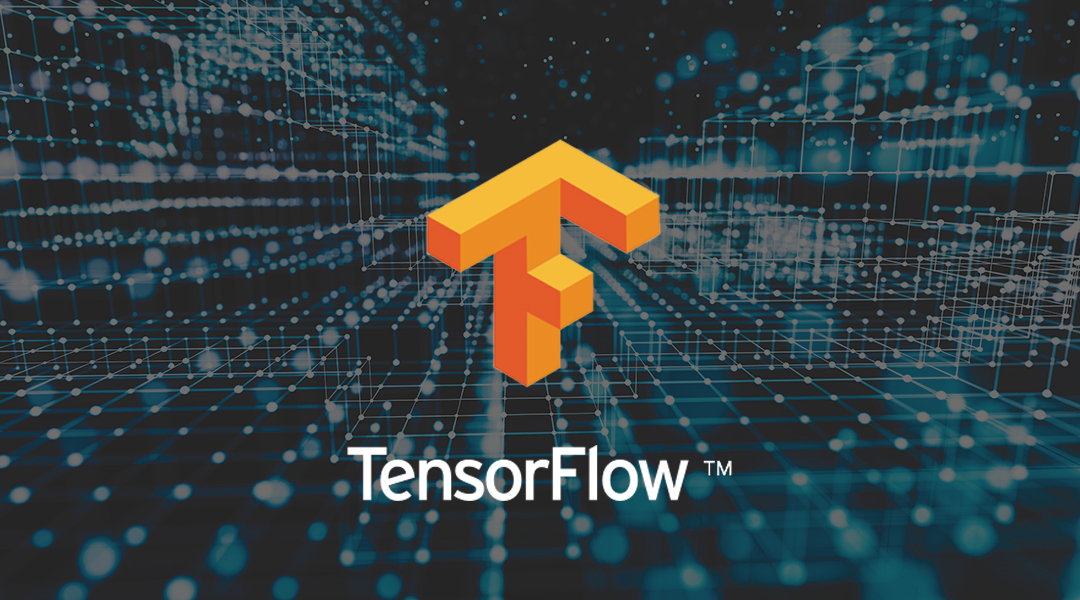
一、Tensorflow导入
我们的代码主要是针对Tensorflow 1.x版本的环境,在Tensorflow 2.x下有部分代码无法正常运行,因此若是Tensorflow 1.x版本,只需简单使用import tensorflow as tf
即可,而对于2.x版本的Tensorflow,我们可以将运行环境转换为1.x,并disable 2.x的特性:
import tensorflow.compat.v1 as tf
tf.disable_v2_behavior
也许有小伙伴不记得自己装过Tensorflow的版本,可以使用tf.__version__
查看,下面我们都默认大家使用的是1.x的Tensorflow版本。
二、Tensorflow三个核心概念
2.1 Graph
在Tensorflow中,计算图是一个有向图,用来描述计算节点一集计算节点之间的关系,所以在TensorFlow中我们存储一个值或者数组的时候,存的其实是这个值或者数组的计算图而不是其本身的数字
关于计算图的操作:
- 新建计算图:
g=tf.Graph()
,但是不同计算图上的张量是不能共享的,这个是存在于变量 - 指定计算图使用的device:
with g.device("/gpu:0")
- 设置默认计算图:
with g.as_default:
- 在会话中可以指定使用的计算图:
with tf.Session(graph=g1)
下面是示例代码,大家可以自己运行一下:
g = tf.Graph()
tf.compat.v1.disable_v2_behavior()
tf.compat.v1.get_default_graph()
with g.device(0):
a = tf.constant([10, 9, 8, 7])
b = tf.constant([1, 2, 3, 4])
c_1 = a + b
print(c_1.graph)
print(a.graph, b.graph)
sess = tf.compat.v1.Session()
print(sess.run(c_1))
a.graph
等的输出应类似<tensorflow.python.framework.ops.Graph object at 0x00000167399CEA90>
,sess.run
则是运行a+b
这个计算
若没有使用GPU,也可以不指定device:
a = tf.constant([1, 2, 3, 4], name="a")
b = tf.constant([2, 3, 5, 6], name="b")
c = a + b
print(a.graph, b.graph, c.graph)
sess = tf.compat.v1.Session()
print(sess.run(c))
下面定义多个图,会发现在后面Session
运行时若指定g1
将会报错,这是因为两次创建的图不一致:
g1 = tf.Graph()
with g1.as_default():
a = tf.constant([1, 2, 3], name="a")
b = tf.get_variable("b", initializer=tf.constant_initializer()(shape=[1]))
g2 = tf.Graph()
with g2.as_default():
a = tf.constant([2, 3], name="a")
b = tf.get_variable("b", initializer=tf.constant_initializer()(shape=[3]))
with tf.Session(graph=g2) as sess:
tf.global_variables_initializer().run()
c = a + 1
print("常量的情况下", sess.run(c))
with tf.variable_scope("", reuse=True):
print("变量情况下", sess.run(tf.get_variable("b")))
with tf.Session(graph=g2) as sess:
tf.global_variables_initializer().run()
c = a + 1
print("常量的情况下", sess.run(c))
with tf.variable_scope("", reuse=True):
print("变量情况下", sess.run(tf.get_variable("b")))
2.2 Tensor
张量可以简单理解为多维数组,其中零阶张量表示标量,一阶张量为向量,第n
阶张量可以理解为一个n
维数组,但是张量在Tensorflow中的实现并不是直接采用数组的形式,它只是对Tensorflow中运算结果的引用,在张量中并没有真正保存数字,它保存的是如何得到这些数字的计算过程。
a = tf.constant(2, name="a")
b = tf.constant([2, 3, 4], name="b")
c = a * b
with tf.Session() as sess:
print(sess.run(c))
2.3 Session
在Tensorflow中,计算图的计算过程都是在会话下进行的,同一个会话内的数据是可以共享的,会话结束计算的中间量就会消失,在Tensorflow中需要指定会话。Session
有三种调用方式:
最常用的一种:
with tf.Session() as sess:
sess.run(result)
另外不太常见的使用方式如下:
sess = tf.Session()
with sess.as_default():
print(result.eval())
还有一种交互式的使用方式(保证安全性),此时会生成默认会话,不需指定:
sess = tf.InteractiveSession()
三、Tensorflow常用基础API
3.1 简单API
tf.size() # This operation returns an integer representing the number of elements in input.
tf.shape() # Returns the shape of a tensor.
tf.reshape() # Reshapes a tensor.
tf.squeeze() # Removes dimensions of size 1 from the shape of a tensor.
tf.expand_dims() # Inserts a dimension of 1 into a tensor's shape.
tf.concat() # Concatenates tensors along one dimension.
tf.split() # Splits a tensor into sub tensors.
tf.tile() # Constructs a tensor by tiling a given tensor.
tf.slice() # Extracts a slice from a tensor.
tf.stack() # Stacks a list of rank-R tensors into one rank-(R+1) tensor. v1.0之前为tf.pack()
tf.gather() # Gather slices from params according to indices.
tf.pad() # Pads a tensor.
tf.reduce_sum() # Computes the sum of elements across dimensions of a tensor.
tf.reduce_mean() # Computes the mean of elements across dimensions of a tensor.
tf.reduce_max() # Computes the maximum of elements across dimensions of a tensor.
tf.argmax() # Returns the index with the largest value across axes of a tensor.
tf.argmin() # Returns the index with the smallest value across axes of a tensor.
tf.fill() # Creates a tensor filled with a scalar value.
tf.random_shuffle() # Randomly shuffles a tensor along its first dimension.
tf.truncated_normal()
tf.random_normal()
3.2 tf.InteractiveSession()
tf.InteractiveSession()
是一种交互式的session
方式,它让自己成为了默认的session
,也就是说用户在不需要指明用哪个session
运行的情况下,就可以运行起来,这就是默认的好处。这样的话就是run()
和eval()
函数可以不指明session
啦。
import tensorflow as tf
import numpy as np
a=tf.constant([[1., 2., 3.],[4., 5., 6.]])
b=np.float32(np.random.randn(3,2))
c=tf.matmul(a,b)
init=tf.global_variables_initializer()
sess=tf.InteractiveSession()
print (c.eval())
若想使用tf.Session()
,则必须加上with sess.as_default():
3.3 tf.nn
模块
该模块的内容由于知乎不支持表格,需要阅读或复制的可以移步我的公众号链接:Tensorflow基础知识介绍(一) ,下面直接截图展示:
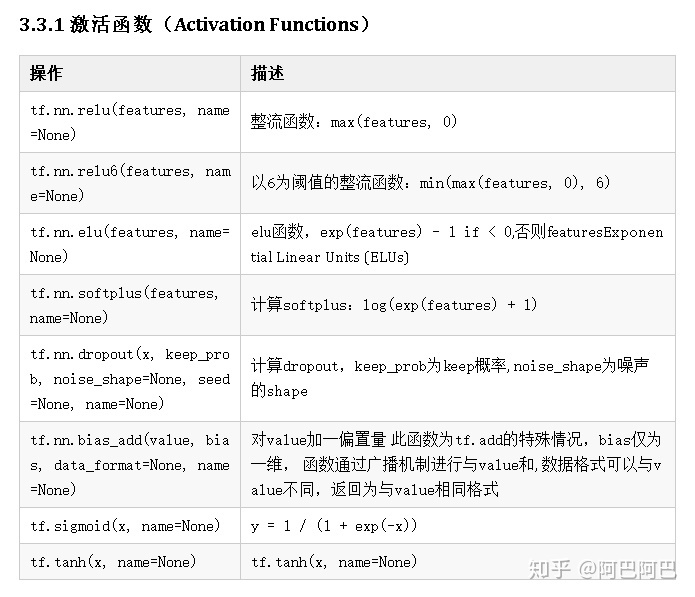
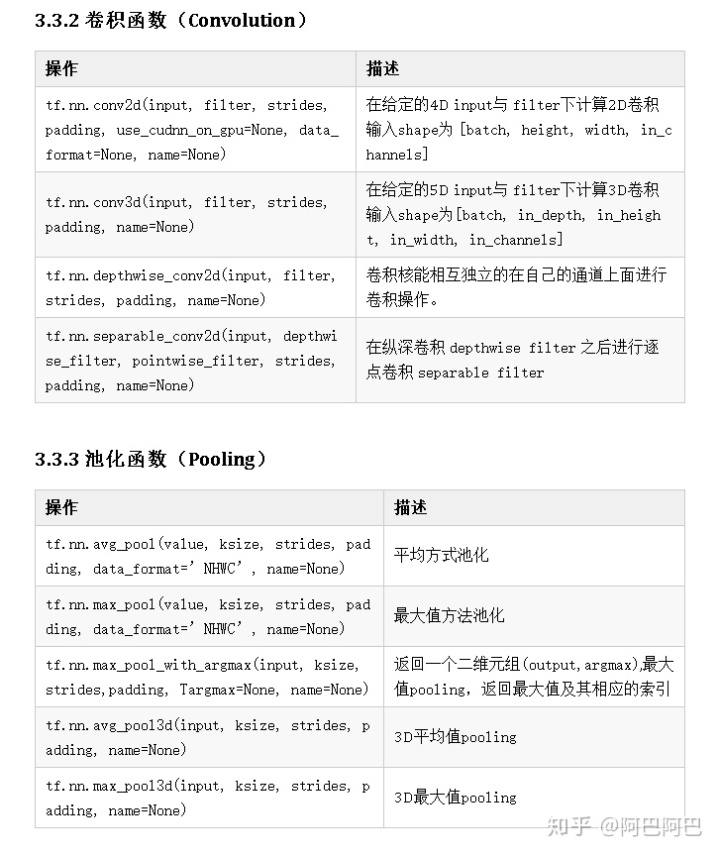
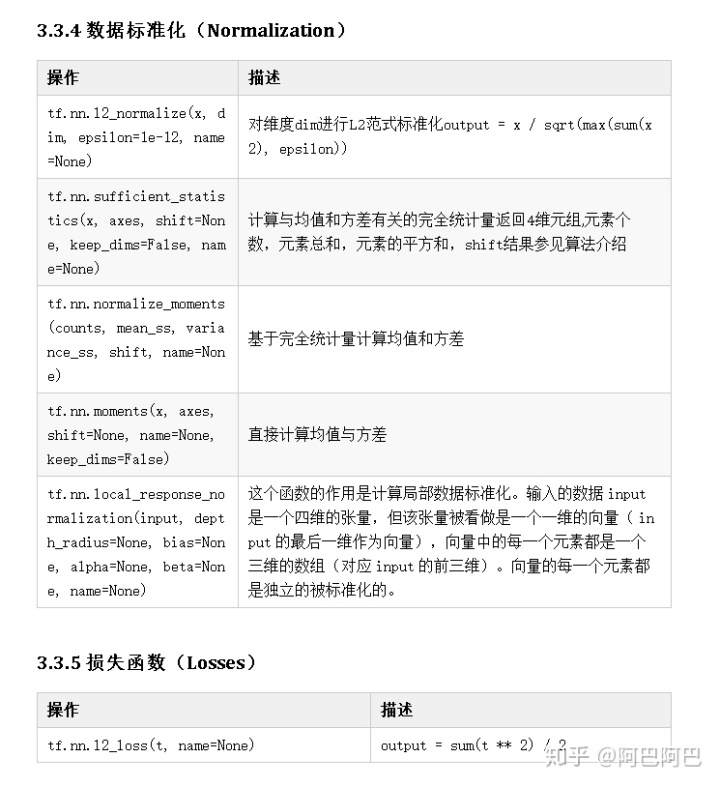
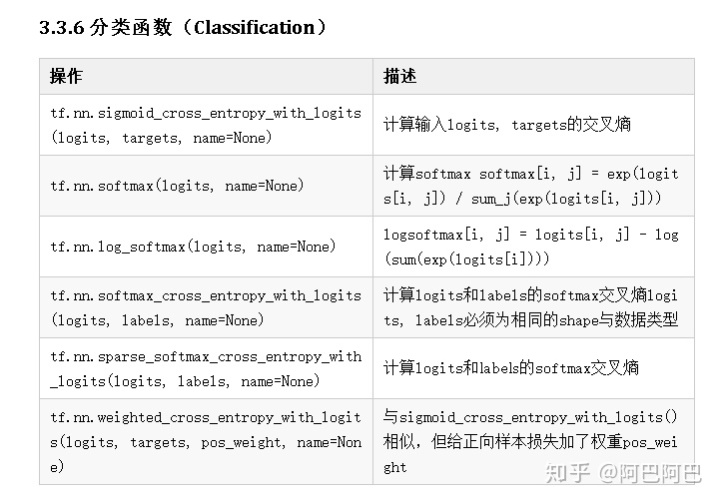