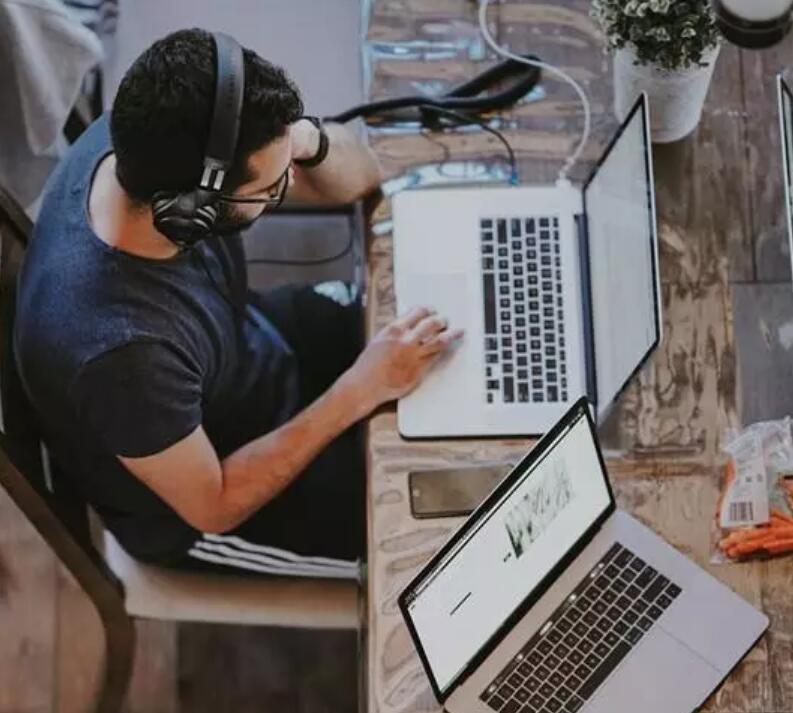
前两个示例在C中,最后一个在C ++中。在我的第一种方法中,我使用3个互斥锁和3个条件变量。通过以下示例,您可以在C和C ++中计划或控制任意数量的线程。首先,看看下面的第一个帖子。这里锁定了互斥锁lock1(以便其他线程无法访问代码)开始执行(代码未添加,只是注释),最后在完成等待cond1的任务后,同样,第二个线程锁定了互斥锁lock2,开始执行其业务逻辑,最后,等待来自cond2的条件和第三个线程锁定互斥锁lock3,开始执行其业务逻辑,最后等待cond3的条件。
我不是在这里添加任何业务逻辑,因为这只是一个例子。在注释掉的部分中,您可以添加将以并行模式执行的业务逻辑。假设thread3依赖于thread1的最终输出,它将被插入一个表中,而thread3将在创建其最终结果之前读取该信息,而thread2依赖于thread3的最终结果来生成其最终结果。因此,在将数据插入表之后,thread1通过条件变量向thread3发出信号,以继续其最终过程。这意味着thread1控制thread3。由于thread2依赖于thread3的最终结果,因此thread3控制thread2的执行。在这里,我们可以允许thread1独立执行,因为它的操作不依赖于任何其他线程,但是,例如,对于线程控制,我们在这里控制所有线程。因此,thread1是从thread2控制的。
要启动控制过程,我们首先发布thread1。在主线程中(即主函数;每个程序都有一个主线程,在C / C ++中,一旦控件传递给主方法/函数通过内核,这个主线程由操作系统自动创建)我们正在调用pthread_cond_signal(&cond1);
。从主线程调用此函数后,正在等待cond1的thread1将被释放,它将继续执行。一旦完成最后的任务,它就会打电话pthread_cond_signal(&cond3);
。现在,正在等待cond3条件的线程,即thread3,将被释放,它将开始执行其最后阶段并将调用 pthread_cond_signal(&cond2);
并且它将释放在cond2条件下等待的线程,在本例中为thread2。这是我们在多线程环境中调度和控制线程执行的方式。
#include<pthread.h> int controller = 0; void print(char *p) { printf("%s",p); } void * threadMethod1(void *arg) { while(1) { if(controller == 3) break; } print("I am thread 1stn"); controller = 1; pthread_exit(NULL); } void * threadMethod2(void *arg) { while(1) { if(controller == 1) break; } print("I am thread 2ndn"); controller = 2; pthread_exit(NULL); } void * threadMethod3(void *arg) { while(1) { if(controller == 0) break; } print("I am thread 3rdn"); controller = 3; pthread_exit(NULL); } int main(void) { pthread_t tid1, tid2, tid3; int i = 0; printf("Before creating the threadsn"); if( pthread_create(&tid1, NULL, threadMethod1, NULL) != 0 ) printf("Failed to create thread1n"); if( pthread_create(&tid2, NULL, threadMethod2, NULL) != 0 ) printf("Failed to create thread2n"); sleep(3); if( pthread_create(&tid3, NULL, threadMethod3, NULL) != 0 ) printf("Failed to create thread3n"); /* pthread_join(tid1,NULL); pthread_join(tid2,NULL); pthread_join(tid3,NULL);*/ sleep(10); exit(0); }
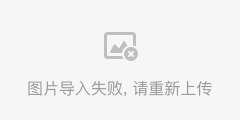
现在我的第三个例子是C ++。在这里,我使用的方法与我在第一个C示例中应用的方法相同。如果您直接参加此示例,请阅读我在C中的第一个示例以了解该方法。在下面的示例中,我在Visual Studio 2013中开发了代码,并且我没有将代码分发到单独的标头和CPP文件中。另外,我已经声明并定义了所有内联方法,因为这只是一个例子。另外,你可能会想,“为什么我在课堂结构相同时宣布三个课程?” 不要混淆,我使用相同的类定义 仅用于示例目的。查看业务逻辑的注释行。在这里,每个类都将具有不同的业务功能和逻辑。此示例适用于具有三个不同类的三个不同线程; 假设所有类都有不同的功能和业务逻辑。
#include "stdafx.h"//remove this header file if you are compiling with different compiler #include <thread> #include <mutex> #include <condition_variable> #include <iostream> #include "windows.h"//remove this header file if you are compiling with different compiler std::condition_variable _tcond1; std::condition_variable _tcond2; std::condition_variable _tcond3; class SimpleThread1 { private: std::mutex _lockprint; bool isThreadAlive = true; public: SimpleThread1(){} SimpleThread1(SimpleThread1 &st){}; void StartProcessing() { std::unique_lock<std::mutex> locker(_lockprint); //Add your business logic(parallel execution codes) here _tcond1.wait(locker); std::cout << "I am thread :1"<<std::endl; _tcond3.notify_one(); } void operator()() { while (isThreadAlive) StartProcessing(); } void stopeThread() { isThreadAlive = false; } }; class SimpleThread2 { private: std::mutex _lockprint; bool isThreadAlive = true; public: SimpleThread2(){} SimpleThread2(SimpleThread2 &st) {}; void StartProcessing() { std::unique_lock<std::mutex> locker(_lockprint); //Add your business logic(parallel execution codes) here _tcond2.wait(locker); std::cout << "I am thread :2"<< std::endl; _tcond1.notify_one(); } void operator()() { while (isThreadAlive) StartProcessing(); } void stopeThread() { isThreadAlive = false; } }; class SimpleThread3 { private: std::mutex _lockprint; bool isThreadAlive = true; public: SimpleThread3(){} SimpleThread3(SimpleThread3 &st) {}; void StartProcessing() { std::unique_lock<std::mutex> locker(_lockprint); //Add your business logic(parallel execution codes) here _tcond3.wait(locker); std::cout << "I am thread :3"<< std::endl; _tcond2.notify_one(); } void operator()() { while (isThreadAlive) StartProcessing(); } void stopeThread() { isThreadAlive = false; } }; int main() { SimpleThread1 st1; SimpleThread2 st2; SimpleThread3 st3; std::thread t1(st1); std::thread t2(st2); std::thread t3(st3); _tcond1.notify_one(); t1.detach(); t2.detach(); t3.detach(); Sleep(1000);//replace it with sleep(10) for linux/unix st1.stopeThread(); st2.stopeThread(); st3.stopeThread(); return 0; }
}