本节将使用TensorFlow实现一个卷积神经网络,具体模型见下图:
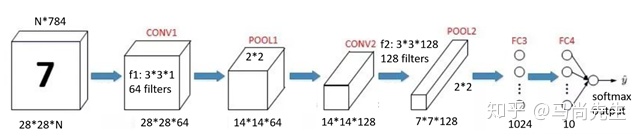
一,数据导入
导入mnist数据集的方法同前面一样一样的!
import
二,参数的初始化
数据集mnist图像输入为灰度图,所以channel为1,这样像素点数 28*28*1=784,最终需要分类的标签为0到9,十个标签。下面分别是卷积层和全连接层参数定义和初始化。
1,初始化代码
n_input
2,卷积层权重参数定义
权重weight字典参数中定义了卷积层和全连接层的权重参数。
wc1:[3,3,1,64]
- 第一个3 指定的 filter 中的h,
- 第二个3指定的 filter 中的w,
- 第三个参数1是连接的输入数据的深度,这里输入的是灰度图所以为1,
- 第四个参数64为输出的特征图像个数,这里指定为64.同样的wc2 参数的定义也类似。
wc2:[3,3,64,128]
- 第1,2 个参数不变。
- 第三个参数,输入数据的深度应为64,因为第一层卷积后输出特征图像的个数为64.
- 第四个参数,指定第二层输出特征图像为128个。
下图是官方解释:
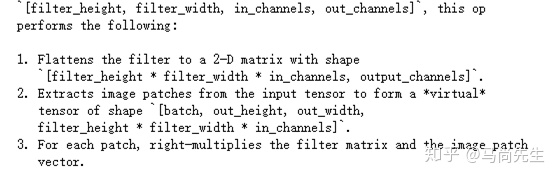
3,全连接层参数定义
wd1:[7*7*128,1024]
- 7*7*128 计算下本例子:
公式解释:28 为图像的h,3为 filter 的 h,2 为两倍的 padding,分母 1 为 stride,最后加上1,得到的结果为 28。这说明卷积层并没有减少图像的大小,而是 pooling 层减少了图像的大小,pooling 是从一个 2*2 的窗口矩阵到一个 1*1 的矩阵,图像的 h 和 w 均减小了一半,经过两个同样的 pool 层,所以 28/4=7。第二个 pool2 输出图像的大小为 7*7*128, 128 是定义的 filter 的个数。
Note:这里的 stride 和 padding 都为1。
- 1024 为定义输出的特征图形的个数,为下一个全连接层的输入。
wd2:[1024,n_output]
为第二个全连接层的权重参数,1024 为定义的filter的个数,n_output 为输出的标签个数。
偏质项初始化:
分别是每个层的要输出的特征图像个数,最后是要分类的10个标签数。
三,卷积和池化操作
1,卷积和池化函数定义代码
先看下定义的代码直观的感受下!
def
2,卷积参数相关解释
首先把输入的数据转化为TensorFlow支持的格式,具体的格式为:
tf.reshape(_input, shape=[-1,28,28,1])
- shape = [n,h,w,c]
- n:batch_size 的大小,h:输入图像的h, w:输入图像的w,c:输入图像的channel。
- -1 代表的意思是TensorFlow自己进行推断。
tf.nn.conv2d(_input_r, _w['wc1'], strides=[1,1,1,1], padding='SAME')
- _input_r: reshape之后的数据格式
- _w:初始化定义后的权重参数
- strides=[1,1,1,1] 参数介绍:
- Strides 分别表示是: batch_size, 图像h, 图像w,图像的channel。
- Padding 的两种模式:补全式SAME 进行填充和偷懒式 valid 不进行填充
3,池化相关参数解释
tf.nn.max_pool (_conv1, ksize=[1,2,2,1], strides=[1,2,2,1], padding='SAME')
- _conv1 为卷积层的输出
- ksize=[1,2,2,1]
- 窗口的大小,1 batch_size,第二个1 channel, 2,2 是池化的矩阵窗口大小
- Strides 和padding意思同前。
tf.nn.dropout(_pool1, _keepratio)
- 参数 _pool1 是池化层的输出, _keepratio 保持率。
- 随机的去掉一些神经元
Note:print(help(tf.nn.conv2d))查看官方帮助文档。
四,卷积模型框架搭建
x
五,模型训练和测试
和前面一样,采用交叉熵作为函数的损失函数,通过梯度下降法进行参数的优化,reduce_mean 进行精确度的计算。
do_train = 1
sess = tf.Session()
sess.run(init)
save_step = 1
saver = tf.train.Saver()
sess = tf.Session()
sess.run(init)
training_epochs = 15
batch_size = 16
display_step = 1
if do_train ==1:
for epoch in range (training_epochs):
avg_cost =0.1
#total_batch = int(mnist.train.num_examples/batch_size)
total_batch = 10
# iteration
for i in range (total_batch):
batch_xs, batch_ys = mnist.train.next_batch(batch_size)
#feeds = {x: batch_xs, y: batch_ys, keepratio: 0.7}
sess.run(optm, feed_dict={x: batch_xs, y: batch_ys, keepratio:0.7})
#sess.run(optm, feed_dict={x: batch_xs, y: batch_ys, keepratio:0.7})
avg_cost += sess.run(cost, feed_dict={x: batch_xs, y: batch_ys, keepratio:1.0})/total_batch
#dispaly
if epoch % display_step == 0:
print ('Epoch: %03d/%03d cost: %.9f' %(epoch, training_epochs,avg_cost))
#feeds = {x: batch_xs, y:batch_ys}
train_acc = sess.run(optm, feed_dict={x: batch_xs, y: batch_ys, keepratio: 1.0})
#print ('Train accuracy: %.3f' % (train_acc))
#feeds = {x: mnist.test.images, y:mnist.test.labels}
#test_acc = sess.run(accr, feed_dict=feeds)
#print ('test accuracy: %.3f' %(test_acc))
#save Net
if epoch % save_step == 0:
saver.save(sess, 'save/nets/cnn_mnist_basic.ckpt-' +str(epoch))
print ('Optimization finished')
else:
epoch = training_epochs-1
saver.restore(sess,'save/nets/cnn_mnist_basic.ckpt-' +str(epoch))
test_acc = sess.run(accr, feed_dict={x: batch_xs, y: batch_ys, keepratio: 1.0})
print ('Test accuracy: %.3f' %(test_acc))
六,训练结果和预测结果
令 do_train = 1, 取Epoch =15 进行训练然后将结果保存到文件夹中。在之后的测试过程中可以直接的把保存的训练模型download下来,进行预测从而节省训练的时间。
Epoch: 000/015 cost: 6.142920852
Epoch: 001/015 cost: 2.372950006
Epoch: 002/015 cost: 1.567487848
Epoch: 003/015 cost: 1.341774982
Epoch: 004/015 cost: 1.452789736
Epoch: 005/015 cost: 1.172398078
Epoch: 006/015 cost: 1.030768359
Epoch: 007/015 cost: 0.881382990
Epoch: 008/015 cost: 0.735200250
Epoch: 009/015 cost: 0.629416350
Epoch: 010/015 cost: 0.681320167
Epoch: 011/015 cost: 0.621121602
Epoch: 012/015 cost: 0.547099918
Epoch: 013/015 cost: 0.561984229
Epoch: 014/015 cost: 0.481403020
Optimization finished
下面是直接取第15次 Epoch: 014/015 cost: 0.481403020,模型训练的结果进行测试集的预测,结果如下。
INFO:tensorflow:Restoring parameters from save/nets/cnn_mnist_basic.ckpt-14
Test accuracy: 0.875