文章目录
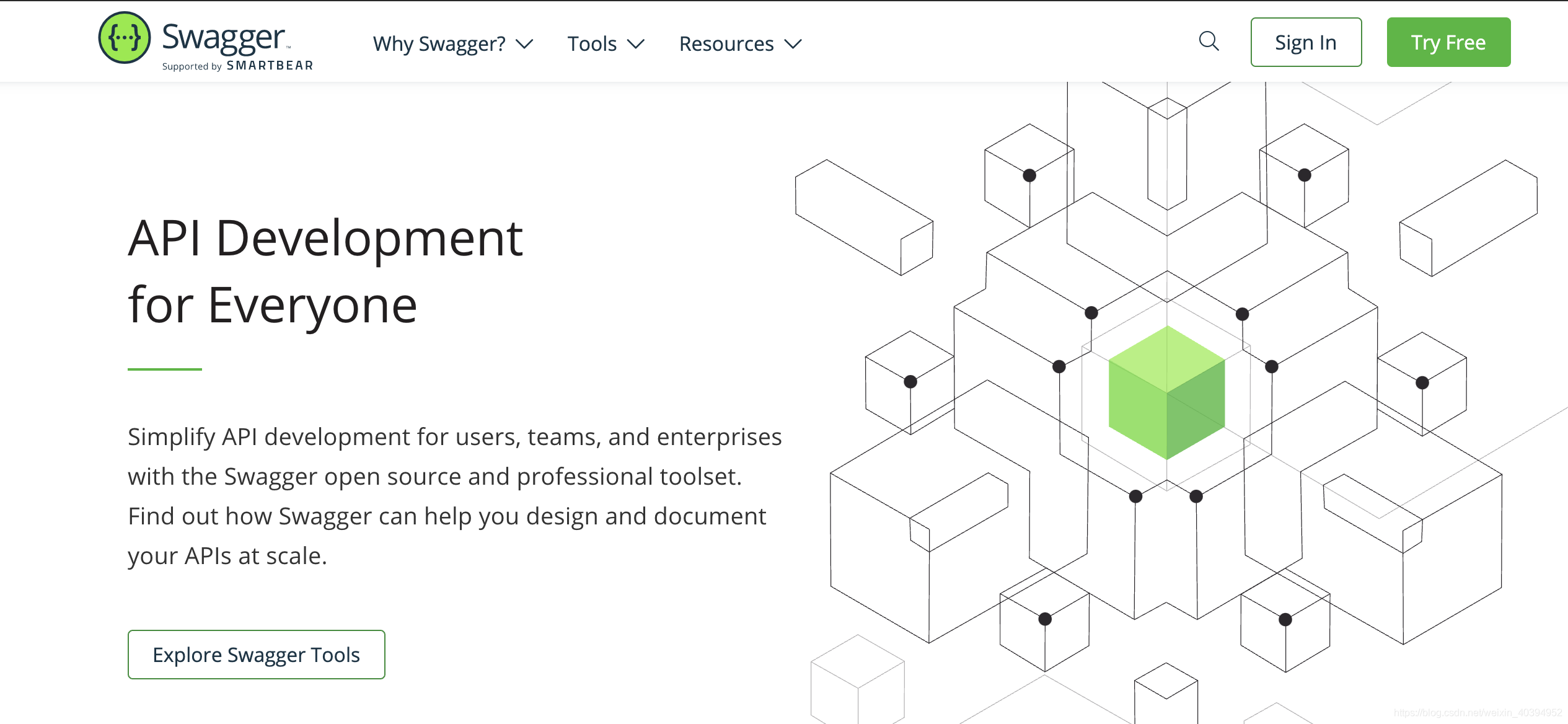
一、前言
Swagger 是一个用于生成、描述和调用 RESTful 接口的 Web 服务。通俗的来讲,Swagger 就是将项目中所有(想要暴露的)接口展现在页面上,并且可以进行接口调用和测试的服务。
Swagger官网:https://swagger.io/
二、Swagger常用注解
2.1 @Api
作用:放在请求的类上,说明类的作用;
示例:
@RestController
@Api(tags = "员工管理模块")
public class EmployeeController {
2.2 @ApiOperation
作用:用在请求的方法上,说明方法的作用;
示例:
@DeleteMapping(value = "deleteById")
@ApiOperation(value = "根据id删除数据", notes = "删除用户")
public String delete(Integer id){
return "删除成功";
}
2.3 @ApiImplicitParams、@ApiImplicitParam
作用:方法参数说明;
示例:
@PostMapping(value = "/save")
//说明是什么方法(可以理解为方法注释)
@ApiOperation(value = "添加用户", notes = "添加用户")
@ApiImplicitParams({
@ApiImplicitParam(name = "user",value = "添加用户数据")
}
)
public String saveUser(Employee user){
return "保存成功";
}
或者:
@GetMapping(value = "findById")
@ApiOperation(value = "根据id获取用户信息", notes = "根据id查询用户信息")
@ApiImplicitParam(name = "id", value = "用户id")
public Employee getUser(Integer id){
return null;
}
2.4 @ApiResponses、@ApiResponse
作用:方法返回值的状态码说明
示例:
@PostMapping(value = "/save")
//说明是什么方法(可以理解为方法注释)
@ApiOperation(value = "添加用户", notes = "添加用户")
@ApiImplicitParams({
@ApiImplicitParam(name = "user",value = "添加用户数据")
}
)
@ApiResponses({
@ApiResponse(code = 200, message = "添加用户成功"),
@ApiResponse(code = 400, message = "请求参数没填好"),
@ApiResponse(code = 404, message = "请求路径没有或页面跳转路径不对")
})
public String saveUser(Employee user){
return "保存成功";
}
2.5 @ApiModel
作用:用于JavaBean上面,用于描述JavaBean 的功能;
示例:
@ApiModel(description = "员工信息")
public class Employee {
2.6 @ApiModelProperty
作用:用在JavaBean类的属性上面,说明属性的含义;
示例:
@ApiModelProperty(value = "员工id",required=true)
private String id;
@ApiModelProperty(value = "员工姓名",required=true)
private String name;
三、SpringBoot整合Swagger
3.1 引入依赖
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger-ui</artifactId>
<version>2.9.2</version>
</dependency>
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger2</artifactId>
<version>2.9.2</version>
</dependency>
3.2 配置类
@Configuration
@EnableSwagger2
public class SwaggerConfig {
@Bean
public Docket createRestApi() {
return new Docket(DocumentationType.SWAGGER_2)
.apiInfo(apiInfo())
//是否开启 (true 开启 false隐藏。生产环境建议隐藏)
//.enable(false)
.select()
//扫描的路径包,设置basePackage会将包下的所有被@Api标记类的所有方法作为api
.apis(RequestHandlerSelectors.basePackage("com.panda"))
//指定路径处理PathSelectors.any()代表所有的路径
.paths(PathSelectors.any())
.build();
}
private ApiInfo apiInfo() {
return new ApiInfoBuilder()
//设置文档标题(API名称)
.title("SpringBoot集成Swagger2学习")
//文档描述
.description("接口说明")
//服务条款URL
.termsOfServiceUrl("http://localhost:8080/")
//版本号
.version("1.0.0")
.build();
}
}
3.3 使用注解标注接口信息
@RestController
@Api(tags = "员工管理模块")
public class EmployeeController {
@PostMapping(value = "/save")
//说明是什么方法(可以理解为方法注释)
@ApiOperation(value = "添加用户", notes = "添加用户")
@ApiImplicitParams({
@ApiImplicitParam(name = "user",value = "添加用户数据")
}
)
@ApiResponses({
@ApiResponse(code = 200, message = "添加用户成功"),
@ApiResponse(code = 400, message = "请求参数没填好"),
@ApiResponse(code = 404, message = "请求路径没有或页面跳转路径不对")
})
public String saveUser(Employee user){
return "保存成功";
}
@GetMapping(value = "findById")
@ApiOperation(value = "根据id获取用户信息", notes = "根据id查询用户信息")
@ApiImplicitParam(name = "id", value = "用户id")
public Employee getUser(Integer id){
return null;
}
@DeleteMapping(value = "deleteById")
@ApiOperation(value = "根据id删除数据", notes = "删除用户")
public String delete(Integer id){
return "删除成功";
}
}
@ApiModel(description = "员工信息")
public class Employee {
@ApiModelProperty(value = "员工id",required=true)
private String id;
@ApiModelProperty(value = "员工姓名",required=true)
private String name;
3.4 查看接口文档
启动项目,访问http://localhost:8080/swagger-ui.html#/
查看接口文档。