//1. 数据库认证
//1.1 配置认证管理器
package com.qf.authentication.config;
import org.springframework.context.annotation.Bean;
import org.springframework.security.authentication.dao.DaoAuthenticationProvider;
import org.springframework.security.config.annotation.authentication.builders.AuthenticationManagerBuilder;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.builders.WebSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
import org.springframework.security.crypto.bcrypt.BCryptPasswordEncoder;
import org.springframework.security.crypto.password.PasswordEncoder;
@EnableWebSecurity //启用security
public class SecurityConfig extends WebSecurityConfigurerAdapter {
//创建密码加密器,并纳入Spring IOC容器管理,该Bean的名字就是方法名
@Bean
public PasswordEncoder passwordEncoder(){
return new BCryptPasswordEncoder();
}
//认证管理器配置
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
//数据库数据认证提供器
DaoAuthenticationProvider daoAuthenticationProvider = new DaoAuthenticationProvider();
//设置认证使用的用户详情服务,业就是查询用户信息的服务
daoAuthenticationProvider.setUserDetailsService();
//设置密码使用的加密器
daoAuthenticationProvider.setPasswordEncoder(passwordEncoder());
//设置认证管理构建器使用的认证提供器
auth.authenticationProvider(daoAuthenticationProvider);
}
}
//1.2 创建查询用户信息服务
package com.qf.authentication.service;
import org.springframework.security.core.userdetails.UserDetailsService;
//用户业务层,继承了UserDetailsService,方便与security结合
public interface UserService extends UserDetailsService {}
//1.3
package com.qf.authentication.service.impl;
import com.qf.authentication.service.UserService;
import org.springframework.security.core.userdetails.UserDetails;
import org.springframework.security.core.userdetails.UsernameNotFoundException;
import org.springframework.stereotype.Service;
@Service
public class UserServiceImpl implements UserService {
@Override
public UserDetails loadUserByUsername(String username) throws UsernameNotFoundException {
return null;
}
}
//1.4 创建用户实体
package com.qf.authentication.model;
import lombok.Data;
import org.springframework.security.core.authority.SimpleGrantedAuthority;
import org.springframework.security.core.userdetails.UserDetails;
import java.util.List;
@Data
public class User implements UserDetails {
private String username; //账号
private String password; //密码
private List<SimpleGrantedAuthority> authorities; //拥有的权限
@Override
public boolean isAccountNonExpired() { //账号是否未过期
return true;
}
@Override
public boolean isAccountNonLocked() { //账号是否未被锁定
return true;
}
@Override
public boolean isCredentialsNonExpired() {//凭据是否未过期
return true;
}
@Override
public boolean isEnabled() {//账号是否可用
return true;
}
}
//1.5 完善用户信息服务
@Override
public UserDetails loadUserByUsername(String username) throws UsernameNotFoundException {
User user = new User();
user.setUsername("admin");
user.setPassword(passwordEncoder.encode("123456"));
user.setAuthorities(Arrays.asList(new SimpleGrantedAuthority("ROLE_ADMIN"), new SimpleGrantedAuthority("ROLE_USER")));
return user;
}
//1.6 完善认证管理器配置
//认证管理器配置
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
//数据库数据认证提供器
DaoAuthenticationProvider daoAuthenticationProvider = new DaoAuthenticationProvider();
//设置认证使用的用户详情服务,业就是查询用户信息的服务
daoAuthenticationProvider.setUserDetailsService(userService);
//设置密码使用的加密器
daoAuthenticationProvider.setPasswordEncoder(passwordEncoder());
//设置认证管理构建器使用的认证提供器
auth.authenticationProvider(daoAuthenticationProvider);
}
//1.7 HTTP认证配置
package com.qf.authentication.config;
import com.qf.authentication.service.UserService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.annotation.Bean;
import org.springframework.security.authentication.dao.DaoAuthenticationProvider;
import org.springframework.security.config.annotation.authentication.builders.AuthenticationManagerBuilder;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.builders.WebSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
import org.springframework.security.crypto.bcrypt.BCryptPasswordEncoder;
import org.springframework.security.crypto.password.PasswordEncoder;
@EnableWebSecurity //启用security
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Autowired
private UserService userService;
//创建密码加密器,并纳入Spring IOC容器管理,该Bean的名字就是方法名
@Bean
public PasswordEncoder passwordEncoder(){
return new BCryptPasswordEncoder();
}
//认证管理器配置
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
//数据库数据认证提供器
DaoAuthenticationProvider daoAuthenticationProvider = new DaoAuthenticationProvider();
//设置认证使用的用户详情服务,业就是查询用户信息的服务
daoAuthenticationProvider.setUserDetailsService(userService);
//设置密码使用的加密器
daoAuthenticationProvider.setPasswordEncoder(passwordEncoder());
//设置认证管理构建器使用的认证提供器
auth.authenticationProvider(daoAuthenticationProvider);
}
//Http认证配置
@Override
protected void configure(HttpSecurity http) throws Exception {
http.csrf().disable();//关闭跨站请求模拟
//设置表单登录使用的登录地址、登录请求的URL地址、登录成功和失败分别使用的处理器 permitAll表示该操作不需要security的权限控制
http.formLogin().loginPage("/").loginProcessingUrl("/login")
.successHandler().failureHandler().permitAll();
http.authorizeRequests().anyRequest().authenticated();
//设置退出操作使当前session失效 permitAll表示该操作不需要security的权限控制
http.logout().invalidateHttpSession(true).permitAll();
}
}
//1.8 创建认证处理器
package com.qf.authentication.handler;
import org.springframework.security.core.Authentication;
import org.springframework.security.web.authentication.AuthenticationSuccessHandler;
import org.springframework.stereotype.Component;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
//认证成功的处理器
@Component
public class LoginSuccessHandler implements AuthenticationSuccessHandler {
@Override
public void onAuthenticationSuccess(HttpServletRequest request, HttpServletResponse response, Authentication authentication) throws IOException, ServletException {
response.sendRedirect("/main.html");
}
}
package com.qf.authentication.handler;
import org.springframework.security.core.AuthenticationException;
import org.springframework.security.web.authentication.AuthenticationFailureHandler;
import org.springframework.stereotype.Component;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
//认证失败的处理器
@Component
public class LoginFailureHandler implements AuthenticationFailureHandler {
@Override
public void onAuthenticationFailure(HttpServletRequest request, HttpServletResponse response, AuthenticationException exception) throws IOException, ServletException {
response.sendRedirect("/");
}
}
//1.9 完善HTTP认证配置
//Http认证配置
@Override
protected void configure(HttpSecurity http) throws Exception {
http.csrf().disable();//关闭跨站请求模拟
//设置表单登录使用的登录地址、登录请求的URL地址、登录成功和失败分别使用的处理器 permitAll表示该操作不需要security的权限控制
http.formLogin().loginPage("/").loginProcessingUrl("/login")
.successHandler(loginSuccessHandler).failureHandler(loginFailureHandler).permitAll();
http.authorizeRequests().anyRequest().authenticated();
//设置退出操作使当前session失效 permitAll表示该操作不需要security的权限控制
http.logout().invalidateHttpSession(true).permitAll();
}
//1.10 页面创建
<!DOCTYPE html>
<html <span style="color:#0000cc">lang="en">
<head>
<meta <span style="color:#0000cc">charset="UTF-8">
<title>Security登录</title>
</head>
<body>
<form <span style="color:#0000cc">action="login" <span style="color:#0000cc">method="post">
<input <span style="color:#0000cc">type="text" <span style="color:#0000cc">name="username">
<input <span style="color:#0000cc">type="password" <span style="color:#0000cc">name="password">
<input <span style="color:#0000cc">type="submit" <span style="color:#0000cc">value="登录">
</form>
</body>
</html>
//main.html
<!DOCTYPE html>
<html <span style="color:#0000cc">lang="en">
<head>
<meta <span style="color:#0000cc">charset="UTF-8">
<title>Security登录成功</title>
</head>
<body>
认证通过了
</body>
</html>
spring security demo
最新推荐文章于 2024-07-09 12:51:24 发布
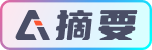