项目需求:抄表数可输入小数点后4位
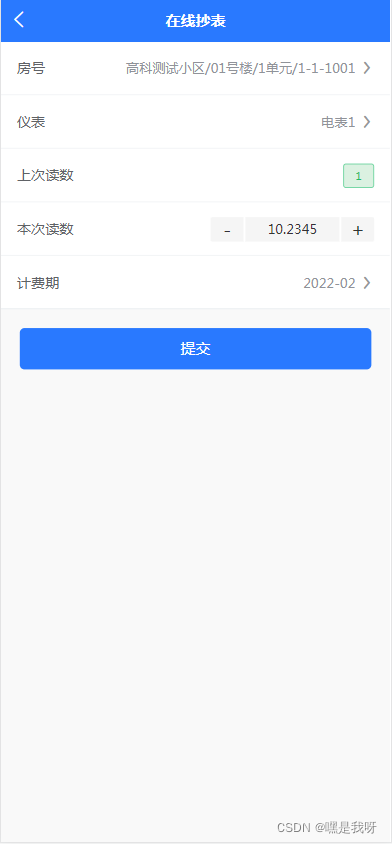
修改uniapp中uni-number-box.vue文件,如下:
<template>
<view class="uni-numbox">
<view @click="_calcValue('minus')" class="uni-numbox__minus uni-numbox-btns" :style="{background}">
<text class="uni-numbox--text" :class="{ 'uni-numbox--disabled': inputValue <= min || disabled }">-</text>
</view>
<input :disabled="disabled" @input="onKeyInput" @blur="_onBlur" class="uni-numbox__value" type="number"
v-model="inputValue" />
<view @click="_calcValue('plus')" class="uni-numbox__plus uni-numbox-btns" :style="{background}">
<text class="uni-numbox--text" :class="{ 'uni-numbox--disabled': inputValue >= max || disabled }">+</text>
</view>
</view>
</template>
<script>
/**
* NumberBox 数字输入框
* @description 带加减按钮的数字输入框
* @tutorial https://ext.dcloud.net.cn/plugin?id=31
* @property {Number} value 输入框当前值
* @property {Number} min 最小值
* @property {Number} max 最大值
* @property {Number} step 每次点击改变的间隔大小
* @property {String} background 背景色
* @property {Boolean} disabled = [true|false] 是否为禁用状态
* @event {Function} change 输入框值改变时触发的事件,参数为输入框当前的 value
*/
export default {
name: "UniNumberBox",
emits: ['change'],
props: {
value: {
type: [Number, String],
default: 1
},
min: {
type: Number,
default: 0
},
max: {
type: Number,
default: 100
},
step: {
type: Number,
default: 1
},
background: {
type: String,
default: '#f5f5f5'
},
disabled: {
type: Boolean,
default: false
}
},
data() {
return {
inputValue: 0
};
},
watch: {
inputValue(newVal, oldVal) {
if (+newVal !== +oldVal) {
this.$emit("change", newVal);
}
}
},
created() {
this.inputValue = +this.value;
},
methods: {
//输入框实时获取输入内容
onKeyInput: function(event) {
this.vlues = event.target.value;
},
//加、减操作(minus:减;plus:加)
_calcValue(type) {
if (this.disabled) {
return;
}
const scale = this._getDecimalScale();
let value = this.inputValue * scale;
let step = this.step * scale;
if (type === "minus") {
value -= step;
if (value < (this.min * scale)) {
return;
}
if (value > (this.max * scale)) {
value = this.max * scale
}
} else if (type === "plus") {
value += step;
if (value > (this.max * scale)) {
return;
}
if (value < (this.min * scale)) {
value = this.min * scale
}
}
if ((value + '').length > 5) {
value = value.toFixed(4); //toFixed保留小数点位数方法
}
this.inputValue = String(value / scale);
},
_getDecimalScale() {
let scale = 1;
// 浮点型
if (~~this.step !== this.step) {
scale = Math.pow(10, (this.step + "").split(".")[1].length);
}
return scale;
},
_onBlur(event) {
let value = event.detail.value;
if (!value) {
return;
}
value = +value;
if (value > this.max) {
value = this.max;
} else if (value < this.min) {
value = this.min;
}
this.inputValue = value;
/*小数点后保留四位*/
if (value > 0) {
event.target.value = event.target.value.match(/\d+(\.\d{0,4})?/)[0];
} else {
event.target.value = -event.target.value.match(/\d+(\.\d{0,4})?/)[0];
}
//重新赋值给input
this.$nextTick(() => {
this.inputValue = event.target.value;
})
}
}
};
</script>
<style lang="scss" scoped>
$box-height: 26px;
$bg:
$br: 2px;
$color:
.uni-numbox {
/*
display: flex;
/*
flex-direction: row;
}
.uni-numbox-btns {
/*
display: flex;
/*
flex-direction: row;
align-items: center;
justify-content: center;
padding: 0 8px;
background-color: $bg;
/*
cursor: pointer;
/*
}
.uni-numbox__value {
margin: 0 2px;
background-color: $bg;
width: 100px;
height: $box-height;
text-align: center;
font-size: 14px;
border-left-width: 0;
border-right-width: 0;
color: $color;
}
.uni-numbox__minus {
border-top-left-radius: $br;
border-bottom-left-radius: $br;
width: 64rpx;
}
.uni-numbox__plus {
border-top-right-radius: $br;
border-bottom-right-radius: $br;
width: 64rpx;
}
.uni-numbox--text {
// fix nvue
line-height: 20px;
font-size: 20px;
font-weight: 300;
color: $color;
}
.uni-numbox .uni-numbox--disabled {
color:
/*
cursor: not-allowed;
/*
}
</style>
调用组件:
<template>
<view>
<u-cell-group>
<u-cell-item :arrow="false" title="本次读数">
<uni-number-box :value="meterValue" :min="0" :max="99999999999999" @change="changeScore" slot="right-icon"></uni-number-box>
</u-cell-item>
</u-cell-group>
</view>
</template>
<script>
import uniNumberBox from "@/components/uni-number-box/uni-number-box.vue"
export default {
components: {
uniNumberBox
},
data() {
return {
meterValue: 0
};
},
methods: {
//本次读数回调
changeScore(e) {
console.log(e)
this.meterValue = e;
}
}
}
</script>
<style lang="scss" scoped>
</style>