【注】本文译自: https://www.tutorialspoint.com/spring_boot/spring_boot_file_handling.htm
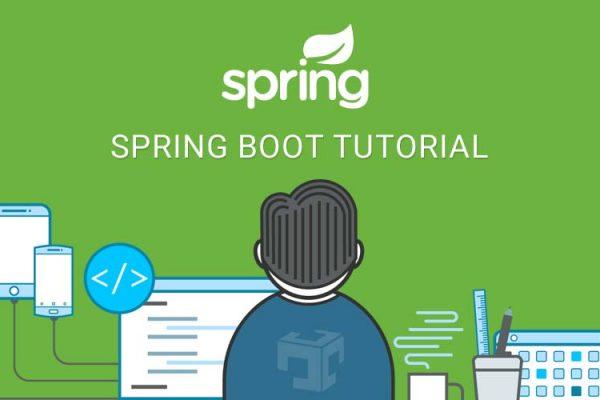
本文学习如何使用 web 服务进行文件上传和下载。
文件上传
上传一个文件,可以使用 MultipartFile 作为请求参数,并且这个 API 应当消费 Multi-Part 表单数据值。示例代码如下:
@RequestMapping(value = "/upload", method = RequestMethod.POST, consumes = MediaType.MULTIPART_FORM_DATA_VALUE)public String fileUpload(@RequestParam("file") MultipartFile file) { return null;}
完整代码如下:
package com.tutorialspoint.demo.controller;import java.io.File;import java.io.FileOutputStream;import java.io.IOException;import org.springframework.http.MediaType;import org.springframework.web.bind.annotation.RequestMapping;import org.springframework.web.bind.annotation.RequestMethod;import org.springframework.web.bind.annotation.RequestParam;import org.springframework.web.bind.annotation.RestController;import org.springframework.web.multipart.MultipartFile;@RestControllerpublic class FileUploadController { @RequestMapping(value = "/upload", method = RequestMethod.POST, consumes = MediaType.MULTIPART_FORM_DATA_VALUE) public String fileUpload(@RequestParam("file") MultipartFile file) throws IOException { File convertFile = new File("/var/tmp/"+file.getOriginalFilename()); convertFile.createNewFile(); FileOutputStream fout = new FileOutputStream(convertFile); fout.write(file.getBytes()); fout.close(); return "File is upload successfully"; }}
文件下载
文件下载应当使用 InputStreamResource。我们要在响应中设置 HttpHeader Content-Disposition,并且要指定应用的响应媒体类型(Media Type)。
注意: 以下面的例子中,在应用运行时指定路径上的文件应当是可用的。
@RequestMapping(value = "/download", method = RequestMethod.GET) public ResponseEntity downloadFile() throws IOException { String filename = "/var/tmp/mysql.png"; File file = new File(filename); InputStreamResource resource = new InputStreamResource(new FileInputStream(file)); HttpHeaders headers = new HttpHeaders(); headers.add("Content-Disposition", String.format("attachment; filename="%s"", file.getName())); headers.add("Cache-Control", "no-cache, no-store, must-revalidate"); headers.add("Pragma", "no-cache"); headers.add("Expires", "0"); ResponseEntity responseEntity = ResponseEntity.ok().headers(headers).contentLength(file.length()).contentType( MediaType.parseMediaType("application/txt")).body(resource); return responseEntity;}
完整代码如下:
package com.tutorialspoint.demo.controller;import java.io.File;import java.io.FileInputStream;import java.io.IOException;import org.springframework.core.io.InputStreamResource;import org.springframework.http.HttpHeaders;import org.springframework.http.MediaType;import org.springframework.http.ResponseEntity;import org.springframework.web.bind.annotation.RequestMapping;import org.springframework.web.bind.annotation.RequestMethod;import org.springframework.web.bind.annotation.RestController;@RestControllerpublic class FileDownloadController { @RequestMapping(value = "/download", method = RequestMethod.GET) public ResponseEntity downloadFile() throws IOException { String filename = "/var/tmp/mysql.png"; File file = new File(filename); InputStreamResource resource = new InputStreamResource(new FileInputStream(file)); HttpHeaders headers = new HttpHeaders(); headers.add("Content-Disposition", String.format("attachment; filename="%s"", file.getName())); headers.add("Cache-Control", "no-cache, no-store, must-revalidate"); headers.add("Pragma", "no-cache"); headers.add("Expires", "0"); ResponseEntity responseEntity = ResponseEntity.ok().headers(headers).contentLength( file.length()).contentType(MediaType.parseMediaType("application/txt")).body(resource); return responseEntity; }}
主 Spring Boot 应用类如下:
package com.tutorialspoint.demo;import org.springframework.boot.SpringApplication;import org.springframework.boot.autoconfigure.SpringBootApplication;@SpringBootApplicationpublic class DemoApplication { public static void main(String[] args) { SpringApplication.run(DemoApplication.class, args); }} Maven build – pom.xml 代码如下:<?xml version = "1.0" encoding = "UTF-8"?> 4.0.0 com.tutorialspoint demo 0.0.1-SNAPSHOT jar demo Demo project for Spring Boot org.springframework.boot spring-boot-starter-parent 1.5.8.RELEASE UTF-8 UTF-8 1.8 org.springframework.boot spring-boot-starter-web org.springframework.boot spring-boot-starter-test test org.springframework.boot spring-boot-maven-plugin
Gradle Build – build.gradle 代码如下:
buildscript { ext { springBootVersion = '1.5.8.RELEASE' } repositories { mavenCentral() } dependencies { classpath("org.springframework.boot:spring-boot-gradle-plugin:${springBootVersion}") }}apply plugin: 'java'apply plugin: 'eclipse'apply plugin: 'org.springframework.boot'group = 'com.tutorialspoint'version = '0.0.1-SNAPSHOT'sourceCompatibility = 1.8repositories { mavenCentral()}dependencies { compile('org.springframework.boot:spring-boot-starter-web') testCompile('org.springframework.boot:spring-boot-starter-test')}
现在你可以使用 Maven 或 Gradle 命令创建可执行 executable JAR 文件并运行 Spring Boot 应用了:
Maven 命令如下:
mvn clean install
在 “BUILD SUCCESS” 之后,你可以在 target 目录下找到 JAR 文件。
Gradle 可以使用以下命令:
gradle clean build
在 “BUILD SUCCESSFUL” 之后,你可以在 build/libs 目录下找到 JAR 文件。
现在,使用以下命令运行 JAR 文件:
java –jar
应用将在 Tomcat 8080 端口启动,如下 所示:

现在在 POSTMAN 应用中输入以下 URL’s in POSTMAN,可以看到下图所示的输出:
文件上传: http://localhost:8080/upload
文件下载: http://localhost:8080/download
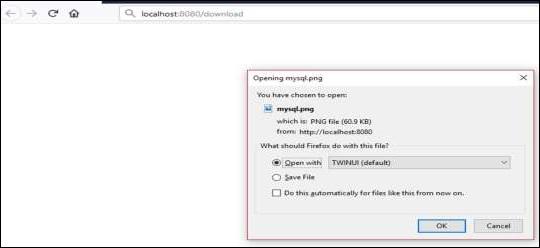