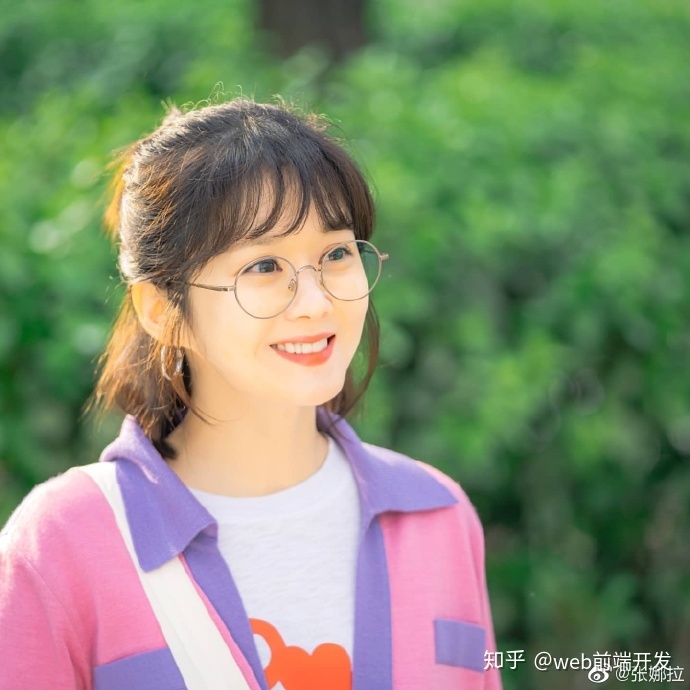
一、Vue.directive
Vue.directive(id,[definition]);
1)参数
{ string } id
{ Function | Object } [ definition ]
(2)用法
注册或获取全局指令。
<!-- 注册 -->
Vue.directive('my-directive',{
bind:function(){},
inserted:function(){},
update:function(){},
componentUpdated:function(){},
unbind:function(){},
})
<!-- 注册(指令函数) -->
Vue.directive('my-directive',function(){
<!-- 这里将会被bind和update调用 -->
})
<!-- getter方法,返回已注册的指令 -->
var myDirective = Vue.directive('my-directive');
(3) 除了核心功能默认内置的指令外(v-model和v-show),Vue.js也允许注册自定义指令。虽然代码复用和抽象的主要形式是组件,但是有些情况下,仍然需要对普通DOM元素进行底层操作,这时就会用到自定义指令。
(4)Vue.directive方法的作用是注册或获取全局指令,而不是让指令生效。其区别是注册指令需要做的事是将指令保存在某个位置,而让指令生效是将指令从某个位置拿出来执行它。
(5)实现
<!-- 用于保存指令的位置 -->
Vue.options = Object.create(null);
Vue.options['directives'] = Object.create(null);
Vue.directive = function(id,definition){
if(!definition){
return this.options['directive'][id];
}else{
if(typeof definition === 'function'){
definition = { bind: definition,update: definition};
}
this.optipns['directive'][id] = definition;
return definition;
}
}
1、在Vue构造函数上创建了options属性来存放选项,并在选项上新增了directive方法用于存在指令。
2、Vue.directive方法接受两个参数id和definition,它可以注册或获取指令,这取决于definition参数是否存在。
3、如果definition参数不存在,则使用id从this.options['directive']中读出指令并将它返回。
4、如果definition参数存在,则说明是注册操作,那么进而判断definition参数的类型是否是函数。
5、如果是函数,则默认监听bind和update两个事件,所以代码中将definition函数分别赋值给对象中的bind和update这两个方法,并使用这个对象覆盖definition。
6、如果definition不是函数,则说明它是用户自定义的指令对象,此时不需要做任何操作,直接将用户提供的指令对象保存在this.optipns['directive']上即可。
二、Vue.filter
Vue.filter(id,[definition]); (1)参数
{ string } id
{ Function | Object } [definition]
(2)用法
注册或获取全局过滤器
<!-- 注册 -->
Vue.filter('my-filter',function(value){
<!-- 返回处理后的值 -->
})
<!-- getter方法,返回已注册的过滤器 -->
var myFilter = Vue.filter('my-filter');
(3)Vue.js允许自定义过滤器,可被用于一些常见的文本格式化。过滤器可以用在两个地方:双花括号插值和v-bind表达式。过滤器应该被添加到Javascript表达式的尾部,由“管道”符号指示
<!-- 在双花括号中 -->
{{ message | capitalize }}
<!-- 在v-bind中 -->
<div v-bind:id="rawId | formatId "></div>
(4)Vue.filter的作用仅仅是注册或获取全局过滤器。
(5)实现
Vue.options['filters'] = Object.create(null);
Vue.filter = function(id,definition){
if(!definition){
return this.options['filters'][id];
}else{
this.optipns['filters'][id] = definition;
return definition;
}
}
1、在Vue.options中新增了filters属性用于存放过滤器,并在Vue.js上新增了filter方法,它接受两个参数id和definition。
2、Vue.filters方法可以注册或获取过滤器,这取决于definition参数是否存在。
3、如果definition不存在,则使用id从this.options['filters']中读出过滤器并将它返回。
4、如果definition存在,则说明是注册操作,直接将该参数保存到this.optipns['filters']中。
三、Vue.component
Vue.component(id,[definition]);
1)参数
{ string } id
{ Function | Object } [definition]
(2)用法
注册或获取全局组件。注册组件时,还会自动使用给定的id设置组件的名称。
<!-- 注册组件,传入一个扩展过的构造器 -->
Vue.component('my-component',Vue.extend({/*...*/}));
<!-- 注册组件,传入一个选项对象(自动调用Vue.extend) -->
Vue.component('my-component',{/*...*/});
<!-- 获取注册的组件(始终返回构造器) -->
var MyComponent = Vue.component('my-component');
Vue.extend前面我们已经讲过,不了解的可以看下这篇文章: 学习vue源码(2) 手写Vue.extend方法
(3)Vue.component只是注册或获取组件
(4)原理
Vue.options['components'] = Object.create(null);
Vue.component = function(id,definition){
if(!definition){
return this.options['components'][id];
}else{
if(isPlainObject(definition)){
definition.name = definition.name || id;
definition = Vue.extend(definition);
}
this.optipns['components'][id] = definition;
return definition;
}
}
1、在Vue.options中新增了components属性用于存放组件,并在Vue.js上新增了component方法,它接收两个参数id和definition。
2、Vue.component方法可以注册或获取过滤器,这取决于definition参数是否存在。
3、如果definition不存在,则使用id从this.options['components']中读出组件并将它返回。
4、如果definition存在,则说明是注册操作,那么需要将组件保存到this.optipns['components']中。
5、由于definition参数支持两种参数,分别是选项对象和构造器,而组件其实是一个构造函数,是使用Vue.extend生成的子类,所以需要将参数definition同一处理成构造器。
6、如果definition参数是Object类型,则调用Vue.extend方法将它变成Vue的子类,使用Vue.component方法注册组件。
7、如果选项对象中没有设置组件名,则自动使用给定的id设置组件的名称。
四、合并Vue.directive、Vue.filter、Vue.component代码
Vue.options = Object.create(null);
<!-- ASSET_TYPES = ['component','directive','filter'] -->
ASSET_TYPES.forEach(type=>{
Vue.options[type+'s'] = Object.create(null);
})
ASSET_TYPES.forEach(type=>{
Vue[type] = function(id,definition){
if(!definition){
return this.options[type+'s'][id];
}else{
<!-- 组件 -->
if(type==='component' && isPlainObject(definition)){
definition.name = definition.name || id;
definition = Vue.extend(definition);
}
<!-- 指令 -->
if(type==='directive' && typeof definition === 'function'){
definition = { bind: definition,update: definition};
}
this.optipns['components'][id] = definition;
return definition;
}
}
})
文章参考:深入浅出Vue