知识点: | |
---|
| 1、事件委托,监听内容的实时输入 |
| 2、监听发布按钮点击,创建方法,动态增加显示节点 |
| 3、a标签里动态加数字,parseInt取整数 |
| 4、创建节点方法,动态传递text值,要注意书写格式,会有点繁琐 |
| 5、动态的显示时间,转换时间的显示格式 |
<script type="text/javascript">
$(function() {
$('body').delegate('.text', 'propertychange input', function() {
if ($(this).val().length > 0) {
$('.m-send').prop('disabled', false);
}else {
$('.m-send').prop('disabled', true);
}
});
$('.m-send').click(function() {
var $text = $('.text').val();
var $weibo = crearEles($text);
$('.m-content-info').prepend($weibo);
});
$('body').delegate('.infoTop','click', function() {
$(this).text(parseInt($(this).text()) + 1);
})
$('body').delegate('.infoDown','click', function() {
$(this).text(parseInt($(this).text()) + 1);
})
$('body').delegate('.infoDel','click', function() {
$(this).parents('.info-box').remove();
})
function crearEles(text) {
var $weibo = $("<div class=\'info-box\'>\n"+
"<div class=\'m-info-type\'>" + text + "</div>\n" +
"<div class=\'m-info-item clearfix\'>\n"+
"<div class=\'m-left-img\'>\n"+
"<span>" + formartTime() + "</span>\n"+
"</div>\n"+
"<div class=\'m-right-img\'>\n"+
"<a href=\'javascript:;\' class=\'infoTop\'>0</a>\n"+
"<a href=\'javascript:;\' class=\'infoDown\'>0</a>\n"+
"<a href=\'javascript:;\' class=\'infoDel\'>0</a>\n"+
"</div>\n"+
"</div>\n"+
"</div>");
return $weibo;
};
function formartTime() {
var date = new Date();
var arr = [date.getFullYear() + "-",
date.getMonth() + 1 + "-",
date.getDate() + " ",
date.getHours() + ":",
date.getMinutes() + ":",
date.getSeconds()
];
return arr.join('');
}
})
</script>
<div class="contaniner">
<div class="content">
<div class="m-centent-title">
<textarea class="text"></textarea>
<input type="button" class="m-send" name="" value="立即发布" disabled>
</div>
<div class="m-content-info">
<div class="info-box">
<div class="m-info-type">111</div>
<div class="m-info-item clearfix">
<div class="m-left-img">
<span>2019-01-08</span>
</div>
<div class="m-right-img">
<a href="javascript:;">0</a>
<a href="javascript:;">0</a>
<a href="javascript:;">删除</a>
</div>
</div>
</div>
</div>
</div>
<div class="left">左</div>
<div class="right">右</div>
</div>
<style type="text/css">
*{
margin: 0;
padding: 0;
}
.clearfix {
zoom: 1;
}
.clearfix:after {
content: '.';
display: block;
height: 0;
clear: both;
line-height: 0;
overflow: hidden;
visibility: hidden;
}
.contaniner {
position: relative;
padding: 0 200px;
zoom: 1;
overflow: hidden;
background: #fff;
}
.contaniner > div {
float: left;
}
.contaniner .content {
position: relative;
width: 100%;
min-height: 400px;
background: #fff;
}
.left {
position: relative;
left: -200px;
width: 200px;
padding-bottom: 9999px;
margin-bottom: -9999px;
margin-left: -100%;
background: #ffcc00;
}
.right {
position: relative;
right: -200px;
width: 200px;
padding-bottom: 9999px;
margin-bottom: -9999px;
margin-left: -200px;
background: #ffbcc8;
}
.m-content-info {
padding: 15px;
}
.m-info-item {
width: 100%;
overflow: hidden;
}
.m-left-img {
float: left;
font-size: 14px;
}
.m-right-img {
float: right;
font-size: 14px;
}
.m-right-img a {
display: inline-block;
padding-left: 30px;
min-height: 30px;
margin: 0 10px;
line-height: 2.2;
color: #999;
background: url('./img/css_sprites.png') no-repeat 0 0;
background-size: 40px;
}
.m-right-img a:nth-child(1) {
background-position: -5px -5px;
}
.m-right-img a:nth-child(2) {
background-position: -5px -45px;
}
.m-right-img a:nth-child(3) {
background-position: -5px -85px;
}
.m-centent-title {
padding: 15px;
text-align: right;
border: 1px solid #ddd;
}
.m-centent-title .text {
width: 100%;
height: 80px;
text-align: left;
resize: none;
outline: none;
}
.m-send {
display: inline-block;
padding: 10px 30px;
margin: 15px 0;
border: none;
color: #fff;
background: #ffd300;
}
</style>
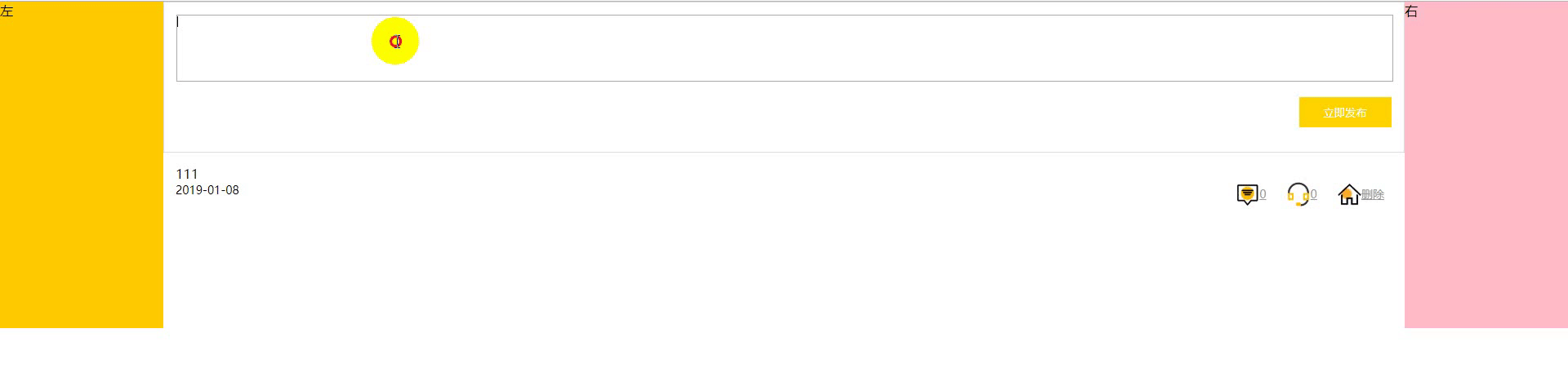