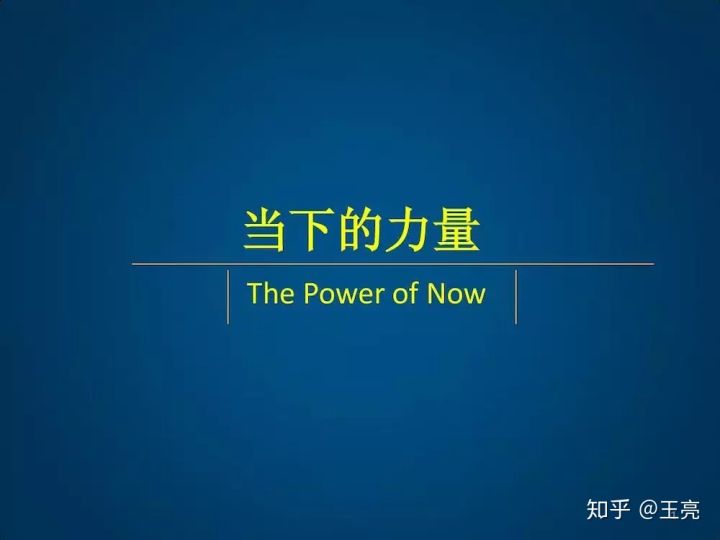
链表分为两种,静态链表和动态链表
一、静态链表
#include <stdio.h>
#include <string.h>
struct stu{
char name[20];
int num;
struct stu *next;
};
int main()
{
struct stu s1,s2,s3,*p,*head;
int n=0;
strcpy(s1.name, "litao");
s1.num =2019001;
strcpy(s2.name, "huitao");
s2.num =2019002;
strcpy(s3.name, "qiantao");
s3.num =2019003;
head = &s1;
s1.next = &s2;
s2.next = &s3;
s3.next = NULL ;
p =head;
while(p!=NULL)
{
n++;
printf("%d节点信息:姓名:%st 学号:%dn",n,p->name,p->num);
p=p->next ;
}
return 0;
}
二、分配一块区域,输入一个学生数据
#include <stdio.h>
#include <stdlib.h>
struct stu
{
char name[20];
int num;
float score;
struct stu *next;
};
int main()
{
struct stu *p;
p = (struct stu *)malloc(sizeof(struct stu)); //动态开辟一个stu结构类型存储空间,并把首地址赋给指针p;
//输入数据
printf("姓名:");
gets(p->name);
printf("学号和分数:");scanf("%d%f",&p->num,&p->score);
printf("输出信息:n");
printf("姓名:%st学号:%dt分数:%fn",p->name,p->num,p->score);
free(p);
return 0;
}
本程序,定义了结构stu,定义了stu结构体类型指针变量p。然后分配一块stu内存区,并把首地址赋予p,使指针指向该区域。再以p为指向结构类型的指针变量对各成员赋值,并用printf输出各成员的值, 最后用free函数释放指针变量p指向的内存空间。整个程序包含了申请内存的空间、使用内存空间、释放内存空间三个步骤,实现存储空间的动态分配。
三、创建单链表及遍历链表(在主函数完成)
#include <stdio.h>
#include <malloc.h>
struct stu
{
char name[20]; int score;
struct stu *next;
};
int main()
{
struct stu *p,*q,*head;
int n, i=0;
printf("输入学生人数:");
scanf("%d",&n);
while(i<n)
{
p = (struct stu *)malloc(sizeof(struct stu));
printf("输入学生%d信息:",i+1);
scanf("%s%d",p->name,&p->score);
if(i==0)
{
head=q=p; q->next =NULL;
}
else
{
p->next =NULL; q->next = p;
q = p;
}
i++;
}
p = head;i=1;
while(p!=NULL)
{
printf("学生%d姓名:%st成绩:%dn",i,p->name,p->score);
i++;
p = p->next;
}
free(p);
}
四、创建单链表及遍历链表
分别利用创建creatlink函数,打印printlink函数
#include <stdio.h>
#include <stdlib.h>
struct stu
{
char name[20];
int score;
struct stu *next;
};
struct stu *creatLink(struct stu *head) //注意返回类型是同类型指针
{
struct stu *p,*q;
int n ; //表示输入人数
int i=0;
printf("输入学生人数:");
scanf("%d",&n);
while(i<n)
{
p =(struct stu *)malloc(sizeof(struct stu));
printf("学生%d:",i+1);
scanf("%s%d",p->name,&p->score);
if(i==0)
{
head=q=p;
q->next = NULL;
}
else
{
p->next = NULL;
q->next = p;
q = p;
}
i++;
}
return head;
}
void printLink(struct stu *head)
{
struct stu *p;
int n=0;
p = head;
if(head == NULL)
{ printf("空链表");exit(0);}
else
{
while(p!=NULL)
{
printf("学生%d姓名:%st 成绩:%dn",n+1,p->name,p->score);
n++;
p = p->next;
}
}
}
int main()
{
struct stu *head;
head = NULL; //注意这里必须要赋值,空指针
printf("创建链表n");
head = creatLink(head);
printf("链表创建完成,输出学生信息:n");
printLink(head);
return 0;
}
五、查找姓名函数findstu
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
struct stu
{
char name[20];
int score;
struct stu *next;
};
struct stu *creatLink(struct stu *head)
{
struct stu *p,*q;
int n ; //表示输入人数
int i=0;
printf("输入学生人数:");
scanf("%d",&n);
while(i<n)
{
p =(struct stu *)malloc(sizeof(struct stu));
printf("学生%d:",i+1);
scanf("%s%d",p->name,&p->score);
if(i==0)
{
head=q=p;
q->next = NULL;
}
else
{
p->next = NULL;
q->next = p;
q = p;
}
i++;
}
return head;
}
void printLink(struct stu *head)
{
struct stu *p;
int n=0;
p = head;
if(head == NULL)
{ printf("空链表");exit(0);}
else
{
while(p!=NULL)
{
printf("学生%d姓名:%st 成绩:%dn",n+1,p->name,p->score);
n++;
p = p->next;
}
}
}
void findstu(struct stu *head, char *ch)
{
struct stu *p;
p = head;
if(head == NULL)
{ printf("这是空链表,请返回。n"); exit(0);}
while(p!=NULL && strcmp(p->name,ch)!=0)
p = p->next;
if(p!=NULL)
{
printf("%s同学信息已找到!完整信息如下:",p->name);
printf("姓名:%st分数:%dn",p->name,p->score);
}
else
printf("没有找到该学生的信息n");
}
int main()
{
struct stu *head;
char name[20];
head = NULL;
printf("创建链表n");
head = creatLink(head);
printf("链表创建完成n");
printf("n请输入要查找学生的姓名:");
scanf("%s",name); //不能用gets,要注意了
findstu(head,name);
printf("输出学生信息:n");
printLink(head);
return 0;
}
六、删除节点
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
struct stu
{
char name[20];
int score;
struct stu *next;
};
struct stu *creatLink(struct stu *head)
{
struct stu *p,*q;
int n ; //表示输入人数
int i=0;
printf("输入学生人数:");
scanf("%d",&n);
while(i<n)
{
p =(struct stu *)malloc(sizeof(struct stu));
printf("学生%d:",i+1);
scanf("%s%d",p->name,&p->score);
if(i==0)
{
head=q=p;
q->next = NULL;
}
else
{
p->next = NULL;
q->next = p;
q = p;
}
i++;
}
return head;
}
void printLink(struct stu *head)
{
struct stu *p;
int n=0;
p = head;
if(head == NULL)
{ printf("空链表");exit(0);}
else
{
while(p!=NULL)
{
printf("学生%d姓名:%st 成绩:%dn",n+1,p->name,p->score);
n++;
p = p->next;
}
}
}
void findstu(struct stu *head, char *ch)
{
struct stu *p;
p = head;
if(head == NULL)
{ printf("这是空链表,请返回。n"); exit(0);}
while(p!=NULL && strcmp(p->name,ch)!=0)
p = p->next;
if(p!=NULL)
{
printf("%s同学信息已找到!完整信息如下:",p->name);
printf("姓名:%st分数:%dn",p->name,p->score);
}
else
printf("没有找到该学生的信息n");
}
struct stu *delnode(struct stu *head ,char *ch)
{
struct stu *p,*q;
q = head;
p = head;
if(head == NULL)
{
printf("这是空链表,请返回!");exit(0);
}
while(p!=NULL)
{
if(strcmp(p->name,ch)!=0)
{
q = p;
p = p->next;;
}
else
break;
}
if(p == head)
{
head = p->next;
}
else if(p == NULL)
{
printf("没有找到该学生。n");
}
else
{
q->next = p->next; free(p);printf("节点已被删除n");
}
return head;
}
int main()
{
struct stu *head;
char name[20];
head = NULL;
printf("创建链表n");
head = creatLink(head);
printf("链表创建完成n");
printf("n请输入要查找学生的姓名:");
scanf("%s",name);
findstu(head,name);
printf("n请输入要删除学生的姓名:n");
scanf("%s",name);
head=delnode(head,name);
printf("输出学生信息:n");
// findstu(head,name);
printLink(head);
return 0;
}
7. 添加学生信息
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define LEN sizeof(struct student)
struct student
{
int no;
char name[20];
int score;
struct student *next;
};
struct student *creatlist(struct student *head)
{
struct student *p,*q; //p为开辟新节点,q为指向链表最后一个节点
int n ; //表示输入学生节点个数;
int i=0;
printf("输入学生信息的个数:");
scanf("%d",&n);
while(i<n)
{
p = (struct student *)malloc(LEN);
printf("输入学生%d信息:",i+1);
scanf("%s%d%d",p->name,&p->no,&p->score);
if(i==0)
{
head=q=p;
q->next = NULL;
}
else
{
p->next = NULL;
q->next = p;
q = p;
}
i++;
}
return head;
}
void printlist(struct student *head)
{
struct student *p = head;
int n=0;
if(head == NULL )
{
printf("这是空链表,请返回!"); exit(0);
}
while(p!=NULL)
{
printf("学生%d信息:n学生姓名:%st学号:%dt成绩:%dn",n+1,p->name,p->no,p->score);
n++;
p = p->next;
}
}
void findStudent(struct student *head,char *str)
{
struct student *p;
p = head;
if(head == NULL)
{
printf("这是空链表,请返回!"); exit(0);
}
while(p!=NULL && strcmp(p->name ,str)!=0)
p = p->next;
if(p==NULL) //表示到表尾
{ printf("没有找到该学生信息!");}
else
{
printf("该学生的信息已找到,其完整信息如下:n");
printf("学生姓名:%st学号:%dt成绩:%dn",p->name,p->no,p->score);
}
}
struct student *delstudent(struct student *head, char name[])
{
struct student *p,*q;
p = head;
q = head;
if(head == NULL)
{
printf("错误,链表是空的!");exit(0);
}
while(p!=NULL)
{
if(strcmp(p->name,name)!=0)
{
q = p;
p = p->next;;
}
else
break;
}
if(p == head)
{
head = p->next; free(p);printf("节点已被删除n");
}
else if(p == NULL) //到表尾
{
printf("没有找到该学生。n");
}
else
{
q->next = p->next; free(p);printf("节点已被删除n");
}
return head;
}
struct student *insertStu(struct student *head, struct student *node)
{
struct student *p,*q;
p = head;
while(node->no > p->no && p->next!=NULL)
{
q = p;
p = p->next; //要找到插入位置
}
if(p == head) //在第一个节点之前插入
{ head = node; node->next = p;}
else if(p->next == NULL)
{
p->next = node ; //在表尾插入
node->next = NULL;
}
else
{
q->next = node; //在其它位置插入
node->next = p;
}
return head;
}
int main()
{
struct student *head,*s;
char name[20];
head = NULL;
printf("创建链表!n");
head = creatlist(head);
printf("n请输入要查找学生姓名:");
scanf("%s",name);
findStudent(head,name);
printf("n请输入要删除学生姓名:");
scanf("%s",name);
head = delstudent(head,name);
printf("n请输入要添加学生信息:");
s = (struct student *)malloc(LEN);
printf("学生姓名:");scanf("%s",s->name);
printf("n学号和分数:");scanf("%d%d",&s->no,&s->score);
head = insertStu(head,s);
printf("n输出学生信息:n");
printlist(head);
return 0;
}