注解配置
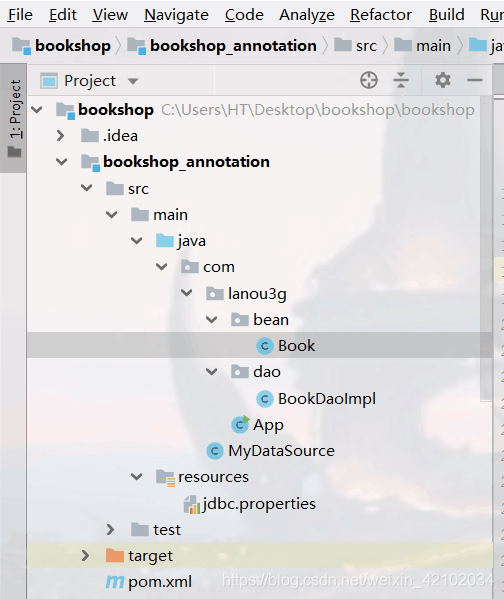
package com.lanou3g.dao;
import com.lanou3g.bean.Book;
import org.apache.commons.dbutils.QueryRunner;
import org.apache.commons.dbutils.ResultSetHandler;
import org.apache.commons.dbutils.handlers.BeanListHandler;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Qualifier;
import org.springframework.stereotype.Component;
import javax.sql.DataSource;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.List;
@Component // 用这个注解标注的类会自动被ioc容器管理,成为一个bean
public class BookDaoImpl {
@Autowired // 按照类型来自动注入属性
@Qualifier("dataSource") //强制按照名称自动注入属性值
private DataSource dataSource;
public List<Book> queryAllBook() {
QueryRunner runner = new QueryRunner(dataSource);
String sql = "select * from book;";
ResultSetHandler<List<Book>> rsh = new BeanListHandler(Book.class);
List<Book> bookList = new ArrayList<>();
try {
bookList = runner.query(sql, rsh);
} catch (SQLException e) {
e.printStackTrace();
}
return bookList;
}
}
package com;
import com.mchange.v2.c3p0.ComboPooledDataSource;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.context.annotation.PropertySource;
import javax.sql.DataSource;
import java.beans.PropertyVetoException;
@Configuration //相当于用注解配置了一个xml配置文件(相当于xml中的<beans>)
//引入了一个外部的properties文件(相当于xml中的
//<context:property-placeholder location="classpath:jdbc.properties" />)
@PropertySource("classpath:jdbc.properties")
public class MyDataSource {
//@Value用于取引入进来的properties文件中key对应的值
@Value("${jdbc.url}")
private String url;
@Value("${jdbc.driver}")
private String driver;
@Value("${jdbc.user}")
private String user;
@Value("${jdbc.password}")
private String password;
@Value("${c3p0.maxPoolSize}")
private int maxPoolSize;
@Value("${c3p0.minPoolSize}")
private int minPoolSize;
@Value("${c3p0.maxIdleTime}")
private int maxIdleTime;
@Bean //配置了一个bean(相当于在xml中写了一个<bean>)
public DataSource dataSource() {
ComboPooledDataSource dataSource = new ComboPooledDataSource();
dataSource.setJdbcUrl(url);
try {
dataSource.setDriverClass(driver);
} catch (PropertyVetoException e) {
}
dataSource.setUser(user);
dataSource.setPassword(password);
dataSource.setMaxPoolSize(maxPoolSize);
dataSource.setMinPoolSize(minPoolSize);
dataSource.setMaxIdleTime(maxIdleTime);
return dataSource;
}
}
package com.lanou3g;
import com.MyDataSource;
import com.lanou3g.dao.BookDaoImpl;
import org.springframework.context.annotation.AnnotationConfigApplicationContext;
import org.springframework.context.annotation.ComponentScan;
import org.springframework.context.annotation.Configuration;
import org.springframework.context.annotation.Import;
import javax.sql.DataSource;
/**
* 程序入口
* 该工程演示了用纯注解的配置方式使用Spring
*/
@Configuration //相当于用注解配置了一个xml配置文件(相当于xml中的<beans>)
// 如果说其他的注解配置bean不在扫描包路径下,就需要通过@Import注解显式导入
// 如果咱们把MyDataSource类放到扫描包路径下(比如com.lanou3g下)就不需要@Import导入了
@Import(MyDataSource.class)
//这里面之所以要导入,是因为MyDataSource.class不再com.lanou3g下面,扫描不到
@ComponentScan(basePackages = "com.lanou3g")
public class App {
public static void main(String[] args) {
AnnotationConfigApplicationContext ctx = new AnnotationConfigApplicationContext(App.class);
ctx.registerShutdownHook();
BookDaoImpl bookDao = ctx.getBean(BookDaoImpl.class);
System.out.println(bookDao.queryAllBook());
}
}
/**
* 整个App类就相当于xml中的
* <beans>
* <import reources="xxxx.xml" />
* <context:component-scan base-package="com.lanou3g" />
* </beans>
*
*/
xml配置
- 在resource中建application.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context.xsd
">
<!-- 引入外部的properties -->
<context:property-placeholder location="classpath:jdbc.properties" />
<!-- 引用外部的properties中的属性 -->
<!-- 配置数据源 -->
<bean id="dataSource" class="com.mchange.v2.c3p0.ComboPooledDataSource">
<property name="jdbcUrl" value="${jdbc.url}" />
<property name="driverClass" value="${jdbc.driver}" />
<property name="user" value="${jdbc.user}" />
<property name="password" value="${jdbc.password}" />
<property name="maxPoolSize" value="${c3p0.maxPoolSize}" />
<property name="minPoolSize" value="${c3p0.minPoolSize}" />
<property name="maxIdleTime" value="${c3p0.maxIdleTime}" />
</bean>
<!-- 配置dao,注入数据源 -->
<bean id="bookDao" class="com.lanou3g.dao.BookDaoImpl">
<property name="dataSource" ref="dataSource" />
</bean>
<!-- 配置dao,注入数据源 -->
<bean id="bookTypeDao" class="com.lanou3g.dao.BookTypeDaoImpl">
<property name="dataSource" ref="dataSource" />
</bean>
</beans>
package com.lanou3g;
import com.lanou3g.bean.Book;
import com.lanou3g.dao.BookDaoImpl;
import org.springframework.context.support.ClassPathXmlApplicationContext;
import java.util.List;
/**
* 程序入口
*
* 该工程演示了用纯xml的配置方式使用Spring
*
*/
public class App {
public static void main(String[] args) {
ClassPathXmlApplicationContext ctx = new ClassPathXmlApplicationContext("applicationContext.xml");
BookDaoImpl bookDao = ctx.getBean(BookDaoImpl.class);
List<Book> bookList = bookDao.queryAllBook();
System.out.println(bookList);
// 优雅的关闭IOC容器
ctx.registerShutdownHook();
}
}