效果
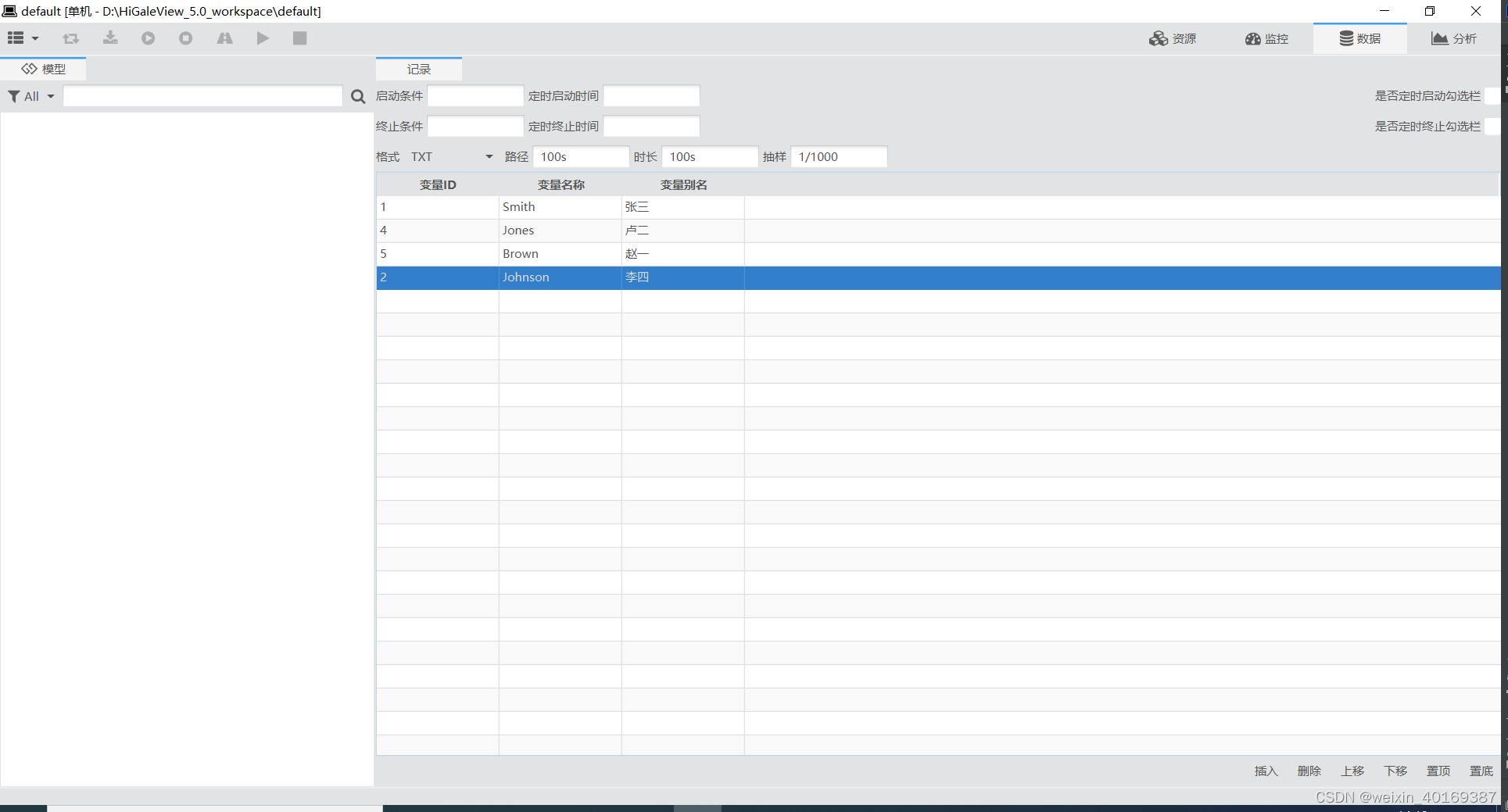
FXML部分
<?xml version="1.0" encoding="UTF-8"?>
<?import javafx.geometry.Insets?>
<?import javafx.scene.control.*?>
<?import javafx.scene.layout.*?>
<?import org.kordamp.ikonli.javafx.FontIcon?>
<SplitPane stylesheets="@WorkAreaContentView.css" dividerPositions="0.25" xmlns="http://javafx.com/javafx" xmlns:fx="http://javafx.com/fxml" fx:controller="com.hirain.higale.plugin.acquisition.application.view.WorkAreaContentViewController">
<items>
<TabPane>
<tabs>
<Tab closable="false" text="模型">
<graphic>
<FontIcon iconLiteral="fa-gg"/>
</graphic>
<content>
<VBox spacing="4.0">
<children>
<HBox>
<children>
<MenuButton mnemonicParsing="false" text="All">
<graphic>
<FontIcon iconLiteral="fa-filter"/>
</graphic>
<items>
<MenuItem mnemonicParsing="false" text="model-0"/>
<MenuItem mnemonicParsing="false" text="model-1"/>
<MenuItem mnemonicParsing="false" text="model-2"/>
<MenuItem mnemonicParsing="false" text="model-3"/>
<MenuItem mnemonicParsing="false" text="model-4"/>
</items>
</MenuButton>
<TextField HBox.hgrow="ALWAYS"/>
<Button mnemonicParsing="false">
<graphic>
<FontIcon iconLiteral="fa-search"/>
</graphic>
</Button>
</children>
</HBox>
<TreeView fx:id="modelTreeView" VBox.vgrow="ALWAYS"/>
</children>
<padding>
<Insets top="4.0"/>
</padding>
</VBox>
</content>
</Tab>
</tabs>
</TabPane>
<SplitPane dividerPositions="0.75" orientation="VERTICAL">
<items>
<TabPane tabClosingPolicy="UNAVAILABLE">
<tabs>
<Tab text="记录">
<content>
<BorderPane>
<top>
<VBox>
<HBox alignment="CENTER_LEFT" spacing="4.0" BorderPane.alignment="CENTER">
<children>
<Label text="启动条件"/>
<TextField fx:id="startConditionText" prefWidth="100" text=""/>
<Label text="定时启动时间"/>
<TextField fx:id="StartTimeText" prefWidth="100" text=""/>
<HBox HBox.hgrow="ALWAYS"/>
<Label text="是否定时启动勾选栏"/>
<CheckBox fx:id="startTimeCheckBox" />
</children>
<padding>
<Insets bottom="4.0" top="4.0"/>
</padding>
</HBox>
<HBox alignment="CENTER_LEFT" spacing="4.0" BorderPane.alignment="CENTER">
<children>
<Label text="终止条件"/>
<TextField fx:id="endConditionText" prefWidth="100" text=""/>
<Label text="定时终止时间"/>
<TextField fx:id="endTimeText" prefWidth="100" text=""/>
<HBox HBox.hgrow="ALWAYS"/>
<Label text="是否定时终止勾选栏"/>
<CheckBox fx:id="endTimeCheckBox"/>
</children>
<padding>
<Insets bottom="4.0" top="4.0"/>
</padding>
</HBox>
<HBox alignment="CENTER_LEFT" spacing="4.0" BorderPane.alignment="CENTER">
<children>
<Label text="格式"/>
<ComboBox fx:id="fileFormatComboBox" prefWidth="100" value="TXT"/>
<Label text="路径"/>
<TextField fx:id="filePathText" prefWidth="100" text="100s"/>
<Label text="时长"/>
<TextField fx:id="fileTimeText" prefWidth="100" text="100s"/>
<Label text="抽样"/>
<TextField fx:id="samplingRatioText" prefWidth="100" text="1/1000"/>
</children>
<padding>
<Insets bottom="4.0" top="4.0"/>
</padding>
</HBox>
</VBox>
</top>
<center>
<TableView fx:id="dataTable">
<columns>
<TableColumn fx:id="variableId" prefWidth="125.0" sortable="false" text="变量ID"/>
<TableColumn fx:id="variableName" prefWidth="125.0" sortable="false" text="变量名称"/>
<TableColumn fx:id="variableAlias" prefWidth="125.0" sortable="false" text="变量别名"/>
</columns>
</TableView>
</center>
<bottom>
<HBox alignment="CENTER_RIGHT" spacing="4.0" BorderPane.alignment="CENTER">
<children>
<Button fx:id="insertButton" text="插入"/>
<Button fx:id="deleteButton" text="删除"/>
<Button fx:id="moveUpButton" text="上移"/>
<Button fx:id="moveDownButton" text="下移"/>
<Button fx:id="moveTopButton" text="置顶"/>
<Button fx:id="moveBottomButton" text="置底"/>
</children>
<padding>
<Insets bottom="4.0" top="4.0"/>
</padding>
</HBox>
</bottom>
</BorderPane>
</content>
</Tab>
</tabs>
</TabPane>
</items>
</SplitPane>
</items>
</SplitPane>
controller层
package com.hirain.higale.plugin.acquisition.application.view;
import com.hirain.higale.common.javafx.View;
import com.hirain.higale.common.javafx.ViewController;
import com.hirain.higale.common.javafx.reactor.JavafxReactor;
import com.hirain.higale.framework.stereotype.StatefulComponent;
import javafx.beans.property.SimpleStringProperty;
import javafx.collections.FXCollections;
import javafx.collections.ObservableList;
import javafx.fxml.FXML;
import javafx.fxml.Initializable;
import javafx.scene.control.*;
import javafx.scene.control.cell.PropertyValueFactory;
import java.net.URL;
import java.util.Collections;
import java.util.List;
import java.util.ResourceBundle;
@StatefulComponent
@ViewController(view = @View(location = "WorkAreaContentView.fxml"))
public class WorkAreaContentViewController implements Initializable {
@FXML
private TreeView<String> modelTreeView;
@FXML
private TextField startConditionText;
@FXML
private TextField StartTimeText;
@FXML
private TextField endConditionText;
@FXML
private TextField endTimeText;
@FXML
private TextField filePathText;
@FXML
private TextField fileTimeText;
@FXML
private TextField samplingRatioText;
@FXML
private CheckBox startTimeCheckBox;
@FXML
private CheckBox endTimeCheckBox;
@FXML
private ComboBox fileFormatComboBox;
@FXML
private TableView<DataRecord> dataTable = new TableView<DataRecord>();
@FXML
private TableColumn variableId = new TableColumn();
@FXML
private TableColumn variableName = new TableColumn();
@FXML
private TableColumn variableAlias = new TableColumn();
@FXML
private Button insertButton;
@FXML
private Button deleteButton;
@FXML
private Button moveUpButton;
@FXML
private Button moveDownButton;
@FXML
private Button moveTopButton;
@FXML
private Button moveBottomButton;
private ObservableList<DataRecord> data;
@Override
public void initialize(URL location, ResourceBundle resources) {
initializeDataRecordTable();
listenDataRecordTable();
}
public void listenDataRecordTable(){
JavafxReactor.actionEventsOf(insertButton).subscribe(event -> {
dataTable.getSelectionModel().getSelectedIndices().forEach( selectIndex ->{
dataTable.getItems().add(selectIndex,new DataRecord("","",""));
dataTable.getSelectionModel().select(selectIndex);
});
});
JavafxReactor.actionEventsOf(deleteButton).subscribe(event -> {
dataTable.getSelectionModel().getSelectedIndices().forEach( selectIndex ->{
dataTable.getItems().remove(dataTable.getItems().get(selectIndex));
});
});
JavafxReactor.actionEventsOf(moveUpButton).subscribe(event -> {
dataTable.getSelectionModel().getSelectedIndices().forEach( selectIndex ->{
int foreIndex = selectIndex.intValue() == 0 ? 0 : selectIndex.intValue() - 1;
Collections.swap(dataTable.getItems(),foreIndex,selectIndex.intValue());
dataTable.getSelectionModel().select(foreIndex);
});
});
JavafxReactor.actionEventsOf(moveDownButton).subscribe(event -> {
dataTable.getSelectionModel().getSelectedIndices().forEach( selectIndex ->{
int afterIndex = selectIndex.intValue() == dataTable.getItems().size() - 1 ? dataTable.getItems().size() - 1 : selectIndex.intValue() + 1;
Collections.swap(dataTable.getItems(),afterIndex,selectIndex.intValue());
dataTable.getSelectionModel().select(afterIndex);
});
});
JavafxReactor.actionEventsOf(moveTopButton).subscribe(event -> {
dataTable.getSelectionModel().getSelectedIndices().forEach( selectIndex ->{
int foreIndex = 0;
Collections.swap(dataTable.getItems(),foreIndex,selectIndex.intValue());
dataTable.getSelectionModel().select(foreIndex);
});
});
JavafxReactor.actionEventsOf(moveBottomButton).subscribe(event -> {
dataTable.getSelectionModel().getSelectedIndices().forEach( selectIndex ->{
int afterIndex = dataTable.getItems().size()-1;
Collections.swap(dataTable.getItems(),afterIndex,selectIndex.intValue());
dataTable.getSelectionModel().select(afterIndex);
});
});
}
public void initializeDataRecordTable(){
variableId.setCellValueFactory(new PropertyValueFactory<DataRecord, Integer>("id"));
variableName.setCellValueFactory(new PropertyValueFactory<DataRecord, String>("name"));
variableAlias.setCellValueFactory(new PropertyValueFactory<DataRecord, String>("alias"));
dataTable.getItems().setAll(getDataRecordInfo());
}
public List<DataRecord> getDataRecordInfo(){
data = FXCollections.observableArrayList(
new DataRecord("1", "Smith", "张三"),
new DataRecord("2", "Johnson", "李四"),
new DataRecord("3", "Williams", "王五"),
new DataRecord("4", "Jones", "卢二"),
new DataRecord("5", "Brown", "赵一")
);
return data;
}
public static class DataRecord {
private final SimpleStringProperty id;
private final SimpleStringProperty name;
private final SimpleStringProperty alias;
private DataRecord(String fName, String lName, String email) {
this.id = new SimpleStringProperty (fName);
this.name = new SimpleStringProperty(lName);
this.alias = new SimpleStringProperty(email);
}
public String getId() {
return id.get();
}
public SimpleStringProperty idProperty() {
return id;
}
public void setId(String id) {
this.id.set(id);
}
public String getName() {
return name.get();
}
public SimpleStringProperty nameProperty() {
return name;
}
public void setName(String name) {
this.name.set(name);
}
public String getAlias() {
return alias.get();
}
public SimpleStringProperty aliasProperty() {
return alias;
}
public void setAlias(String alias) {
this.alias.set(alias);
}
}
}