单例模式
饿汉式
package com.design.single;
public class Hungry {
private byte[] data1 = new byte[1024 * 1024];
private byte[] data2 = new byte[1024 * 1024];
private byte[] data3 = new byte[1024 * 1024];
private byte[] data4 = new byte[1024 * 1024];
private Hungry(){
}
private final static Hungry hungry = new Hungry();
public static Hungry getInstance() {
return hungry;
}
}
懒汉式
package com.design.single;
public class Lazy {
private Lazy(){
System.out.println(Thread.currentThread().getName() + "ok");
}
private volatile static Lazy lazy;
public static Lazy getInstance() {
if (lazy == null) {
synchronized (Lazy.class) {
if (lazy == null) {
lazy = new Lazy();
}
}
}
return lazy;
}
public static void main(String[] args) {
for (int i = 0; i < 10; i++) {
new Thread(()->{
Lazy.getInstance();
}).start();
}
}
}
单例不安全,因为反射会破坏单例模式
所以用枚举
将class文件反编译成java文件
jad -sjava fileName.class
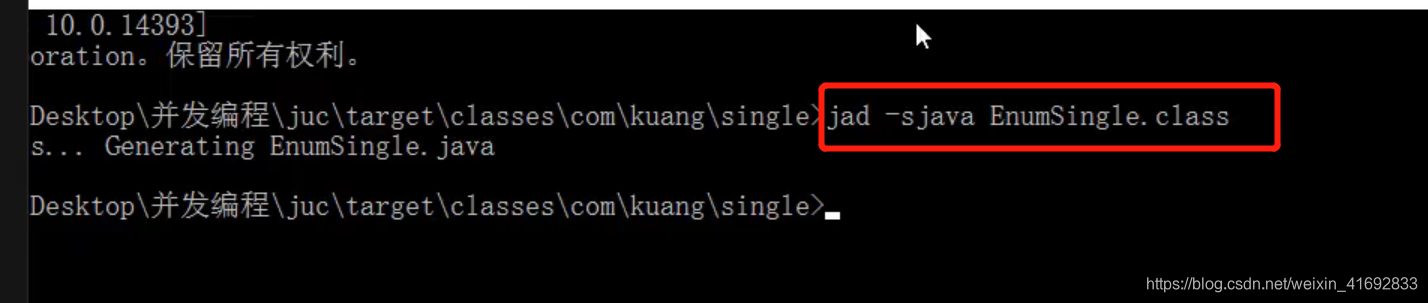