一、内存分区模型
- 代码区:存放函数体的二进制代码,由操作系统进行管理的
- 全局区:存放全局变量和静态变量以及常量
- 栈区:由编译器自动分配释放,存放函数的参数值,局部变量等
- 堆区:由程序员分配和释放,若程序员不是放,程序结束时由操作系统回收
- 不同区域存放的数据赋予不同的声明周期
1.1 程序运行前
- 程序编译后,生成了.exe可执行程序,未执行该程序前分为两个区域:
- 代码区
- 存放CPU执行的机器指令
- 代码区是共享的,对于频繁执行的程序,内存中只有一份代码即可
- 代码区是只读的,防止程序意外的修改它的指令
- 全局区
- 全局变量和静态变量存放在此处
- 全局区还包含了常量区,字符串常量和其他常量也存放在此
- 该区域的数据在程序结束后由操作系统释放
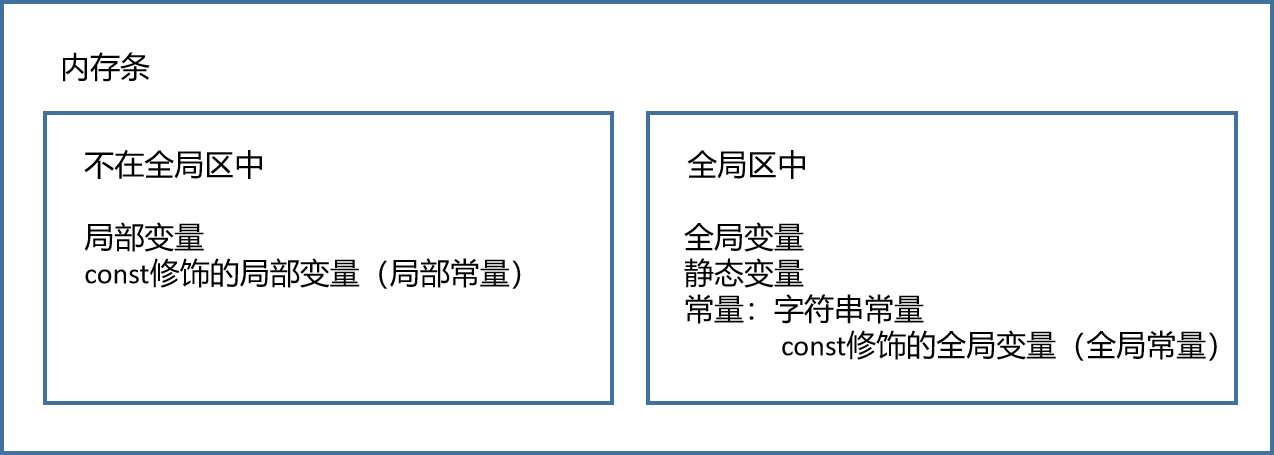
// 全局变量
int g_a = 10;
int g_b = 10;
// const 修饰的全局常量
const int c_g_a = 10;
const int c_g_b = 10;
int main() {
// 全局区:全局变量、静态变量、常量
cout << "全局变量g_a的地址:" << (int)&g_a << endl; // -1122836480
cout << "全局变量g_b的地址:" << (int)&g_b << endl; // -1122836476
// 静态变量
static int s_a = 10;
static int s_b = 10;
cout << "静态变量a的地址:" << (int)&s_a << endl; // -1122836472
cout << "静态变量b的地址:" << (int)&s_b << endl; // -1122836468
// 常量
// 字符串常量
cout << "字符串常量的地址:" << (int)&"Hello World!" << endl; // -1122849008
// const 修饰的常量
// const 修饰的全局常量
cout << "const 修饰的全局常量a的地址:" << (int)&c_g_a << endl; // -1122849692
cout << "const 修饰的全局常量b的地址:" << (int)&c_g_b << endl; // -1122849688
// 局部变量
int a = 10;
int b = 10;
cout << "局部变量a的地址:" << (int)&a << endl; // 1095760164
cout << "局部变量b的地址:" << (int)&b << endl; // 1095760196
// const 修饰的局部变量
const int c_l_a = 10;
const int c_l_b = 10;
cout << "const修饰局部常量c_l_a的地址:" << (int)&c_l_a << endl;// 1095760228
cout << "const修饰局部常量c_l_a的地址:" << (int)&c_l_b << endl;// 1095760260
system("pause");
return 0;
}
1.2 程序运行后
- 栈区
- 由编译器在自动分配释放,存放函数的参数值,局部变量等
- 不要返回局部变量的地址,栈区开辟的数据由编译器自动释放
int* func(int b) { // 形参数据也会放在栈区
b = 100;
int a = 10; // 局部变量存放在栈区,栈区数据在函数执行后自动释放
return &b; // 返回局部变量的地址
}
int main() {
int* p = func(10);
cout << *p << endl; // 100 第一次打印正确数据因为编译器会保留
cout << *p << endl; // 795287552 第二次这个数据就不再保留
}
- 堆区
- 由程序员分配释放,若程序员不释放,程序结束时由操作系统回收
- 再C++中主要利用new再堆区开辟内存
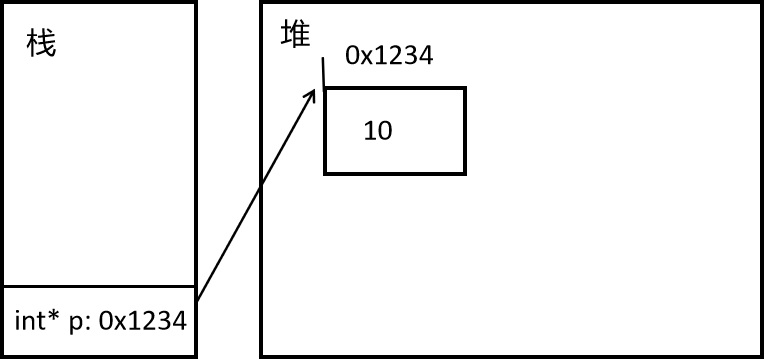
int* func() {
// 利用 new 关键字 可以将数据开辟到堆区
// 指针本质也是局部变量放在栈中,指针保存的数据是放在堆区
int* p = new int(10);
return p;
}
int main() {
// 在堆区开辟新数据
int* p = func();
cout << *p << endl; // 10
}
1.3 new操作符
- 利用new操作符在堆区开辟数据
- 堆区开辟的数据需利用delete操作符手动释放
int* func() {
// 在堆区创建整形数据
int* p = new int(10); // new返回该数据类型的指针
return p;
}
// new的基本用法
void test01() {
int* p = func();
cout << *p << endl; // 堆区数据由程序员管理开辟,由程序员管理释放
// 如果释放堆区的数据,利用关键字delete
delete p;
//cout << *p << endl; // 内存已经被释放,再次访问是非法操作会报错
}
// 在堆区开辟数组
void test02() {
// 创建是个整形数组
int* arr = new int[10];
for (int i = 0; i < 10; i++) {
arr[i] = i;
}
for (int i = 0; i < 10; i++) {
cout << arr[i] << endl;
}
// 释放堆区数组
delete[] arr;
}
int main() {
test01();
test02();
}
二、引用
2.1 引用的基本使用
int a = 10;
int& b = a;
cout << a << endl; // 10
cout << b << endl; // 10
b = 100; // 操作别名或原名都是操作同一块内存
cout << a << endl; // 100
cout << b << endl; // 100
2.2 引用的注意事项
int a = 10;
//int& b; // 错误,引用必须初始化
int& b = a;
int c = 20;
b = c; // 赋值操作,不是更改引用
cout << a << endl; // 20
cout << b << endl; // 20
cout << c << endl; // 20
2.3 引用做函数参数
//交换函数
// 1. 值传递
void swap01(int a, int b) {
int temp = a;
a = b;
b = temp;
}
// 2. 地址传递
void swap02(int* a, int* b) {
int temp = *a;
*a = *b;
*b = temp;
}
// 3. 引用传递
void swap03(int& a, int& b) {
int temp = a;
a = b;
b = temp;
}
int main() {
int a = 10;
int b = 20;
swap01(a, b); // a = 10, b = 20, 值传递形参修改实参不改
swap02(&a, &b); // a = 20, b = 10; 地址传递形参修饰实参
swap03(a, b); // a = 20, b = 10; 引用传递形参修饰实参
cout << a << endl;
cout << b << endl;
}
2.4 引用做函数返回值
int& test01() {
int a = 10; // 局部变量存放在栈区
return a;
}
int& test02() {
static int a = 20;// 静态变量存放在全局区,全局区上的数据在程序结束后系统释放
return a;
}
int main() {
// 引用做函数返回值
// 1. 不要返回局部变量的引用
int& ref = test01();
cout << ref << endl; // 10 结果正确因为编译器做了保留
cout << ref << endl; // 结果错误因为a的内存已经被释放
int& ref2 = test02();
cout << ref2 << endl; // 20
// 2. 函数的调用可以作为左值
test02() = 1000; // 如果函数返回值是引用,这个函数调用可以作为左值
cout << ref2 << endl; // 1000
}
2.5 引用的本质
- 引用的本质是指针常量,只能改变指向的值,不能改变指向
void func(int& ref) {
ref = 100; // ref 是引用,转换为 *ref = 100
}
int main() {
int a = 10;
int& ref = a; // 自动转换为 int* const ref = &a; 指针指向不可以改,指向的值可以改
ref = 20; // 内部发现ref是引用,自动转换为 *ref = 20;
cout << a << endl; // 20
cout << ref << endl; // 20
func(a);
cout << a << endl; // 100
cout << ref << endl; // 100
return 0;
}
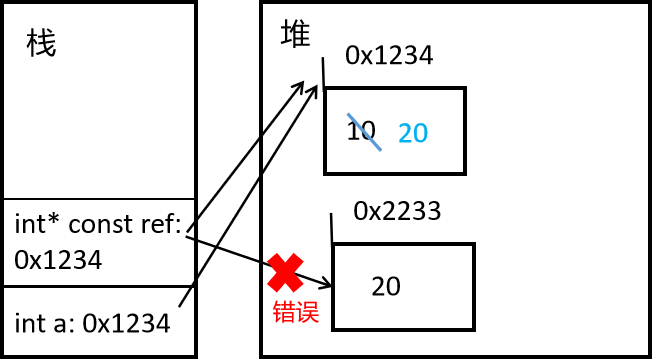
2.6 常量引用
void showValue(const int& val) {
//val = 1000; // 错误,不允许修改 打印防止误操作
cout << val << endl; // 1000
}
int main() {
// 常量引用
// 使用场景:用来修饰形参,防止误操作
int a = 10;
//int& ref = 10; // 错误:10在常量区,引用必须引用一块合法的内存空间
const int& ref = 10; // 加const后编译器将代码修改为 int temp = 10; const int& ref = temp;
//ref = 20; // 错误:加const之后变为只读,不可以修改
int b = 100;
showValue(b);
return 0;
}
三、函数提高
3.1 函数默认参数
- 函数的形参列表中的形参可以有默认值
- 如果某位置已经有了默认参数,那么从这个位置向后的参数都必须有默认值
- 函数的声明和函数的实现只能有一个有默认参数
// 如果传入形参有值则用形参值,如果没有传入形参值则用默认值
int func(int a, int b = 20, int c = 30) {
return a + b + c;
}
// 错误:如果某位置已经有了默认参数,那么从这个位置向后的参数都必须有默认值
int func1(int a, int b = 20, int c = 30, int d) {
return a + b + c + d;
}
// 错误:函数的声明和函数的实现只能有一个有默认参数
int func2(int a = 10, int b = 20);
int func2(int a = 20, int b = 20) {
return a + b;
}
int main() {
cout << func(10) << endl; // 60
cout << func(10, 30) << endl; // 70
cout << func2(10, 10) << endl; // 错误:func2重定义默认参数
system("pause");
return 0;
}
3.2 函数占位参数
- 参数列表中含有占位参数用来占位,调用函数时必须填补该位置
// 目前占位参数还用不到,后面会用到
// 占位参数还可以有默认参数
void func(int a,int) {
cout << "func()" << endl;
}
void func2(int a, int = 10) {
cout << "func2()" << endl;
}
int main() {
//func(10); // 错误
func(10,20);
}
3.3 函数重载
- 函数名相同,提高函数复用性
- 重载条件
- 同一个作用域下
- 函数名相同
- 参数列表不同(参数类型、参数个数、参数顺序不同)
- 注意
- 返回值不作为重载条件
- 引用作为重载条件
- 函数重载碰到默认参数
void func() {
cout << "func()1" << endl;
}
void func(double a) { // 参数类型不同
cout << "func()2" << endl;
}
void func(double b, int a) { // 参数个数不同
cout << "func()3" << endl;
}
void func(int a, double b) { // 参数顺序不同
cout << "func()4" << endl;
}
/*
int func(int a, double b) { // 错误,返回值不同不作为重载条件
cout << "func()4" << endl;
}
*/
int main() {
func(); // func()1
func(3.14); // func()2
func(3.14, 10); // func()3
func(10, 3.14); // func()4
}
// 引用作为重载条件
void func1(int& a) {
cout << "func1()1" << endl;
}
void func1(const int& a) {
cout << "func1()2" << endl;
}
// 函数重载碰到默认参数
void func2(int a) {
cout << "func2()1" << endl;
}
void func2(int a, int b = 10) {
cout << "func2()2" << endl;
}
int main() {
int a = 10;
func1(a); // func1()1, a是变量可读可写,
func1(10); // func1()2,10是常量,调用func1()1的话不合法
func2(10);// 报错:当函数重载遇到默认参数会出现二异性,只能避免
}
四、类和对象
- 面向对象的三大特性:封装、继承、多态
- 万事万物皆对象
4.1 封装
1)封装的意义
- 将属性和行为作为一个整体表现事物,统称为类的成员
- 类的属性称为成员变量、成员属性
- 类的行为称为成员函数、成员方法
// 设计一个圆类,求圆的周长
const double PI = 3.14;
class Circle {
// 访问权限
public:
// 属性
int radius;
// 行为: 获取圆的周长
double perimeter() {
return 2 * PI * radius;
}
};
int main() {
// 创建圆类的对象,实例化:通过一个类创建一个对象的过程
Circle circle;
// 给圆对象的属性进行赋值
circle.radius = 10;
cout << "圆形的周长为:" << circle.perimeter() << endl;
system("pause");
return 0;
}
- 将属性和行为加以权限控制
- public: 成员类内类外都可以访问
- protected: 类内可以访问,类外不可以访问,子类可以访问父类中的保护内容
- private: 类内可以访问,类外不可以访问,子类不可以访问父类中的保护私有内容
class Student {
public:
string name;
int id;
void show() {
cout << name << ", " << id << endl;
}
void setName(string name) {
this->name = name;
}
};
int main() {
Student s1;
s1.setName("张三");
s1.id = 1001;
s1.show();
Student s2;
s2.name = "李四";
s2.id = 1002;
s2.show();
return 0;
}
class Person {
public: // 公共权限
string name;
protected: // 保护权限
string car;
private: // 私有权限
string psw;
public:
void func() { // 类内部三种权限都可以访问
name = "Alice";
car = "Toyota";
psw = "123456";
}
};
int main() {
Person p;
p.name = "Bob";
//p.car = "Benz"; // 错误:保护权限的内容类外不能访问
//p.psw = "234"; // 错误:私有权限的内容类外不能访问
}
2) struct 和 class的区别
- 默认访问权限不同
- struct默认权限为公共
- class默认权限为私有
class C {
int m_C;
};
struct S {
int m_S;
};
int main() {
C c1;
c1.m_C = 100; // 报错:默认权限是私有
S s1;
s1.m_S = 100; // 不报错,默认权限是公共
}
3)成员属性设置为私有
- 自己控制读写权限
- 对于写的权限,可以检测数据的有效性
class Person {
private:
string name; // 只读
int age; // 可读可写
string psw; // 只写
public:
// 只读
string getName() {
return name;
}
// 可读
int getAge() {
age = 0; // 默认年龄
return age;
}
// 可写,控制写入数据有效性
void setAge(int age) {
if (age > 150 || age < 0) {
cout << "写入数据不合法" << endl;
} else {
this->age = age;
}
}
// 只写
void setPsw(string psw) {
this->psw = psw;
}
};
int main() {
Person p;
string p_name = p.getName();
cout << p_name << endl;
p.setAge(200);
p.setAge(18);
int p_age = p.getAge();
cout << p_age << endl;
}
class Cube {
public:
int length;
int width;
int height;
int surface_area() {
return (length * width + width * height + length * height) * 2;
}
int volume() {
return length * width * height;
}
// 使用成员函数判断
bool isEqual(Cube& c2) {
if (height == c2.height && width == c2.width && length == c2.length) {
return true;
} else {
return false;
}
}
};
// 使用全局函数判断
bool isEqual(Cube &c1, Cube &c2) {
if (c1.height == c2.height && c1.width == c2.width && c1.length == c2.length) {
return true;
} else {
return false;
}
}
int main() {
Cube c1;
c1.height = 10;
c1.width = 10;
c1.length = 10;
Cube c2;
c2.height = 10;
c2.width = 10;
c2.length = 10;
int s1 = c1.surface_area();
cout << s1 << endl;
cout << isEqual(c1, c2) << endl;
cout << c1.isEqual(c2) << endl;
}
4)练习:判断点和圆的位置关系
#pragma once
#include<iostream>
using namespace std;
class Point {
public:
int get_x();
int get_y();
void set_x(int x);
void set_y(int y);
private:
int x;
int y;
};
#pragma once
#include<iostream>
using namespace std;
#include "point.h"
class Circle {
public:
int get_radium();
void set_radium(int radium);
Point get_center();
void set_center(Point center);
private:
int radium;
Point center;
};
#include"point.h"
int Point::get_x() {
return x;
}
int Point::get_y() {
return y;
}
void Point::set_x(int x) {
this->x = x;
}
void Point::set_y(int y) {
this->y = y;
}
#include"circle.h"
int Circle::get_radium() {
return radium;
}
void Circle::set_radium(int radium) {
this->radium = radium;
}
Point Circle::get_center() {
return center;
}
void Circle::set_center(Point center) {
this->center = center;
}
#include<iostream>
#include"point.h"
#include"circle.h"
using namespace std;
// 判断点到圆心距离
double getDistance(Point p, Circle c) {
return sqrt((p.get_x() - c.get_center().get_x()) * (p.get_x() - c.get_center().get_x())
+ (p.get_y() - c.get_center().get_y()) * (p.get_y() - c.get_center().get_y()));
}
// 判断点和圆的位置关系
string getPosition(Point p, Circle c) {
double dis = getDistance(p, c);
if (dis == c.get_radium()) {
return "点在圆上";
} else if (dis > c.get_radium()) {
return "点在圆外";
} else {
return "点在圆内";
}
}
int main() {
Point p;
p.set_x(0);
p.set_y(5);
Circle c;
c.set_radium(10);
c.get_center().set_x(0);
c.get_center().set_y(0);
cout << getPosition(p, c) << endl;
}
4.2 对象的初始化和清理
- 生活中电子设备会有出厂设置,以及格式化删除个人数据
- C++中每个对象也有初始设置以及对象销毁前的清理数据设置
1) 构造函数和析构函数
- 编译器会自动调用构造函数和析构函数
- 如果不提供构造函数和析构函数编译器会提供,编译器提供的构造函数和析构函数是空实现
- 构造函数:创建对象时为对象的成员属性赋值,编译器自动调用,无需手动调用
- 类名() {}
- 没有返回值也不写void
- 函数名与类名相同
- 构造函数可以有参数,可以发生重载
- 程序自动调用,无需手动调用,只会调用一次
- 析构函数:用于对象的销毁,系统自动调用,执行清理工作
- ~类名(){}
- 没有返回值也不写void
- 函数名与类名相同,在名称前加~
- 析构函数不可以有参数,不可以发生重载
- 程序在对象销毁前自动调用析构函数,无需手动调用,只会调用一次
class Person {
public:
// 构造函数
Person() {
cout << "Person构造函数的调用" << endl;
}
// 析构函数
~Person() {
cout << "Person析构函数的调用" << endl;
}
};
void test01() {
Person p;
}
int main() {
test01(); // 构造函数和析构函数都会被调用
Person p1; // 只调用构造函数,没有调用析构函数
system("pause");
return 0;
}
2) 构造函数的分类及调用
- 两种分类方式
- 按参数分:有参构造和无参构造
- 按类型分:普通构造和拷贝构造
- 三种调用方式
#include<iostream>
using namespace std;
// 构造函数的分类及调用
class Person {
public:
// 无参构造
Person() {
cout << "Person无参构造函数调用" << endl;
}
// 有参构造
Person(int a) {
age = a;
cout << "Person的有参构造函数调用" << endl;
}
// 拷贝构造函数: 将传入对象p的所有属性拷贝到新对象身上
Person(const Person &p) {
cout << "Person的拷贝构造函数调用" << endl;
age = p.age;
}
~Person() {
cout << "Person构造函数的调用" << endl;
}
int age;
};
// 调用
void test01() {
// 括号法:
Person p1; // 调用无参
Person p2(10); // 调用有参
Person p3(p2); // 拷贝调用
// 注意:调用默认构造函数时候不要加()
Person p4(); // 编译器会认为是函数的声明,不会认为是创造对象
cout << p2.age << endl; // 10
cout << p3.age << endl; // 10
// 显示法
Person p21;
Person p22 = Person(10); // 调用有参
Person p23 = Person(p2); // 调用拷贝
Person(10); // 匿名对象 特点:当前行执行结束后,系统会立即回收掉匿名对象
cout << "aaa" << endl; // aaa会在匿名对象销毁后打印
// 注意:不要利用拷贝构造函数初始化匿名对象
Person(p23); // 报错: Person p3重定义,编译器认为是p3对象的声明 Person p3;
// 隐式转换法
Person p31 = 10; // 有参调用,Person p31 = Person(10);
Person p32 = p31; // 拷贝调用
}
int main() {
test01();
system("pause");
return 0;
}
3) 拷贝构造函数调用时机
- 使用一个已经创建完毕的对象来初始化一个新的对象
- 以值传递的方式给函数参数传值
- 以值方式返回局部对象
class Person {
public:
Person() {
cout << "默认构造" << endl;
}
Person(int age) {
cout << "有参构造" << endl;
}
Person(const Person& p) {
age = p.age;
cout << "拷贝构造" << endl;
}
~Person() {
cout << "析构函数" << endl;
}
int age;
};
// 1. 使用一个已经创建完毕的对象来初始化新对象
void test01() {
Person p1(20);
Person p2(p1);
cout << "p2的年龄为:" << p2.age << endl;
}
// 2. 以值传递的方式给函数的参数传值
void doWork(Person p) {
}
void test02() {
Person p; // 默认构造
doWork(p); // 拷贝构造
}
// 3. 值方式返回局部对象
Person doWork2() {
Person p1;
cout << (int*)&p1 << endl; // 000000C38FAFF774
return p1;
}
void test03() {
Person p = doWork2();
cout << (int*)&p << endl; // 000000C38FAFF774
}
int main() {
test01();
test02();
test03();
system("pause");
return 0;
}
4) 构造函数的调用规则
- 默认情况C++编译器至少给一个类添加3个函数
- 默认构造函数(无参,函数体为空)
- 默认析构函数(无参,函数体为空)
- 默认拷贝构造函数,对属性进行值拷贝
- 构造函数调用规则
- 如果用户定义有参构造函数,C++不会提供默认无参构造,但提供默认拷贝
- 如果用户定义拷贝构造函数,C++不会再提供其他构造函数
5) 深拷贝与浅拷贝
- 浅拷贝:简单的赋值拷贝操作,但会出现堆区内存重复释放的错误
- 如果属性有在堆区间开辟的,一定自己提供拷贝构造函数,防止浅拷贝带来的问题
class Person {
public:
Person(int age, int height) {
this->age = age;
this->height = new int(height); // 堆区开辟数据
cout << "有参构造" << endl;
}
Person(const Person& p) {
this->age = p.age;
this->height = p.height; // 浅拷贝
cout << "拷贝构造" << endl;
}
~Person() {
// 析构代码,将堆区开辟的数据释放
if (height != NULL) {
delete height;
height = NULL;
}
cout << "析构函数" << endl;
}
int age;
int* height; // 把身高数据开辟到堆区
};
void test() {
Person p1(20, 160);
cout << p1.age << "," << *p1.height << endl;
Person p2(p1);
cout << p2.age << "," << *p2.height << endl; // 堆区的内存重复释放
}
// p1,p2指向相同的存放相同堆区地址的height变量
// p1, p2存放在栈区,释放时p2先被释放,p2释放时会将存在堆区的height变量释放置空
// 接着p1被释放时,指向的堆区height变量已经被置空,堆区内存重复被释放报错
int main() {
test();
system("pause");
return 0;
}
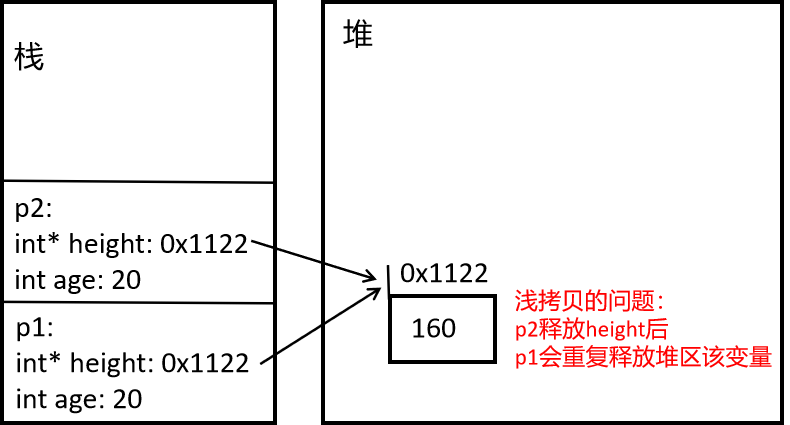
Person(const Person& p) {
cout << "Person拷贝构造函数调用" << endl;
this->age = p.age;
//this->height = p.height; // 浅拷贝,编译器默认
// 深拷贝操作
this->height = new int(*p.height);
}
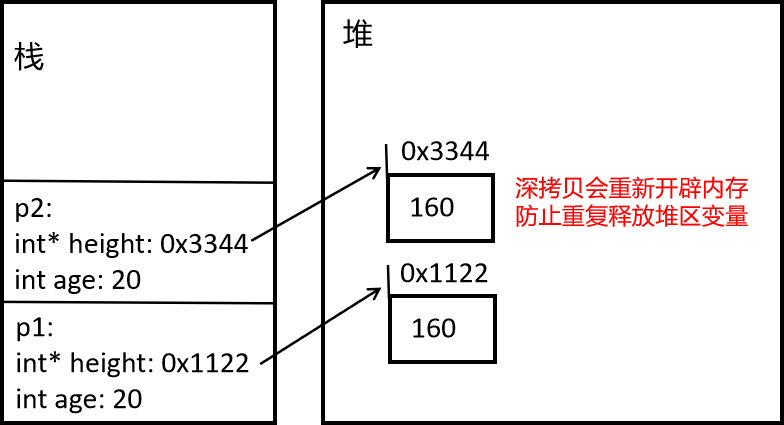
6) 初始化列表
class Person {
public:
// 初始化列表初始化属性
Person() : m_A(10), m_B(20), m_C(30) {
// 实现体
}
Person(int a, int b, int c) : m_A(a), m_B(b), m_C(c) {
// 实现体
}
int m_A;
int m_B;
int m_C;
};
void test() {
Person p1;
Person p2(30, 20, 10);
}
int main() {
test();
return 0;
}
7) 类对象作为类的成员
- 类中的成员可以是另一个类的对象,则称该成员为对象成员
- B类中有对象A作为成员,A为对象成员
class A {}
class B {
A a;
}
- 当其他类对象作为本类成员,构造时先构造其他类对象再构造自身,析构与构造顺序相反
#include<iostream>
using namespace std;
#include<string>
class Phone {
public:
Phone(string brand) {
m_brand = brand;
cout << "Phone构造" << endl;
}
~Phone() {
cout << "Phone析构" << endl;
}
string m_brand;
};
class Person {
public:
Person(string name, string brand) :m_name(name), m_phone(brand) { // Phone m_brand = brand; 隐式转换法
cout << "Person构造" << endl;
}
~Person() {
cout << "Person析构" << endl;
}
string m_name;
Phone m_phone;
};
void test01() {
Person p("Alice", "iPhone");
cout << p.m_name << "用着" << p.m_phone.m_brand << endl;
}
int main() {
test01();
return 0;
}
// Phone构造
// Person构造
// Alice用着iPhone
// Person析构
// Phone析构
8) 静态成员
- 成员变量和成员函数前加static,称为静态成员
- 分类
- 静态成员变量
- 所有对象共享同一份数据
- 在编译阶段分配内存
- 类的声明,类的初始化
- 静态成员函数
- 所有对象共享一个函数
- 静态成员函数只能访问静态成员变量
class Person {
public:
// 静态成员函数
static void func() {
m_A = 100; // 静态成员函数可以访问静态成员变量,因为m_A不属于某个特定对象
//m_B = 200; // 错误:静态成员函数不可以访问非静态成员变量,无法区分哪个对象的m_B属性
cout << "public static void func调用" << endl;
}
static int m_A; // 静态成员变量,类内声明
int m_B; // 非静态成员变量
// 静态成员函数有访问权限
private:
static void fun2() {
cout << "private static void func调用" << endl;
}
};
int Person::m_A = 0; // 静态成员变量类外初始化
void test01() {
// 静态成员变量两种访问方式:
// 1. 通过对象访问
Person p;
p.func();
// 2. 通过类名访问
Person::func();
//Person::func2(); // 错误:类外访问不到私有静态成员函数
}
4.3 C++对象模型和this指针
1) 成员变量和成员函数分开存储
- C++中类的成员变量和成员函数分开存储
- 只有非静态成员变量才属于类的对象上
- 每个非静态成员函数只有一份成员实例,多个同类型对象会公用一块代码
#include<iostream>
using namespace std;
class Person {
int m_A; // 非静态成员变量,属于类的对象上
static int m_B; // 静态成员变量,不属于类的对象上
void func() {} // 非静态成员函数,不属于类的对象上
static void func2() {} // 静态成员函数,不属于类的对象上
};
int Person::m_B = 10;
void test01() {
Person p;
// C++编译器会给每个空对象分配一个字节空间,是为了区分空对象占内存的位置
// 每个空对象也应有一个独一无二的内存地址
cout << sizeof(p) << endl; // 空对象占用的内存空间为:1
}
void test02() {
Person p;
cout << sizeof(p) << endl; // 4,有无其他不属于类的对象上的变量/函数都是4,按照int分配内存
}
int main() {
test02();
system("pause");
return 0;
}
2) this指针概念
- this指针指向被调用的成员函数所属的对象
- this指针隐含在每一个非静态的成员函数内
- this指针不需要定义,直接用即可
- 用途:
- 当形参和成员变量同名时,用this来区分成员变量
- 在类的非静态成员函数中返回对象本身,可使用return *this
class Person {
public:
// this指向被调用的成员函数(p1)所属的成员对象(p1.age)
Person(int age) {
this->age = age;
}
// 返回对象本身用*this
Person& addAge(Person& p) {
this->age += p.age;
return *this; // this是指向p2的指针,*this是指向p2这个对象的实体
}
Person addAge1(Person& p) { // 返回的不是引用,每次都会返回一个新对象
this->age += p.age;
return *this;
}
int age;
};
void test01() {
Person p1(18);
cout << p1.age << endl;
}
void test02() {
Person p1(10);
Person p2(10);
p2.addAge(p1).addAge(p1).addAge(p1); // 链式编程
cout << p2.age << endl; // 40
p2.age = 10;
p2.addAge1(p1).addAge1(p1).addAge1(p1); // 链式编程
cout << p2.age << endl; // 20
}
int main() {
test02();
return 0;
3) 空指针访问成员函数
- 空指针可以调用成员函数,需要注意有没有用到this指针
class Person {
public:
void showClassName() {
cout << "This is a Person class" << endl;
}
void showPersonAge() {
if (this == NULL) return;
cout << m_age << endl; // 默认为this->m_age,this没有指向确切的数据, 更没有对应的属性
}
int m_age;
};
void test01() {
Person* p = NULL;
p->showClassName();
p->showPersonAge(); // 读取访问权限冲突
}
int main() {
test01();
return 0;
}
4)const修饰成员函数
- 常函数
- 成员函数后加const
- 常函数内不可以修改成员属性,(常字限定只读)
- 成员属性声明时加关键字mutable后,在常函数中依然可以修改
- 常对象
// 常函数
class Person {
public:
// Person* const this; this指针的本质是指针常量,指针指向不可以修改
// const Person* const this; 此时指针指向的值也不可修改
// 在成员函数后面加const修饰的是this指向,让指针指向的值也不可修改
void showPerson() const { // 加const后不可以修改m_A的值
//this->m_A = 100;
//this = NULL; // 错误,指针指向不可修改
this->m_B = 100;
}
void func() {
m_A = 100; // 普通函数中可以修改属性
}
int m_A;
mutable int m_B; // 加mutable后即使在常函数中也可以修改这个值
};
void test01() {
Person p;
p.showPerson();
}
// 常对象
void test02() {
const Person p; // 在对象前加const,变为常对象,不允许修改指针指向的值
//p.m_A = 100; // 错误
p.m_B = 200; // mutable修饰后,在常对象下可以修改
// 常函数和常对象只能调用常函数
p.showPerson();
//p.func(); // 调用普通函数报错,因为普通函数中可以修改属性,而常对象/常函数不允许修改普通属性
}
4.4 友元
- 让函数或者类访问另一个类中私有成员
- 关键字:friend
- 三种实现:
class Building {
// 全局函数做友元
friend void goodFriend(Building* building);
public:
Building() {
m_diningRoom = "客厅";
m_bedroom = "卧室";
}
string m_diningRoom;
private:
string m_bedroom;
};
// 全局函数
void goodFriend(Building *building) {
cout << "正在访问:" << building->m_diningRoom << endl;
cout << "正在访问:" << building->m_bedroom << endl;
}
void test01() {
Building building;
goodFriend(&building);
}
int main() {
test01();
}
class Building;
class GoodFriend {
public:
GoodFriend();
void visit(); // 参观函数访问Buidling中的属性
Building* building;
};
class Building {
// 类做友元
friend class GoodFriend;
public:
Building();
string m_diningRoom;
private:
string m_bedroom;
};
// 类外写成员函数
Building::Building() {
m_diningRoom = "客厅";
m_bedroom = "卧室";
}
GoodFriend::GoodFriend() {
// 创建建筑物对象
building = new Building;
}
void GoodFriend::visit() {
cout << "GoodFriend正在访问:" << building->m_diningRoom << endl;
cout << "GoodFriend正在访问:" << building->m_bedroom << endl;
}
void test01() {
GoodFriend gf;
gf.visit();
}
int main() {
test01();
return 0;
}
class Building;
class GoodFriend {
public:
GoodFriend();
void visit(); // 访问Building的私有成员
void visit2(); // 不可以访问Building的私有成员
Building* building;
};
class Building {
// 成员函数做友元
friend void GoodFriend::visit();
public:
Building();
string m_diningRoom;
private:
string m_bedroom;
};
Building::Building() {
m_diningRoom = "客厅";
m_bedroom = "卧室";
}
GoodFriend::GoodFriend() {
building = new Building();
}
void GoodFriend::visit() {
cout << "GoodFriend::visit()正在访问:" << building->m_diningRoom << endl;
cout << "GoodFriend::visit()正在访问:" << building->m_bedroom << endl;
}
void GoodFriend::visit2() {
cout << "GoodFriend::visit2()正在访问:" << building->m_diningRoom << endl;
//cout << "GoodFriend::visit()正在访问:" << building->m_bedroom << endl; // 报错
}
void test01() {
GoodFriend gf;
gf.visit();
gf.visit2();
}
int main() {
test01();
return 0;
}
4.5 运算符重载
- 对已有元素安抚进行重新定义赋予其另一个种功能,以适应不同的数据类型
- 运算符重载也可以发生函数重载
1)加号运算符重载
// 加号运算符重载
class Person {
public:
// 1. 成员函数重载
Person operator+(Person& p) {
Person temp;
temp.m_A = this->m_A + p.m_A;
temp.m_B = this->m_B + p.m_B;
return temp;
}
int m_A;
int m_B;
};
// 2. 全局函数重载
Person operator+ (Person& p1, Person& p2) {
Person temp;
temp.m_A = p1.m_A + p2.m_A;
temp.m_B = p1.m_B + p2.m_B;
return temp;
}
// 函数重载版本
Person operator+ (Person& p1, int num) {
Person temp;
temp.m_A = p1.m_A + num;
temp.m_B = p1.m_B + num;
return temp;
}
void test01() {
Person p1;
p1.m_A = 10;
p1.m_B = 20;
Person p2;
p2.m_A = 20;
p2.m_B = 10;
Person p3 = p1 + p2;
// 成员函数本质:Person p3 = p1.operator+(p2);
// 全局函数本质:Person p3 = operator+(p1, p2);
cout << p3.m_A << ", " << p3.m_B << endl;
// 运算符重载也可以发生函数重载
Person p4 = p1 + 100;
cout << p4.m_A << ", " << p4.m_B << endl;
}
int main() {
test01();
return 0;
}
2) 左移运算符重载
class Person {
friend ostream& operator<<(ostream& cout, Person& p);
public:
Person(int a, int b) {
m_A = a;
m_B = b;
}
private:
// 成员函数重载左移运算符
// 不会利用成员函数重载左移运算符,因为难以实现 cout << p
//void operator<<() {}
int m_A;
int m_B;
};
// 全局函数重载左移运算符
// 本质: operator<< (cout, p) 简化为: cout << p
ostream& operator<<(ostream& cout, Person& p) {
cout << "m_A = " << p.m_A << ", m_B = " << p.m_B;
return cout;
}
void test01() {
Person p(10, 10);
cout << p << endl;
}
int main() {
test01();
system("pause");
return 0;
}
3) 递增递减运算符重载
// 自定义整型
class MyInteger {
friend ostream& operator<< (ostream& cout, MyInteger myInt);
public:
MyInteger() {
m_num = 0;
}
// 重载++a运算符
MyInteger& operator++() {
// 返回引用为了一直对一个数据对象进行操作
m_num++;
return *this; //将自身返回
}
// 重载a++运算符
// int代表占位参数,用于区分前置递增和后置递增
MyInteger operator++(int) {
// 后置返回值,不返回引用
// 后置如果返回引用,返回的是局部变量的引用,局部对象在函数执行结束后会被释放
MyInteger temp = *this;
m_num++;
return temp;
}
private:
int m_num;
};
// 重载左移运算符
ostream& operator<< (ostream& cout, MyInteger myInt) {
cout << myInt.m_num;
return cout;
}
void test01() {
MyInteger myInt;
cout << ++(++myInt) << endl; // 2
cout << myInt << endl; // 2, 如果重写函数返回的是值,这里myInt的值会是1
}
void test02() {
MyInteger myInt;
cout << myInt++ << endl; // 0
cout << myInt << endl; // 1
}
int main() {
test01();
test02();
return 0;
}
class MyInteger2 {
friend ostream& operator<< (ostream& cout, MyInteger2 myInt);
public:
MyInteger2() {
m_num = 100;
}
// --a
MyInteger2& operator--() {
m_num--;
return *this;
}
// a--
MyInteger2 operator--(int) {
MyInteger2 temp = *this;
m_num--;
return temp;
}
private:
int m_num;
};
ostream& operator<< (ostream& cout, MyInteger2 myInt) {
cout << myInt.m_num;
return cout;
}
void test01() {
MyInteger2 myInt;
cout << --myInt << endl; // 99
cout << myInt-- << endl; //99
cout << myInt << endl; //98
}
int main() {
test01();
return 0;
}
4) 赋值运算符重载
- C++编译器至少给一个类添加4个函数
- 默认构造函数
- 默认析构函数
- 默认拷贝函数
- 赋值运算符 operator=, 属性进行赋值拷贝
class Person {
public:
Person(int age) {
m_age = new int(age); // 年龄真实数据存在堆区,需要手动释放
}
~Person() {
if (m_age != NULL) {
delete m_age;
m_age = NULL;
}
}
// 重载赋值运算符
Person& operator=(Person& p) {
// 如果返回Person,相当于按照自身调用拷贝构造返回一个新的副本
// 只有返回Person& 才是返回对象本身
// 编译器提供浅拷贝,堆区内存重复释放,用深拷贝解决浅拷贝的问题
// m_age = p.m_age;
// 应该先判断是否有属性在堆区,如果有先释放干净,然后深拷贝
if (m_age != NULL) {
delete m_age;
m_age = NULL;
}
// 深拷贝
m_age = new int(*p.m_age);
return *this; // 返回对象本体
}
int* m_age;
};
void test01() {
Person p1(18);
Person p2(20);
Person p3(30);
p3 = p2 = p1; // 赋值操作
cout << *p1.m_age << endl;
cout << *p2.m_age << endl;
cout << *p3.m_age << endl;
}
int main() {
test01();
int a = 10;
int b = 20;
int c = 30;
c = b = a;
cout << a << ", " << b << ", " << c << endl; // 10, 10, 10
return 0;
}
5) 关系运算符重载
class Person {
public:
Person(string name, int age) {
m_name = name;
m_age = age;
}
// 重载==
bool operator==(Person& p2) {
if (this->m_name == p2.m_name && this->m_age == p2.m_age) {
return true;
} else return false;
}
// 重载!=
bool operator!=(Person& p2) {
if (this->m_name == p2.m_name && this->m_age == p2.m_age) {
return false;
} else return true;
}
string m_name;
int m_age;
};
void test01() {
Person p1("Tom", 19);
Person p2("Tom", 19);
if (p1 == p2) {
cout << "p1 == p2" << endl;
} else {
cout << "p1 != p2" << endl;
}
}
int main() {
test01();
return 0;
}
6) 函数调用运算符重载
- 函数调用运算符(),也成为仿函数,没有固定写法,非常灵活
// 打印输出类
class MyPrint {
public:
// 重载函数调用运算符
void operator()(string test) {
cout << test << endl;
}
};
void myPrint01(string test) {
cout << test << endl;
}
void test01() {
MyPrint myPrint;
myPrint("Hello World!"); // 重载(),由于使用时类似函数调用,因此称为仿函数
myPrint01("Hello World!"); // 函数调用
}
// 仿函数非常灵活,没有固定写法
// 加法类
class MyAdd {
public:
int operator()(int num1, int num2) {
return num1 + num2;
}
};
void test02() {
MyAdd myAdd;
int result = myAdd(100, 200); // 仿函数
cout << result << endl;
// 匿名函数对象: 类名(),匿名对象当前行执行完内存立即被释放
cout << MyAdd()(100, 200) << endl;
}
int main() {
test01();
test02();
return 0;
}
4.6 继承
1) 继承的基本语法
- 减少重复代码
- 语法:class 子类 : 继承方式 父类名
- 子类也成为派生类,父类也称为基类,父类体现共性,子类体现个性
class BasePage {
public:
void header() {
cout << "public head" << endl;
}
void footer() {
cout << "public footer" << endl;
}
void left() {
cout << "public left" << endl;
}
};
class Java : public BasePage {
public:
void content() {
cout << "Java Content" << endl;
}
};
class Python : public BasePage {
public:
void content() {
cout << "Python Content" << endl;
}
};
class CPP : public BasePage {
public:
void content() {
cout << "CPP Content" << endl;
}
};
void test01() {
Java jv;
jv.header();
jv.content();
jv.left();
jv.footer();
cout << "----------------" << endl;
Python py;
py.header();
py.content();
py.left();
py.footer();
cout << "----------------" << endl;
CPP cpp;
cpp.header();
cpp.content();
cpp.left();
cpp.footer();
cout << "----------------" << endl;
}
int main() {
test01();
return 0;
}
2) 继承方式
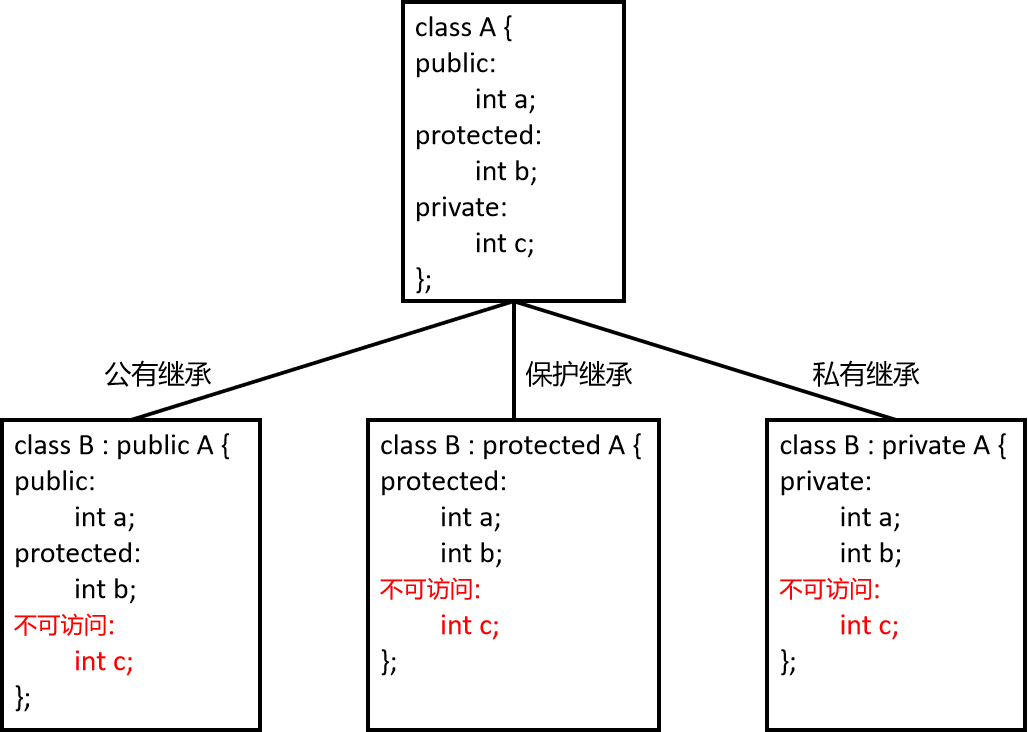
class Base1 {
public:
int m_a;
protected:
int m_b;
private:
int m_c;
};
// 公共继承
class Son1 :public Base1 {
public:
void func() {
m_a = 10;
m_b = 10;
//m_c = 10; // 父类中私有成员子类无法访问
}
};
// 保护继承
class Son2 :protected Base1 {
public:
void func() {
m_a = 10; // 父类中public和protected权限内容到子类中都变为protected
m_b = 10;
//m_c = 10; // 父类中私有成员子类无法访问
}
};
// 私有继承
class Son3 :private Base1 {
public:
void func() {
m_a = 100; // 父类中public和protected权限内容到子类中都变为private
m_b = 100;
//m_c = 10; // 父类中私有成员子类无法访问
}
};
class GrandSon3 :private Son3 {
public:
void func() {
//m_a = 100; // 父类中private权限内容到子类无法访问
//m_b = 100;
}
};
void test21() {
Son1 s1;
s1.m_a = 100;
//s1.m_b = 100; // 在Son1中m_b属于protected权限,类外无法访问
Son2 s2;
//s2.m_a = 100; // 在Son2中m_a属于protected权限,类外无法访问
//s2.m_b = 100; // 在Son2中m_b属于protected权限,类外无法访问
Son3 s3;
//s3.m_a = 100; // 在Son3中m_a属于private权限,类外无法访问
}
3) 继承中的对象模型
class Base {
public:
int m_a;
protected:
int m_b;
private:
int m_c;
};
class Son :public Base {
public:
int m_d;
};
void test01() {
cout << sizeof(Son) << endl; // 16
// 在父类中所有的非静态成员属性都会被子类继承
// 父类中私有成员属性是被编译器隐藏了,因此访问不到,但确实被继承下去了
}
- 利用开发人员命令提示工具查看对象模型
- 跳转盘符 D:
- 跳转文件路径 cd 具体路径
- 查看命名 cl /d1 reportSingleClassLayout类名 ”文件名.cpp“
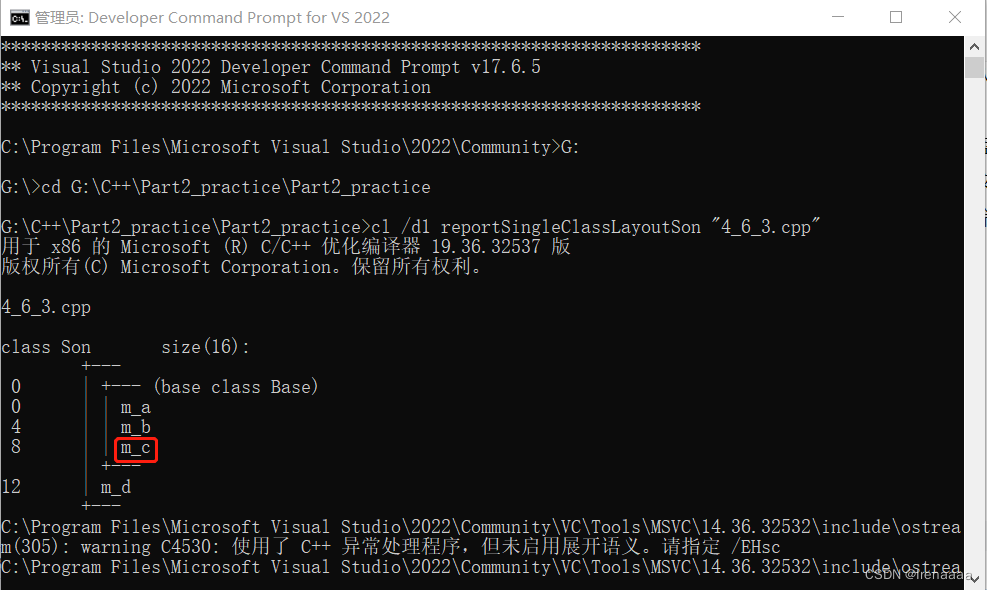
4) 继承中构造和析构顺序
- 构造顺序:先构造父类,再构造子类
- 析构顺序:与构造顺序相反
class Base {
public:
Base() {
cout << "Base构造" << endl;
}
~Base() {
cout << "Base析构" << endl;
}
};
class Son :public Base {
public:
Son() {
cout << "Son构造" << endl;
}
~Son() {
cout << "Son析构" << endl;
}
};
void test() {
Son s1;
}
int main() {
test();
return 0;
}
//Base构造
//Son构造
//Son析构
//Base析构
5) 继承中同名的成员处理方式
- 通过子类对象访问子类同名成员,直接访问
- 通过子类对象访问父类同名成员,需要加作用域
- 子类中与父类同名成员函数会隐藏所有父类中的同名函数,需要加作用域访问父类同名函数
class Base {
public:
Base() {
m_a = 100;
}
void func() {
cout << "Base::func()" << endl;
}
void func(int a) {
cout << "Base::func(int a)" << endl;
}
int m_a;
};
class Son : public Base {
public:
Son() {
m_a = 200;
}
void func() {
cout << "Son::func()" << endl;
}
int m_a;
};
void test() {
Son s;
// 子类对象访问父类对象中同名成员属性需要加作用域
cout << s.m_a << endl; // 200
cout << s.Base::m_a << endl; // 100
// 子类中出现与父类同名成员函数,会隐藏所有父类中的同名函数
// 需要加作用域访问父类中被隐藏的同名函数
s.func(); // "Son::func()"
s.Base::func(); // "Base::func()"
//s.func(100); // 错误
s.Base::func(100); // "Base::func(int a)"
}
int main() {
test();
return 0;
}
6) 继承中同名静态成员处理方法
- 静态成员与非静态成员同名时处理方式一致
- 访问子类同名成员,直接访问即可
- 访问父类同名成员,需要加作用域
- 子类出现和父类同名的静态成员函数,也会隐藏父类所有成员函数,需要加作用域调用
class Base {
public:
static int m_a; // 类内声明,类外初始化
static void func() {
cout << "Base::func()" << endl;
}
};
class Son : public Base{
public:
static int m_a; // 类内声明,类外初始化
static void func() {
cout << "Son::func()" << endl;
}
};
int Base::m_a = 100;
int Son::m_a = 200;
void test() {
// 通过对象访问静态对象
Son s;
cout << s.m_a << endl; // 200
cout << s.Base::m_a << endl; // 100
// 通过类名访问静态对象
cout << Son::m_a << endl; // 200
cout << Son::Base::m_a << endl; // 200
// 通过对象访问静态函数
s.func();
s.Base::func();
// 通过类名访问静态函数
Son::func();
Son::Base::func();
}
int main() {
test();
return 0;
}
7) 多继承语法
- C++允许一个类继承多个类
- 实际开发中不建议使用多继承
class Base1 {
public:
Base1() {
m_a = 100;
}
int m_a;
};
class Base2 {
public:
Base2() {
m_a = 200;
}
int m_a;
};
class Son : public Base1, public Base2 {
public:
Son() {
m_c = 300;
m_d = 400;
}
int m_c;
int m_d;
};
void test() {
Son s;
cout << sizeof(s) << endl; // 16
//cout << s.m_a << endl; // 错误: 二异性,当父类们中出现同名成员调用时需要添加作用域
cout << s.Base1::m_a << endl; // 100
cout << s.Base2::m_a << endl; // 200
}
int main() {
test();
return 0;
}
8) 菱形继承
- 两个派生类继承同一个基类,又有某个类同时继承这两个派生类,这种继承称为菱形继承
- 虚继承:解决菱形继承的问题,在继承之前加关键字virtual变为虚继承,Animal称为虚基类
- vbptr:virtual base pointer 虚基类指针,指向vbtable(virtual base table)虚基类表,两个指针通过偏移量找到唯一数据
- 虚继承前
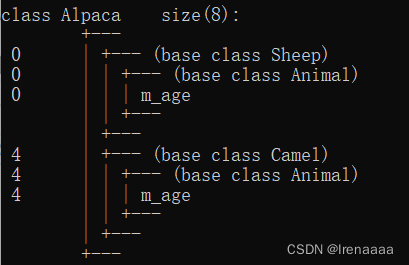
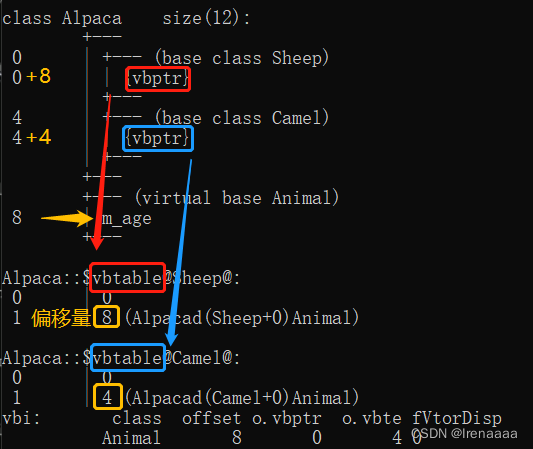
class Animal {
public:
int m_age;
};
// 利用虚继承,解决菱形继承的问题
// 在继承之前加关键字virtual变为虚继承,Animal称为虚基类
class Sheep :virtual public Animal {};
class Camel :virtual public Animal {};
class Alpaca :public Sheep, public Camel {};
void test() {
Alpaca alpaca;
alpaca.Sheep::m_age = 18;
alpaca.Camel::m_age = 20;
// 菱形继承相当于两个父类拥有相同数据,需要使用作用域加以区分
// 但这份数据我们只需要一份,菱形数据导致了资源浪费
cout << alpaca.Sheep::m_age << endl; // 虚继承前:18,使用虚继承后:20
cout << alpaca.Camel::m_age << endl; // 虚继承前:20,使用虚继承后:20
cout << alpaca.m_age << endl; // 虚继承前:无法访问,使用虚继承后:20
}
int main() {
test();
return 0;
}
4.7 多态
1) 多态的基本概念
- 分类
- 静态多态:函数重载和运算符重载,复用函数名
- 动态多态:原生类和虚函数实现运行时多态
- 动态多态的区别:
- 静态多态的函数地址早绑定,编译阶段确定函数地址
- 动态多态的函数地址晚绑定,运行阶段确定函数地址
- 动态多态满足条件:
- 有继承关系
- 子类重写父类虚函数
- 动态多态的使用
- 父类的指针或引用指向子类对象
class Animal {
public:
// 虚函数speak()进行地址晚绑定,实现多态
virtual void speak() {
cout << "Animals can speak." << endl;
}
// 非静态成员函数,不属于类的对象
// void speak() {
// cout << "Animals can speak." << endl;
//}
};
class Cat :public Animal {
public:
void speak() {
cout << "Cats can speak." << endl;
}
};
// 地址早绑定:在编译阶段就确定函数地址,多态需要地址晚绑定,在运行阶段绑定函数
void doSpeak(Animal &animal) { // 父类的指针或引用指向子类对象
animal.speak();
}
void test01() {
Cat cat;
doSpeak(cat);
// Animal类中的speak()没有virtual,没有动态绑定,会打印Animal类中的doSpeak()
}
void test02() {
cout << sizeof(Animal) << endl; // 无virtual:1,有virtual:8
}
int main() {
test01();
test02();
return 0;
}
2) 多态案例1——计算器类
- 多态优点
- 代码组织结构清晰
- 可读性强
- 利于前期和后期的扩展以及维护
// 普通写法
class Calculator {
public:
int getResult(string op) {
if (op == "+") {
return m_num1 + m_num2;
} else if (op == "-") {
return m_num1 - m_num2;
} else if (op == "*") {
return m_num1 * m_num2;
}
// 如果扩展新功能,需要修改源码
// 开闭原则:对扩展进行开发,对修改进行关闭
}
int m_num1;
int m_num2;
};
void test01() {
Calculator c;
c.m_num1 = 10;
c.m_num2 = 20;
cout << c.getResult("+") << endl;
cout << c.getResult("-") << endl;
cout << c.getResult("*") << endl;
}
// 利用多态实现
// 计算器抽象类
class AbstractCalculator {
public:
virtual int getResult() {
return 0;
}
int m_num1;
int m_num2;
};
// 加法计算器
class Add : public AbstractCalculator {
public:
virtual int getResult() {
return m_num1 + m_num2;
}
};
// 减法计算器
class Sub : public AbstractCalculator {
public:
virtual int getResult() {
return m_num1 - m_num2;
}
};
// 乘法计算器
class Mul : public AbstractCalculator {
public:
virtual int getResult() {
return m_num1 * m_num2;
}
};
void test02() {
// 父类指针指向子类对象
AbstractCalculator* ac = new Add;
ac->m_num1 = 10;
ac->m_num2 = 10;
cout << ac->getResult() << endl;
// 使用后进行销毁
delete ac;
ac = new Sub;
ac->m_num1 = 10;
ac->m_num2 = 10;
cout << ac->getResult() << endl;
delete ac;
ac = new Mul;
ac->m_num1 = 10;
ac->m_num2 = 10;
cout << ac->getResult() << endl;
delete ac;
}
int main() {
test02();
return 0;
}
3) 纯虚函数和抽象类
- 纯虚函数
- 在父类中的虚函数通常没有意义,主要调用子类重写的内容
- 纯虚函数写法:virtural 返回值类型 函数名(参数列表) = 0;
- 当类中有了一个纯虚函数,则这个类称为抽象类
- 抽象类特点:
- 无法实例化对象
- 子类必须重写抽象类中的纯虚函数,否则也属于抽象类
class Base {
public:
// 抽象类:无法实例化对象
virtual void func() = 0;
};
// 抽象类的子类必须重写父类中的纯虚函数,否则子类也是抽象类
class Son : public Base {
public:
virtual void func() {
cout << "Son::func()" << endl;
};
};
void test01() {
//Base b; // 抽象类无法实例化
//new Base; // 抽象类无法实例化
Base* base = new Son;
base->func();
}
int main() {
test01();
return 0;
}
4) 多态案例2——制作饮品
class AbstractDrinking {
public:
virtual void Boil() = 0;
virtual void Brew() = 0;
virtual void PourInCup() = 0;
virtual void PutSomething() = 0;
void makeDrinking() {
Boil();
Brew();
PourInCup();
PutSomething();
}
};
class Coffee :public AbstractDrinking {
public:
void Boil() {
cout << "煮农夫山泉" << endl;
}
void Brew() {
cout << "煮咖啡" << endl;
}
void PourInCup() {
cout << "倒入咖啡杯" << endl;
}
void PutSomething() {
cout << "加入咖啡伴侣" << endl;
}
};
class Tea :public AbstractDrinking {
public:
void Boil() {
cout << "煮矿泉水" << endl;
}
void Brew() {
cout << "冲茶叶" << endl;
}
void PourInCup() {
cout << "倒入茶杯" << endl;
}
void PutSomething() {
cout << "加入枸杞" << endl;
}
};
void doWork(AbstractDrinking* abs) {
abs->makeDrinking();
delete abs; // 堆区数据手动释放
}
void test01() {
doWork(new Coffee); // AbstractDrinking* abs = new Coffee;
doWork(new Tea);
}
int main() {
test01();
return 0;
}
5) 虚析构和纯虚析构
#include<iostream>
using namespace std;
// 零件
class Cpu {
public:
virtual void calculate() = 0;
};
class VideoCard {
public:
virtual void display() = 0;
};
class Memory {
public:
virtual void storage() = 0;
};
// 电脑
class Computer {
public:
Computer(Cpu* cpu, VideoCard* vc, Memory* memory) {
m_cpu = cpu;
m_vc = vc;
m_memory = memory;
}
void work() {
m_cpu->calculate();
m_vc->display();
m_memory->storage();
}
~Computer() {
if (m_cpu != NULL) {
delete m_cpu;
}
if (m_vc != NULL) {
delete m_vc;
}
if (m_memory != NULL) {
delete m_memory;
}
m_cpu = NULL;
m_vc = NULL;
m_memory = NULL;
}
private:
Cpu* m_cpu; // Cpu零件的指针
VideoCard* m_vc;
Memory* m_memory;
};
// 厂商
class IntelCpu : public Cpu {
public:
void calculate() {
cout << "Intel Cpu" << endl;
}
};
class IntelVc: public VideoCard {
public:
void display() {
cout << "Intel VideoCard" << endl;
}
};
class IntelMemory : public Memory {
public:
void storage() {
cout << "Intel Memory" << endl;
}
};
class LenovoCpu : public Cpu {
public:
void calculate() {
cout << "Lenovo Cpu" << endl;
}
};
void test() {
// 创建零件
Cpu* lenovoCpu = new LenovoCpu; // 零件在堆区创建
VideoCard* intelVc = new IntelVc;
Memory* intelMem = new IntelMemory;
// 创建电脑
Computer* computer = new Computer(lenovoCpu, intelVc, intelMem); // 电脑在堆区创建
//电脑共工作
computer->work();
delete computer;
Computer* computer2 = new Computer(new IntelCpu, new IntelVc, new IntelMemory); // 电脑在堆区创建
computer2->work();
delete computer2;
}
int main() {
test();
return 0;
}
五、文件操作
- 程序运行时产生的数据都是临时数据,程序一旦运行结束都被释放,通过文件可将数据持久化
- 文件操作需要包含头文件<fstream>
- 文件类型
- 文本文件:文件以文本的ASKII码习惯是存储在计算机中
- 二进制文件:文件以文本的二进制型式存储在计算机中,用户一般不能直接读懂它们
- 操作文件的三大类
- ofstream:写操作
- ifstream:读操作
- fstream:读写操作
5.1 文本文件
1) 写文件
- 具体步骤
- 包含头文件:#include<fstream>
- 创建流对象:ofstream ofs;
- 打开文件:ofs.open{"文件路径", 打开方式};
- 写数据:ofs << "写入的数据";
- 关闭文件:ofs.close();
- 文件打开方式
打开方式 | 解释 |
---|
ios::in | 为读文件而打开文件 |
ios::out | 为写文件而打开文件 |
ios::ate | 初始位置:文件尾 |
ios::app | 追加方式写文件 |
ios::trunc | 如果文件存在先处理,再创建 |
ios::binary | 二进制文件 |
- 文件打开方式可以配合使用,利用 | 操作符
- 用二进制方式写文件:ios::binary | ios::out
#include<iostream>
using namespace std;
#include<fstream>
// 文本文件 写文件
void test01() {
// 1. 包含头文件fstream
// 2. 创建流对象
ofstream ofs; // output file stream
// 3. 指定打开的方式
ofs.open("test.txt", ios::out); // 如果不指定路径,默认为与项目相同路径的文件夹下
// 4, 写内容
ofs << "姓名:张三" << endl;
ofs << "性别:男" << endl;
ofs << "年龄:18" << endl;
// 5. 关闭文件
ofs.close();
}
int main() {
test01();
return 0;
}
- 文件操作必须包含头文件fstream
- 读文件可以利用ofstream或者fstream类
- 打开文件时需要指定操作文件的路径以及打开方式
- 利用<<可以向文件内写数据
- 操作完毕要关闭文件
2) 读文件
- 具体步骤
- 包含头文件:#include<fstream>
- 创建流对象:ifstream ifs;
- 打开文件并判断文件是否打开成功:ifs.open("文件路径", 打开方式);
- 读数据:四种方式读取
- 关闭文件:ifs.close();
#include<iostream>
using namespace std;
#include<fstream>
#include<string>
void test02() {
// 1. 包含头文件
// 2. 创建流对象
ifstream ifs;
// 3. 打开文件,并判断文件是否打开成功
ifs.open("test.txt", ios::in);
if (!ifs.is_open()) {
cout << "文件打开失败" << endl;
return;
}
// 4. 读数据
// 第一种方式
char buf[1024] = { 0 };
while (ifs >> buf) { // 读不到数据回返回false
cout << buf << endl;
}
// 第二种方式
char buf[1024] = { 0 };
while (ifs.getline(buf, sizeof(buf))) {
cout << buf << endl;
}
// 第三种方式
string buf;
while (getline(ifs, buf)) {
cout << buf << endl;
}
// 第四种方式
char c;
while ((c = ifs.get())!= EOF) { // End of the File
cout << c;
}
// 5. 关闭文件
ifs.close();
}
int main() {
test02();
return 0;
}
- 读文件操作可以利用ifstram或者fstream类
- 利用is_open函数可以判断文件是否打开成功
- close关闭文件
5.2 二进制文件
- 以二进制的方式对文件进行读写操作
- 打开方式要指定为ios::binary
1) 写文件
- ostream write(const char* buffer, int len);
- 文件输出流对象可以通过write(),以二进制方式写数据
#include<iostream>
using namespace std;
#include<fstream>
#include<string>
class Person {
public:
char m_Name[64]; // c++中写字符串用string容易出错,最好使用字符数组
int m_age;
};
void test03() {
// 1. 包含头文件
// 2. 创建流对象
ofstream ofs;
// 可以使用open()指定打开方式,也可以在创建对象时指定
//ofstream ofs("person.txt", ios::out | ios::binary);
// 3. 打开文件
ofs.open("person.txt", ios::out | ios::binary);
// 4. 写文件
Person p = { "张三",18 };
ofs.write((const char*)&p,sizeof(Person));
}
int main() {
test03();
return 0;
}
2) 读文件
- istream& read(char* buffer, int len);
- 参数解释:字符指针buffer指向内存中一段
#include<iostream>
using namespace std;
#include<fstream>
#include<string>
class Person {
public:
char m_name[64]; // c++中写字符串用string容易出错,最好使用字符数组
int m_age;
};
void test04() {
// 1. 包含头文件
// 2. 创建流对象
ifstream ifs;
// 3. 打开文件,判断文件是否打开成功
ifs.open("person.txt", ios::in | ios::binary);
if (!ifs.is_open()) {
cout << "文件打开失败" << endl;
return;
}
// 4. 读文件
Person p;
ifs.read((char*)&p, sizeof(Person));
// 输出数据
cout << "姓名:" << p.m_name << ",年龄:" << p.m_age << endl;
// 5. 关闭流
ifs.close();
}
int main() {
test04();
return 0;
}