

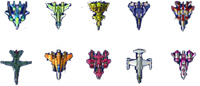


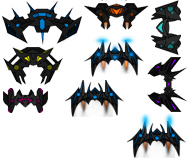
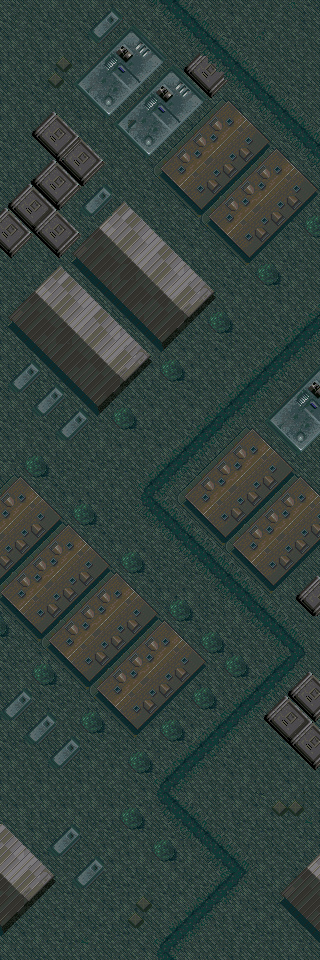
#ifndef Xcoco2d_GameBeigin_h
#define Xcoco2d_GameBeigin_h
#include "cocos2d.h"
class GameBeigin : public cocos2d::CCLayer {
public :
virtual bool init();
static cocos2d::CCScene* scene();
void menuCloseCallback(CCObject* pSender);
void menuOption(CCObject* pSender);
void menuExitCallback(CCObject* pSender);
void musicstop();
void musicplay();
void back();
CREATE_FUNC(GameBeigin);
private:
cocos2d::CCSprite *music;
};
#endif
#include "SimpleAudioEngine.h"
#include "GameBeigin.h"
#include "GameScene.h"
using namespace cocos2d;
//using namespace CocosDenshion;
CCScene* GameBeigin::scene()
{
CCScene *scene = CCScene::create();
GameBeigin *layer = GameBeigin::create();
scene->addChild(layer);
return scene;
}
bool GameBeigin::init()
{
if (!CCLayer::init()) {
return false;
}
CCSize winSize = CCDirector::sharedDirector()->getWinSize();
//添加背景;
CCSprite *spriteBackgound = new CCSprite();
spriteBackgound->initWithFile("background.png");
spriteBackgound->setPosition(ccp(160, 240));
this->addChild(spriteBackgound);
spriteBackgound->release();
//添加背景粒子效果;
CCParticleSystemQuad *system= new CCParticleSystemQuad();
system->initWithFile("space.plist");
system->setPosition(ccp(160.0, 240.0));
system->setTag(77);
this->addChild(system);
system->release();
//添加标题;
CCSprite *headLine = new CCSprite();
headLine->initWithFile("headLine.png", CCRectMake(0, 0, 300.0, 88.0));
headLine->setPosition(ccp(winSize.width / 2, winSize.height / 2 + 100));
this->addChild(headLine);
headLine->release();
//添加菜单;
CCMenuItemImage *newGame = new CCMenuItemImage();
newGame->initWithNormalImage("newGame.png",
"newGame.png",
"newGame.png",
this, menu_selector(GameBeigin::menuCloseCallback));
newGame->setPosition(ccp(0, 0));
CCMenuItemImage *option = new CCMenuItemImage();
option->initWithNormalImage("option.png",
"option.png",
"option.png",
this, menu_selector(GameBeigin::menuOption));
option->setPosition(ccp(0, -50));
CCMenuItemImage *exitGame = new CCMenuItemImage();
exitGame->initWithNormalImage("exitGame.png",
"exitGame.png",
"exitGame.png",
this, menu_selector(GameBeigin::menuExitCallback));
exitGame->setPosition(ccp(0, -100));
CCMenu *menu = CCMenu::create(newGame, option, exitGame, NULL);
menu->setPosition(ccp(winSize.width / 2, winSize.height / 2));
this->addChild(menu, 2);
menu->setTag(4);
newGame->release();
option->release();
exitGame->release();
return true;
}
void GameBeigin::menuCloseCallback(CCObject* pSender)
{
this->removeChildByTag(77);
CCTransitionCrossFade * t = CCTransitionCrossFade::create(1.0, GameScene::scene());
CCDirector::sharedDirector()->replaceScene(t);
CocosDenshion::SimpleAudioEngine::sharedEngine()->resumeAllEffects();
}
void GameBeigin::menuOption(CCObject* pSender)
{
this->removeChildByTag(4);
CCSize size = CCDirector::sharedDirector()->getWinSize();
CCLayerColor * backround = CCLayerColor::create(ccc4(0, 0, 0, 125));
this->addChild(backround,2);
CCLabelTTF * t = CCLabelTTF::create("音乐设置", "Helvetica-BoldOblique", 25);
t->setPosition(ccp(size.width/2, size.height/2+40));
t->setColor(ccc3(255, 255, 0));
this->addChild(t,2);
CCMenuItemFont * musicstop = CCMenuItemFont::create("stop", this, menu_selector(GameBeigin::musicstop)) ;
musicstop->setColor(ccc3(255, 255, 0));
musicstop->setPosition(ccp(0, -50));
CCMenuItemFont * musicplay = CCMenuItemFont::create("play", this, menu_selector(GameBeigin::musicplay)) ;
musicplay->setColor(ccc3(255, 255, 0));
musicplay->setPosition(ccp(0, 0));
CCMenuItemFont * back = CCMenuItemFont::create("exit", this, menu_selector(GameBeigin::back)) ;
back->setColor(ccc3(255, 0, 0));
back->setPosition(ccp(0, -100));
CCMenu * menu = CCMenu::create(musicplay,musicstop,back);
//menu->setPosition(ccp(size.width/2, size.height/2));
this->addChild(menu,2);
CCLOG("%f,%f",menu->getPositionX(),menu->getPositionY());
CCLOG("%f,%f",musicplay->getPositionX(),musicplay->getPositionY());
}
void GameBeigin::musicstop()
{
CocosDenshion::SimpleAudioEngine::sharedEngine()->stopBackgroundMusic();
}
void GameBeigin::musicplay()
{
CocosDenshion::SimpleAudioEngine::sharedEngine()->playBackgroundMusic("SpaceGame.caf");
}
void GameBeigin::back()
{
CCTransitionFlipAngular*y= CCTransitionFlipAngular::create(1.0, GameBeigin::scene());
CCDirector::sharedDirector()->replaceScene(y);
}
void GameBeigin::menuExitCallback(CCObject* pSender)
{
CCDirector::sharedDirector()->end();
#if (CC_TARGET_PLATFORM == CC_PLATFORM_IOS)
exit(0);
#endif
}
#ifndef Xcoco2d_GameScene_h
#define Xcoco2d_GameScene_h
#include "cocos2d.h"
#include "MyPlane.h"
#include "EnemyPlane.h"
using namespace cocos2d;
class GameScene : public cocos2d::CCLayer {
private:
MyPlane *plane;
EnemyPlane *enemys;
CCLabelAtlas *scoreValue;
CCLabelAtlas *lifeValue;
CCArray *enemyPlanes;
public:
virtual bool init();
static CCScene* scene();
CREATE_FUNC(GameScene);
virtual bool ccTouchBegan(CCTouch *pTouch, CCEvent *pEvent);
virtual void ccTouchMoved(CCTouch *pTouch, CCEvent *pEvent);
virtual void ccTouchEnded(CCTouch *pTouch, CCEvent *pEvent);
virtual void registerWithTouchDispatcher(void);
void addEnemyPlane();
void toMainMenu();
void resumeGame();
void pauseToMenu();
void updatePlane();
void updateSprite();
void updatePlaneLife();
void planeExplosion(CCSprite *sp, int tag);
void spriteMoveFinished(CCObject *sender);
void gameover();
void transtion();
char* itoa(int num, char *str);
};
#endif
#include "GameBeigin.h"
#include "GameScene.h"
#include "Background.h"
#include "SimpleAudioEngine.h"
using namespace cocos2d;
CCScene* GameScene::scene()
{
CCScene *scene = CCScene::create();
GameScene *layer = GameScene::create();
scene->addChild(layer);
return scene;
}
bool GameScene::init()
{
if (!(CCLayer::init())) {
return false;
}
srand(time(NULL));
this->setTouchEnabled(true);
this->enemyPlanes = CCArray::create();
this->enemyPlanes->retain();
//添加背景:Background;
Background *bgd = Background::create("Road.png");
this->addChild(bgd);
//添加主角飞机:Planes;
this->plane = MyPlane::create("plane.png", CCRectMake(0, 90, 50, 26), 1);
this->addChild(plane);
//添加敌机:EnemyPlane:
this->schedule(schedule_selector(GameScene::addEnemyPlane), 0.5);
//碰撞检测:
this->schedule(schedule_selector(GameScene::updatePlane));
this->schedule(schedule_selector(GameScene::updateSprite));
//设置游戏中的菜单:
CCMenuItemFont *menuFont = CCMenuItemFont::create("Menu", this, menu_selector(GameScene::pauseToMenu));
menuFont->setPosition(ccp(0.0, 0.0));
menuFont->setColor(ccc3(153, 204, 81));
menuFont->setScale(0.5);
CCMenu *menu = CCMenu::create(menuFont, NULL);
menu->setPosition(ccp(20, 470));
this->addChild(menu,1);
//显示杀敌数:
char s[5];
itoa(this->plane->score, s);
scoreValue = CCLabelAtlas::create(s, "fps_images.png", 12, 32, '.');
scoreValue->setPosition(ccp(280.0, 460.0));
this->addChild(scoreValue);
//显示生命值:
lifeValue = CCLabelAtlas::create("111", "life.png", 10, 10, '1');
lifeValue->setPosition(ccp(5, 5));
this->addChild(lifeValue);
return true;
}
//游戏界面菜单的实现;
void GameScene::pauseToMenu()
{
CCDirector::sharedDirector()->pause();
//添加游戏场景里的菜单;
CCMenuItemImage *resume =new CCMenuItemImage();
resume->initWithNormalImage("menuResume.png",
"menuResume.png",
"menuResume.png",
this, menu_selector(GameScene::resumeGame));
resume->setPosition(ccp(0, 50));
CCMenuItemImage *toMainMenu = new CCMenuItemImage();
toMainMenu->initWithNormalImage("menuBack.png",
"menuBack.png",
"menuBack.png",
this, menu_selector(GameScene::toMainMenu));
toMainMenu->setPosition(ccp(0, -50));
CCMenu *menu = CCMenu::create(resume, toMainMenu, NULL);
menu->setTag(88);
menu->setPosition(ccp(160, 240));
this->addChild(menu, 2);
resume->release();
toMainMenu->release();
}
void GameScene::toMainMenu()
{
CocosDenshion::SimpleAudioEngine::sharedEngine()->pauseAllEffects();
CCDirector::sharedDirector()->resume();
this->removeChildByTag(88);
CCTransitionFlipAngular*y= CCTransitionFlipAngular::create(1.0, GameBeigin::scene());
CCDirector::sharedDirector()->replaceScene(y);
}
void GameScene::resumeGame()
{
CCDirector::sharedDirector()->resume();
this->removeChildByTag(88);
}
void GameScene::gameover()
{
CCSize size = CCDirector::sharedDirector()->getWinSize();
if(!this->plane->life)
{
CCDirector::sharedDirector()->pause();
CCLayerColor * color = CCLayerColor::create(ccc4(0, 0, 0, 120));
color->setTag(33);
this->addChild(color);
this->unschedule(schedule_selector(GameScene::updatePlane));
this->unschedule(schedule_selector(GameScene::updateSprite));
this->stopAllActions();
CCSprite * s = CCSprite::create("score.png" );
s->setTag(66);
s->setScaleX(1.25);
s->setScaleY(1.5);
s->setPosition(ccp(size.width/2,size.height/2));
this->addChild(s, 2);
CCLabelTTF * score = CCLabelTTF::create("Score", "Helvetica-BoldOblique", 34);
score->setPosition(ccp(100,180));
score->setColor(ccc3(255, 0, 0));
s->addChild(score);
CCLabelTTF * value = CCLabelTTF::create(scoreValue->getString(), "Helvetica-BoldOblique", 25);
value->setColor(ccc3(255, 255, 0));
value->setPosition(ccp(100,100));
s->addChild(value);
CCMenuItemFont * menue = CCMenuItemFont::create("back", this, menu_selector(GameScene::transtion));
CCMenu * m = CCMenu::create(menue,NULL);
m->setPosition(100,40);
s->addChild(m);
}
}
void GameScene::transtion()
{
CCDirector::sharedDirector()->resume();
this->removeChildByTag(33);
this->removeChildByTag(66);
CCTransitionCrossFade * t = CCTransitionCrossFade::create(1.0, GameBeigin::scene());
CCDirector::sharedDirector()->replaceScene(t);
}
//添加敌机;
void GameScene::addEnemyPlane()
{
CCLog("%d",this->plane->bulletArray->count());
CCLog("%d",this->enemyPlanes->count());
int i = rand() % 5;
int j = rand() % 2;
CCRect planeRect = CCRectMake(42*i, 50*j, 30, 40);
this->enemys = EnemyPlane::create("enemyPlane.png", planeRect, 1, true, 2);
this->addChild(enemys);
this->enemyPlanes->addObject(enemys);
int t= enemys->randomPoint(3);
CCMoveTo *action1 = CCMoveTo::create(t, ccp(enemys->randomPoint(2), -50));
CCCallFuncN *action2 = CCCallFuncN::create(this, callfuncN_selector(GameScene::spriteMoveFinished));
CCDelayTime *action = CCDelayTime::create(t);
CCSequence *sequence = CCSequence::create(action, action2, NULL);
this->enemys->planSprite->runAction(action1);
this->enemys->runAction(sequence);
}
//移除屏幕上的敌机;
void GameScene::spriteMoveFinished(CCObject *sender)
{
EnemyPlane *bt = (EnemyPlane *)sender;
bt->removeAllChildren();
this->enemyPlanes->removeObject(bt);
this->removeChild(bt);
}
//飞机爆炸效果-粒子系统的实现;
void GameScene::planeExplosion(CCSprite *sp, int tag)
{
if (tag == 1)
{
CCParticleSystemQuad *system=new CCParticleSystemQuad();
system->initWithFile("explosion.plist");
system->setPosition(sp->getPosition());
this->addChild(system);
CocosDenshion::SimpleAudioEngine::sharedEngine()->playEffect("explosion_large.caf");
system->release();
}
else
{
CCParticleSystemQuad *system=new CCParticleSystemQuad();
system->initWithFile("ExplodingRing.plist");
system->setPosition(sp->getPosition());
this->addChild(system);
CocosDenshion::SimpleAudioEngine::sharedEngine()->playEffect("explosion_small.caf");
system->release();
}
}
//触摸事件控制主角飞机移动;
bool GameScene::ccTouchBegan(CCTouch *pTouch, CCEvent *pEvent)
{
this->plane->moveToP(this->convertToNodeSpace(pTouch->getLocation()), 1);
return true;
}
void GameScene::ccTouchMoved(CCTouch *pTouch, CCEvent *pEvent)
{
CCPoint touchLocation = this->convertToNodeSpace(pTouch->getLocation());
CCPoint oldTouchLocation = pTouch->getPreviousLocationInView();
oldTouchLocation = CCDirector::sharedDirector()->convertToGL(oldTouchLocation);
oldTouchLocation = this->convertToNodeSpace(oldTouchLocation);
CCPoint translation = ccpSub(touchLocation, oldTouchLocation);
this->plane->moveToP(translation, 2);
}
void GameScene::ccTouchEnded(CCTouch *pTouch, CCEvent *pEvent)
{
}
void GameScene::registerWithTouchDispatcher(void)
{
CCDirector::sharedDirector()->getTouchDispatcher()->addTargetedDelegate(this, 0, true);
}
//主角子弹与敌机的碰撞测试:
void GameScene::updatePlane()
{
CCArray *bullets = this->plane->bulletArray;
CCArray *bulletToDelete = CCArray::create();
bulletToDelete->retain();
for(int i = 0; i < bullets->count(); i++)
{
CCSprite *bullet = (CCSprite *)bullets->objectAtIndex(i);
CCRect bulletRect = CCRectMake( bullet->getPositionX(),
bullet->getPositionY(),
bullet->getTextureRect().size.width,
bullet->getTextureRect().size.height);
CCArray *enemies = this->enemyPlanes;
CCArray *enemiesToDelete = CCArray::create();
enemiesToDelete->retain();
for (int j = 0; j < enemies->count(); j++)
{
EnemyPlane *enemy =(EnemyPlane *)enemies->objectAtIndex(j);
CCRect enemyRect = CCRectMake(enemy->planSprite->getPositionX() - 10,
enemy->planSprite->getPositionY(),
enemy->planSprite->getTextureRect().size.width - 5,
enemy->planSprite->getTextureRect().size.height);
if (bulletRect.intersectsRect(enemyRect))
{
enemiesToDelete->addObject(enemy);
}
}
for (int j = 0; j < enemiesToDelete->count(); j++)
{
EnemyPlane *sp = (EnemyPlane *)enemiesToDelete->objectAtIndex(j);
int life = sp->life;
life = life - this->plane->bullet->force;
if (life <= 0)
{
this->plane->score++;
char s[5];
itoa(this->plane->score, s);
scoreValue->setString(s);
this->planeExplosion(sp->planSprite, 1);
this->spriteMoveFinished(sp);
}
sp->life = life;
}
if (enemiesToDelete->count() > 0)
{
bulletToDelete->addObject(bullet);
}
enemiesToDelete->release();
}
for (int j = 0; j < bulletToDelete->count(); j++)
{
this->plane->spriteMoveFinished(bulletToDelete->objectAtIndex(j));
}
bulletToDelete->release();
}
//敌方飞机或子弹与主角的碰撞测试:
void GameScene::updateSprite()
{
CCRect leaderPlaneRect = CCRectMake(this->plane->planSprite->getPositionX() + 5,
this->plane->planSprite->getPositionY() - 10,
this->plane->planSprite->getTextureRect().size.width - 5,
this->plane->planSprite->getTextureRect().size.height);
CCArray *enemyToDelete = CCArray::create();
enemyToDelete->retain();
for (int i = 0; i < this->enemyPlanes->count(); i++)
{
EnemyPlane *enemyToTest = (EnemyPlane *)this->enemyPlanes->objectAtIndex(i);
CCRect enemyToTestRect = CCRectMake( enemyToTest->planSprite->getPositionX() + 15,
enemyToTest->planSprite->getPositionY(),
enemyToTest->planSprite->getTextureRect().size.width,
enemyToTest->planSprite->getTextureRect().size.height - 5);
if (enemyToTest->bulletArray->count() > 0)
{
CCArray *enemyBulletToDelete = CCArray::create();
enemyBulletToDelete->retain();
for (int j = 0; j < enemyToTest->bulletArray->count(); j++)
{
bullet *enemyBulletToTest = (bullet *)enemyToTest->bulletArray->objectAtIndex(j);
CCRect enemyBulletToTestRect = CCRectMake(enemyBulletToTest->getPositionX() + 20,
enemyBulletToTest->getPositionY(),
enemyBulletToTest->getTextureRect().size.width,
enemyBulletToTest->getTextureRect().size.height);
if (enemyBulletToTestRect.intersectsRect(leaderPlaneRect))
{
enemyBulletToDelete->addObject(enemyBulletToTest);
}
}
for (int k = 0; k < enemyBulletToDelete->count(); k++)
{
bullet *crashedBullet = (bullet *)enemyBulletToDelete->objectAtIndex(k);
this->plane->life -= crashedBullet->force;
this->gameover();
this->updatePlaneLife();
this->planeExplosion(this->plane->planSprite, 2);
enemyToTest->spriteMoveFinished(crashedBullet);
}
enemyBulletToDelete->release();
}
if (enemyToTestRect.intersectsRect(leaderPlaneRect)) {
enemyToDelete->addObject(enemyToTest);
}
}
if (enemyToDelete->count() > 0)
{
for (int k = 0; k < enemyToDelete->count(); k++)
{
EnemyPlane *crashedEnemyPlane = (EnemyPlane *)enemyToDelete->objectAtIndex(k);
this->planeExplosion(crashedEnemyPlane->planSprite, 2);
this->spriteMoveFinished(crashedEnemyPlane);
this->plane->life --;
this->updatePlaneLife();
}
}
enemyToDelete->release();
}
void GameScene::updatePlaneLife()
{
switch (this->plane->life)
{
case 0:
this->lifeValue->setString("");
break;
case 1:
this->lifeValue->setString("1");
break;
case 2:
this->lifeValue->setString("11");
break;
case 3:
this->lifeValue->setString("111");
break;
default:
break;
}
}
char* GameScene::itoa(int num, char *str)
{
int i = 0;
char index[] = "0123456789";
do {
str[i++] = index[num % 10];
num /= 10;
} while (num);
str[i] = '\0';
char temp;
for (int j = 0; j <= (i - 1) / 2; j++) {
temp = str[j];
str[j] = str[i-j-1];
str[i-j-1] = temp;
}
return str;
}
#ifndef Thunder_Background_h
#define Thunder_Background_h
#include "cocos2d.h"
class Background : public cocos2d::CCLayerColor {
private:
cocos2d::CCSprite *background;
cocos2d::CCSprite *background1;
cocos2d::CCPoint point;
cocos2d::CCPoint point1;
public:
virtual bool init(const char *pszFileName);
static Background *create(const char *pszFileName);
void backgroundMove();
void background1Move();
void setBackground(cocos2d::CCSprite *sprite);
};
#endif
#include "Background.h"
using namespace cocos2d;
bool Background::init(const char *pszFileName)
{
if (!(CCLayerColor::initWithColor(ccc4(0, 0, 0, 255)))) {
return false;
}
CCSize winsize = CCDirector::sharedDirector()->getWinSize();
background = CCSprite::create(pszFileName);
background->setPosition(ccp(winsize.width / 2, 0));
this->addChild(background);
background1 = CCSprite::create(pszFileName);
background1->setPosition(ccp(winsize.width / 2, 960));
this->addChild(background1);
this->scheduleOnce(schedule_selector(Background::backgroundMove), 1);
this->scheduleOnce(schedule_selector(Background::background1Move), 1);
return true;
}
Background *Background::create(const char *pszFileName)
{
Background *pRet = new Background();
if (pRet && pRet->init(pszFileName))
{
pRet->autorelease();
return pRet;
}
else
{
CC_SAFE_DELETE(pRet);
return NULL;
}
}
void Background::backgroundMove()
{
CCMoveTo *action1 = CCMoveTo::create(16, ccp(160, -960));
CCMoveTo *action3 = CCMoveTo::create(16, ccp(160, 0));
CCCallFuncN *action2 = CCCallFuncN::create(this, callfuncN_selector(Background::setBackground));
CCSequence *sequence = CCSequence::create(action1, action2, action3, NULL);
CCRepeatForever *repeat = CCRepeatForever::create(sequence);
background->runAction(repeat);
}
void Background::background1Move()
{
CCMoveTo *action1 = CCMoveTo::create(16, ccp(160, -960));
CCMoveTo *action3 = CCMoveTo::create(16, ccp(160, 0));
CCCallFuncN *action2 = CCCallFuncN::create(this, callfuncN_selector(Background::setBackground));
CCSequence *sequence = CCSequence::create(action3, action1, action2, NULL);
CCRepeatForever *repeat = CCRepeatForever::create(sequence);
background1->runAction(repeat);
}
void Background::setBackground(cocos2d::CCSprite *sprite)
{
sprite->setPosition(ccp(160, 960));
}
#ifndef Thunder_File_h
#define Thunder_File_h
#include "cocos2d.h"
#include "bullet.h"
using namespace cocos2d;
class MyPlane:public CCLayer
{
public:
bullet *bullet;
CCSprite *planSprite;
CCArray *bulletArray;
int life;
int score;
int typeBullet;
public:
virtual bool init(const char *pszFileName, const cocos2d::CCRect &rect, int bulletType);
static MyPlane *create(const char *pszFileName, const cocos2d::CCRect &rect, int bulletType);
int randomPoint(int tag);
void moveToP(CCPoint p, int tag);
virtual void launchButtlet();
void spriteMoveFinished(CCObject *sender);
};
#endif
#include "MyPlane.h"
using namespace cocos2d;
bool MyPlane::init(const char *pszFileName, const cocos2d::CCRect &rect, int bulletType)
{
if (!(CCLayer::init())) {
return false;
}
this->typeBullet = bulletType;
this->score = 0;
this->life = 3;
this->setPosition(ccp(0, 0));
//初始化飞机精灵;
this->planSprite = CCSprite::create(pszFileName, rect);
this->planSprite->setPosition(ccp(160, 40));
this->addChild(planSprite);
//初始化数组;
this->bulletArray = CCArray::create();
bulletArray->retain();
//发射子弹;
this->schedule(schedule_selector( MyPlane::launchButtlet), 0.2);
return true;
}
MyPlane *MyPlane::create(const char *pszFileName, const cocos2d::CCRect &rect, int bulletType)
{
MyPlane *pRet = new MyPlane();
if (pRet && pRet->init(pszFileName, rect, bulletType))
{
pRet->autorelease();
return pRet;
}
else
{
CC_SAFE_DELETE(pRet);
return NULL;
}
}
void MyPlane::launchButtlet()
{
this->bullet = bullet::create(typeBullet);
bullet->setPosition(ccp(this->planSprite->getPositionX(),this->planSprite->getPositionY()+this->planSprite->getContentSize().height/2));
this->addChild(bullet);
this->bulletArray->addObject(bullet);
CCMoveTo *action = CCMoveTo::create((480-this->planSprite->getPositionY())/240, CCPointMake(this->planSprite->getPositionX(), 500));
CCCallFuncN *action2 = CCCallFuncN::create(this, callfuncN_selector(MyPlane::spriteMoveFinished));
CCSequence *sequence = CCSequence::create(action, action2, NULL);
bullet->runAction(sequence);
}
void MyPlane::spriteMoveFinished(CCObject *sender) {
CCSprite *bt = (CCSprite *)sender;
bulletArray->removeObject(bt);
this->removeChild(bt);
}
void MyPlane::moveToP(CCPoint p, int tag) {
//ccTouchBegan的飞机移动;
if (tag == 1) {
planSprite->stopActionByTag(10);
float s = sqrtf(powf(p.x - planSprite->getPositionX(), 2) +
powf(p.y - planSprite->getPositionY(), 2));
CCMoveTo *moveAction = CCMoveTo::create(s / 300, p);
moveAction->setTag(10);
planSprite->runAction(moveAction);
}else if(tag == 2){ //ccTouchMove的飞机移动;
CCPoint newPoint = ccpAdd(planSprite->getPosition(), p);
this->planSprite->setPosition(newPoint);
}
}
int MyPlane::randomPoint(int tag)
{
int rand;
switch (tag) {
case 0:
rand = random() % 3 + 2;
break;
case 1:
rand = random() % 320;
break;
case 2:
rand = random() % 480 - 80;
break;
case 3:
rand = random() % 3 + 2;
break;
default:
break;
}
return rand;
}
#ifndef Thunder_EnemyPlane_h
#define Thunder_EnemyPlane_h
#include "MyPlane.h"
#include "bullet.h"
using namespace cocos2d;
class EnemyPlane:public MyPlane
{
public:
virtual bool init(const char *pszFileName, const CCRect &rect, int life, bool isLaunchBullet, int bulletType);
static EnemyPlane *create(const char *pszFileName, const CCRect &rect, int life, bool isLaunchBullet, int bulletType);
virtual void launchButtlet();
};
#endif
#include "EnemyPlane.h"
using namespace cocos2d;
bool EnemyPlane::init(const char *pszFileName, const CCRect &rect, int life, bool isLaunchBullet, int bulletType)
{
if (!(CCLayer::init())) {
return false;
}
this->life = life;
this->typeBullet = bulletType;
this->setPosition(ccp(0, 0));
//初始化数组;
this->bulletArray = CCArray::create();
bulletArray->retain();
//初始化敌机精灵;
this->planSprite = CCSprite::create();
this->planSprite->initWithFile(pszFileName, rect);
this->planSprite->setPosition(ccp(randomPoint(1), 500.0));
this->addChild(planSprite);
if (isLaunchBullet) {
this->schedule(schedule_selector(EnemyPlane::launchButtlet), 0.8);
}
return true;
}
EnemyPlane *EnemyPlane::create(const char *pszFileName, const cocos2d::CCRect &rect, int life, bool isLaunchBullet, int bulletType)
{
EnemyPlane *pRet = new EnemyPlane();
if (pRet && pRet->init(pszFileName, rect, life, isLaunchBullet,bulletType))
{
pRet->autorelease();
return pRet;
}
else
{
CC_SAFE_DELETE(pRet);
return NULL;
}
}
void EnemyPlane::launchButtlet()
{
this->bullet = bullet::create(typeBullet);
bullet->setPosition(ccp(this->planSprite->getPositionX(),this->planSprite->getPositionY()-this->planSprite->getContentSize().height/2));
this->addChild(bullet);
this->bulletArray->addObject(bullet);
CCMoveTo *action = CCMoveTo::create(this->planSprite->getPositionY()/200, CCPointMake(randomPoint(1), -10));
CCCallFuncN *action2 = CCCallFuncN::create(this, callfuncN_selector(MyPlane::spriteMoveFinished));
CCSequence *sequence = CCSequence::create(action, action2, NULL);
bullet->runAction(sequence);
}
#ifndef __Thunder__bullet__
#define __Thunder__bullet__
#include "cocos2d.h"
using namespace cocos2d;
class bullet:public CCSprite
{
public:
int force;
virtual bool init(int typeBullet);
static bullet *create(int typeBullet);
};
#endif
#include "bullet.h"
using namespace cocos2d;
bullet *bullet::create(int typeBullet)
{
bullet *pRet = new bullet();
if (pRet && pRet->init(typeBullet))
{
pRet->autorelease();
return pRet;
}
else
{
CC_SAFE_DELETE(pRet);
return NULL;
}
}
bool bullet::init(int typeBullet)
{
switch (typeBullet) {
case 1:
CCSprite::initWithFile("boss1_bullet3.png", CCRectMake(1, 1, 13, 13));
this->force = 1;
break;
case 2:
CCSprite::initWithFile("dl_pe_a_04a.png", CCRectMake(0, 0, 6, 13));
this->force = 1;
break;
case 3:
break;
default:
break;
}
return true;
}