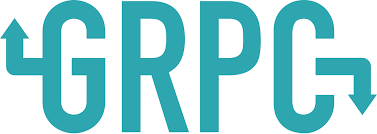
gRPC is a modern open source high performance RPC framework that can run in any environment. It can efficiently connect services in and across data centers with pluggable support for load balancing, tracing, health checking and authentication. It is also applicable in last mile of distributed computing to connect devices, mobile applications and browsers to backend services.
1. gRPC 是什么
官方文档:链接,中文文档:链接,GitHub 网址:链接。
想了解 gRPC 是什么,就得先了解什么是 RPC。RPC 全称为 Remote Procedure Call,即远程调用框架(从字面意思上看就是“远程调用服务端所提供的方法/函数”),该协议允许运行于一台计算机的程序调用另一台计算机的子程序,而程序员无需额外地为这个交互作用编程。
简单来说就是,通过利用 RPC,我们可以轻松调用服务端所提供的函数,而无需了解其内部定义。(关于RPC、REST API、Socket 的区别,可以查看这篇博文:RPC是什么?RPC与REST、Socket的区别?php中流行的rpc框架有哪些?)
那么 gRPC 是什么呢?gRPC是一个高性能、通用的开源RPC框架,其由 Google 主要面向移动应用开发并基于HTTP/2协议标准而设计,基于 ProtoBuf(Protocol Buffers) 序列化协议开发,且支持众多开发语言。 其最基本的开发步骤是定义 proto 文件, 定义请求 Request 和 响应 Response 的格式,然后定义一个服务 Service, Service 可以包含多个方法。
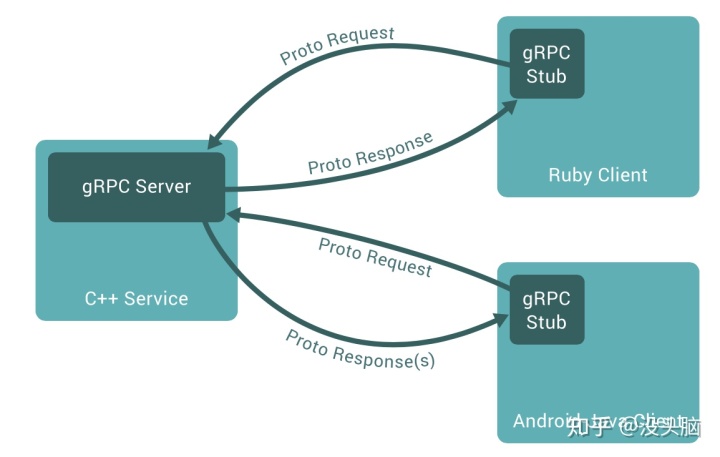
此外,gRPC 支持流式开发,即客户端流式发送、服务器流式返回以及客户端/服务器同时流式处理, 也就是单向流和双向流。(关于流式开发,可以阅读这篇博文:gRPC的那些事 - streaming)
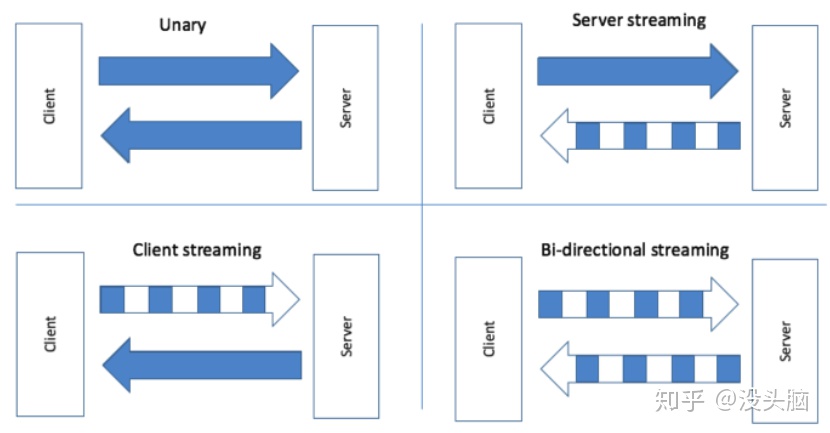
2. 安装 gRPC
# gRPC 的安装:
$ pip install grpcio
3. gRPC 简单例子
- 编写 proto 文件并进行编译:
syntax = "proto3";
message EmotionClsRequest {
bytes image = 1;
message Shape {
int32 height = 1;
int32 width = 2;
int32 channel = 3;
}
Shape shape = 2;
}
message EmotionClsResponse {
message Box {
int32 left = 1;
int32 top = 2;
int32 right = 3;
int32 bottom = 4;
}
message Emotion {
float Surprised = 1;
float Fear = 2;
float Disgust = 3;
float Happy = 4;
float Sad = 5;
float Angry = 6;
float Neutral = 7;
}
message Face {
Box box = 1;
float score = 2;
Emotion emotion = 3;
int32 errorCode = 4;
}
repeated Face faces = 1;
}
service EmotionClassifier {
rpc Classification (EmotionClsRequest) returns (EmotionClsResponse) {}
}
可以看到,在这个proto 文件中,我们定义了消息 EmotionClsRequst,消息 EmotionClsResponse,服务 EmotionClassifier 以及其中的 RPC 方法 Classification 的信息格式(相关语法可查看后续的 Protocol Buffers 介绍)。此外,我们在此所定义的服务中 Classification 为简单的 RPC 方法,若想定义流式 RPC 方法,可这样编写:
service EmotionClassifier{
rpc Classification1(EmotionClsRequest) returns (stream EmotionClsResponse){} // 应答流式 RPC , 客户端发送请求到服务器,拿到一个流去读取返回的消息序列。 客户端读取返回的流,直到里面没有任何消息。
rpc Classification2(stream EmotionClsRequest) returns (stream EmotionClsResponse){} // 请求流式 RPC , 客户端写入一个消息序列并将其发送到服务器,同样也是使用流。一旦客户端完成写入消息,它等待服务器完成读取返回它的响应。
rpc Classification3(stream EmotionClsRequest) returns (stream EmotionClsResponse){} // 双向流式 RPC 是双方使用读写流去发送一个消息序列。
}
接着,对 proto 文件进行编译:
python -m grpc_tools.protoc --python_out=. --grpc_python_out=. -I. emotionCls.proto
该命令将在当前目录下生成 emotionCls_pb2.py 和 emotionCls_pb2_grpc.py。其中,--python_out 指定所输出的 *_pb2.py 文件路径,--grpc_python_out 指定所输出的 *_pb2_grpc.py 文件路径,-I 是指定.proto文件所在路径。
- 编写服务端(server)代码
from
- 编写客户端(client)代码
import
4. 关于 Protocol Buffers
Protocol Buffers 是一种轻便高效的结构化数据存储格式(由 Google 提供的一种数据序列化协议),可以用于结构化数据序列化,很适合做数据存储或 RPC 数据交换格式。它可用于通讯协议、数据存储等领域的语言无关、平台无关、可扩展的序列化结构数据格式。
如果想了解 Protocol Buffers 具体语法,建议大家阅读以下博文:
-
- [译]Protobuf 语法指南
- Protocol Buffers 3.0 技术手册
- Protocol Buffers Developer Guide
参考资料:
-
- 谁能用通俗的语言解释一下什么是 RPC 框架?
- RPC 是什么
- gRPC是什么
如果你看到了这篇文章的最后,并且觉得有帮助的话,麻烦你花几秒钟时间点个赞,或者受累在评论中指出我的错误。谢谢!作者信息:知乎:没头脑CSDN:Code_MartGithub:Tao Pu