node.c
#include "node.h"
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include "student.h"
node_t *node_creat()
{
node_t *head = malloc(sizeof(node_t));
if (head == NULL)
{
return NULL;
}
head->data = NULL;
head->next = NULL;
return head;
}
int node_inser_head(node_t *head,const void * data,int size)
{
if (head == NULL || head->data != NULL || data == NULL || size <= 0)
{
return -1;
}
node_t *temp_head = head;
node_t *temp_node_new = malloc(sizeof(node_t));
if (temp_node_new == NULL)
{
printf("空间申请失败!\n");
return -1;
}
temp_node_new->data = malloc(size);
if (temp_node_new->data == NULL)
{
free(temp_node_new);
return -1;
}
memcpy(temp_node_new->data, data, size);
temp_node_new->next=temp_head->next;
temp_head->next=temp_node_new;
return 0;
}
int node_inser_end(node_t *head, const void *data, int size)
{
if (head == NULL || head->data != NULL || data == NULL || size <= 0)
{
return -1;
}
node_t *temp_head = head;
while (temp_head->next != NULL)
{
temp_head = temp_head->next;
}
node_t *temp_node_new = malloc(sizeof(node_t));
if (temp_node_new == NULL)
{
printf("空间申请失败!\n");
return -1;
}
temp_node_new->data = malloc(size);
if (temp_node_new->data == NULL)
{
free(temp_node_new);
return -1;
}
memcpy(temp_node_new->data, data, size);
temp_head->next = temp_node_new;
temp_node_new->next = NULL;
return 0;
}
int node_inser_node(node_t *node,const void * data,int size)
{
if (node == NULL || data == NULL || size <= 0)
{
return -1;
}
node_t *temp_node = node;
node_t *temp_node_new = malloc(sizeof(node_t));
if (temp_node_new == NULL)
{
printf("空间申请失败!\n");
return -1;
}
temp_node_new->data = malloc(size);
if (temp_node_new->data == NULL)
{
free(temp_node_new);
return -1;
}
memcpy(temp_node_new->data, data, size);
temp_node_new->next=temp_node->next;
temp_node->next=temp_node_new;
return 0;
}
void node_foreach(node_t *head, void (*Show)(void *))
{
if (head == NULL)
{
return;
}
node_t *temp_head = head;
while (temp_head != NULL)
{
if (temp_head->data != NULL)
{
Show(temp_head->data);
}
temp_head = temp_head->next;
}
}
void node_free(node_t **head)
{
node_t *temp_head = *head;
node_t *temp_next = NULL;
while (temp_head != NULL)
{
temp_next = temp_head->next;
if (temp_head->data != NULL)
{
free(temp_head->data);
free(temp_head);
}
temp_head = temp_next;
}
free(*head);
*head = NULL;
}
node_t *node_find(node_t *head, const void *data, int mode, int (*cmp)(const void *, const void *, int))
{
if (head == NULL || head->data != NULL || data == NULL || mode <= 0)
{
return NULL;
}
node_t *temp_head = head;
while (temp_head != NULL)
{
if (temp_head->data != NULL)
{
if (cmp(temp_head->data, data, mode) == 0)
{
return temp_head;
}
}
temp_head = temp_head->next;
}
return NULL;
}
int node_updata(node_t *node, const void *data, int mode, int (*updata)(void *, const void *, const int))
{
if (node == NULL || data == NULL || node->data == NULL || mode <= 0)
{
return -1;
}
node_t *temp_node = node;
if (updata(temp_node->data, data, mode) == 0)
{
return 0;
}
else
{
return 1;
}
}
int node_delete(node_t *head, node_t *node)
{
if (node == NULL || head->data != NULL || head->next == NULL)
{
return -1;
}
node_t *temp_head = head;
while (temp_head != node)
{
if (temp_head->next == node)
{
temp_head->next = node->next;
free(node->data);
free(node);
return 0;
}
temp_head = temp_head->next;
}
return 1;
}
node.h
#ifndef NODE_H
#define NODE_H
typedef struct Node
{
void *data;
struct Node *next;
}node_t;
node_t *node_creat();
int node_inser_end(node_t *head,const void * data,int size);
int node_inser_head(node_t *head,const void * data,int size);
int node_inser_node(node_t *node,const void * data,int size);
void node_foreach(node_t *head,void(*Show)(void *));
void node_free(node_t **head);
node_t *node_find(node_t *head,const void *data,int mode,int (*cmp)(const void *,const void *,int));
int node_updata(node_t *node,const void *data,int mode,int (*updata)(void *,const void *,const int));
int node_delete( node_t *head,node_t *node);
#endif
student.c
#include "student.h"
#include <stdio.h>
#include <string.h>
void student_Print(void *data)
{
student_t *v = data;
printf("姓名:%s\t年龄:%d\t身高:%d\t\n", v->name, v->age, v->high);
}
int student_Cmp(const void *data_1, const void *data_2, const int mode)
{
const student_t *v = data_1;
const int *num2 = data_2;
switch (mode)
{
case 1:
return strcmp(v->name, data_2);
case 2:
return (*v).age - (*num2);
case 3:
return (*v).high - (*num2);
case 4:
return memcmp(v, data_2, sizeof(student_t));
default:
return -1;
}
}
int student_Updata(void *data_1, const void *data_2, const int mode)
{
student_t *v = data_1;
const int *num2 = data_2;
switch (mode)
{
case 1:
strcpy(v->name,data_2);
return 0;
case 2:
v->age = *num2;
return 0;
case 3:
v->high = *num2;
return 0;
case 4:
memcpy(v, data_2, sizeof(student_t));
return 0;
default:
return -1;
}
}
student.h
#ifndef STUDENT_H
#define STUDENT_H
typedef struct Student
{
char name[20];
int age;
int high;
} student_t;
void student_Print(void *data);
int student_Cmp(const void *data_1, const void *data_2, const int mode);
int student_Updata(void *data_1,const void *data_2,const int mode);
#endif
main.c
#include <stdio.h>
#include "node.h"
#include "student.h"
#include <string.h>
int main()
{
node_t *head = node_creat();
student_t stu[6];
strcpy(stu[0].name, "张三丰");
stu[0].age = 100;
stu[0].high = 200;
strcpy(stu[1].name, "张四丰");
stu[1].age = 101;
stu[1].high = 201;
strcpy(stu[2].name, "张五丰");
stu[2].age = 102;
stu[2].high = 202;
strcpy(stu[3].name, "张六丰");
stu[3].age = 103;
stu[3].high = 203;
strcpy(stu[4].name, "张七丰");
stu[4].age = 104;
stu[4].high = 204;
node_inser_end(head, &stu[0], sizeof(student_t));
node_inser_head(head, &stu[1], sizeof(student_t));
node_inser_end(head, &stu[2], sizeof(student_t));
node_inser_head(head, &stu[3], sizeof(student_t));
node_inser_end(head, &stu[4], sizeof(student_t));
printf("---------------------------------\n");
node_foreach(head, student_Print);
printf("---------------------------------\n");
char findname[] = "张五丰";
const int findage = 104;
const int findhigh = 204;
node_t *findnode = node_find(head, findname, 1, student_Cmp);
if (findnode != NULL)
{
student_t *v = findnode->data;
printf("找到了!\n");
printf("姓名:%s\t年龄:%d\t身高:%d\t\n", v->name, v->age, v->high);
char newname[] = "张八丰";
const int newage = 110;
const int newhigh = 210;
const student_t w = {"张八丰", 110, 210};
int temp;
temp = node_updata(findnode, newname, 1, student_Updata);
if (temp == 0)
{
printf("修改成功!\n");
}
else
{
printf("修改失败! \n");
}
}
else
{
printf("没找到\n");
}
printf("---------------------------------\n");
node_foreach(head, student_Print);
printf("---------------------------------\n");
if (node_delete(head, findnode) == 0)
{
printf("删除成功!\n");
}
else
{
printf("删除失败!\n");
}
printf("---------------------------------\n");
node_foreach(head, student_Print);
printf("---------------------------------\n");
strcpy(findname, "张三丰");
findnode = node_find(head, findname, 1, student_Cmp);
strcpy(stu[5].name, "张九丰");
stu[5].age = 800;
stu[5].high = 300;
if (node_inser_node(findnode, &stu[5], sizeof(student_t)) == 0)
{
printf("插入成功!\n");
}
else
{
printf("插入失败!\n");
}
printf("---------------------------------\n");
node_foreach(head, student_Print);
printf("---------------------------------\n");
node_free(&head);
if (head == NULL)
{
printf("释放成功!\n");
}
else
{
printf("释放失败!\n");
}
return 0;
}
运行结果
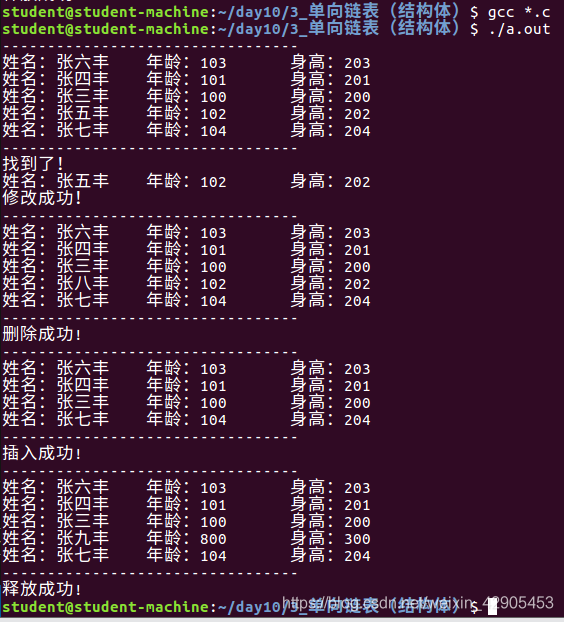