strict 作用
TypeScript
中的严格模式跟 JavaScript
中说的严格模式(即 use strict
)不是一个概念,它表示是否开启下面一系列的类型检查规则:
"noImplicitAny": true,
"strictNullChecks": true,
"strictFunctionTypes": true,
"strictBindCallApply": true,
"strictPropertyInitialization": true,
"noImplicitThis": true,
"alwaysStrict": true
- 如果你设置
strict
为true
的话,那么上面所有的配置默认全是true
- 如果你设置
strict
为false
的话,那么上面的所有配置默认全是false
- 你如果既设置了
strict
又单独设置了其中的部分配置,那么以单独设置的配置为准
strictNullChecks
默认情况下,null
和 undefined
可以被赋值给其它任意类型,因为 null
和 undefined
是任何类型的子类型。
当 strictNullChecks
配置为 false
时,下面的代码不会提示报错。
let age: number = undefined
age = null
age = 18
age = undefined
export {}
当 strictNullChecks
配置为 true
时,上面的代码会提示报错,
它告诉我们不能将 undefined
赋值给 number
类型。
TS2322: Type 'undefined' is not assignable to type 'number'.
当我们开启 strictNullChecks
时,null
和 undefined
就不能赋值给任何类型了,对于它们的校验相当于开启了严格模式
noImplicitAny
如下代码,我们定义了一个函数 test
,并且它有一个参数 a
,但是我们并没有显式的给 a
定义类型,这时 typescript
将自动推导 a
的类型为 any
,
相当于给 a
隐式的加了 any
类型。
如果 noImplicitAny
配置为 false
,下面这段代码能够正常运行,但当我们给 noImplicitAny 配置为 true 时,下面这段代码将提示 TS7006: Parameter 'a' implicitly has an 'any' type.
export function test (a) {
return a
}
strictFunctionTypes
严格函数类型检查
如下代码
export const x: number | string = Math.random() > 0.5 ? '2020' : 2021;
const y: number = x;
将 number | string
类型的 x
赋值给 number
类型的 y
这是不被允许的
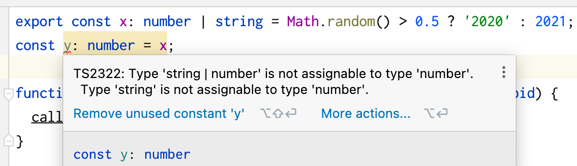
但是在函数中,这种形式却是可以的,回调函数中的 date
参数是 number | string
类型的,但是调用时,date
是 string
类型的,
当 strictFunctionTypes
没有开启时,typescript
认为是没有问题的
export function getCurrentYear(callback: (date: string | number) => void) {
callback(Math.random() > 0.5 ? '2020' : 2020);
}
getCurrentYear((date: string) => {
console.log(date.charAt(0));
});
如果开启 strictFunctionTypes
为 true
,报错如下
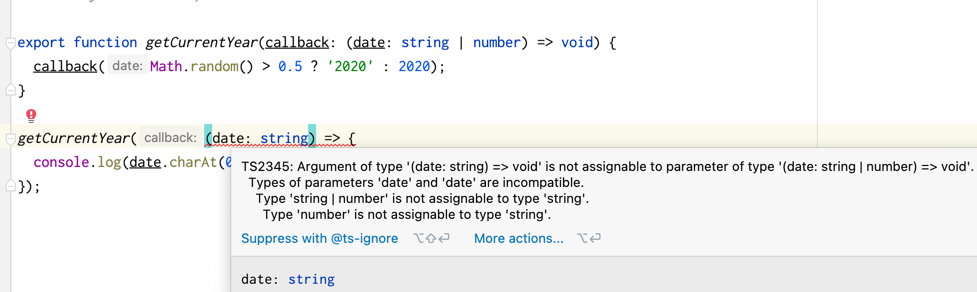
strictBindCallApply 作用
严格绑定检查。意思是在使用 bind
、call
和 apply
语法的时候,是否进行参数检查
示例
如下代码,当 strictBindCallApply
未开启时,typescript
认为下列代码没有错误,但实际上,fnn
的两个参数都是必传的,显然这种提示是不合理的。
export function fnn (name: string, age: number) {
return name + age;
}
fnn.call(null, 'wfly');
fnn.apply(null, ['wfly']);
fnn.bind(null, 'wfly')();
当我们设置 strictBindCallApply
为 true
时,typescript
会对这种参数缺少进行报错,如下图所示
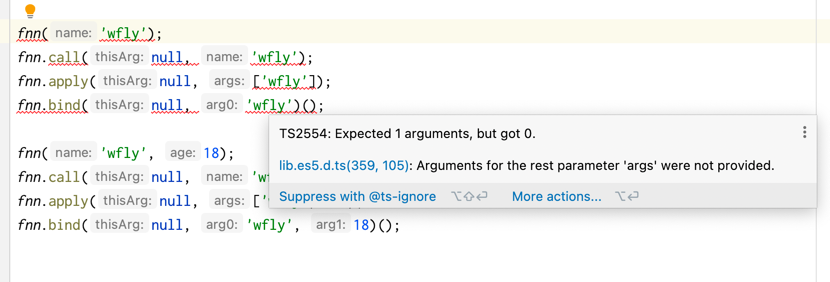
strictPropertyInitialization
该选项用于检查类的属性是否被初始化,如果开启则必须进行初始化,开启此属性需要 strictNullChecks
一并开启
示例
如下代码,当 strictPropertyInitialization
未开启时,此代码不会提示报错
export class Person {
name: string;
}
当 strictPropertyInitialization
开启时,会提示 TS2564: Property 'name' has no initializer and is not definitely assigned in the constructor.
正确写法,给 name
赋一个初始值
export class Person {
name: string = '';
}
noImplicitThis
是否允许出现隐式 any
类型的 this
示例
如下代码,getName
函数返回值是一个函数,其中这个函数的返回值中用到了 this,这个 this 是未知的, 当 noImplicitThis
未开启时,此代码不会提示报错
export class Person {
name = '';
constructor (_name: string) {
this.name = _name;
}
getName () {
console.log(this, this.name);
return function () {
console.log(this.name);
};
}
}
new Person('小明').getName().call({ name: '小红' });
当 noImplicitThis
开启时,会提示如下报错
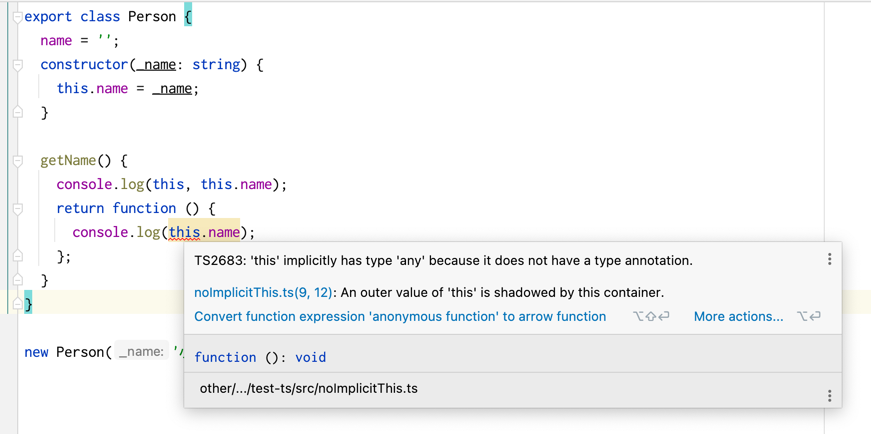
alwaysStrict
是否开启严格模式,开启的话编译后的 js
会带上 use strict
,之前是 js
文件的会带上,ts
编译为 js
没有带上。