目录
- 1.1变量
- 1.2基本数据类型
- 1.3运算符优先级
- 1.4关系运算
- 1.5判断
- 1.6判断
- 1.7 for循环
- 1.8 逻辑运算
- 2 数组
- 2.1 二维数组
- 3 字符类型
- 3.1字符计算
- 3.2逃逸字符
- 3.3包裹类型
- 3.4字符串变量
- 4 函数
- 4.1本地变量的规则
- 5 对象
- 5.1用类创建对象
- 5.2定义类
- 5.3成员变量与函数
- 5.4封闭的访问类型
- 5.5开发的访问类型
- 5.6 包
- 5.7 类变量和类函数
- 5.8 记事本
- 5.9 父类子类
- 5.10 多态变量
- 5.11 向上造型
- 5.12 多态
- 5.13 Object类
- 5.14 城堡小游戏
- 5.15 框架和数据
- 5.16 抽象
- 5.17 细胞自动机
- 5.18 数据与表现分离
- 5.19 狐狸和兔子
- 5.20 接口
- 5.21 布局管理器
- 5.22 控制反转
- 5.23 内部类
- 5.24 匿名类
- 6.1 异常
- 6.2 捕获异常
- 6.3 异常抛出和异常
- 6.4 异常捕获时的匹配
- 6.5异常遇到继承
- 6.6 流
- 6.7 格式化输入输出
1.1变量
定义变量<类型><变量名>,关键字final常量。
1.2基本数据类型
int,整数类型。float,浮点类型。
1.3运算符优先级
1.4关系运算
- ==:相等
- !=:不相等
-
:大于
-
=大于或等于
- <:小于
- <=小于或等于
优先级:和!=优先级比其他低,而连续的关系运算是从左到右进行的
5>36>4
判断两个浮点数。
System.out.println(Math.abs(a-b)<1e-6);
1.5判断
while(){
}
package com.java;
import java.util.Scanner;
public class Helloworld {
public static void main(String[] args){
Scanner in =new Scanner(System.in);
int number;
number=in.nextInt();
int result=0;
while (number>0){
int digit =number%10;
result=result*10+digit;
System.out.println(digit);
number=number/10;
}
System.out.println(result);
}
}
do
{}while();
1.6判断
if-else;
switch-case(控制表达式){
case 常量:
语句
break;
default:
语句
}
控制表达式只能是整数型结果
1.7 for循环
for(初始化,条件,单步动作)
阶乘
import java.util.Scanner;
public class Helloworld {
public static void main(String[] args){
Scanner in =new Scanner(System.in);
int number=in.nextInt();
int factors=1;
for(int i=1;i<=number;i++) {
factors*=i;
}
System.out.println(factors);
}
}
素数,只能被1或自己整除的数,不包括1.
break,continue
可以在循环钱放一个标号来表示这个循环。
import java.util.Scanner;
public class Helloworld {
public static void main(String[] args){
Scanner in =new Scanner(System.in);
int n=in.nextInt();
int isPrime=1;
OUT://标号
for(int i=2;i<n;i++)
{
if(n%i==0)
{
isPrime=0;
break OUT;//跳出for循环
continue;跳过循环这轮语句进入下一轮
}
}
if (isPrime==1)
{
System.out.println("是素数");
}
else
{
System.out.println("不是素数");
}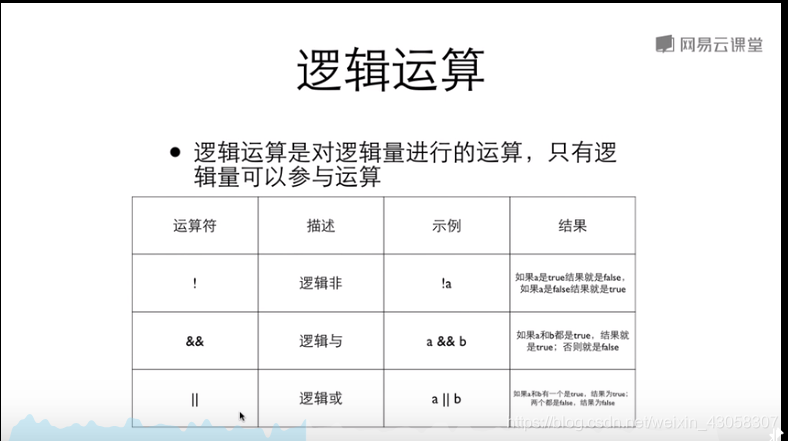
}
}
1.8 逻辑运算
boolean
bool类型,为true or false;
2 数组
是一种容器也是一种数据结构,其中所有的元素具有相同的数据类型,一旦创建不能改变数组大小。
定义数组变量
<类型>[]<名称>= new <类型>[元素个数];
int[] grades =new int[100];
- 数组变量是数组的管理者而非数组本身
- 数组必须创建出来然后交给数组变量管理
- 数组变量直接的赋值是管理权限的赋予
- 数组变量之间的比较是判断是否管理同一个数组
复制数组
必须遍历数组。
搜索
for-each循环适用于数组
for(int k :data)//对于data中每个元素取出赋给k
{}
2.1 二维数组
int [][] a= new int[3][5]//3行5列
初始化
int [][] a ={
{1,2,3,4},
{1,2,3},
};
3 字符类型
单个字符是一种特殊的类型:char
用单引号表示字符字面量:‘a’,‘1’,
3.1字符计算
字符比较:a>A
3.2逃逸字符
制表位:
每行的固定位置,一个\t使得输出从下一个制表位开始,用\t才能使得上下两行对齐。
3.3包裹类型
基础类型 | 包裹类型 |
---|---|
boolean | Boolean |
char | Character |
int | Integer |
double | Double |
包裹类型.isDigit判断是否数字,isLowerCase
Math类
Math.abs()求绝对值。Math.round()取整。random()随机数,pow(底数,指数)求次方
3.4字符串变量
String 是一个类,String的变量是对象的管理者并非拥有者。就像数组一样。
比较两个字符串使用.equals方法。使用==来比较两个对象是否是同一个。
.compareTo()比较大小。访问String里面的字符。s.charAt(index).index范围为0-length()-1
.substring(start,end)取出start到end-1之间的字符。s.indexOf©得到c字符所在的位置。
s.indexof(c,n)从n号位置来寻找c字符。s.lastIndexOf©从右边开始找。
对String的操作不能修改其本身,只能是创建一个新的字符串对其操作。
4 函数
public static void(范围类型) sum‘函数名’()
{
函数内容
}
必须传递函数所需的参数和正确参数类型。
宽类型可以接受窄类型。char->int->double。
函数参数传递的是值。
4.1本地变量的规则
- 本地变量是定义在块内的
- 可以定义在函数块内或者是语句块内。甚至是随意大括号来定义
- 程序进入这个块之前,变量不存在,离开这个块。变量也消失了。
- 在块外面定义的变量在块内也可以使用。
5 对象
- 对象(这只猫)
- 表达东西或者事件
- 运行时响应消息(提供服务)
- 类(所有猫)
- 定义所有猫的属性
- 就是Java中的类型
- 可以用来定义变量
5.1用类创建对象
对象=属性+服务
所谓封装就是把数据和对数据的操作放在一起
5.2定义类
以自动售货机为例。
属性:price balance,total
方法:showprompt.insertMoney,getFood
5.3成员变量与函数
在函数中可以直接写成员变量的名字来访问成员变量。
this
是成员函数的一个特殊的固有的本地变量,它表达了调用这个函数的那个对象。
通过.运算符调用某个对象的函数,在成员函数内部调用直接的其他函数。
本地变量
定义在函数内部的变量是本地变量。
本地变量的生存期和作用于都是在函数内部
成员变量的生存期是对象的生存期,作用域是类内部的成员函数。
构造函数
如果有一个成员函数的名字和类的名字完全相同,则在创建这个类的每一个对象的时候会自动调用这个函数->构造函数。这个函数不能有返回类型。
重载
- 一个类可以有多个构造函数,只要他们的参数表不同。
- 创建对象的时候给出不同的参数值,就会自动调用不同的构造函数。
- 通过this()还可以调用其他构造函数
- 一个类里的同名单参数表不同的函数构成了重载关系。
5.4封闭的访问类型
private
只有这个类内部可以访问
类内部指类的成员函数和定义初始化
这个限制是对类的而不是对对象。换句话说,同一个类的对象可以互相访问私有属性。
5.5开发的访问类型
任何人都可以访问
任何人指的是在任何类的函数或定义初始化中可以使用。使用是指调用,访问或定义变量。
5.6 包
package
相当于一个文件夹。假设包里面的类里面的方法或者属性没有加上Public属性,则不能被其他包所访问。只能被属于同一个包的类访问。
引用其他包的类:import 包.类or*
5.7 类变量和类函数
private static int step =1;
类拥有类变量但是对象并没有这个类变量。
类拥有类函数,但是对象并没有这个类函数。
5.8 记事本
实现的功能:储存记录,不限制记录的数量,知道已经储存的数量,能查看每一条数据,能删数据,列出所有数据。
接口设计:add(String node) getSize();getNote(int index): removeNote(int index);list();
容器类
满足add方法不断增加数组。
ArrayList notes=new ArrayList;
容器类有两个类型:容器类型和元素的类型。
对象数组
对象数组中的每个元素都是对象的管理者而非对象本身。
集合容器
HashSet<String> s=new HashSet<String>();
s.add("first");
s.add("second");
s.add("first");
for (String k:s)
{
System.out.println(k);
}
输出
first
second
集合中没有重复的元素。
HASH表
private HashMap<Integer,String> coinnames=new HashMap<Integer,String>();
5.9 父类子类
继承
public class DVD extends Item{//从Item派生出的子类
private String title;
private String artist;
private int playingTime;
private boolean gotIt=false;
private String comment;
public DVD(String title, String artist, int playingTime, String comment) {
super(title);
this.title = title;
this.artist = artist;
this.playingTime = playingTime;
this.comment = comment;
}
public static void main(String[] args)
{
}
//注释掉print()后任然可以输出
//public void print() {
//System.out.println(title+":"+artist);
//}
}
public class Item {
protected String title;
public Item(String title) {
this.title = title;
}
}
import java.util.ArrayList;
public class Database {
private ArrayList<Item> listItem=new ArrayList<Item>();
public void add(Item item)
{
listItem.add(item);
}
public void list()
{
for (Item item:listItem)
{
item.print();
}
}
public static void main(String[] args)
{
Database db=new Database();
db.add(new CD("abc", "efg",4, 60, "..."));
db.add(new CD("abced", "efg11111111",14, 160, "...."));
db.add(new DVD("abc", "efg11111111", 160, "...."));
db.list();
}
}
子类继承了所有父类的东西.
子类初始化时,先进行父类初始化,然后再进行子类初始化.
super(title);子类初始化时,调用父类的构造器将title传递给父类进行初始化。
private String title;
private String artist;
public DVD(String title, String artist, int playingTime, String comment) {
super(title,playingTime,false,comment);
this.artist = artist;
this.title=title;
}
public static void main(String[] args)
{
DVD dvd =new DVD("a","b",1,"..");
}
如果子类和父类具有相同的东西,会出现两个相同的。但是两者并不相互影响。
例如具有相同的title,由于先进行父类初始化,此时title的到的a是父类的。而this.title子类的为null。
5.10 多态变量
子类和子类型
- 类定义了类型
- 子类定义了子类型
- 子类的对象可以被当做父类的对象来使用
- 赋值给父类的变量
- 传递给需要父类对象的函数
- 放进存放父类的容器里
子类型与赋值
子类的对象可以赋值给父类的变量。
Vehicle v1 =new Vehicle();
Vehicle v2 =new Car();
Vehicle v3 =new Bicycle();
子类和参数传递
public class Database
{
public void add(Item item)
{
}
}
DVD dvd=new DVD()
database.add(dvd);
子类型和容器
多态变量
- Java的对象变量是多态的,它们能保存不止一种类型的对象,
- 它们可以保存的是声明类型的对象。或声明类型的子类的对象。
- 当把子类的对象赋给父类的变量的时候,就发生了向上造型。
5.11 向上造型
造型cast
子类的对象可以赋值给父类的变量
Java不存在对象对对象的赋值!!
父类的对象不能赋值给子类的变量!
Vechicle v;
Car c = new Car();
v=c;//可以
c=v;//编译错误
可以用造型
c=(Car) v;
(只有当V这个变量实际管理的是Car才行)
造型
- 用括号围起来类型放在值的签名
- 对象本身并没有发生任何变化
所以不是“类型转换”
运行时有机制来检查这样的转换是否合理
向上造型
- 拿一个子类的对象,当做父类的对象来用。
- 向上造型是默认的,不需要运算符。
- 向上造型总是安全的。
5.12 多态
for (Item item:listItem)
{
item.print();
}
item根据存放类型,调用不同的print()。
一种是声明类型item,一种是实际存放的类型(DVD,CD).故Item是多态的。
函数的绑定
- 当通过对象变量调用函数的时候,调用哪个函数这件事情叫做绑定
- 静态绑定:根据变量的声明类型来决定
- 动态绑定:根据变量的动态类型来决定
- 在成员函数中调用其他成员函数也是通过this这个对象变量来调用的。
覆盖voerride
- 子类和父类中存在名称和参数表完全相同的函数,这一对函数构成覆盖关系
- 通过父类的变量调用存在覆盖关系的函数时,会调用变量当时所管理的对象所属的类的函数。
5.13 Object类
Object函数
toString(),equals()
重写object类的equals()函数
public boolean equals(Object obj){
CD cc = (CD)obj;//向下造型
return artist.equals(cc.artist);
}
5.14 城堡小游戏
GAME.java
package castle;
import java.util.*;
public class Game {
private Room currentRoom;
public Game()
{
creatRooms();
}
private void creatRooms()
{
Room outside, lobby,pub,study,bedroom;
// 制造房间
outside = new Room("城堡外");
lobby = new Room("大堂");
pub = new Room("小酒吧");
study = new Room("书房");
bedroom = new Room("卧室");
// 初始化房间的出口
outside.setExits(null,lobby,study,pub);
lobby.setExits(null,null,null,outside);
pub.setExits(outside,bedroom,null,null);
bedroom.setExits(null,null,null,study);
currentRoom = outside; //从城堡门外开始
}
private void printWelcome()
{
System.out.println();
System.out.println("欢迎来到城堡!");
System.out.println("这是一个超级无聊的游戏。");
System.out.println("如果需要帮助,请输入'help'");
System.out.println();
System.out.println("现在你在:" + currentRoom);
System.out.println("欢迎来到城堡");
System.out.println("出口有:");
if(currentRoom.northExit !=null)
System.out.print("north");
if(currentRoom.eastExit !=null)
System.out.print("east");
if(currentRoom.southExit !=null)
System.out.print("south");
if(currentRoom.westExit !=null)
System.out.print("west");
System.out.println();
}
// 以下为用户命令
private void printHelp()
{
System.out.println("迷路了吗?你可以做的命令有:go bye help");
System.out.println("如:\tgo east");
}
private void goRoom(String direction)
{
Room nextRoom = null;
if(direction.equals("north")){
nextRoom = currentRoom.northExit;
}
if(direction.equals("east")){
nextRoom = currentRoom.eastExit;
}
if(direction.equals("south")){
nextRoom = currentRoom.southExit;
}
if(direction.equals("west")){
nextRoom = currentRoom.westExit;
}
if(nextRoom == null){
System.out.println("那里没有门!");
}
else{
currentRoom = nextRoom;
System.out.println("你在"+ currentRoom);
System.out.println("出口有:");
if(currentRoom.northExit != null)
System.out.println("norh");
if(currentRoom.eastExit != null)
System.out.println("east");
if(currentRoom.southExit != null)
System.out.println("south");
if(currentRoom.westExit != null)
System.out.println("west");
System.out.println();
}
}
public static void main(String[] args) {
Scanner in = new Scanner(System.in);
Game game = new Game();
game.printWelcome();
while (true){
String line = in.nextLine();
String[] words = line.split(" ");
if ( words[0].equals("help")){
game.printHelp();
}else if ( words[0].equals("go")){
game.goRoom(words[1]);
}else if ( words[0].equals("bye")){
break;
}
}
System.out.println("感谢您的光临。再见!");
in.close();
}
}
ROOM.java
package castle;
public class Room {
public String description;
public Room northExit;
public Room southExit;
public Room eastExit;
public Room westExit;
public Room(String description)
{
this.description = description;
}
public void setExits(Room north,Room east,Room south,Room west)
{
if(north != null)
northExit = north;
if(east != null)
eastExit = east;
if(south != null)
southExit = east;
if(west != null)
westExit = west;
}
@Override
public String toString()
{
return description;
}
public void southExit(Object object, Room lobby, Room study, Room pub) {
// TODO Auto-generated method stub
}
}
消除代码复制
在GAME类中重复出现了以下代码。
System.out.println("你在"+ currentRoom);
System.out.println("出口有:");
if(currentRoom.northExit != null)
System.out.println("norh");
if(currentRoom.eastExit != null)
System.out.println("east");
if(currentRoom.southExit != null)
System.out.println("south");
if(currentRoom.westExit != null)
System.out.println("west");
System.out.println();
可将以上代码转为一个函数。
public void showPrompt(){
System.out.println("你在"+ currentRoom);
System.out.println("出口有:");
if(currentRoom.northExit != null)
System.out.println("norh");
if(currentRoom.eastExit != null)
System.out.println("east");
if(currentRoom.southExit != null)
System.out.println("south");
if(currentRoom.westExit != null)
System.out.println("west");
System.out.println();
}
程序扩展性
如果要增加一个方向,例如down或up
- 使用容器来实现灵活性,Room的方向是用成员变量表示的,增加或减少方向就要改变代码。
- 用Hash表来表示方向,方向就不是硬编码。
用封装来降低耦合
- 类和类之间的关系称作耦合
- Room类和Game类有大量的代码和出口相关
- 尤其是Game类中使用了Room类的成员变量
- 耦合越低越好,保持距离是形成良好代码的关键
public String getExitDesc()
{
StringBuffer sb=new StringBuffer();
if (northExit!=null)
sb.append("north");
if (eastExit!=null)
sb.append("east");
if (westExit!=null)
sb.append("west");
if (southExit!=null)
sb.append("south");
return sb.toString();
}
public Room getExit(String direction)
{
Room ret =null;
if(direction.equals("north")){
ret = northExit;
}
if(direction.equals("east")){
ret = eastExit;
}
if(direction.equals("south")){
ret = southExit;
}
if(direction.equals("west")){
ret = westExit;
}
return ret;
}
将game类中的代码封装到Room类中,降低了两个类之间的耦合
5.15 框架和数据
以框架和数据来提供可扩展性
- 命令的解析是否可以脱离if-else
- 定义一个Handler来处理命令
- 用Hash表来保存命令和Handler之间的关系
5.16 抽象
public abstract class Shape{
public abstract void draw(Graphics a);
抽象函数:表达概念而无法实现具体代码的函数
抽象类:表达概念而无法构造出实体的类
- 带有abstract修饰符的函数
- 有抽象函数的类一定是抽象类
- 抽象类不能制造对象
- 但是可以定义变量
任何继承了抽象类的非抽象类的对象可以赋给这个变量
实现抽象函数
继承自抽象类的子类必须覆盖父类中的抽象函数,否则自己成为抽象类
public abstract class Shape{
public abstract void draw(Graphics a);
public abstract void move(Graphics a);
两种抽象
与具体相对,表示概念非实体
与细节相对,表示一定程度上忽略细节而着眼大局
5.17 细胞自动机
- 死亡:如果活着的邻居数量<2或>3,则死亡
- 新生:如果正好有3个邻居活着,则新生
- 其他情况保持原状
5.18 数据与表现分离
程序的业务逻辑与表现无关
表现可以是图形的也可以是文本的
表现可以是当地的也可以是远程的
View和Field的关系
- 表现与数据的关系
- View只根据Fieid画出图形
- Field只管数据的存放
- 一旦数据更新以后,通知View重新画出整个画面
- 不去精心设计哪个局部需要更新
- 简化了程序逻辑
- 在计算机运算速度提高的基础上实现的
责任驱动的设计
- 将程序要实现的功能分配到合适的类/对象中去是设计中非常重要的一环
网格化
- 图形界面本身具有更高的解析度
- 但是将画面网格化以后,数据就更容易处理了
5.19 狐狸和兔子
- 狐狸和兔子都有年龄
- 当年龄到了一定的上限就会自然死亡
- 狐狸可以随机决定在周围的兔子中吃掉一个
- 狐狸和兔子可以随机决定生一个小的,放在旁边的空的格子里
- 如果不吃也不生,狐狸和兔子可以随机决定向旁边的格子移一步。
**Cell类的地位很尴尬 **
- 在Cells程序中它表达了细胞
- 但是同时他也表达了放在网格中的一个格子
- Fox和Rabbit是否应该从Cell继承
5.20 接口
- 接口是纯抽象类
- 所有的成员函数都是抽象函数
- 所有的成员变量都是public static final
- 接口规定了长什么样,但是不管里面有什么
实现接口
- 类用extends,接口用implements
- 类可以实现很多接口
- 接口可以继承接口,但不能继承类
- 接口不能实现接口
面向接口的编程方式
设计程序时先定义接口,在实现类
任何需要在函数间传入传出的一定是接口而不是具体的类
5.21 布局管理器
5.22 控制反转
引用:https://www.iteye.com/blog/jinnianshilongnian-1413846
Ioc—Inversion of Control,即“控制反转”,不是什么技术,而是一种设计思想。在Java开发中,Ioc意味着将你设计好的对象交给容器控制,而不是传统的在你的对象内部直接控制。如何理解好Ioc呢?理解好Ioc的关键是要明确“谁控制谁,控制什么,为何是反转(有反转就应该有正转了),哪些方面反转了”,那我们来深入分析一下:
●谁控制谁,控制什么:传统Java SE程序设计,我们直接在对象内部通过new进行创建对象,是程序主动去创建依赖对象;而IoC是有专门一个容器来创建这些对象,即由Ioc容器来控制对象的创建;谁控制谁?当然是IoC 容器控制了对象;控制什么?那就是主要控制了外部资源获取(不只是对象包括比如文件等)。
●为何是反转,哪些方面反转了:有反转就有正转,传统应用程序是由我们自己在对象中主动控制去直接获取依赖对象,也就是正转;而反转则是由容器来帮忙创建及注入依赖对象;为何是反转?因为由容器帮我们查找及注入依赖对象,对象只是被动的接受依赖对象,所以是反转;哪些方面反转了?依赖对象的获取被反转了。
5.23 内部类
在类的内部实现的类
- 定义在别的类内部,函数内部的类
- 内部类能直接访问外部的全部资源
- 包括任何私有的成员
- 外部是函数时,只能访问那个函数里的final的变量
5.24 匿名类
- 在NEW对象的时候给出类的定义形成了匿名类
- 匿名类可以继承某类,也可以实现某接口
- Swing的消息机制广泛使用匿名类
注入反转
- 由按钮公布一个收听者接口和一对注册/注销函数
- 你的代码实现那个接口,将收听者对象注册在按钮上
- 一旦按钮被按下,就会反过来调用你收听者对象的某个函数。
6.1 异常
pulic class ArrayIndex{
public static void main(String[] args){
int[] a =new int[10];
int idx;
Scanner in =new Scanner(System.in);
idx =in.nextInt();
a[idx] = 10;
System.out.println("hello");
}
}
抛出异常。 ArrayIndexOutOfBoundsException
6.2 捕获异常
pulic class ArrayIndex{
public static void main(String[] args){
int[] a =new int[10];
int idx;
Scanner in =new Scanner(System.in);
idx =in.nextInt();
try{
a[idx] = 10;
System.out.println("hello");
} catch(ArrayIndexOutOfBoundsException e{//捕获异常
System.out.println("Caught");
}
}
}
捕捉到了异常做什么?
- 拿到异常对象之后
- String getMessage();
- String toString()
- void printStackTrace();
再度抛出异常
try{
}
catch (ArrayIndexOutOfBoundsException e){
System.out.println();
throw e; 抛出异常
6.3 异常抛出和异常
class OpenException extends Throwable{
}
pulic class ArrayIndex{
public static int open(){
return -1;
}
public static void readFile(){
if(open()==-1){
throw new OpenException();
}
public static void main(String[] args){
}
}
异常声明
-
如果你的函数可能抛出异常,就必须在函数头部加以声明
-
void f() throw TooBig
-
你可以声明并不会镇的抛出异常
-
如果你调用一个声明会抛出异常的函数,那么你必须:
- 把函数的调用放在try块中,并设置catch来捕捉所有可能抛出的异常;或声明自己会抛出无法处理的异常
什么可以抛
- 把函数的调用放在try块中,并设置catch来捕捉所有可能抛出的异常;或声明自己会抛出无法处理的异常
-
任何继承了Throwable类的对象
-
Exception类继承了Throwable
- throw new Exception();
- throw new Exception(“HELP”)
6.4 异常捕获时的匹配
- Is-A的关系
- 就是说,抛出子类异常会被捕捉父类的异常的catch给捉到
6.5异常遇到继承
- 当覆盖一个函数的时候,子类不能声明抛出比父类的版本更多的异常
- 在子类的构造函数中,必须声明父类可能抛出的全部异常。
6.6 流
流的基础类
- inputStream
- OutputStream
InputStream
read():int read(),read(byte b[]),read(byte[],int off,int len) 读字节。
skip(long n)
int available()
mark()
reset()
boolean
markSupported()
close()
流过滤器
以一个介质流对象为基础层层构建过滤器流,最终形成的流对象能在数据的输入输出过程中,逐层使用过滤器流的方法来读写数据。
Data
- DataInputStream
- DataOutputStream
- 用以读写二进制方式表达的基本数据类型的数据
文件流
- FileInputStream
- FileOutputStream
- 对文件作读写操作
- 实际少使用
-常用的是以在内存数据或通信数据上建立的流,如数据库的二进制数据读写或网络端口通信
更具体的文件读写往往有更专业的类,例如配置文件和日志文件。
System.out.println("hello wrold");
byte[] buf =new byte[10]
for(int i=0;i<buf.length;i++)
{buf[i]=(byte)i}
try{
FileOutputStream out =new FileOutputStream("a.dat");
out.write(buf);
out.close();
}catch(FileNotFoundException e){
e.printStackTrace();
}catch(IoException e){
e.printStackTrace();
}
文本流
Writer
PrintWriter pw =new PrintWriter(
new BufferedWriter(
new OutputStreamWriter(
new FileOutputStream(“abc.txt”)));
Reader
- 常用的是BufferedReader
- readLine()
LineNumberReader
- 可以得到行号
- getLineNumber();
FileReader
- InputSteamReader类的子类,所有方法都从父类中继承而来
- FileReader(File file)
- 在给定从中读取数据的File的情况下创建一个新的FileReader.
- FileReader(String fileName)
- 在给定从左读取数据的文件名的情况下创建一个新FileReader,FileReader不能指定编码装换方式。
汉子编码
- InputStreamReader(InputSteam in)
- InputStreamReader(InputSteam in,Charset cs)使用给定字符集
- InputStreamReader(InputSteam in,CharsetDecoder dec)使用给定字符集解码器
- InputStreamReader(InputSteam in,String charseName)使用指定字符集
6.7 格式化输入输出
- format(“格式”,…);
- printf(“格式”,…);
- print(各种基本类型);
- println(各种基本类型);
Scanner
- 在InputStream或Reader上建立一个Scanner对象可以从流中的文本中解析出以文本表达的各种基本类型
- next()
根据数据选择类型
阻塞/非阻塞
- read()函数是阻塞的,在读到所需的内容之前会停下来等
- 使用read()的更高级的函数,如nextInt(),readLine()都是这样的
- 所以常用单独的线程来做socket读的等待,或使用nio的channe选择机制
- 对于socket,可以设置SO时间
- setSoTimeOut(int timeOut)
对象串行化
- ObjectInputStream类
- readObject()
- ObjectOutputStream类
- writeObject()
- Serializable接口