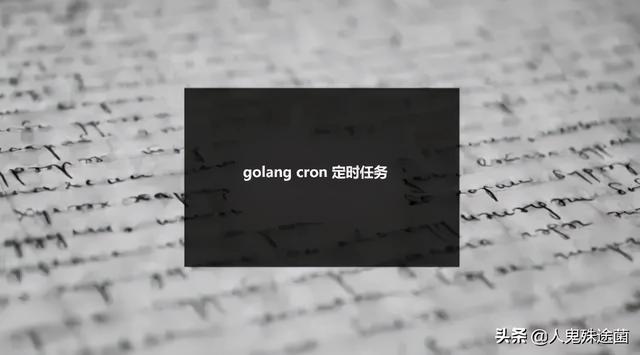
cron 功能
在Golang中也有这样一个工具的封装。提一下cron主要能干什么:
比如我们手机里面设置的闹钟,我们可以设置成每天早上7:00,每周周一到周三晚上一点,我们可以把cron形象的看作一个闹钟,会在我们规定的时间自动执行一些我们设置好的动作。
作为一个大学生,你可能追求过某某女孩子,你可能有这样一个需求:每天早上微信给她发早安和晚上给她发晚安。这可能是个不错的点子。
这都是浮云,还是努力学习,学会怎么用才是关键,不然你也写不出来。
cron 用法
首先先把第三方库下载下来:
$ go get -v -u github.com/robfig/cron
我们来看一个小的demo:每隔一秒打印"hello world"
package mainimport ("log""github.com/robfig/cron")func main() {log.Println("Starting...")c := cron.New() // 新建一个定时任务对象c.AddFunc("* * * * * *", func() {log.Println("hello world")}) // 给对象增加定时任务c.Start()select {}}
这个小功能用time.Ticker也可以实现,我们为什么要使用cron来完成呢?别急,接着往下面看就知道了。
cron表达式
我们在上面demo中使用了AddFunc,第一个参数我们传递了一个字符串是:"* * * * * *",这六个*是指什么呢?
┌─────────────second 范围 (0 - 60) │ ┌───────────── min (0 - 59) │ │ ┌────────────── hour (0 - 23) │ │ │ ┌─────────────── day of month (1 - 31) │ │ │ │ ┌──────────────── month (1 - 12) │ │ │ │ │ ┌───────────────── day of week (0 - 6) (0 to 6 are Sunday to │ │ │ │ │ │ Saturday) │ │ │ │ │ │ │ │ │ │ │ │ * * * * * *
匹配符号
- 星号(*) :表示 cron 表达式能匹配该字段的所有值。如在第5个字段使用星号(month),表示每个月
- 斜线(/):表示增长间隔,如第2个字段(minutes) 只是 3-59/15,表示每小时的第3分钟开始执行一次,之后 每隔 15 分钟执行一次(即 3(3+0*15)、18(3+1*15)、33(3+2*15)、48(3+3*15) 这些时间点执行),这里也可以表示为:3/15
- 逗号(,):用于枚举值,如第6个字段值是 MON,WED,FRI,表示 星期一、三、五 执行
- 连字号(-):表示一个范围,如第3个字段的值为 9-17 表示 9am 到 5pm 直接每个小时(包括9和17)
- 问号(?):只用于 日(Day of month) 和 星期(Day of week),表示不指定值,可以用于代替 *
例子
比如我们的手机卡假设都是在每个月的开始时间就更新资费:
"0 0 0 1 * *" // 表示每个月1号的00:00:00"0 1 1 1 * *" // 表示每个月1号的01:01:00
每隔5秒执行一次:"*/5 * * * * ?"
每隔1分钟执行一次:"0 */1 * * * ?"
每天23点执行一次:"0 0 23 * * ?"
每天凌晨1点执行一次:"0 0 1 * * ?"
每月1号凌晨1点执行一次:"0 0 1 1 * ?"
在26分、29分、33分执行一次:"0 26,29,33 * * * ?"
每天的0点、13点、18点、21点都执行一次:"0 0 0,13,18,21 * * ?"
pre-defined schedules
github.com/robfig/cron 包还给我门提供了一些预定义的模式:
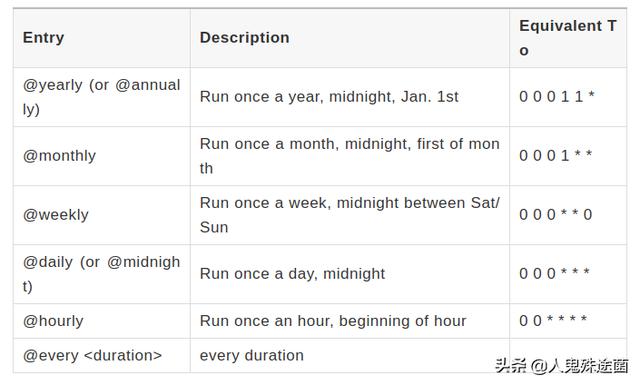
c.AddFunc("@every 1h30m", func() { fmt.Println("Every hour thirty") })
golang cron主要的设计思路
主要类型或接口说明
- Cron:包含一系列要执行的实体;支持暂停【stop】;添加实体等
type Cron struct {entries []*Entrystop chan struct{} // 控制 Cron 实例暂停add chan *Entry // 当 Cron 已经运行了,增加新的 Entity 是通过 add 这个 channel 实现的snapshot chan []*Entry // 获取当前所有 entity 的快照running bool // 当已经运行时为true;否则为false}
注意,Cron 结构没有导出任何成员。注意:有一个成员 stop,类型是 struct{},即空结构体。
2、Entry:调度实体
type Entry struct {// The schedule on which this job should be run.// 负责调度当前 Entity 中的 Job 执行Schedule Schedule// The next time the job will run. This is the zero time if Cron has not been// started or this entry's schedule is unsatisfiable// Job 下一次执行的时间Next time.Time// The last time this job was run. This is the zero time if the job has never// been run.// 上一次执行时间Prev time.Time// The Job to run.// 要执行的 JobJob Job}
3、Job:每一个实体包含一个需要运行的Job
这是一个接口,只有一个方法:Run
type Job interface { Run()}
由于 Entity 中需要 Job 类型,因此,我们希望定期运行的任务,就需要实现 Job 接口。同时,由于 Job 接口只有一个无参数无返回值的方法,为了使用方便,作者提供了一个类型:
type FuncJob func()
它通过简单的实现 Run() 方法来实现 Job 接口:
func (f FuncJob) Run() { f() }
这样,任何无参数无返回值的函数,通过强制类型转换为 FuncJob,就可以当作 Job 来使用了,AddFunc 方法 就是这么做的。
4、Schedule:每个实体包含一个调度器(Schedule)
负责调度 Job 的执行。它也是一个接口。
type Schedule interface { // Return the next activation time, later than the given time. // Next is invoked initially, and then each time the job is run. // 返回同一 Entity 中的 Job 下一次执行的时间 Next(time.Time) time.Time}
Schedule 的具体实现通过解析 Cron 表达式得到。
库中提供了 Schedule 的两个具体实现,分别是 SpecSchedule 和 ConstantDelaySchedule。
① SpecSchedule
type SpecSchedule struct {Second, Minute, Hour, Dom, Month, Dow uint64}
从开始介绍的 Cron 表达式可以容易得知各个字段的意思,同时,对各种表达式的解析也会最终得到一个 SpecSchedule 的实例。库中的 Parse 返回的其实就是 SpecSchedule 的实例(当然也就实现了 Schedule 接口)。
② ConstantDelaySchedule
type ConstantDelaySchedule struct {Delay time.Duration // 循环的时间间隔}
这是一个简单的循环调度器,如:每 5 分钟。注意,最小单位是秒,不能比秒还小,比如 毫秒。
通过 Every 函数可以获取该类型的实例,如:
constDelaySchedule := Every(5e9)
得到的是一个每 5 秒执行一次的调度器。
函数调用
- 函数
① 实例化 Cron
func New() *Cron {return &Cron{entries: nil,add: make(chan *Entry),stop: make(chan struct{}),snapshot: make(chan []*Entry),running: false,}}
可见实例化时,成员使用的基本是默认值;
② 解析 Cron 表达式
func Parse(spec string) (_ Schedule, err error)
spec 可以是:
- Full crontab specs, e.g. “* * * * * ?”
- Descriptors, e.g. “@midnight”, “@every 1h30m”
② 成员方法
// 将 job 加入 Cron 中// 如上所述,该方法只是简单的通过 FuncJob 类型强制转换 cmd,然后调用 AddJob 方法func (c *Cron) AddFunc(spec string, cmd func()) error// 将 job 加入 Cron 中// 通过 Parse 函数解析 cron 表达式 spec 的到调度器实例(Schedule),之后调用 c.Schedule 方法func (c *Cron) AddJob(spec string, cmd Job) error// 获取当前 Cron 总所有 Entities 的快照func (c *Cron) Entries() []*Entry// 通过两个参数实例化一个 Entity,然后加入当前 Cron 中// 注意:如果当前 Cron 未运行,则直接将该 entity 加入 Cron 中;// 否则,通过 add 这个成员 channel 将 entity 加入正在运行的 Cron 中func (c *Cron) Schedule(schedule Schedule, cmd Job)// 新启动一个 goroutine 运行当前 Cronfunc (c *Cron) Start()// 通过给 stop 成员发送一个 struct{}{} 来停止当前 Cron,同时将 running 置为 false// 从这里知道,stop 只是通知 Cron 停止,因此往 channel 发一个值即可,而不关心值是多少// 所以,成员 stop 定义为空 structfunc (c *Cron) Stop()
如果对每个方法调用还是不了解可以去看一下每隔函数的实现源码
例子:
package mainimport ( "log" "github.com/robfig/cron")type Hello struct { Str string}func(h Hello) Run() { log.Println(h.Str)}func main() { log.Println("Starting...") c := cron.New() h := Hello{"I Love You!"} // 添加定时任务 c.AddJob("*/2 * * * * * ", h) // 添加定时任务 c.AddFunc("*/5 * * * * * ", func() { log.Println("hello word") }) s, err := cron.Parse("*/3 * * * * *") if err != nil { log.Println("Parse error") } h2 := Hello{"I Hate You!"} c.Schedule(s, h2) // 其中任务 c.Start() // 关闭任务 defer c.Stop() select { }}