键盘输入的一个字符
if (i == 0)
{
time_s = time(NULL);
//如果第0次按下就获取下当前的时间
}
if (a == haha[i])
{
printf("%c", a);
k++;
}
else
printf("_");
}
time_e = time(NULL);
printf("n");
printf("用时:%ds,正确个数%d个n",time_e - time_s ,k);
r = 1;
while(r)
{
printf("esc退出,空格继续,其他不好使n");
char ch = mygetch();
if (ch == 27)
{
return 0;
}
else if (ch ==32)
{
r = 0;
}
else
r = 1;
}
}
return 0;
}
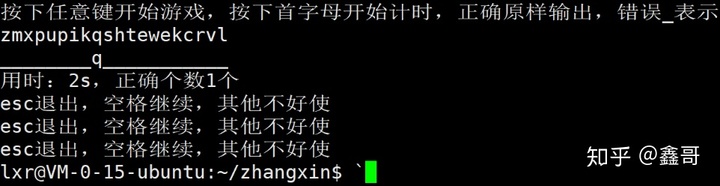
感谢各位小伙伴的支持欢迎点赞关注,谢谢,配合B站使用更佳
鑫哥B站:
千锋项目体验课-自制ipod-2打字游戏框架_哔哩哔哩 (゜-゜)つロ 干杯~-bilibiliwww.bilibili.com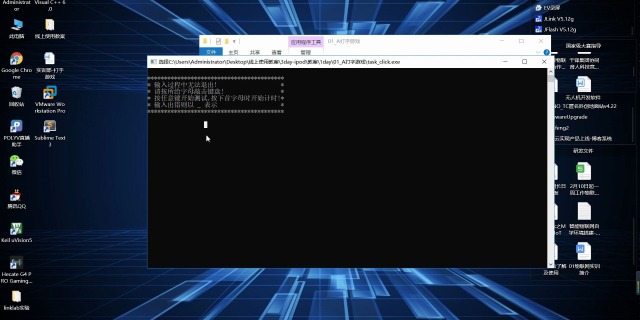