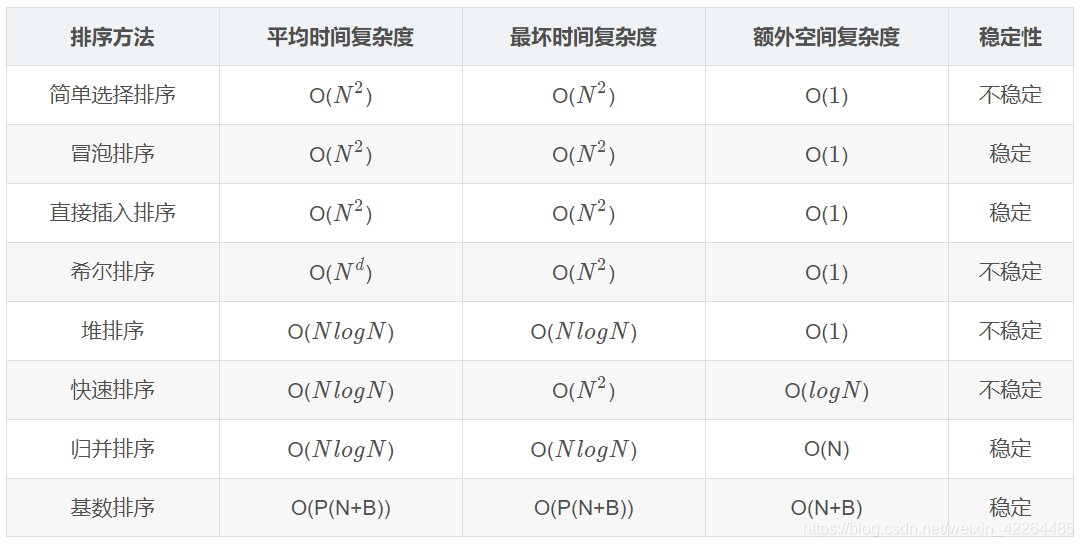
插入排序
#include <iostream>
#include <algorithm>
#include <cstdio>
using namespace std;
int const N = 10 + 5;
int main(){
int n = 10;
int test[N] = {12,9,2,31,10,1,15,7,8,11};
for(int i=1;i<n;i++){
int tmp = test[i],j;
for(j=i;j>0&&test[j-1]>tmp;j--)
test[j] = test[j-1];
test[j] = tmp;
}
for(int i=0;i<n;i++)
printf("%d ",test[i]);
return 0;
}
int is_insert(){ //判断是否是插入排序
int i = 1,j;
while(i <= n && b[i] <= b[i+1]) i++;
j = i + 1;
while(j <= n && a[j] == b[j]) j++;
if(j == n + 1) return i + 1; //如果是插入排序,返回下一个需要排序的元素下标
else return -1;
}
希尔排序
#include <cstdio>
#include <algorithm>
using namespace std;
int const N = 10 + 5;
void shell_sort(int test[N],int n){
int Sedgewick[] = {929, 505, 209, 109, 41, 19, 5, 1, 0};
int s=0;
while(Sedgewick[s++] > n);
for(;Sedgewick[s]>0;s++){
for(int i=Sedgewick[s];i<n;i++){
int tmp = test[i],j;
for(j=i;j>=Sedgewick[s]&&test[j-Sedgewick[s]]>tmp;j-=Sedgewick[s])
test[j] = test[j-Sedgewick[s]];
test[j] = tmp;
}
}
}
int main(){
int n = 10;
int test[N] = {12,9,2,31,10,1,15,7,8,11};
shell_sort(test,n);
for(int i=0;i<n;i++)
printf("%d ",test[i]);
}
冒泡排序
#include <cstdio>
#include <algorithm>
using namespace std;
int const N = 10 + 5;
int main(){
int n = 10;
int test[N] = {12,9,2,31,10,1,15,7,8,11};
for(int i=n-1;i>=0;i--){
bool flag = false;
for(int j=0;j<i;j++){
if(test[j] > test[j+1]){
swap(test[j],test[j+1]);
flag = true;
}
}
if(!flag) break;
}
for(int i=0;i<n;i++)
printf("%d ",test[i]);
}
快速排序
#include <bits/stdc++.h>
using namespace std;
int const N = 20;
int Partition(int a[N],int low,int high){
int pivotkey = a[low];
while(low < high){
while(low < high && a[high] >= pivotkey) high--;
swap(a[low],a[high]);
while(low < high && a[low] <= pivotkey) low++;
swap(a[low],a[high]);
}
return low;
}
void Qsort(int a[N],int low,int high){
if(low < high){
int pivot = Partition(a,low,high);
Qsort(a,low,pivot-1);
Qsort(a,pivot+1,high);
}
}
void Quick_Sort(int a[N],int n){
Qsort(a,0,n-1);
}
int main(){
int n = 10;
int test[N] = {12,9,2,31,10,1,15,7,8,11};
Quick_Sort(test,n);
for(int i=0;i<n;i++)
printf("%d ",test[i]);
return 0;
}
选择排序
#include <iostream>
#include <algorithm>
#include <cstdio>
using namespace std;
int const N = 10 + 5;
int main(){
int n = 10;
int test[N] = {12,9,2,31,10,1,15,7,8,11};
for(int i=0;i<n;i++){
int k = i;
for(int j=i+1;j<n;j++){
if(test[j] < test[k]) swap(j,k);
}
if(k != i) swap(test[k],test[i]);
}
for(int i=0; i<n; i++)
printf("%d ",test[i]);
return 0;
}
堆排序
#include <bits/stdc++.h>
using namespace std;
int const N = 1000 + 10;
void PercDown(int T[N],int p,int n){
int parent,child;
int tmp = T[p];
for(parent=p;2*parent<=n;parent=child){
child = 2 * parent;
if(child != n && T[child] < T[child+1]) child++;
if(T[child] > tmp) T[parent] = T[child];
else break;
}
T[parent] = tmp;
}
void Heap_Sort(int a[N],int n){
for(int i=n/2;i>=1;i--)
PercDown(a,i,n);
for(int i=n;i>=1;i--){
swap(a[i],a[1]);
PercDown(a,1,i-1);
}
}
int main(){
int n = 10;
int test[15] = {0,20,12,3,8,23,11,17,14,21,10};
Heap_Sort(test,n);
for(int i=1;i<=n;i++)
printf("%d ",test[i]);
return 0;
}
归并排序
#include <bits/stdc++.h>
using namespace std;
int const N = 10 + 5;
void merge(int a[N],int tmp[N],int left,int mid,int right){
int l = left,r = mid,t = left;
while(l <= mid-1 && r <= right){
if(a[l] < a[r]) tmp[t++] = a[l++];
else tmp[t++] = a[r++];
}
while(l <= mid-1) tmp[t++] = a[l++];
while(r <= right) tmp[t++] = a[r++];
for(int i=left;i<=right;i++)
a[i] = tmp[i];
}
void msort(int a[N],int tmp[N],int left,int right){
if(left < right){
int mid = (left + right) >> 1;
msort(a,tmp,left,mid);
msort(a,tmp,mid + 1,right);
merge(a,tmp,left,mid + 1,right);
}
}
void merge_sort(int test[N],int n){
int *tmp = (int*)malloc((n+1)*sizeof(int));
msort(test,tmp,0,n-1);
}
int main(){
int n = 10;
int test[N] = {12,9,2,31,10,1,15,7,8,11};
merge_sort(test,n);
for(int i=0; i<n; i++)
printf("%d ",test[i]);
return 0;
}
基数排序
#include <bits/stdc++.h>
using namespace std;
int const MAXDIGIT = 4;
int const RADIX = 10;
int const N = 100;
typedef struct Node{
int key;
struct Node *next;
}*node;
typedef struct BUCKET{
node head,tail;
}Bucket[RADIX];
int digit(int x,int d){ //获取从右开始第d位数
for(int i=1;i<=d-1;i++)
x /= 10;
return x % 10;
}
void radix_sort(int test[N],int n){
Bucket bucket;
node list = NULL,tmp,p;
for(int i=0;i<RADIX;i++)
bucket[i].head = bucket[i].tail = NULL;
for(int i=0;i<n;i++){ //把数字串成一条链
tmp = (node)malloc(sizeof(struct Node));
tmp->key = test[i];
tmp->next = list;
list = tmp;
}
for(int d=1;d<=MAXDIGIT;d++){ //枚举从右往左第d位
p = list;
while(p){
int di = digit(p->key,d);
tmp = p;
p = p->next;
tmp->next = NULL;
if(bucket[di].head == NULL)
bucket[di].tail = bucket[di].head = tmp;
else{
bucket[di].tail->next = tmp;
bucket[di].tail = tmp;
}
}
list = NULL;
for(int di=RADIX-1;di>=0;di--){ //为list重新排序
if(bucket[di].head){
bucket[di].tail->next = list;
list = bucket[di].head;
bucket[di].head = bucket[di].tail = NULL;
}
}
}
for(int i=0;i<n;i++){
tmp = list;
list = list->next;
test[i] = tmp->key;
free(tmp);
}
}
int main(){
int n = 10;
int test[N] = {12,9,2,31,10,1,15,7,8,11};
radix_sort(test,n);
for(int i=0;i<n;i++)
printf("%d ",test[i]);
}