目录
- 前言
- 一、使用 man 2 查看的系统调用(system calls)
- 二、使用 man 3 查看的库函数(library calls)
- 01. 请点击链接跳转:socket 编程
- 01. 时间相关函数
- 01. `dlopen()`
- 02. `dlsym()`
- 03. `fgets()`
- 04. `getline()`
- 05. `issapce()`
- 06. `memcpy()`
- 07. `memmove()`
- 08. `memset()`
- 09. `popen()`
- 10. `pthread_setname_np()` (同 “一、3. `prctl()`” )
- 11. `remove()`
- 12. `snprintf()`
- 13. `strcat()`
- 14. `strcmp()`
- 15. `strlen()`
- 16. `strncat()`
- 17. `strncpy()`
- 18. `strstr()`
前言
本文整理记录嵌入式 Linux C 应用开发过程中常用到的C库函数,也即glibc。遇到一个就记录一个,私以为如此,会比通读《UNIX环境高级编程》效率高点 😃
一、使用 man 2 查看的系统调用(system calls)
01. 时间相关的系统调用
结构体:
struct timeval
{
time_t tv_sec; /* seconds */ //秒数
suseconds_t tv_usec; /* microseconds */ //秒后的小数部分,以微秒为单位
};
struct timezone
{
int tz_minuteswest; /* minutes west of Greenwich */ //当前时区与GMT的偏移量,以分钟为单位。正值表示东时区,负值表示西时区。
int tz_dsttime; /* type of DST correction */ //不使用夏令时:0,使用夏令时:1,未知:2
};
01.01 gettimeofday()
头文件和函数原型:
#include <sys/time.h>
int gettimeofday(struct timeval *tv, struct timezone *tz);
功能: 获取struct timeval
类型的系统时间。Linux系统编程已经废弃struct timezone
,因此tz
的位置传入NULL
即可。
01.02 settimeofday()
头文件和函数原型:
#include <sys/time.h>
int settimeofday(const struct timeval *tv, const struct timezone *tz);
功能: 依入口参数tv
设置系统时间。Linux系统编程已经废弃struct timezone
,因此tz
的位置传入NULL
即可。
01.03 time()
头文件和函数原型:
#include <time.h>
time_t time(time_t *tloc);
功能: 返回Unix时间戳,如果tloc
不为NULL
,则tloc
指向的内存单元中也保存有当前Unix时间戳。
02. ioctrl()
03. mmap()
头文件和函数原型:
#include <sys/mman.h>
void *mmap(void *addr, size_t length, int prot, int flags, int fd, off_t offset);
void munmap(void *addr, size_t length);
04. prctl()
(同 “二、11. pthread_setname_np()
”)
头文件和函数原型:
#include <sys/prctl.h>
int prctl(int option, unsigned long arg2, unsigned long arg3, unsigned long arg4, unsigned long arg5);
举例:
const char *new_thread_name = "my_thread";
prctl(PR_SET_NAME, new_thread_name); //设置线程名
05. settimeofday()
06. setsockopt()
07. select()
二、使用 man 3 查看的库函数(library calls)
01. 请点击链接跳转:socket 编程
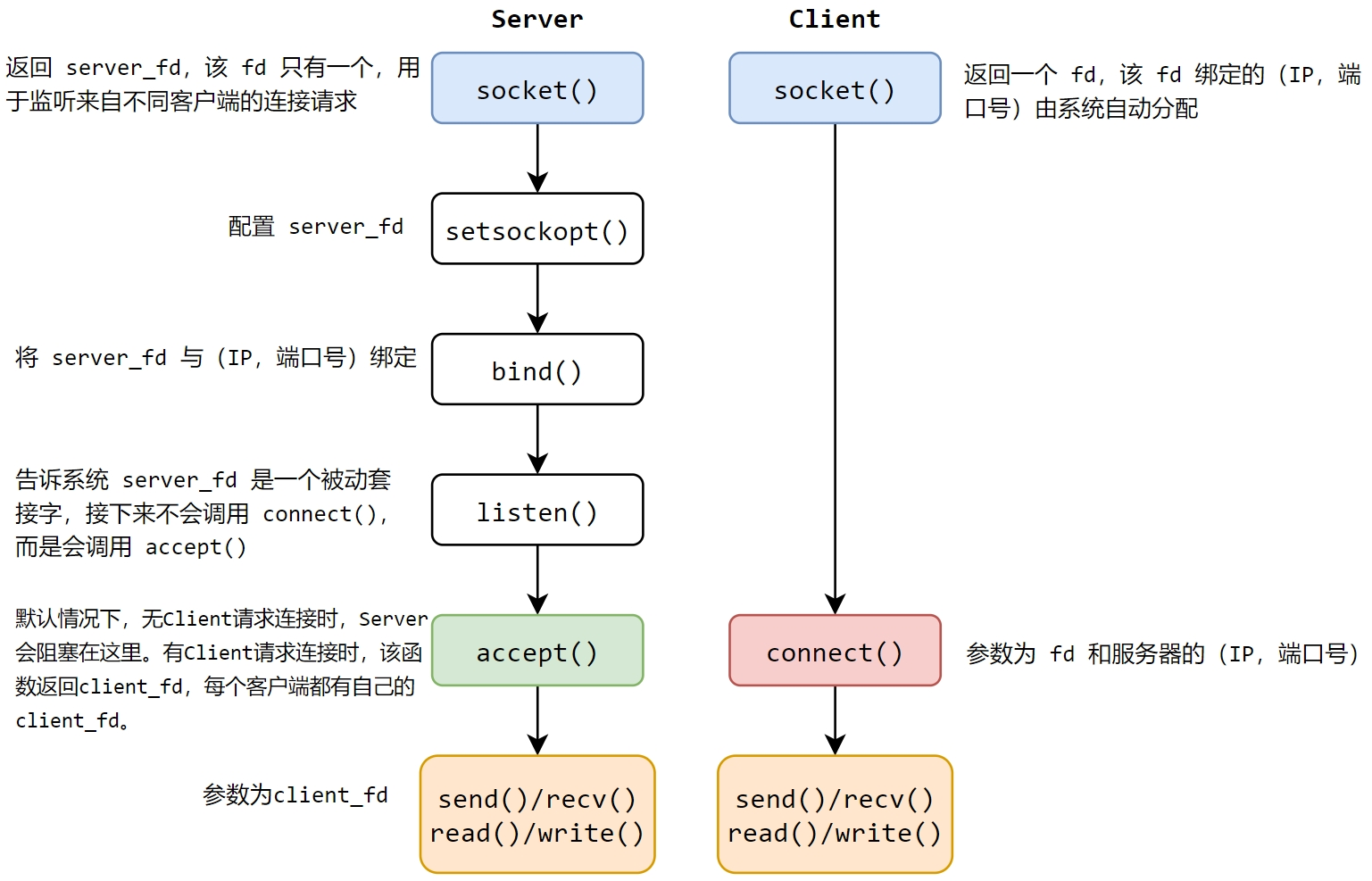
01. 时间相关函数
时间概念:
英文名 | 缩写 | 翻译 | 解释 |
---|---|---|---|
Greenwich Mean Time | GMT | 格林威治时间 | 早期的世界标准时间( 等价于 UTC+0 ) |
Coordinated Universal Time | UTC | 协调世界时 | 现行的世界标准时间( 本初子午线、0经度线 ) |
Local Time | 本地时间 | UTC+时区(东正西负) | |
China Standard Time | CST | 中国标准时间 | UTC+8 |
英文名 | 直翻 | 解释 | 类型 |
---|---|---|---|
Calender Time | 日历时间 | Unix时间戳 | time_t |
Broken-down Time | 分解时间 | 人类可读时间 | struct tm |
头文件:
#include <time.h>
结构体:
struct tm //Broken-down time 的结构体定义(人类可读时间)
{
int tm_sec; /* Seconds (0-60) */
int tm_min; /* Minutes (0-59) */
int tm_hour; /* Hours (0-23) */
int tm_mday; /* Day of the month (1-31) */
int tm_mon; /* Month (0-11) */
int tm_year; /* Year - 1900 */
int tm_wday; /* Day of the week (0-6, Sunday = 0) */
int tm_yday; /* Day in the year (0-365, 1 Jan = 0) */
int tm_isdst; /* Daylight saving time */
};
01.01 asctime()
函数原型:
char *asctime(const struct tm *tm);
char *asctime_r(const struct tm *tm, char *buf);
功能:将分解时间转换为字符串形式的人类可读时间。
01.02 ctime()
函数原型:
char *ctime(const time_t *timep);
char *ctime_r(const time_t *timep, char *buf);
功能:将Unix时间戳转换为字符串形式的人类可读时间。
01.03 gmtime()
函数原型:
struct tm *gmtime(const time_t *timep);
struct tm *gmtime_r(const time_t *timep, struct tm *result);
功能:将Unix时间戳转换为分解时间。
01.04 localtime()
函数原型:
struct tm *localtime(const time_t *timep);
struct tm *localtime_r(const time_t *timep, struct tm *result);
功能:将Unix时间戳转换为已经加上本地时区信息的分解时间。
01.05 mktime()
函数原型:
time_t mktime(struct tm *tm);
功能:将分解时间转换为Unix时间戳。
01. dlopen()
头文件:#include <dlfcn.h>
函数原型:dynamic load open
void *dlopen(const char *filename, int flags);
功能:在运行时加载 filename
共享库(.so文件),flags
是控制加载行为的标志,如 RTLD_LAZY
等。
返回:成功时返回一个非NULL
的、供 dlsym()
和 dlclose()
调用的句柄,失败时返回NULL
。
标志:参数中的 flags
为共享库加载标志,用于控制 dlopen()
加载共享库时符号解析(resolving symbols)的行为。RTLD_LAZY
延迟绑定、按需加载,使得共享库中的符号解析被推迟到它们真正被程序引用时才进行。RTLD_LAZY
减少程序的启动时间、内存占用。
举例:
char *error = NULL;
void *handle = dlopen("~/my_lib/libName.so", RTLD_LAZY);
if(!handle)
{
fprintf(stderr, "%s\n", dlerror());
}
dlerror();
... //业务代码
if((error = dlerror()) != NULL)
{
fprintf(stderr, "%s\n", error);
}
dlclose(handle);
02. dlsym()
头文件:#include <dlfcn.h>
函数原型:dynamic link symbol
void *dlsym(void *handle, const char *symbol);
功能:从共享库中获取函数或变量的地址,handle
是通过 dlopen()
得到的共享库的句柄,symbol
是要查找的函数或变量的名称。
返回:成功返回符号地址,失败返回 NULL
。
03. fgets()
功能:从指定的文件流 stream 中读取一行字符串,存储到字符数组 s 中,size 表示最多读取的字符数(包括字符串终止符 \0)。
返回:返回指向 *s 的指针,但是参数中 s 已经是指针,能够获取读到的字符串,因此返回值没啥L用。
说明:fgets()从 stream 指定的流中读取字符,直至遇到以下三种情况时停止读取:
① 换行符 \n
② 文件结束符 EOF
③ 读取了 size - 1 个字符时,因为最后一个位置要存储字符串结束标志 \0
04. getline()
浏览器搜索该函数,给出的用法大多都是C++的,因此搁这儿记录一下getline()的C语言用法。首先给出man 3 getline
的结果,如上图,确认该函数确实也是C语言中的一个标准库函数,
功能:将 stream 中的一行内容读到大小为 *n 字节、由 *lineptr 指向的内存区域中。
返回:成功时返回读到的字符数,不包括字符串结束标志 \0。失败时返回 -1。
注意:① *lineptr 指向一个动态分配的内存区域(由malloc,calloc或realloc分配的,不能是静态分配的数组)。
② 注意内存在使用完成后,手动开辟,手动释放:free(lineptr);
05. issapce()
函数原型:
int isspace(int c)
功能:用于检查一个字符 c 是否是空白字符 white-space characters。
说明:该函数接受一个整数作为参数,这个整数通常是字符的ASCII值。空白字符包括:①空格 space ②换页符 form-feed \f(没用过)③换行符 newline \n ④回车符 carriage return \r ⑤水平制表符 horizontal tab \t ⑥垂直制表符 vertical tab \v(没用过)。
返回值:属于空白字符时返回非零(通常是1),不是空白字符返回0。
06. memcpy()
头文件和函数原型:
#include <string.h>
void *memcpy(void *dest, const void *src, size_t n);
功能:从内存区域src
复制n
字节到内存区域dest
。
07. memmove()
头文件和函数原型:
#include <string.h>
void *memmove(void *dest, const void *src, size_t n);
功能:从内存区域src
复制n
字节到内存区域dest
。
注意:memmove()
可以处理源内存区域和目标内存区域重叠的情况,而memcpy()
则不能
08. memset()
头文件和函数原型:
#include <string.h>
void *memset(void *s, int c, size_t n);
功能:将s
指向的内存区域的前n
个字节设置为c
的值。
常用于赋值前清空字符串:
char str[20];
memset(str, 0, sizeof(str));
09. popen()
功能:创建一个连接到子进程的管道。
10. pthread_setname_np()
(同 “一、3. prctl()
” )
头文件和函数原型:
#define _GNU_SOURCE
#include <pthread.h>
int pthread_setname_np(pthread_t thread, const char *name);
int pthread_getname_np(pthread_t thread, char *name, size_t len);
Conpile and link with -pthread
11. remove()
头文件和函数原型:
#include <stdio.h>
int remove(const char *pathname);
功能:删除文件或目录。
12. snprintf()
头文件和函数原型:
#include <stdio.h>
int snprintf(char *str, size_t size, const char *format, ...);
功能:向str
中打印字符,最多打印size
个(字符串终止符\0
也在计数范围内)。
13. strcat()
头文件和函数原型:
#include <string.h>
char *strcat(cahr *dest, const char *src);
功能:将字符串src
追加到字符串dest
末尾。
注意:要满足sizeof(dest) - strlen(dest) - 1 ≥ strlen(src)
(那个1
指字符串终止符),否则会产生内存溢出。
14. strcmp()
功能:比较 s1 和 s2 两个字符串的字典序大小。按照ASCII码的顺序逐字符比较两个字符串,直到遇到不同的字符或者到达字符串的末尾(遇到空字符\0)。
返回:strcmp()函数的返回值有以下三种情况:
①如果返回值小于0:则表示 s1 小于 s2 ,即在字典顺序上 s1 位于 s2 之前。
②如果返回值大于0:则表示 s1 大于 s2 ,即在字典顺序上 s1 位于 s2 之后。
③如果返回值等于0:则表示 s1 等于 s2 ,即两个字符串完全相同。
15. strlen()
头文件和函数原型:
#include <string.h>
size_t strlen(const char *s);
功能:计算字符串s
的长度(unsigned int型),不计入字符串终止符\0
(terminating null byte)。
16. strncat()
头文件和函数原型:
#include <string.h>
char *strncat(cahr *dest, const char *src, size_t n);
功能:将源字符串src
追加到目的字符串dest
的末尾。指定一个最大的追加字符数n
,以防止目的字符串dest
溢出。
17. strncpy()
功能:从 src 赋值 n 个字符到 dest
注意:strncpy() 没有自动加字符串终止符 \0,注意手动加上
18. strstr()
头文件和函数原型:
#include <string.h>
char *strstr(const char *haystack, const char *needle);
功能:在字符串haystack
中查找子串needle
。
字面:在草垛(haystack)中找缝衣针(needle)。
返回:如果找到了,则返回子串第一次出现的位置;如果没找到则返回NULL。
举例:
#include <stdio.h>
#include <string.h>
int main()
{
const char *haystack = "Hello, World!";
const char *needle = "World";
char *result = strstr(haystack, needle);
if (result != NULL)
{
printf("Found '%s' at position %ld\n", needle, result - haystack);
}
else
{
printf("'%s' not found\n", needle);
}
return 0;
}
运行结果:
Found 'World' at position 7