#include <stdio.h>
#include <stdlib.h>
#include <malloc.h>
#include <time.h>
#define ERROR 0
#define OK 1
#define Overflow 2
#define Underflow 3
#define NotPresent 4
#define Duplicate 5
typedef int ElemType;
typedef int Status;
//带表头结点单链表的定义
typedef struct node
{
ElemType element;
struct node *link;
}node;
typedef struct
{
struct node *head;
int n;
}headerList;
//带表头结点单链表的初始化
Status Init(headerList *h)
{
h->head = (node*)malloc(sizeof(node));
if(!h->head)
return ERROR;
h->head->link = NULL;
h->n = 0;
return OK;
}
//带表头结点单链表的插入
Status Insert(headerList *L,int i,ElemType x) //在i后面插入x
{
if(i<-1||i>L->n-1)
return ERROR;
node *p = L->head;
int j;
for(j=0;j<=i;j++)
p = p->link;
node *q
带表头结点单链表的初始化、查找、插入、删除、输出、撤销
最新推荐文章于 2023-01-19 18:55:07 发布
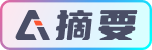