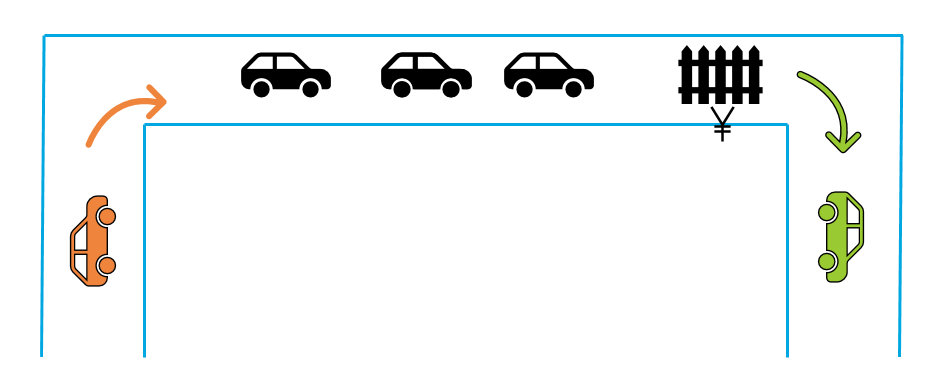
源于生活,抽象生活。
生活中的队列:当然是停车场排队出场啦。
计算机的队列:简答的说就是先入先出(FIFO)。在大脑中要有的概念是:
- 队头(状态):就是排队第一个交钱准备出场的车;
- 队尾(状态):最后一个排队准备交钱的车;
- 入队(动作):add. 把车开入“出停车场的道”。
- 出栈(动作):out.交完钱,把车开出停车场。
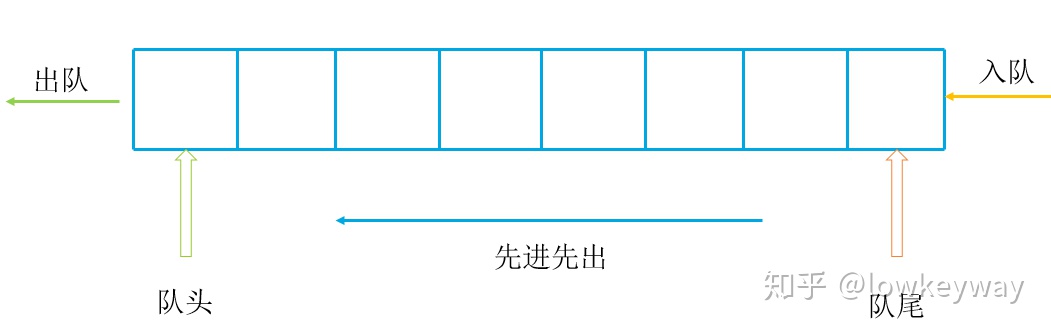
OK。本节只讨论先入先出的单向队列,貌似比栈还简单哈。话不多说,撸起袖子就写代码吧。先想象架构:
对象:队列moneyQueue(交钱队列);元素car(小汽车) 动作:入队列add(开入缴费队列);出队列out(开出停车场) 辅助动作:判空isEmpty(缴费队列上还有没有车),判满(缴费队列还能不能开进去车)
当然,我还是希望能够用模板类来实现。开始写代码吧。
moneyQueue.h
#ifndef __MONEY_QUEUE_H__
#define __MONEY_QUEUE_H__
#include <iostream>
#include <string>
using namespace std;
template <typename T>
class moneyQueue
{
public:
moneyQueue(int size);
~moneyQueue();
bool clear();
bool add(T t);
bool out(T &t);
bool isEmpty();
bool isFull();
int size();
bool show();
private:
T* m_pQueue;
int m_iTop;
int m_iBottom;
int m_iSize;
};
template <typename T>
moneyQueue<T>::moneyQueue(int size)
{
if (0 >= size)
{
cout << "Wrong queue number!" << endl;
return;
}
clear();
m_iSize = size;
m_pQueue = new T[size];
}
template <typename T>
moneyQueue<T>::~moneyQueue()
{
clear();
delete []m_pQueue;
m_pQueue = NULL;
}
template <typename T>
bool moneyQueue<T>::clear()
{
m_iBottom = -1;
m_iTop = 0;
m_iSize = 0;
return true;
}
template <typename T>
bool moneyQueue<T>::add(T t)
{
if (isFull())
{
cout << "The queue is full!" << endl;
return false;
}
m_pQueue[++m_iBottom] = t;
return true;
}
template <typename T>
bool moneyQueue<T>::out(T &t)
{
if (isEmpty())
{
cout << "The queue is empty!" << endl;
return false;
}
t = m_pQueue[0];
for(int i = 0; i < m_iBottom; i++)
{
m_pQueue[i] = m_pQueue[i + 1];
}
m_iBottom--;
return true;
}
template <typename T>
bool moneyQueue<T>::isEmpty()
{
return m_iBottom == -1 ? true : false;
}
template <typename T>
bool moneyQueue<T>::isFull()
{
return m_iBottom + 1 == m_iSize ? true : false;
}
template <typename T>
int moneyQueue<T>::size()
{
return m_iBottom;
}
template<typename T>
bool moneyQueue<T>::show()
{
if (isEmpty())
{
cout << "The queue is empty, nothing to show!" << endl;
return false;
}
for (int i = 0; i <= m_iBottom; i++)
{
cout << m_pQueue[i] << " " ;
}
cout << endl;
return true;
}
#endif // !1
car.h
#ifndef __CAR_H__
#define __CAR_H__
#include <iostream>
#include <ostream>
#include <string>
using namespace std;
class car
{
friend ostream &operator << (ostream &out, car &Car);
public:
car() {};
car(string name, int speed);
~car(){};
private:
string m_sName;
int m_iSpeed;
};
#endif
car.cpp
#include "car.h"
car::car(string name, int speed)
{
m_sName = name;
m_iSpeed = speed;
}
ostream &operator << (ostream &out, car &Car)
{
out << "[" << Car.m_sName << "," << Car.m_iSpeed << "]";
return out;
}
queueMain.cpp
#include <iostream>
#include <string>
#include "moneyQueue.h"
#include "car.h"
using namespace std;
static void myQueue();
int main()
{
myQueue();
system("pause");
return 0;
}
static void myQueue()
{
moneyQueue<int> i(5);
moneyQueue<string> s(5);
moneyQueue<car> c(5);
i.add(1);
i.add(2);
i.add(3);
i.add(4);
i.add(5);
i.show();
int iOut;
i.out(iOut);
cout << "iOut is " << iOut << endl;
i.show();
s.add("H");
s.add("e");
s.add("l");
s.add("l");
s.add("o");
s.show();
string sOut;
s.out(sOut);
cout << "sOut is " << sOut << endl;
s.add("!");
s.show();
car c1("car1", 10);
car c2("car2", 20);
car c3("car3", 30);
car c4("car4", 40);
car c5("car5", 50);
car c6("car6", 60);
car c7("car7", 70);
//car c7;
c.add(c1);
c.add(c2);
c.add(c3);
c.add(c4);
c.add(c5);
c.add(c6);
c.show();
c.out(c7);
cout << "out data is " << c7 << endl;
c.show();
}
运行结果:
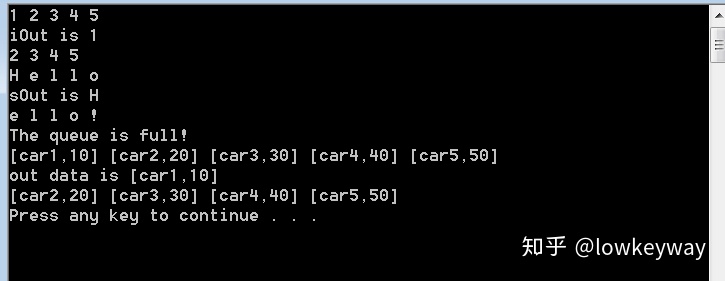