HTTP fetch发送2次请求的原因?面对这道出现频率较高的面试题,我想说的是:发送两次请求的情况确实存在,但这与你所使用的是不是http协议,所采用的是不是fetch真的没有一毛钱关系!
接下来,咱们可以通过代码一一去验证……
一、准备工作
1、创建一个文件夹zhangpeiyue
2、在zhangpeiyue
文件夹内创建两个文件:server.js
与index.html
•
server.js
:搭建一个express服务器,用于提供接口•
index.html
:通过fetch调用接口。
二、前后端符合同源策略的场景
1、通过server.js
创建服务:
const express = require("express");// 通过 body-parser 接收 post 过来的数据const bodyParser = require("body-parser");const app = express();// 接收 post 的数据为 application/json 格式app.use(bodyParser.json());// 将当前文件夹设置为静态资源app.use(express.static(__dirname));app.post("/my",(req,res)=>{ res.json({ ok:1, body:req.body// 将接收到的数据返回给前端 })});app.listen(80,(err)=>{ console.log("success");})
2、启动服务
node server
3、
index.html
嵌入
js
:
// 为避免出现缓存,增加 t 参数fetch("http://127.0.0.1/my?t="+Date.now(),{ method:"post", body:JSON.stringify({ a:1, b:2 }), headers:{ "content-type":"application/json" }}).then(res=>res.json()) .then(response=>console.log("Success:",response)) .catch(error=>console.log("Error",error))
4、浏览器打开
http://127.0.0.1
测试
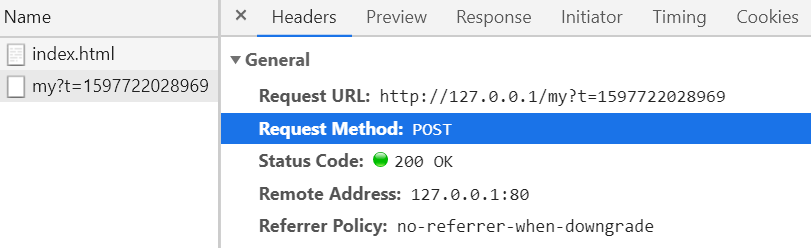
5、结论
• 在同源的情况下并未出现请求两次的情况三、fetch
在跨域的情况下
1、server.js
修改如下:
const express = require("express");// 通过 body-parser 接收 post 过来的数据const bodyParser = require("body-parser");const app = express();// 接收 post 的数据为 application/json 格式app.use(bodyParser.json());// 将当前文件夹设置为静态资源app.use(express.static(__dirname));app.all("*",(req,res,next)=>{ console.log(req.method); res.set({ "Access-Control-Allow-Origin":"*", "Access-Control-Allow-Headers":"content-type" }) next();})app.post("/my",(req,res)=>{ res.json({ ok:1, body:req.body// 将接收到的数据返回给前端 })});app.listen(80,(err)=>{ console.log("success");})
2、将前端
content-type
设置为
application/json
,然后通过开发工具的
http
方式在浏览器打开
index.html
,或自己重新创建一个服务,在浏览器打开
index.html
。你会发现其果然请求了两次,分别为
OPTIONS
请求与
POST
请求:
// 为避免出现缓存,增加 t 参数fetch("http://127.0.0.1/my?t="+Date.now(),{ method:"post", body:JSON.stringify({ a:1, b:2 }), headers:{ "content-type":"application/json" }}).then(res=>res.json()) .then(response=>console.log("Success:",response)) .catch(error=>console.log("Error",error))
•
请求方式:
OPTIONS
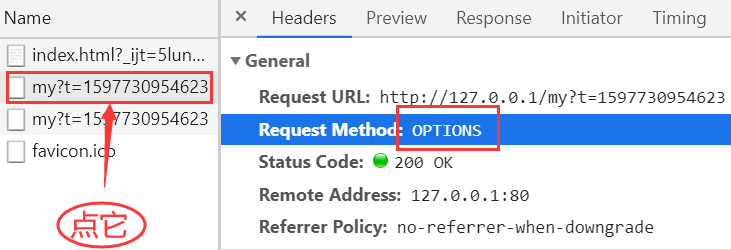
POST
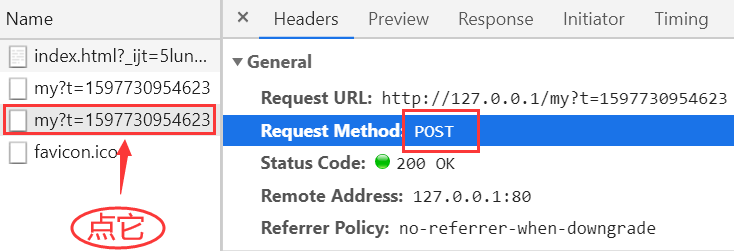
3、将js代码中的content-type
注释掉,然后在非同源的场景下再次访问,你会发现只发送了一次post
请求。
// 为避免出现缓存,增加 t 参数fetch("http://127.0.0.1/my?t="+Date.now(),{ method:"post", body:JSON.stringify({ a:1, b:2 }), headers:{ // "content-type":"application/json" }}).then(res=>res.json()) .then(response=>console.log("Success:",response)) .catch(error=>console.log("Error",error))
•
只发送了
post
请求:
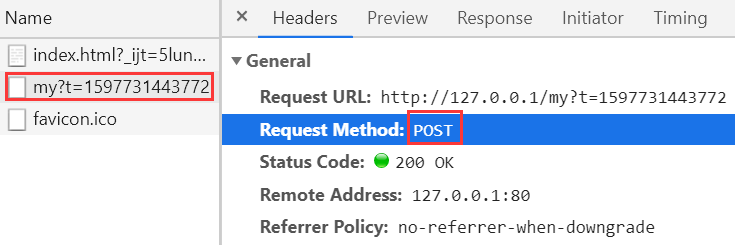
4、将content-type
更改为application/x-www-form-urlencoded
,再次访问,依然只发送了一次POST
请求:
// 为避免出现缓存,增加 t 参数fetch("http://127.0.0.1/my?t="+Date.now(),{ method:"post", body:JSON.stringify({ a:1, b:2 }), headers:{ "content-type":"application/x-www-form-urlencoded" }}).then(res=>res.json()) .then(response=>console.log("Success:",response)) .catch(error=>console.log("Error",error))
•
只发送了
post
请求:
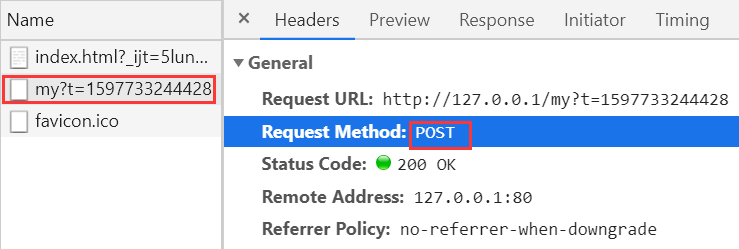
5、将fetch
改为XMLHttpRequest
。将content-type
设置为application/json
。打开index.html
,此时会请求两次,分别为OPTIONS
请求与POST
请求:
const xhr = new XMLHttpRequest();xhr.open("post","http://127.0.0.1/my?t="+Date.now());xhr.setRequestHeader("content-type","application/json")xhr.send(JSON.stringify({a:1,b:2}));xhr.onload = function () { console.log(xhr.responseText)}
•
请求方式:
OPTIONS
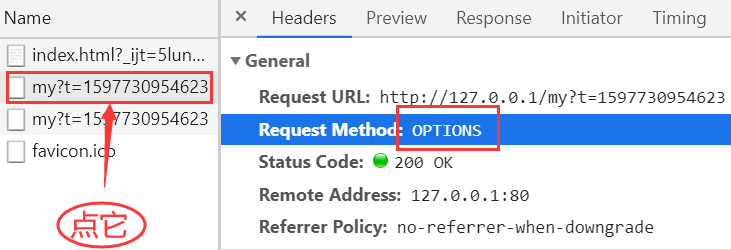
POST
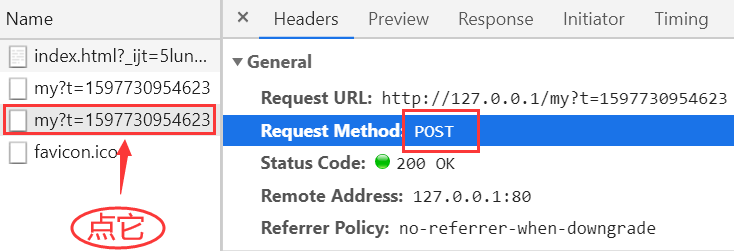
6、将配置content-type
的代码注释掉,结果只发送了一次POST
请求:
const xhr = new XMLHttpRequest();xhr.open("post","http://127.0.0.1/my?t="+Date.now());// xhr.setRequestHeader("content-type","application/json")xhr.send(JSON.stringify({a:1,b:2}));xhr.onload = function () { console.log(xhr.responseText)}
•
请求方式:
POST
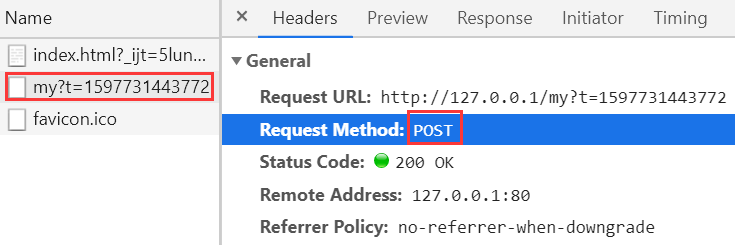
四、接口的协议为https:
1、server.js
:
const express = require("express");// 通过 body-parser 接收 post 过来的数据const bodyParser = require("body-parser");const https = require("https");const fs = require("fs");const app = express();// 创建 https 服务,需要证书与密钥(需要有自己的域名)const httpsServer = https.createServer({ key:fs.readFileSync(__dirname+"/key/weixin.key"), cert:fs.readFileSync(__dirname+"/key/weixin.crt")},app)// 接收 post 的数据为 application/json 格式app.use(bodyParser.json());app.all("*",(req,res,next)=>{ console.log(req.method); res.set({ "Access-Control-Allow-Origin":"*" }) next();})app.post("/my",(req,res)=>{ res.json({ ok:1, body:req.body// 将接收到的数据返回给前端 })});httpsServer.listen(443,()=>{ console.log("httpsServer->success")})
2、
content-type
设置为
application/json
,然后以
http
的形式打开页面。结果会请求两次,分别为
OPTIONS
请求与
POST
请求:
// 为避免出现缓存,增加 t 参数fetch("https://weixin.zhangpeiyue.com/my?t="+Date.now(),{ method:"post", body:JSON.stringify({ a:1, b:2 }), headers:{ "content-type":"application/json" }}).then(res=>res.json()) .then(response=>console.log("Success:",response)) .catch(error=>console.log("Error",error))
•
请求方式:
OPTIONS
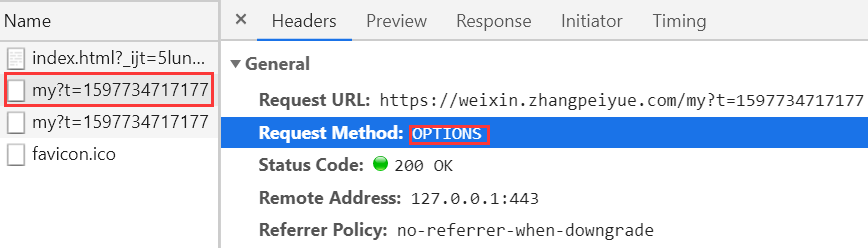
POST
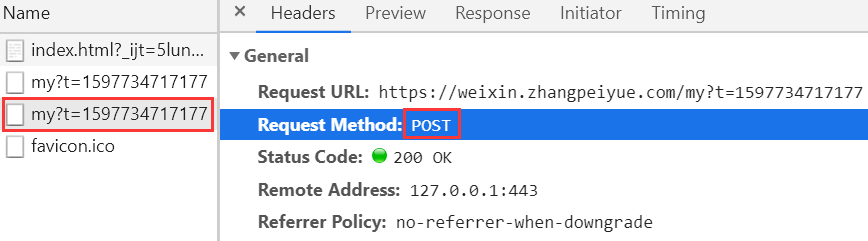
五、总结
发送2次请求需要满足以下2个条件:
1、必须要在跨域的情况下。 2、除GET、HEAD和POST(only with application/x-www-form-urlencoded, multipart/form-data, text/plain Content-Type)以外的跨域请求(我们可以称为预检(Preflighted)的跨域请求)。最后,建议大家可以这样回复面试官:之所以会发送2次请求,那是因为我们使用了带预检(Preflighted)的跨域请求。该请求会在发送真实的请求之前发送一个类型为OPTIONS的预检请求。预检请求会检测服务器是否支持我们的真实请求所需要的跨域资源,唯有资源满足条件才会发送真实的请求。比如我们在请求头部增加了authorization项,那么在服务器响应头中需要放入Access-Control-Allow-Headers,并且其值中必须要包含authorization,否则OPTIONS预检会失败,从而导致不会发送真实的请求。
分享就到这里了,如果这篇文章对你有所帮助的话,欢迎点赞、转发、点再看,在此谢过~