一、 HttpClient 简介
HttpClient 是 Apache Jakarta Common 下的子项目,可以用来提供高效的、最新的、 功能丰富的支持 HTTP 协议的客户端编程工具包,并且它支持 HTTP 协议最新的版本和 建议。
简介
HTTP 协议可能是现在 Internet 上使用得最多、最重要的协议了,越来越多的 Java 应用程序需要直接通过 HTTP 协议来访问网络资源。虽然在 JDK 的 java net 包中已经提 供了访问 HTTP 协议的基本功能,但是对于大部分应用程序来说,JDK 库本身提供的功能 还不够丰富和灵活。
二、 HttpClient 应用
创建jar工程,修改 pom.xml 文件添加 HttpClient 坐标
<dependencies>
<!-- https://mvnrepository.com/artifact/org.apache.httpcomponents/httpclient -->
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
<version>4.3.5</version>
</dependency>
</dependencies>
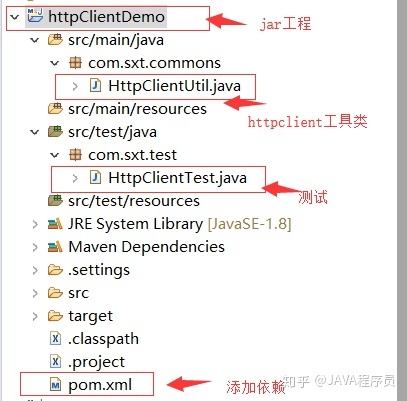
1 发送 GET 请求不带参数
编写测试代码
public class HttpClientTest {
public static void main(String[] args) throws Exception {
HttpClientTest.doGet();
//HttpClientTest.doGetParam();
//HttpClientTest.doPostTest();
//HttpClientTest.doPostParamTest();
//HttpClientTest.doPostParamJsonTest();
//HttpClientTest.HttpClientUtilTest();
}
------------------------------------------------------------------------------------------
// get请求不带参数
public static void doGet() throws Exception {
// 创建一个httpclient对象
CloseableHttpClient cd = HttpClients.createDefault();
// 创建get请求对象,在请求中输入url
HttpGet get = new HttpGet("http://www.baidu.com");
// 发送请求,并返回响应
CloseableHttpResponse res = cd.execute(get);
// 处理响应
// 并获取响应的状态码
int code = res.getStatusLine().getStatusCode();
System.out.println(code);
// 获取响应内容
HttpEntity entity = res.getEntity();
String content = EntityUtils.toString(entity, "utf-8");
System.out.println(content);
//关闭连接
cd.close();
}
2 发送 GET 请求带参数
// get请求带参数
public static void doGetParam() throws Exception {
// 创建一个httpclient对象
CloseableHttpClient cd = HttpClients.createDefault();
//创建一个封装url对象,在该对象中可以给定请求参数
URIBuilder bui=new URIBuilder("https://www.sogou.com/web");
bui.addParameter("query", "西游记");
//创建一个get请求对象
HttpGet get=new HttpGet(bui.build());
// 发送请求,并返回响应
CloseableHttpResponse res = cd.execute(get);
// 处理响应
// 并获取响应的状态码
int code = res.getStatusLine().getStatusCode();
System.out.println(code);
// 获取响应内容
HttpEntity entity = res.getEntity();
String content = EntityUtils.toString(entity, "utf-8");
System.out.println(content);
//关闭连接
cd.close();
}
发送 POST(准备)
创建父工程(pom)和子工程(war)添加相应的pom.xml依赖。完善springmvc和web.xml文件
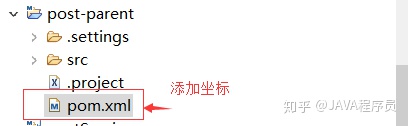
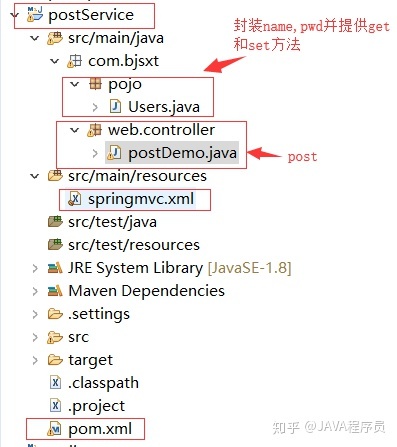
postDemo代码
package com.bjsxt.web.controller;
import java.util.HashMap;
import java.util.Map;
import javax.print.attribute.standard.Media;
import org.springframework.http.MediaType;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.ResponseBody;
import com.bjsxt.pojo.Users;
@Controller
@RequestMapping("/test")
public class postDemo {
//发送 POST 请求不带参数
@RequestMapping(value = "/post",method=RequestMethod.POST)
@ResponseBody
public Object postTest() {
Map<String, String> map=new HashMap<>();
map.put("msg", "Test OK");
return map;
}
//发送 POST 请求带参数
@RequestMapping(value = "/post/param",method=RequestMethod.POST,produces = MediaType.APPLICATION_JSON_VALUE+";charset=utf-8")
@ResponseBody
public Object postParamTest(String name,String pwd) {
System.out.println(name+" --------- "+pwd);
Map<String, String> map=new HashMap<>();
map.put("name",name);
map.put("pwd", pwd);
return map;
}
// POST 请求的参数中传递 JSON 格式数据
@RequestMapping(value = "/post/param/json",method=RequestMethod.POST)
@ResponseBody
public Object postParamTestJson(@RequestBody Users users) {
System.out.println(users.getName()+" ----JSON----- "+users.getPwd());
Map<String, String> map=new HashMap<>();
map.put("name",users.getName());
map.put("pwd", users.getPwd());
return map;
}
}
3.发送 POST 请求不带参数
//post请求不带参数
public static void doPostTest() throws Exception{
// 创建一个httpclient对象
CloseableHttpClient cd = HttpClients.createDefault();
HttpPost post=new HttpPost("http://localhost:8080/test/post");
CloseableHttpResponse res = cd.execute(post);
// 处理响应
// 并获取响应的状态码
int code = res.getStatusLine().getStatusCode();
System.out.println(code);
// 获取响应内容
HttpEntity entity = res.getEntity();
String content = EntityUtils.toString(entity, "utf-8");
System.out.println(content);
//关闭连接
cd.close();
}
4 发送 POST 请求带参数
//发送POST请求带参数
public static void doPostParamTest() throws Exception {
CloseableHttpClient cd = HttpClients.createDefault();
HttpPost post=new HttpPost("http://localhost:8080/test/post/param");
//给定参数
List<BasicNameValuePair> list=new ArrayList<>();
list.add(new BasicNameValuePair("name", "刘德华"));
list.add(new BasicNameValuePair("pwd", "ldh"));
//将参数做字符串的转换
StringEntity se=new UrlEncodedFormEntity(list, "utf-8");
//向请求中绑定参数
post.setEntity(se);
// 处理响应
CloseableHttpResponse res = cd.execute(post);
// 并获取响应的状态码
int code = res.getStatusLine().getStatusCode();
System.out.println(code);
// 获取响应内容
HttpEntity entity = res.getEntity();
String content = EntityUtils.toString(entity, "utf-8");
System.out.println(content);
//关闭连接
cd.close();
}
5 在 POST 请求的参数中传递 JSON 格式数据
//发送POST请求带JSON格式参数
public static void doPostParamJsonTest() throws Exception{
CloseableHttpClient cd = HttpClients.createDefault();
HttpPost post=new HttpPost("http://localhost:8080/test/post/param/json");
String json="{"name":"娜扎","pwd":"1t2e3"}";
StringEntity se=new StringEntity(json, ContentType.APPLICATION_JSON);
//向请求中绑定参数
post.setEntity(se);
// 处理响应
CloseableHttpResponse res = cd.execute(post);
// 并获取响应的状态码
int code = res.getStatusLine().getStatusCode();
System.out.println(code);
// 获取响应内容
HttpEntity entity = res.getEntity();
String content = EntityUtils.toString(entity, "utf-8");
System.out.println(content);
//关闭连接
cd.close();
}
6 HttpClient 自定义工具类的使用
6.1 编写工具类
package com.sxt.commons;
import java.io.IOException;
import java.net.URI;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import org.apache.http.NameValuePair;
import org.apache.http.client.entity.UrlEncodedFormEntity;
import org.apache.http.client.methods.CloseableHttpResponse;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.client.utils.URIBuilder;
import org.apache.http.entity.ContentType;
import org.apache.http.entity.StringEntity;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.message.BasicNameValuePair;
import org.apache.http.util.EntityUtils;
public class HttpClientUtil {
public static String doGet(String url, Map<String, String> param) {
// 创建Httpclient对象
CloseableHttpClient httpclient = HttpClients.createDefault();
String resultString = "";
CloseableHttpResponse response = null;
try {
// 创建uri
URIBuilder builder = new URIBuilder(url);
if (param != null) {
for (String key : param.keySet()) {
builder.addParameter(key, param.get(key));
}
}
URI uri = builder.build();
// 创建http GET请求
HttpGet httpGet = new HttpGet(uri);
// 执行请求
response = httpclient.execute(httpGet);
// 判断返回状态是否为200
if (response.getStatusLine().getStatusCode() == 200) {
resultString = EntityUtils.toString(response.getEntity(), "UTF-8");
}
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
if (response != null) {
response.close();
}
httpclient.close();
} catch (IOException e) {
e.printStackTrace();
}
}
return resultString;
}
public static String doGet(String url) {
return doGet(url, null);
}
public static String doPost(String url, Map<String, String> param) {
// 创建Httpclient对象
CloseableHttpClient httpClient = HttpClients.createDefault();
CloseableHttpResponse response = null;
String resultString = "";
try {
// 创建Http Post请求
HttpPost httpPost = new HttpPost(url);
// 创建参数列表
if (param != null) {
List<NameValuePair> paramList = new ArrayList<>();
for (String key : param.keySet()) {
paramList.add(new BasicNameValuePair(key, param.get(key)));
}
// 模拟表单
UrlEncodedFormEntity entity = new UrlEncodedFormEntity(paramList,"utf-8");
httpPost.setEntity(entity);
}
// 执行http请求
response = httpClient.execute(httpPost);
resultString = EntityUtils.toString(response.getEntity(), "utf-8");
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
response.close();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
return resultString;
}
public static String doPost(String url) {
return doPost(url, null);
}
public static String doPostJson(String url, String json) {
// 创建Httpclient对象
CloseableHttpClient httpClient = HttpClients.createDefault();
CloseableHttpResponse response = null;
String resultString = "";
try {
// 创建Http Post请求
HttpPost httpPost = new HttpPost(url);
// 创建请求内容
StringEntity entity = new StringEntity(json, ContentType.APPLICATION_JSON);
httpPost.setEntity(entity);
// 执行http请求
response = httpClient.execute(httpPost);
resultString = EntityUtils.toString(response.getEntity(), "utf-8");
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
response.close();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
return resultString;
}
}
6.2 测试工具类
//测试HttpClient工具类
public static void HttpClientUtilTest() {
String url="http://localhost:8080/test/post/param";
Map<String, String> param=new HashMap<>();
param.put("name", "达英");
param.put("pwd", "fjgui");
String result = HttpClientUtil.doPost(url, param);
System.out.println(result);
}