demo地址链接
效果演示
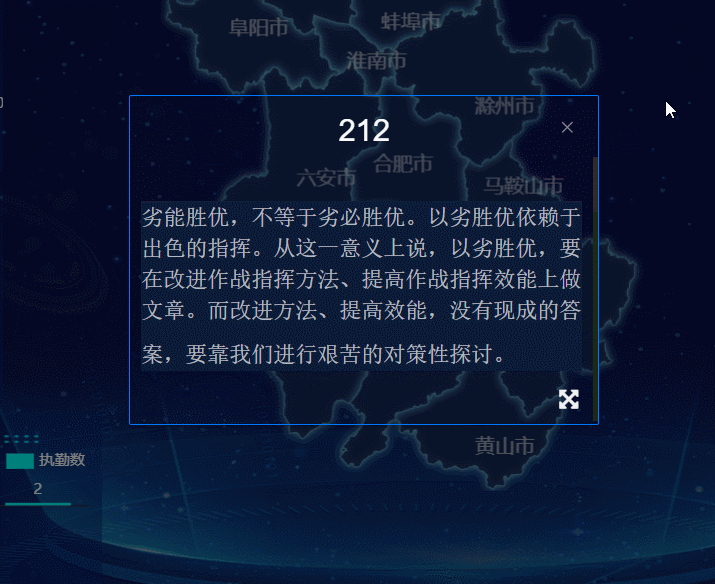
一、创建一个dialog.js文件,并在main.js中进行引入(dialog.js路径位置随个人习惯即可)
import Vue from 'vue'
// v-dialogDrag: 弹窗拖拽属性
Vue.directive('dialogDrag', {
bind (el, binding, vnode, oldVnode) {
// 自定义属性,判断是否可拖拽
if (!binding.value) return
const dialogHeaderEl = el.querySelector('.el-dialog__header')
const dragDom = el.querySelector('.el-dialog')
dialogHeaderEl.style.cssText += ';cursor:move;'
dragDom.style.cssText += ';top:0px;'
// 获取原有属性 ie dom元素.currentStyle 火狐谷歌 window.getComputedStyle(dom元素, null);
const sty = (function () {
if (document.body.currentStyle) {
// 在ie下兼容写法
return (dom, attr) => dom.currentStyle[attr]
} else {
return (dom, attr) => getComputedStyle(dom, false)[attr]
}
})()
dialogHeaderEl.onmousedown = (e) => {
// 鼠标按下,计算当前元素距离可视区的距离
// 鼠标距离窗口左侧距离 - 弹窗头部距离定位父元素(弹窗整体)距离,为0
const disX = e.clientX - dialogHeaderEl.offsetLeft
const disY = e.clientY - dialogHeaderEl.offsetTop
// 浏览器宽高
const screenWidth = document.body.clientWidth // body当前宽度
const screenHeight = document.documentElement.clientHeight // 可见区域高度(应为body高度,可某些环境下无法获取)
const dragDomWidth = dragDom.offsetWidth // 对话框宽度
const dragDomheight = dragDom.offsetHeight // 对话框高度
// 弹窗距离浏览器左边距离
const minDragDomLeft = dragDom.offsetLeft
const maxDragDomLeft = screenWidth - dragDom.offsetLeft - dragDomWidth
// 弹窗距离浏览器顶部距离
const minDragDomTop = dragDom.offsetTop
const maxDragDomTop = screenHeight - dragDom.offsetTop - dragDomheight
// 获取到的值带px 正则匹配替换
let styL = sty(dragDom, 'left')
// 为兼容ie
if (styL === 'auto') styL = '0px'
let styT = sty(dragDom, 'top')
// 注意在ie中 第一次获取到的值为组件自带50% 移动之后赋值为px
if (styL.includes('%')) {
styL = +document.body.clientWidth * (+styL.replace(/%/g, '') / 100)
styT = +document.body.clientHeight * (+styT.replace(/%/g, '') / 100)
} else {
styL = +styL.replace(/px/g, '')
styT = +styT.replace(/px/g, '')
};
document.onmousemove = function (e) {
// 通过事件委托,计算移动的距离
// console.log(e, disY)
let left = e.clientX - disX
let top = e.clientY - disY
// 边界处理
if (-(left) > minDragDomLeft) {
left = -(minDragDomLeft)
} else if (left > maxDragDomLeft) {
left = maxDragDomLeft
}
if (-(top) > minDragDomTop) {
top = -(minDragDomTop)
} else if (top > maxDragDomTop) {
top = maxDragDomTop
}
// 移动当前元素
dragDom.style.cssText += `;left:${left + styL}px;top:${top + styT}px;`
}
document.onmouseup = function (e) {
document.onmousemove = null
document.onmouseup = null
}
return false
}
}
})
// v-dialogChange: 弹窗拉伸属性
Vue.directive('dialogChange', {
bind (el, binding, vnode, oldVnode) {
new Vue({}).$nextTick(() => {
// 自定义属性,判断是否可拉伸
if (!binding.value) return
const dragDom = el.querySelector('.el-dialog')
const dragDomBody = el.querySelector('.el-dialog__body')
dragDomBody.style.overflow = 'auto'
const minHeight = 300
const minWidth = 400
// 右下角设置一个图标点击拖拽缩放大小(注意:此处mouse需要与代码中的类名一致,否则会报错)
let dragMouse = document.querySelector('.mouse')
// 鼠标拖拽
dragMouse.onmousedown = (e) => {
// content区域
const content = dragDom.parentNode.parentNode.parentNode.parentNode
const disX = e.clientX - dragDom.offsetWidth
const disY = e.clientY - dragDom.offsetHeight
document.onmousemove = function (e) {
e.preventDefault() // 移动时禁用默认事件
// 通过事件委托,计算移动的距离e
let width = e.clientX - disX
let height = e.clientY - disY
if (width > content.offsetWidth && height < content.offsetHeight) {
if (height < minHeight) {
height = minHeight
}
dragDom.style.height = `${height}px`
// 减去头部高度
dragDomBody.style.maxHeight = `${height - 60}px`
} else if (width < content.offsetWidth && height > content.offsetHeight) {
if (width < minWidth) {
width = minWidth
}
dragDom.style.width = `${width}px`
} else if (width < content.offsetWidth && height < content.offsetHeight) {
if (height < minHeight) {
height = minHeight
}
if (width < minWidth) {
width = minWidth
}
dragDom.style.width = `${width}px`
dragDom.style.height = `${height}px`
// 减去头部高度
dragDomBody.style.maxHeight = `${height - 60}px`
}
}
document.onmouseup = function (e) {
document.onmousemove = null
document.onmouseup = null
}
return false
}
})
}
})
二、易错点
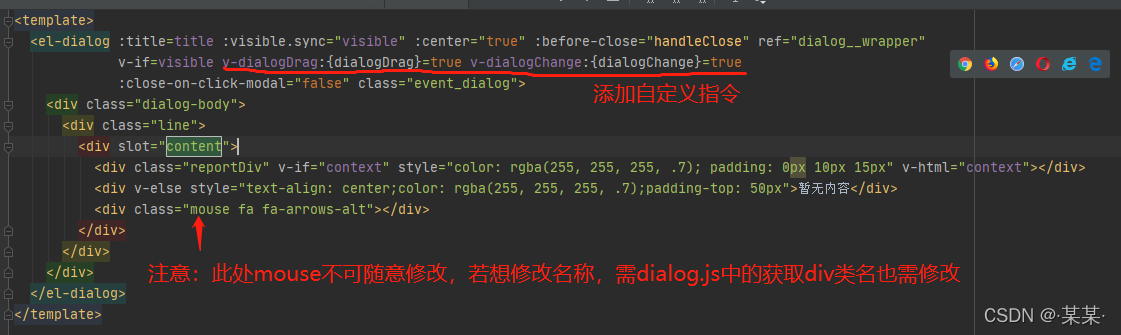
<div class="mouse fa fa-arrows-alt"></div>
<div class="mouse">
</div>
这时按住自定义图片或文字就可以进行缩放了
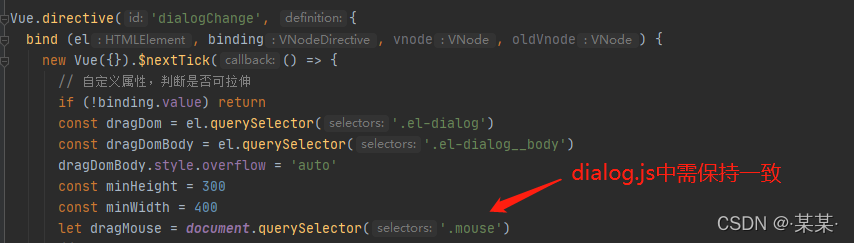
三、完整代码使用
<template>
<!-- 添加缩放、拖动指令 v-dialogDrag:{dialogDrag}=true v-dialogChange:{dialogChange}=true -->
<el-dialog :title=title :visible.sync="visible" :center="true" :before-close="handleClose" ref="dialog__wrapper"
v-if=visible v-dialogDrag:{dialogDrag}=true v-dialogChange:{dialogChange}=true
:close-on-click-modal="false" class="event_dialog">
<div class="dialog-body">
<div class="line">
<div slot="content">
<div class="reportDiv" v-if="context" style="color: rgba(255, 255, 255, .7); padding: 0px 10px 15px" v-html="context"></div>
<div v-else style="text-align: center;color: rgba(255, 255, 255, .7);padding-top: 50px">暂无内容</div>
<!-- 弹窗右下角的缩放图标 -->
<div class="mouse fa fa-arrows-alt"></div>
</div>
</div>
</div>
</el-dialog>
</template>
<script>
import {getStore, setStore} from '@/utils/storage'
export default {
data () {
return {
reportData: null,
title: '',
context: '',
visible: false
}
},
methods: {
init (item) {
// 初始化获取富文本内容
this.visible = true
this.reportData = item
this.title = this.reportData.title
this.context = this.reportData.context
this.$nextTick(() => {
// 设置弹窗大小(若无需保留上次弹窗大小,此段代码可删除)
this.setDialogSize()
})
},
setDialogSize () {
const dragDom = document.querySelector('.event_dialog .el-dialog')
const dragDomBody = document.querySelector('.event_dialog .el-dialog__body')
// 初始化加载上次弹窗位置大小
if (getStore('dialog') !== null) {
let dialog = JSON.parse(getStore('dialog'))
dragDom.style.left = dialog.dialogLeft
dragDom.style.top = dialog.dialogTop
dragDom.style.width = dialog.dialogWidth
dragDom.style.height = dialog.dialogHeight
dragDomBody.style.maxHeight = dialog.dialogBodyMaxHeight
} else {
dragDom.style.left = 0
dragDom.style.top = 0
dragDom.style.width = '843px'
dragDom.style.height = '760px'
dragDomBody.style.maxHeight = '630px'
}
},
// 页面关闭保存弹窗位置及大小(无相关需求业务,也可删除)
handleClose () {
this.$emit('eventClose')
// 关闭的时候缓存窗口偏移、大小
const dragDom = document.querySelector('.event_dialog .el-dialog')
const dragDomBody = document.querySelector('.event_dialog .el-dialog__body')
let dialog = {
dialogLeft: dragDom.style.left,
dialogTop: dragDom.style.top,
dialogWidth: dragDom.style.width,
dialogHeight: dragDom.style.height,
dialogBodyMaxHeight: dragDomBody.style.maxHeight
}
setStore('dialog', dialog)
}
}
}
</script>
<style lang="scss">
.event_dialog {
.el-dialog {
/*height: 760px;*/
background-color: rgba(8, 14, 50, 0.1);
.el-dialog__header .el-dialog__title {
font-size: 28px;
}
.el-dialog__body {
padding: 0;
}
}
}
</style>
<style lang="scss" scoped>
.el-dialog__header .el-dialog__title {
color: #00f4f5!important;
}
.reportDiv {
margin-top: 40px;
font-size: 20px;
}
.mouse {
position: absolute;
bottom: 12px;
right: 18px;
font-size: 20px;
color: #f1f1f1;
}
</style>