<dependency>
<groupId>com.github.wxpay</groupId>
<artifactId>wxpay-sdk</artifactId>
<version>0.0.3</version>
</dependency>
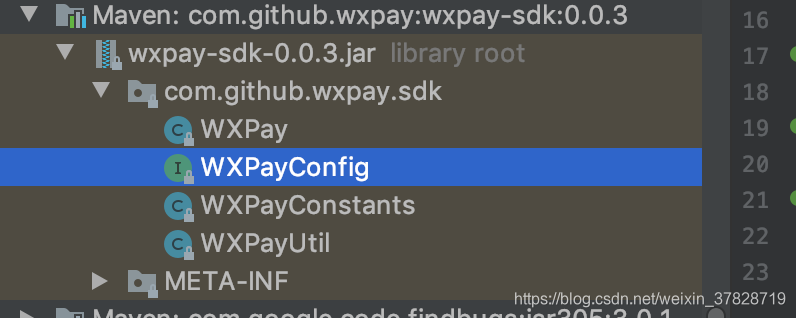
1. 配置实现WXPayConfig
import com.github.wxpay.sdk.WXPayConfig;
import java.io.InputStream;
public class DemoWXPayConfig implements WXPayConfig {
/**
*apiclient_cert.p12是商户证书文件,除PHP外的开发均使用此证书文件
*/
private static final String WX_PAY_CLIENT_CERT_PATH = "申请的apiclient_cert.p12证书存放地址";
/**
* @return
*/
@Override
public String getAppID() {
return "申请的";
}
/**
* @return
*/
@Override
public String getMchID() {
return "申请的";
}
/**
* @return
*/
@Override
public String getKey() {
return "申请的";
}
/**
* @return
*/
@Override
public InputStream getCertStream() {
return this.getClass().getResourceAsStream(WX_PAY_CLIENT_CERT_PATH);
}
/**
* @return
*/
@Override
public int getHttpConnectTimeoutMs() {
return 8000;
}
/**
* @return
*/
@Override
public int getHttpReadTimeoutMs() {
return 10000;
}
}
2.继承WXPay 方法重写
import com.fasterxml.jackson.databind.ObjectMapper;
import com.github.wxpay.sdk.WXPay;
import com.github.wxpay.sdk.WXPayConfig;
import com.github.wxpay.sdk.WXPayConstants.SignType;
import com.github.wxpay.sdk.WXPayUtil;
import java.util.Map;
import static com.github.wxpay.sdk.WXPayConstants.SignType.MD5;
import static com.github.wxpay.sdk.WXPayUtil.generateNonceStr;
import static com.github.wxpay.sdk.WXPayUtil.generateSignature;
import static com.google.common.collect.Maps.newHashMap;
import static com.xxxxxxxxxxx.encode;
public class WXWithdrawPay extends WXPay {
/**
*
*/
private WXPayConfig config;
/**
*
*/
private SignType signType;
/**
*
*/
private boolean useSandbox;
/**
* @param config
*/
public WXWithdrawPay(WXPayConfig config) {
super(config);
this.config = config;
this.signType = MD5;
this.useSandbox = false;
}
/**
* @param config
* @param signType
*/
public WXWithdrawPay(WXPayConfig config, SignType signType) {
super(config, signType);
this.config = config;
this.signType = signType;
this.useSandbox = false;
}
/**
* @param config
* @param signType
* @param useSandbox
*/
public WXWithdrawPay(WXPayConfig config, SignType signType, boolean useSandbox) {
super(config, signType, useSandbox);
this.config = config;
this.signType = signType;
this.useSandbox = useSandbox;
}
/**
* @param reqData
* @param signTypeEnabled
* @return
* @throws Exception
*/
public Map<String, String> fillRequestDataWithoutAppid(Map<String, String> reqData, boolean signTypeEnabled) throws Exception {
reqData.put("mch_id", this.config.getMchID());
reqData.put("nonce_str", generateNonceStr());
if (signTypeEnabled) {
if (MD5.equals(this.signType)) {
reqData.put("sign_type", "MD5");
} else if (SignType.HMACSHA256.equals(this.signType)) {
reqData.put("sign_type", "HMAC-SHA256");
}
}
reqData.put("sign", generateSignature(reqData, this.config.getKey(), this.signType));
return reqData;
}
/**
* @param reqData
* @param signTypeEnabled
* @return
* @throws Exception
*/
public Map<String, String> fillWithdrawToWXRequestDataWithoutAppid(Map<String, String> reqData,
boolean signTypeEnabled) throws Exception {
reqData.put("mch_appid", this.config.getAppID());
reqData.put("mchid", this.config.getMchID());
reqData.put("nonce_str", generateNonceStr());
if (signTypeEnabled) {
if (MD5.equals(this.signType)) {
reqData.put("sign_type", "MD5");
} else if (SignType.HMACSHA256.equals(this.signType)) {
reqData.put("sign_type", "HMAC-SHA256");
}
}
reqData.put("sign", generateSignature(reqData, this.config.getKey(), this.signType));
return reqData;
}
/**
* @param xmlStr
* @return
* @throws Exception
*/
public Map<String, String> processResponseXmlWithoutValidSign(String xmlStr) throws Exception {
String RETURN_CODE = "return_code";
Map<String, String> respData = WXPayUtil.xmlToMap(xmlStr);
if (respData.containsKey(RETURN_CODE)) {
String return_code = respData.get(RETURN_CODE);
if (return_code.equals("FAIL")) {
return respData;
} else if (return_code.equals("SUCCESS")) {
return respData;
} else {
throw new Exception(String.format("return_code value %s is invalid in XML: %s", return_code, xmlStr));
}
} else {
throw new Exception(String.format("No `return_code` in XML: %s", xmlStr));
}
}
/**
* @param reqData
* @return
* @throws Exception
*/
public Map<String, String> withdraw(Map<String, String> reqData) throws Exception {
return this.withdraw(reqData, this.config.getHttpConnectTimeoutMs(), this.config.getHttpReadTimeoutMs());
}
/**
* @param reqData
* @param connectTimeoutMs
* @param readTimeoutMs
* @return
* @throws Exception
*/
public Map<String, String> withdraw(Map<String, String> reqData, int connectTimeoutMs, int readTimeoutMs) throws Exception {
String url = "https://api.mch.weixin.qq.com/mmpaysptrans/pay_bank";
String respXml = this.requestWithCert(url, this.fillRequestDataWithoutAppid(reqData, false), connectTimeoutMs, readTimeoutMs);
return this.processResponseXmlWithoutValidSign(respXml);
}
/**
* @param reqData
* @return
* @throws Exception
*/
public Map<String, String> withdrawToWX(Map<String, String> reqData) throws Exception {
return this.withdrawToWX(reqData, this.config.getHttpConnectTimeoutMs(), this.config.getHttpReadTimeoutMs());
}
/**
* @param reqData
* @param connectTimeoutMs
* @param readTimeoutMs
* @return
* @throws Exception
*/
public Map<String, String> withdrawToWX(Map<String, String> reqData, int connectTimeoutMs, int readTimeoutMs) throws Exception {
String url = "https://api.mch.weixin.qq.com/mmpaymkttransfers/promotion/transfers";
String respXml = this.requestWithCert(url, this.fillWithdrawToWXRequestDataWithoutAppid(reqData, false), connectTimeoutMs, readTimeoutMs);
return this.processResponseXmlWithoutValidSign(respXml);
}
/**
* @return
* @throws Exception
*/
public Map<String, String> queryPublicKey() throws Exception {
return this.queryPublicKey(this.config.getHttpConnectTimeoutMs(), this.config.getHttpReadTimeoutMs());
}
/**
* @param connectTimeoutMs
* @param readTimeoutMs
* @return
* @throws Exception
*/
public Map<String, String> queryPublicKey(int connectTimeoutMs, int readTimeoutMs) throws Exception {
String url = "https://fraud.mch.weixin.qq.com/risk/getpublickey";
Map<String, String> reqData = newHashMap();
String respXml = this.requestWithCert(url, this.fillRequestDataWithoutAppid(reqData, true), connectTimeoutMs, readTimeoutMs);
return this.processResponseXmlWithoutValidSign(respXml);
}
}