- 引入依赖:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-activemq</artifactId>
<version>2.5.14</version>
</dependency>
- 配置类:
package com.yutu.fileupload.config;
import org.apache.activemq.command.ActiveMQTopic;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.jms.config.DefaultJmsListenerContainerFactory;
import org.springframework.jms.config.JmsListenerContainerFactory;
import javax.jms.ConnectionFactory;
import javax.jms.Topic;
@Configuration
public class ConfigBean {
@Value("${myTopic}")
private String myTopicName;
@Bean
public Topic topic(){
return new ActiveMQTopic(myTopicName);
}
@Bean
public JmsListenerContainerFactory<?> topicListenerFactory(ConnectionFactory connectionFactory) {
DefaultJmsListenerContainerFactory factory = new DefaultJmsListenerContainerFactory();
factory.setPubSubDomain(true);
factory.setConnectionFactory(connectionFactory);
return factory;
}
}
- 主题消息的发送类:
package com.yutu.fileupload.mq;
import org.springframework.jms.core.JmsMessagingTemplate;
import org.springframework.stereotype.Component;
import javax.annotation.Resource;
@Component
public class Topic_producer {
@Resource
private JmsMessagingTemplate jmsMessagingTemplate;
public void sendTopic(String msg) {
System.out.println("发送Topic消息内容 :"+msg);
this.jmsMessagingTemplate.convertAndSend("boot-activemq-Topic01", msg);
}
}
- application.yml配置中需要配置为true
server:
port: 18808
spring:
datasource:
url: jdbc:mysql://localhost/nx?useUnicode=true&characterEncoding=UTF-8
driver-class-name: com.mysql.cj.jdbc.Driver
username: root
password: 123456
activemq:
broker-url: tcp://127.0.0.1:61616
user: admin
password: admin
jms:
pub-sub-domain: true #false表示的是队列,true表示的是topic 默认是false,可以不写
# 自定义一个队列名称
myTopic: boot-activemq-Topic01
mapper:
identity: MYSQL
mybatis:
mapper-locations: classpath:mappers
- 消费消息,配置监听的方式:
package com.yutu.fileupload.mq;
import org.springframework.jms.annotation.JmsListener;
import org.springframework.stereotype.Component;
@Component
public class Topic_Consumer {
@JmsListener(destination = "boot-activemq-Topic01", containerFactory = "topicListenerFactory")
public void receiveTopic1(String text) {
System.out.println("receiveTopic1接收到Topic消息 : " + text);
}
}
- 测试类:
package com.paic.mq;
import com.yutu.fileupload.FileUploadApplication;
import com.yutu.fileupload.mq.Topic_producer;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.test.context.junit4.SpringJUnit4ClassRunner;
import org.springframework.test.context.web.WebAppConfiguration;
@SpringBootTest(classes = FileUploadApplication.class)
@RunWith(SpringJUnit4ClassRunner.class)
@WebAppConfiguration
public class SpingbootActiveMQ {
@Autowired
private Topic_producer producer;
@Test
public void sendSimpleTopicMessage() {
this.producer.sendTopic("提现200.00元");
}
}
"C:\Program Files\Java\jdk1.8.0_152\bin\java.exe" -agentlib:jdwp=transport=dt_socket,address=127.0.0.1:64842,suspend=y,server=n -ea -Didea.test.cyclic.buffer.size=1048576 -javaagent:C:\Users\Administrator\AppData\Local\JetBrains\IntelliJIdea2021.2\captureAgent\debugger-agent.jar -Dfile.encoding=UTF-8 -classpath "D:\Program Files\JetBrains\IntelliJ IDEA 2021.2.1\lib\idea_rt.jar;D:\Program Files\JetBrains\IntelliJ IDEA 2021.2.1\plugins\junit\lib\junit5-rt.jar;D:\Program Files\JetBrains\IntelliJ IDEA 2021.2.1\plugins\junit\lib\junit-rt.jar;C:\Program Files\Java\jdk1.8.0_152\jre\lib\charsets.jar;C:\Program Files\Java\jdk1.8.0_152\jre\lib\deploy.jar;C:\Program Files\Java\jdk1.8.0_152\jre\lib\ext\access-bridge-64.jar;C:\Program Files\Java\jdk1.8.0_152\jre\lib\ext\cldrdata.jar;C:\Program Files\Java\jdk1.8.0_152\jre\lib\ext\dnsns.jar;C:\Program Files\Java\jdk1.8.0_152\jre\lib\ext\jaccess.jar;C:\Program Files\Java\jdk1.8.0_152\jre\lib\ext\jfxrt.jar;C:\Program Files\Java\jdk1.8.0_152\jre\lib\ext\localedata.jar;C:\Program Files\Java\jdk1.8.0_152\jre\lib\ext\nashorn.jar;C:\Program Files\Java\jdk1.8.0_152\jre\lib\ext\sunec.jar;C:\Program Files\Java\jdk1.8.0_152\jre\lib\ext\sunjce_provider.jar;C:\Program Files\Java\jdk1.8.0_152\jre\lib\ext\sunmscapi.jar;C:\Program Files\Java\jdk1.8.0_152\jre\lib\ext\sunpkcs11.jar;C:\Program Files\Java\jdk1.8.0_152\jre\lib\ext\zipfs.jar;C:\Program Files\Java\jdk1.8.0_152\jre\lib\javaws.jar;C:\Program Files\Java\jdk1.8.0_152\jre\lib\jce.jar;C:\Program Files\Java\jdk1.8.0_152\jre\lib\jfr.jar;C:\Program Files\Java\jdk1.8.0_152\jre\lib\jfxswt.jar;C:\Program Files\Java\jdk1.8.0_152\jre\lib\jsse.jar;C:\Program Files\Java\jdk1.8.0_152\jre\lib\management-agent.jar;C:\Program Files\Java\jdk1.8.0_152\jre\lib\plugin.jar;C:\Program Files\Java\jdk1.8.0_152\jre\lib\resources.jar;C:\Program Files\Java\jdk1.8.0_152\jre\lib\rt.jar;D:\code\fileupload\target\test-classes;D:\code\fileupload\target\classes;E:\mvnrepositry\mysql\mysql-connector-java\8.0.26\mysql-connector-java-8.0.26.jar;E:\mvnrepositry\org\mybatis\spring\boot\mybatis-spring-boot-starter\2.2.0\mybatis-spring-boot-starter-2.2.0.jar;E:\mvnrepositry\org\springframework\boot\spring-boot-starter\2.5.3\spring-boot-starter-2.5.3.jar;E:\mvnrepositry\org\springframework\boot\spring-boot\2.5.3\spring-boot-2.5.3.jar;E:\mvnrepositry\org\springframework\boot\spring-boot-autoconfigure\2.5.3\spring-boot-autoconfigure-2.5.3.jar;E:\mvnrepositry\org\springframework\boot\spring-boot-starter-logging\2.5.3\spring-boot-starter-logging-2.5.3.jar;E:\mvnrepositry\ch\qos\logback\logback-classic\1.2.4\logback-classic-1.2.4.jar;E:\mvnrepositry\ch\qos\logback\logback-core\1.2.4\logback-core-1.2.4.jar;E:\mvnrepositry\org\apache\logging\log4j\log4j-to-slf4j\2.14.1\log4j-to-slf4j-2.14.1.jar;E:\mvnrepositry\org\apache\logging\log4j\log4j-api\2.14.1\log4j-api-2.14.1.jar;E:\mvnrepositry\org\slf4j\jul-to-slf4j\1.7.32\jul-to-slf4j-1.7.32.jar;E:\mvnrepositry\jakarta\annotation\jakarta.annotation-api\1.3.5\jakarta.annotation-api-1.3.5.jar;E:\mvnrepositry\org\yaml\snakeyaml\1.28\snakeyaml-1.28.jar;E:\mvnrepositry\org\springframework\boot\spring-boot-starter-jdbc\2.5.3\spring-boot-starter-jdbc-2.5.3.jar;E:\mvnrepositry\com\zaxxer\HikariCP\4.0.3\HikariCP-4.0.3.jar;E:\mvnrepositry\org\springframework\spring-jdbc\5.3.9\spring-jdbc-5.3.9.jar;E:\mvnrepositry\org\mybatis\spring\boot\mybatis-spring-boot-autoconfigure\2.2.0\mybatis-spring-boot-autoconfigure-2.2.0.jar;E:\mvnrepositry\org\mybatis\mybatis\3.5.7\mybatis-3.5.7.jar;E:\mvnrepositry\org\mybatis\mybatis-spring\2.0.6\mybatis-spring-2.0.6.jar;E:\mvnrepositry\org\springframework\boot\spring-boot-starter-web\2.5.3\spring-boot-starter-web-2.5.3.jar;E:\mvnrepositry\org\springframework\boot\spring-boot-starter-json\2.5.3\spring-boot-starter-json-2.5.3.jar;E:\mvnrepositry\com\fasterxml\jackson\core\jackson-databind\2.12.4\jackson-databind-2.12.4.jar;E:\mvnrepositry\com\fasterxml\jackson\core\jackson-annotations\2.12.4\jackson-annotations-2.12.4.jar;E:\mvnrepositry\com\fasterxml\jackson\core\jackson-core\2.12.4\jackson-core-2.12.4.jar;E:\mvnrepositry\com\fasterxml\jackson\datatype\jackson-datatype-jdk8\2.12.4\jackson-datatype-jdk8-2.12.4.jar;E:\mvnrepositry\com\fasterxml\jackson\datatype\jackson-datatype-jsr310\2.12.4\jackson-datatype-jsr310-2.12.4.jar;E:\mvnrepositry\com\fasterxml\jackson\module\jackson-module-parameter-names\2.12.4\jackson-module-parameter-names-2.12.4.jar;E:\mvnrepositry\org\springframework\boot\spring-boot-starter-tomcat\2.5.3\spring-boot-starter-tomcat-2.5.3.jar;E:\mvnrepositry\org\apache\tomcat\embed\tomcat-embed-core\9.0.50\tomcat-embed-core-9.0.50.jar;E:\mvnrepositry\org\apache\tomcat\embed\tomcat-embed-el\9.0.50\tomcat-embed-el-9.0.50.jar;E:\mvnrepositry\org\apache\tomcat\embed\tomcat-embed-websocket\9.0.50\tomcat-embed-websocket-9.0.50.jar;E:\mvnrepositry\org\springframework\spring-web\5.3.9\spring-web-5.3.9.jar;E:\mvnrepositry\org\springframework\spring-beans\5.3.9\spring-beans-5.3.9.jar;E:\mvnrepositry\org\springframework\spring-webmvc\5.3.9\spring-webmvc-5.3.9.jar;E:\mvnrepositry\org\springframework\spring-aop\5.3.9\spring-aop-5.3.9.jar;E:\mvnrepositry\org\springframework\spring-context\5.3.9\spring-context-5.3.9.jar;E:\mvnrepositry\org\springframework\spring-expression\5.3.9\spring-expression-5.3.9.jar;E:\mvnrepositry\org\springframework\boot\spring-boot-starter-test\2.5.3\spring-boot-starter-test-2.5.3.jar;E:\mvnrepositry\org\springframework\boot\spring-boot-test\2.5.3\spring-boot-test-2.5.3.jar;E:\mvnrepositry\org\springframework\boot\spring-boot-test-autoconfigure\2.5.3\spring-boot-test-autoconfigure-2.5.3.jar;E:\mvnrepositry\com\jayway\jsonpath\json-path\2.5.0\json-path-2.5.0.jar;E:\mvnrepositry\net\minidev\json-smart\2.4.7\json-smart-2.4.7.jar;E:\mvnrepositry\net\minidev\accessors-smart\2.4.7\accessors-smart-2.4.7.jar;E:\mvnrepositry\org\ow2\asm\asm\9.1\asm-9.1.jar;E:\mvnrepositry\org\slf4j\slf4j-api\1.7.32\slf4j-api-1.7.32.jar;E:\mvnrepositry\jakarta\xml\bind\jakarta.xml.bind-api\2.3.3\jakarta.xml.bind-api-2.3.3.jar;E:\mvnrepositry\jakarta\activation\jakarta.activation-api\1.2.2\jakarta.activation-api-1.2.2.jar;E:\mvnrepositry\org\assertj\assertj-core\3.19.0\assertj-core-3.19.0.jar;E:\mvnrepositry\org\hamcrest\hamcrest\2.2\hamcrest-2.2.jar;E:\mvnrepositry\org\junit\jupiter\junit-jupiter\5.7.2\junit-jupiter-5.7.2.jar;E:\mvnrepositry\org\junit\jupiter\junit-jupiter-api\5.7.2\junit-jupiter-api-5.7.2.jar;E:\mvnrepositry\org\apiguardian\apiguardian-api\1.1.0\apiguardian-api-1.1.0.jar;E:\mvnrepositry\org\opentest4j\opentest4j\1.2.0\opentest4j-1.2.0.jar;E:\mvnrepositry\org\junit\platform\junit-platform-commons\1.7.2\junit-platform-commons-1.7.2.jar;E:\mvnrepositry\org\junit\jupiter\junit-jupiter-params\5.7.2\junit-jupiter-params-5.7.2.jar;E:\mvnrepositry\org\junit\jupiter\junit-jupiter-engine\5.7.2\junit-jupiter-engine-5.7.2.jar;E:\mvnrepositry\org\junit\platform\junit-platform-engine\1.7.2\junit-platform-engine-1.7.2.jar;E:\mvnrepositry\org\mockito\mockito-core\3.9.0\mockito-core-3.9.0.jar;E:\mvnrepositry\net\bytebuddy\byte-buddy\1.10.22\byte-buddy-1.10.22.jar;E:\mvnrepositry\net\bytebuddy\byte-buddy-agent\1.10.22\byte-buddy-agent-1.10.22.jar;E:\mvnrepositry\org\objenesis\objenesis\3.2\objenesis-3.2.jar;E:\mvnrepositry\org\mockito\mockito-junit-jupiter\3.9.0\mockito-junit-jupiter-3.9.0.jar;E:\mvnrepositry\org\skyscreamer\jsonassert\1.5.0\jsonassert-1.5.0.jar;E:\mvnrepositry\com\vaadin\external\google\android-json\0.0.20131108.vaadin1\android-json-0.0.20131108.vaadin1.jar;E:\mvnrepositry\org\springframework\spring-core\5.3.9\spring-core-5.3.9.jar;E:\mvnrepositry\org\springframework\spring-jcl\5.3.9\spring-jcl-5.3.9.jar;E:\mvnrepositry\org\springframework\spring-test\5.3.9\spring-test-5.3.9.jar;E:\mvnrepositry\org\xmlunit\xmlunit-core\2.8.2\xmlunit-core-2.8.2.jar;E:\mvnrepositry\com\alibaba\fastjson\1.2.46\fastjson-1.2.46.jar;E:\mvnrepositry\org\apache\commons\commons-compress\1.21\commons-compress-1.21.jar;E:\mvnrepositry\org\apache\ant\ant\1.10.5\ant-1.10.5.jar;E:\mvnrepositry\org\apache\ant\ant-launcher\1.10.5\ant-launcher-1.10.5.jar;E:\mvnrepositry\org\projectlombok\lombok\1.18.20\lombok-1.18.20.jar;E:\mvnrepositry\junit\junit\4.13.2\junit-4.13.2.jar;E:\mvnrepositry\org\hamcrest\hamcrest-core\2.2\hamcrest-core-2.2.jar;E:\mvnrepositry\cn\hutool\hutool-all\5.5.1\hutool-all-5.5.1.jar;E:\mvnrepositry\commons-codec\commons-codec\1.15\commons-codec-1.15.jar;E:\mvnrepositry\commons-io\commons-io\2.8.0\commons-io-2.8.0.jar;E:\mvnrepositry\org\apache\httpcomponents\httpclient\4.5.13\httpclient-4.5.13.jar;E:\mvnrepositry\org\apache\httpcomponents\httpcore\4.4.14\httpcore-4.4.14.jar;E:\mvnrepositry\log4j\log4j\1.2.17\log4j-1.2.17.jar;E:\mvnrepositry\org\springframework\boot\spring-boot-starter-activemq\2.5.14\spring-boot-starter-activemq-2.5.14.jar;E:\mvnrepositry\org\springframework\spring-jms\5.3.9\spring-jms-5.3.9.jar;E:\mvnrepositry\org\springframework\spring-messaging\5.3.9\spring-messaging-5.3.9.jar;E:\mvnrepositry\org\springframework\spring-tx\5.3.9\spring-tx-5.3.9.jar;E:\mvnrepositry\org\apache\activemq\activemq-broker\5.16.2\activemq-broker-5.16.2.jar;E:\mvnrepositry\org\apache\activemq\activemq-client\5.16.2\activemq-client-5.16.2.jar;E:\mvnrepositry\org\fusesource\hawtbuf\hawtbuf\1.11\hawtbuf-1.11.jar;E:\mvnrepositry\org\apache\activemq\activemq-openwire-legacy\5.16.2\activemq-openwire-legacy-5.16.2.jar;E:\mvnrepositry\jakarta\jms\jakarta.jms-api\2.0.3\jakarta.jms-api-2.0.3.jar;E:\mvnrepositry\jakarta\management\j2ee\jakarta.management.j2ee-api\1.1.4\jakarta.management.j2ee-api-1.1.4.jar" com.intellij.rt.junit.JUnitStarter -ideVersion5 -junit4 com.paic.mq.SpingbootActiveMQ,sendSimpleTopicMessage
Connected to the target VM, address: '127.0.0.1:64842', transport: 'socket'
10:12:43.969 [main] DEBUG org.springframework.test.context.junit4.SpringJUnit4ClassRunner - SpringJUnit4ClassRunner constructor called with [class com.paic.mq.SpingbootActiveMQ]
10:12:43.981 [main] DEBUG org.springframework.test.context.BootstrapUtils - Instantiating CacheAwareContextLoaderDelegate from class [org.springframework.test.context.cache.DefaultCacheAwareContextLoaderDelegate]
10:12:44.012 [main] DEBUG org.springframework.test.context.BootstrapUtils - Instantiating BootstrapContext using constructor [public org.springframework.test.context.support.DefaultBootstrapContext(java.lang.Class,org.springframework.test.context.CacheAwareContextLoaderDelegate)]
10:12:44.157 [main] DEBUG org.springframework.test.context.BootstrapUtils - Instantiating TestContextBootstrapper for test class [com.paic.mq.SpingbootActiveMQ] from class [org.springframework.boot.test.context.SpringBootTestContextBootstrapper]
10:12:44.207 [main] INFO org.springframework.boot.test.context.SpringBootTestContextBootstrapper - Neither @ContextConfiguration nor @ContextHierarchy found for test class [com.paic.mq.SpingbootActiveMQ], using SpringBootContextLoader
10:12:44.216 [main] DEBUG org.springframework.test.context.support.AbstractContextLoader - Did not detect default resource location for test class [com.paic.mq.SpingbootActiveMQ]: class path resource [com/paic/mq/SpingbootActiveMQ-context.xml] does not exist
10:12:44.217 [main] DEBUG org.springframework.test.context.support.AbstractContextLoader - Did not detect default resource location for test class [com.paic.mq.SpingbootActiveMQ]: class path resource [com/paic/mq/SpingbootActiveMQContext.groovy] does not exist
10:12:44.217 [main] INFO org.springframework.test.context.support.AbstractContextLoader - Could not detect default resource locations for test class [com.paic.mq.SpingbootActiveMQ]: no resource found for suffixes {-context.xml, Context.groovy}.
10:12:44.410 [main] DEBUG org.springframework.test.context.support.ActiveProfilesUtils - Could not find an 'annotation declaring class' for annotation type [org.springframework.test.context.ActiveProfiles] and class [com.paic.mq.SpingbootActiveMQ]
10:12:44.892 [main] DEBUG org.springframework.boot.test.context.SpringBootTestContextBootstrapper - @TestExecutionListeners is not present for class [com.paic.mq.SpingbootActiveMQ]: using defaults.
10:12:44.893 [main] INFO org.springframework.boot.test.context.SpringBootTestContextBootstrapper - Loaded default TestExecutionListener class names from location [META-INF/spring.factories]: [org.springframework.boot.test.mock.mockito.MockitoTestExecutionListener, org.springframework.boot.test.mock.mockito.ResetMocksTestExecutionListener, org.springframework.boot.test.autoconfigure.restdocs.RestDocsTestExecutionListener, org.springframework.boot.test.autoconfigure.web.client.MockRestServiceServerResetTestExecutionListener, org.springframework.boot.test.autoconfigure.web.servlet.MockMvcPrintOnlyOnFailureTestExecutionListener, org.springframework.boot.test.autoconfigure.web.servlet.WebDriverTestExecutionListener, org.springframework.boot.test.autoconfigure.webservices.client.MockWebServiceServerTestExecutionListener, org.springframework.test.context.web.ServletTestExecutionListener, org.springframework.test.context.support.DirtiesContextBeforeModesTestExecutionListener, org.springframework.test.context.event.ApplicationEventsTestExecutionListener, org.springframework.test.context.support.DependencyInjectionTestExecutionListener, org.springframework.test.context.support.DirtiesContextTestExecutionListener, org.springframework.test.context.transaction.TransactionalTestExecutionListener, org.springframework.test.context.jdbc.SqlScriptsTestExecutionListener, org.springframework.test.context.event.EventPublishingTestExecutionListener]
10:12:44.970 [main] INFO org.springframework.boot.test.context.SpringBootTestContextBootstrapper - Using TestExecutionListeners: [org.springframework.test.context.web.ServletTestExecutionListener@5a9f4771, org.springframework.test.context.support.DirtiesContextBeforeModesTestExecutionListener@282cb7c7, org.springframework.test.context.event.ApplicationEventsTestExecutionListener@7d898981, org.springframework.boot.test.mock.mockito.MockitoTestExecutionListener@48d61b48, org.springframework.boot.test.autoconfigure.SpringBootDependencyInjectionTestExecutionListener@68d279ec, org.springframework.test.context.support.DirtiesContextTestExecutionListener@258d79be, org.springframework.test.context.transaction.TransactionalTestExecutionListener@14f9390f, org.springframework.test.context.jdbc.SqlScriptsTestExecutionListener@6c0d7c83, org.springframework.test.context.event.EventPublishingTestExecutionListener@176b75f7, org.springframework.boot.test.mock.mockito.ResetMocksTestExecutionListener@5965be2d, org.springframework.boot.test.autoconfigure.restdocs.RestDocsTestExecutionListener@409c54f, org.springframework.boot.test.autoconfigure.web.client.MockRestServiceServerResetTestExecutionListener@3e74829, org.springframework.boot.test.autoconfigure.web.servlet.MockMvcPrintOnlyOnFailureTestExecutionListener@4f6f416f, org.springframework.boot.test.autoconfigure.web.servlet.WebDriverTestExecutionListener@3b8f0a79, org.springframework.boot.test.autoconfigure.webservices.client.MockWebServiceServerTestExecutionListener@71e693fa]
10:12:44.974 [main] DEBUG org.springframework.test.annotation.ProfileValueUtils - Retrieved @ProfileValueSourceConfiguration [null] for test class [com.paic.mq.SpingbootActiveMQ]
10:12:44.977 [main] DEBUG org.springframework.test.annotation.ProfileValueUtils - Retrieved ProfileValueSource type [class org.springframework.test.annotation.SystemProfileValueSource] for class [com.paic.mq.SpingbootActiveMQ]
10:12:44.979 [main] DEBUG org.springframework.test.annotation.ProfileValueUtils - Retrieved @ProfileValueSourceConfiguration [null] for test class [com.paic.mq.SpingbootActiveMQ]
10:12:44.980 [main] DEBUG org.springframework.test.annotation.ProfileValueUtils - Retrieved ProfileValueSource type [class org.springframework.test.annotation.SystemProfileValueSource] for class [com.paic.mq.SpingbootActiveMQ]
10:12:44.980 [main] DEBUG org.springframework.test.annotation.ProfileValueUtils - Retrieved @ProfileValueSourceConfiguration [null] for test class [com.paic.mq.SpingbootActiveMQ]
10:12:44.980 [main] DEBUG org.springframework.test.annotation.ProfileValueUtils - Retrieved ProfileValueSource type [class org.springframework.test.annotation.SystemProfileValueSource] for class [com.paic.mq.SpingbootActiveMQ]
10:12:45.004 [main] DEBUG org.springframework.test.annotation.ProfileValueUtils - Retrieved @ProfileValueSourceConfiguration [null] for test class [com.paic.mq.SpingbootActiveMQ]
10:12:45.004 [main] DEBUG org.springframework.test.annotation.ProfileValueUtils - Retrieved ProfileValueSource type [class org.springframework.test.annotation.SystemProfileValueSource] for class [com.paic.mq.SpingbootActiveMQ]
10:12:45.008 [main] DEBUG org.springframework.test.annotation.ProfileValueUtils - Retrieved @ProfileValueSourceConfiguration [null] for test class [com.paic.mq.SpingbootActiveMQ]
10:12:45.008 [main] DEBUG org.springframework.test.annotation.ProfileValueUtils - Retrieved ProfileValueSource type [class org.springframework.test.annotation.SystemProfileValueSource] for class [com.paic.mq.SpingbootActiveMQ]
10:12:45.010 [main] DEBUG org.springframework.test.annotation.ProfileValueUtils - Retrieved @ProfileValueSourceConfiguration [null] for test class [com.paic.mq.SpingbootActiveMQ]
10:12:45.010 [main] DEBUG org.springframework.test.annotation.ProfileValueUtils - Retrieved ProfileValueSource type [class org.springframework.test.annotation.SystemProfileValueSource] for class [com.paic.mq.SpingbootActiveMQ]
10:12:45.027 [main] DEBUG org.springframework.test.context.support.AbstractDirtiesContextTestExecutionListener - Before test class: context [DefaultTestContext@d771cc9 testClass = SpingbootActiveMQ, testInstance = [null], testMethod = [null], testException = [null], mergedContextConfiguration = [WebMergedContextConfiguration@36b4091c testClass = SpingbootActiveMQ, locations = '{}', classes = '{class com.yutu.fileupload.FileUploadApplication}', contextInitializerClasses = '[]', activeProfiles = '{}', propertySourceLocations = '{}', propertySourceProperties = '{org.springframework.boot.test.context.SpringBootTestContextBootstrapper=true}', contextCustomizers = set[org.springframework.boot.test.context.filter.ExcludeFilterContextCustomizer@574b560f, org.springframework.boot.test.json.DuplicateJsonObjectContextCustomizerFactory$DuplicateJsonObjectContextCustomizer@3943a2be, org.springframework.boot.test.mock.mockito.MockitoContextCustomizer@0, org.springframework.boot.test.web.client.TestRestTemplateContextCustomizer@44a7bfbc, org.springframework.boot.test.autoconfigure.actuate.metrics.MetricsExportContextCustomizerFactory$DisableMetricExportContextCustomizer@2beee7ff, org.springframework.boot.test.autoconfigure.properties.PropertyMappingContextCustomizer@0, org.springframework.boot.test.autoconfigure.web.servlet.WebDriverContextCustomizerFactory$Customizer@2e222612, org.springframework.test.context.web.socket.MockServerContainerContextCustomizer@59af0466, org.springframework.boot.test.context.SpringBootTestArgs@1, org.springframework.boot.test.context.SpringBootTestWebEnvironment@7722c3c3], resourceBasePath = 'src/main/webapp', contextLoader = 'org.springframework.boot.test.context.SpringBootContextLoader', parent = [null]], attributes = map['org.springframework.test.context.web.ServletTestExecutionListener.activateListener' -> true]], class annotated with @DirtiesContext [false] with mode [null].
10:12:45.033 [main] DEBUG org.springframework.test.annotation.ProfileValueUtils - Retrieved @ProfileValueSourceConfiguration [null] for test class [com.paic.mq.SpingbootActiveMQ]
10:12:45.033 [main] DEBUG org.springframework.test.annotation.ProfileValueUtils - Retrieved ProfileValueSource type [class org.springframework.test.annotation.SystemProfileValueSource] for class [com.paic.mq.SpingbootActiveMQ]
10:12:45.195 [main] DEBUG org.springframework.test.context.support.TestPropertySourceUtils - Adding inlined properties to environment: {spring.jmx.enabled=false, org.springframework.boot.test.context.SpringBootTestContextBootstrapper=true}
. ____ _ __ _ _
/\\ / ___'_ __ _ _(_)_ __ __ _ \ \ \ \
( ( )\___ | '_ | '_| | '_ \/ _` | \ \ \ \
\\/ ___)| |_)| | | | | || (_| | ) ) ) )
' |____| .__|_| |_|_| |_\__, | / / / /
=========|_|==============|___/=/_/_/_/
:: Spring Boot :: (v2.5.3)
2022-10-26 10:12:46.544 INFO 14260 --- [ main] com.paic.mq.SpingbootActiveMQ : Starting SpingbootActiveMQ using Java 1.8.0_152 on TC-20180820SHWB with PID 14260 (started by Administrator in D:\code\fileupload)
2022-10-26 10:12:46.550 INFO 14260 --- [ main] com.paic.mq.SpingbootActiveMQ : No active profile set, falling back to default profiles: default
Logging initialized using 'class org.apache.ibatis.logging.stdout.StdOutImpl' adapter.
Parsed mapper file: 'file [D:\code\fileupload\target\classes\mappers\UserMapper.xml]'
2022-10-26 10:12:54.772 INFO 14260 --- [ main] com.paic.mq.SpingbootActiveMQ : Started SpingbootActiveMQ in 9.542 seconds (JVM running for 12.753)
发送Topic消息内容 :提现200.00元
receiveTopic1接收到Topic消息 : 提现200.00元
Disconnected from the target VM, address: '127.0.0.1:64842', transport: 'socket'
Process finished with exit code 0
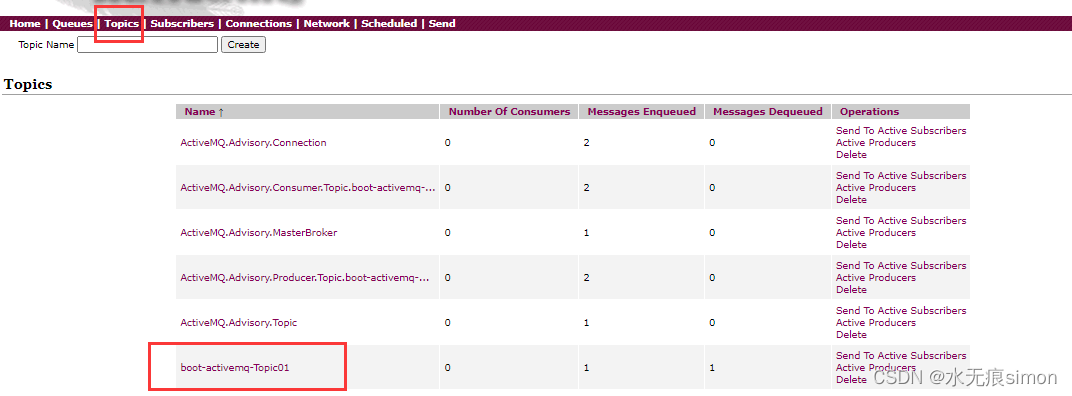