1.一个饲养员给动物喂食物的例子体现JAVA中的面向对象思想,接口(抽象类)的用处 package com.softeem.demo; /** *@author leno *动物的接口 */ interface Animal { public void eat(Food food); } /** *@author leno *一种动物类:猫 */ class Cat implements Animal { public void eat(Food food) { System.out.println("小猫吃" + food.getName()); } } /** *@author leno *一种动物类:狗 */ class Dog implements Animal { public void eat(Food food) { System.out.println("小狗啃" + food.getName()); } } /** *@author leno *食物抽象类 */ abstract class Food { protected String name; public String getName() { return name; } public void setName(String name) { this.name = name; } } /** *@author leno *一种食物类:鱼 */ class Fish extends Food { public Fish(String name) { this.name = name; } } /** *@author leno *一种食物类:骨头 */ class Bone extends Food { public Bone(String name) { this.name = name; } } /** *@author leno *饲养员类 * */ class Feeder { /** *饲养员给某种动物喂某种食物 *@param animal *@param food */ public void feed(Animal animal, Food food) { animal.eat(food); } } /** *@author leno *测试饲养员给动物喂食物 */ public class TestFeeder { public static void main(String[] args) { Feeder feeder = new Feeder(); Animal animal = new Dog(); Food food = new Bone("肉骨头"); feeder.feed(animal, food); //给狗喂肉骨头 animal = new Cat(); food = new Fish("鱼"); feeder.feed(animal, food); //给猫喂鱼 } } 2.做一个单子模式的类,只加载一次属性文件 package com.softeem.demo; import java.io.FileInputStream; import java.io.FileNotFoundException; import java.io.IOException; import java.io.InputStream; import java.util.Properties; /** * @authorleno 单子模式,保证在整个应用期间只加载一次配置属性文件 */ public class Singleton { private static Singleton instance; private static final String CONFIG_FILE_PATH = "E://config.properties"; private Properties config; private Singleton() { config = new Properties(); InputStream is; try { is = new FileInputStream(CONFIG_FILE_PATH); config.load(is); is.close(); } catch (FileNotFoundException e) { // TODO Auto-generated catch block e.printStackTrace(); } catch (IOException e) { // TODO Auto-generated catch block e.printStackTrace(); } } public static Singleton getInstance() { if (instance == null) { instance = new Singleton(); } return instance; } public Properties getConfig() { return config; } public void setConfig(Properties config) { this.config = config; } } 3.用JAVA中的多线程示例银行取款问题 package com.softeem.demo; /** *@author leno *账户类 *默认有余额,可以取款 */ class Account { private float balance = 1000; public float getBalance() { return balance; } public void setBalance(float balance) { this.balance = balance; } /** *取款的方法需要同步 *@param money */ public synchronized void withdrawals(float money) { if (balance >= money) { System.out.println("被取走" + money + "元!"); try { Thread.sleep(1000); } catch (InterruptedException e) { // TODO Auto-generated catch block e.printStackTrace(); } balance -= money; } else { System.out.println("对不起,余额不足!"); } } } /** *@author leno *银行卡 */ class TestAccount1 extends Thread { private Account account; public TestAccount1(Account account) { this.account = account; } @Override public void run() { account.withdrawals(800); System.out.println("余额为:" + account.getBalance() + "元!"); } } /** *@authorleno *存折 */ class TestAccount2 extends Thread { private Account account; public TestAccount2(Account account) { this.account = account; } @Override public void run() { account.withdrawals(700); System.out.println("余额为:" + account.getBalance() + "元!"); } } public class Test { public static void main(String[] args) { Account account = new Account(); TestAccount1 testAccount1 = new TestAccount1(account); testAccount1.start(); TestAccount2 testAccount2 = new TestAccount2(account); testAccount2.start(); } } 4.用JAVA中的多线程示例生产者和消费者问题 package com.softeem.demo; class Producer implements Runnable { private SyncStack stack; public Producer(SyncStack stack) { this.stack = stack; } public void run() { for (int i = 0; i < stack.getProducts().length; i++) { String product = "产品" + i; stack.push(product); System.out.println("生产了: " + product); try { Thread.sleep(200); } catch (InterruptedException e) { e.printStackTrace(); } } } } class Consumer implements Runnable { private SyncStack stack; public Consumer(SyncStack stack) { this.stack = stack; } public void run() { for (int i = 0; i < stack.getProducts().length; i++) { String product = stack.pop(); System.out.println("消费了: " + product); try { Thread.sleep(1000); } catch (InterruptedException e) { e.printStackTrace(); } } } } class SyncStack { private String[] products = new String[10]; private int index; public synchronized void push(String product) { if (index == product.length()) { try { wait(); } catch (InterruptedException e) { // TODO Auto-generated catch block e.printStackTrace(); } } notify(); products[index] = product; index++; } public synchronized String pop() { if (index == 0) { try { wait(); } catch (InterruptedException e) { // TODO Auto-generated catch block e.printStackTrace(); } } notify(); index--; String product = products[index]; return product; } public String[] getProducts() { return products; } } public class TestProducerConsumer { public static void main(String[] args) { SyncStack stack = new SyncStack(); Producer p = new Producer(stack); Consumer c = new Consumer(stack); new Thread(p).start(); new Thread(c).start(); } } 5.编程实现序列化的Student(sno,sname)对象在网络上的传输 package com.softeem.demo; import java.io.IOException; import java.io.ObjectInputStream; import java.io.ObjectOutputStream; import java.io.Serializable; import java.net.ServerSocket; import java.net.Socket; class Student implements Serializable { private int sno; private String sname; public Student(int sno, String sname) { this.sno = sno; this.sname = sname; } public int getSno() { return sno; } public void setSno(int sno) { this.sno = sno; } public String getSname() { return sname; } public void setSname(String sname) { this.sname = sname; } @Override public String toString() { return "学号:" + sno + ";姓名:" + sname; } } class MyClient extends Thread { @Override public void run() { try { Socket s = new Socket("localhost", 9999); ObjectInputStream ois = new ObjectInputStream(s.getInputStream()); Student stu = (Student) ois.readObject(); String msg = "客户端程序收到服务器端程序传输过来的学生对象>> " + stu; System.out.println(msg); ois.close(); s.close(); } catch (IOException e) { // TODO Auto-generated catch block e.printStackTrace(); } catch (ClassNotFoundException e) { // TODO Auto-generated catch block e.printStackTrace(); } } } class MyServer extends Thread { @Override public void run() { try { ServerSocket ss = new ServerSocket(9999); Socket s = ss.accept(); ObjectOutputStream ops = new ObjectOutputStream(s.getOutputStream()); Student stu = new Student(1, "赵本山"); ops.writeObject(stu); ops.close(); s.close(); ss.close(); } catch (IOException e) { // TODO Auto-generated catch block e.printStackTrace(); } } } public class TestTransfer { public static void main(String[] args) { new MyServer().start(); new MyClient().start(); } }
java 成功案例,java经典例子
最新推荐文章于 2024-06-26 15:09:51 发布
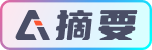