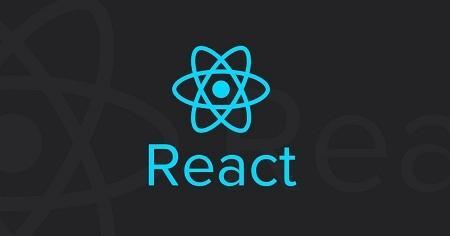
大家在用react开发项目时,常常会对react的两种规范的语法有所困惑。今天为大家整理一下主要的几个不同点。
系统库的引用
ES5中的引用需要先使用require导入React包,成为对象,再去进行真正引用
//ES5var React = require("react");var { Component, PropTypes} = React; //引用React抽象组件var ReactNative = require("react-native");var { Image, Text,} = ReactNative; //引用具体的React Native组件
在ES6里,可以使用import方法来直接实现系统库引用,不需要额外制作一个类库对象
//ES6import React, { Component, PropTypes,} from 'react';import { Image, Text} from 'react-native'
导出及引用单个类
ES5中,要导出一个类给别的模块用,一般通过module.exports来实现。引用时,则依然通过require方法来获取。
//ES5导出var MyComponent = React.createClass({ ...});module.exports = MyComponent;//ES5导入var MyComponent = require('./MyComponent');
ES6中,则可以使用用export default来实现相同的功能,使用import方法来实现导入。
注意:ES5和ES6的导入导出方法是成对出现的,不可以混用。
比如:使用export default来导出,只能通过import 来导入。若使用require来导入,编译将不能通过。
//ES6导出(一般都继承于Component类)export default class MyComponent extends Component{ ...}//ES6导入import MyComponent from './MyComponent';
定义组件
ES5中,组件类的定义通过React.createClass实现。
注意:ES5中React.createClass后面是需要小括号的,且结尾必须有分号。
//ES5var Photo = React.createClass({ render: function() { return ( ); },});
在ES6里,让组件类去继承React.Component类就可以了。
注意:这里结尾时不会出现小括号,也不需要添加分号。
//ES6class Photo extends React.Component { render() { return ( ); }}
组件内部定义方法
ES5中采用的是 ###:function()的形式,方法大括号末尾需要添加逗号。
//ES5 var Photo = React.createClass({ componentWillMount: function(){ }, render: function() { return ( ); },});
ES6中省略了【: function】这一段,并且结尾不需要加逗号来实现分隔。
注意:使用ES6定义的规则的话,外层必须用【class #### extend React.Component】的方式来申明这个类,否则会报错。
//ES6class Photo extends React.Component { componentWillMount() { } render() { return ( ); }}
定义组件的属性类型和默认属性
在ES5里,属性类型和默认属性分别通过propTypes成员和getDefaultProps方法来实现(这两个方法应该是固定名称的)
//ES5 var Video = React.createClass({ getDefaultProps: function() { return { autoPlay: false, maxLoops: 10, }; }, propTypes: { autoPlay: React.PropTypes.bool.isRequired, maxLoops: React.PropTypes.number.isRequired, posterFrameSrc: React.PropTypes.string.isRequired, videoSrc: React.PropTypes.string.isRequired, }, render: function() { return ( ); },});
在ES6里,统一使用static成员来实现
//ES6class Video extends React.Component { static defaultProps = { autoPlay: false, maxLoops: 10, }; // 注意这里有分号 static propTypes = { autoPlay: React.PropTypes.bool.isRequired, maxLoops: React.PropTypes.number.isRequired, posterFrameSrc: React.PropTypes.string.isRequired, videoSrc: React.PropTypes.string.isRequired, }; // 注意这里有分号 render() { return ( ); } // 注意这里既没有分号也没有逗号}
ES6中也可以在组件类声明完成后追加其静态方法。虽不推荐,但写法上也没问题
//ES6class Video extends React.Component { render() { return ( ); }}Video.defaultProps = { autoPlay: false, maxLoops: 10,};Video.propTypes = { autoPlay: React.PropTypes.bool.isRequired, maxLoops: React.PropTypes.number.isRequired, posterFrameSrc: React.PropTypes.string.isRequired, videoSrc: React.PropTypes.string.isRequired,};
初始化STATE
在ES5中,初始化state的方法是固定的getInitialState
//ES5 var Video = React.createClass({ getInitialState: function() { return { loopsRemaining: this.props.maxLoops, }; },})
ES6中存在两种写法:
第一种,直接构造state函数
class Video extends React.Component { state = { loopsRemaining: this.props.maxLoops, }}
第二种,更易理解的在构造函数中初始化(这样你还可以根据需要做一些计算)
class Video extends React.Component { constructor(props){ super(props); this.state = { loopsRemaining: this.props.maxLoops, }; }}
把方法作为回调提供
很多习惯于ES6的用户反而不理解在ES5下可以这么做:
//ES5var PostInfo = React.createClass({ handleOptionsButtonClick: function(e) { // Here, 'this' refers to the component instance. this.setState({showOptionsModal: true}); }, render: function(){ return ( {this.props.label} ) },});
在ES5下,React.createClass会把所有的方法都bind一遍,这样可以提交到任意的地方作为回调函数,而this不会变化。但官方现在逐步认为这反而是不标准、不易理解的。
在ES6下,你需要通过bind来绑定this引用,或者使用箭头函数(它会绑定当前scope的this引用)来调用
//ES6class PostInfo extends React.Component{ handleOptionsButtonClick(e){ this.setState({showOptionsModal: true}); } render(){ return ( this.handleOptionsButtonClick(e)} > {this.props.label} ) },}
Mixins
在ES5下,我们经常使用mixin来为我们的类添加一些新的方法,譬如PureRenderMixin
var PureRenderMixin = require('react-addons-pure-render-mixin');React.createClass({ mixins: [PureRenderMixin], render: function() { return
foo
; }});
然而现在官方已经不再打算在ES6里继续推行Mixin,他们说:Mixins Are Dead. Long Live Composition。
尽管如果要继续使用mixin,还是有一些第三方的方案可以用,譬如这个方案(https://github.com/brigand/react-mixin)。
不过官方推荐,对于库编写者而言,应当尽快放弃Mixin的编写方式,上文中提到Sebastian Markbåge的一段代码推荐了一种新的编码方式:
//Enhance.jsimport { Component } from "React";export var Enhance = ComposedComponent => class extends Component { constructor() { this.state = { data: null }; } componentDidMount() { this.setState({ data: 'Hello' }); } render() { return ; }};
//HigherOrderComponent.jsimport { Enhance } from "./Enhance";class MyComponent { render() { if (!this.data) return
Waiting...
; return
{this.data}
; }}export default Enhance(MyComponent); // Enhanced component
ES6+带来的其它好处
解构&属性延展
结合使用ES6+的解构和属性延展,我们给孩子传递一批属性更为方便了。这个例子把className以外的所有属性传递给div标签:
class AutoloadingPostsGrid extends React.Component { render() { var { className, ...others, // contains all properties of this.props except for className } = this.props; return (
Load more
); }}
下面这种写法,则是传递所有属性的同时,用覆盖新的className值:
…
这个例子则相反,如果属性中没有包含className,则提供默认的值,而如果属性中已经包含了,则使用属性中的值
…
本文部分内容来源于CSDN博主「chanzhu」的原创文章。更多内容请点击了解更多。