基本绘图操作
1. matplotlib简介
matplotlib作为python中的一种绘图模块,可以绘制2D和3D图像,主要用于数据可视化图标的绘制。利用matplotlib可以绘制常用的散点图、折线图、直方图、条形图、功谱图等。
2. matplotlib模块中的pyplot函数集合
matplotlib.pyplot是一个命令型函数集合,它可以让我们像使用MATLAB一样使用matplotlib。pyplot中的每一个函数都会对画布图像作出相应的改变,如创建画布、在画布中创建一个绘图区、在绘图区上画几条线、给图像添加文字说明等。
2.1 导入pyplot
from matplotlib import pyplot as plt
或者
import matplotlib.pyplot as plt
2.2 基本操作
2.2.1 figure() 创建图像、设置图像大小等
(1)作用:创建一张新图像或者激活一张现有的图像
plt.figure(num=None, figsize=None, dpi=None, facecolor=None,
edgecolor=None, frameon=True, FigureClass=<class
'matplotlib.figure.Figure'>, clear=False, **kwargs)
(2)参数:
【1】num:int or str or Figure, optional
A unique identifier for the figure.
If a figure with that identifier already exists, this figure is
made active and returned. An integer refers to the
Figure.number attribute, a string refers to the figure label.
If there is no figure with the identifier or num is not given,
a new figure is created, made active and returned. If num is an
int, it will be used for the Figure.number attribute,
otherwise, an auto-generated integer value is used (starting at
1 and incremented for each new figure). If num is a string, the
figure label and the window title is set to this value.
【2】figsize: (float, float), default: rcParams["figure.figsize"]
(default: [6.4, 4.8]) Width, height in inches.
【3】dpi:float, default: rcParams["figure.dpi"] (default: 100.0)
The resolution of the figure in dots-per-inch.
【4】facecolor: color, default: rcParams["figure.facecolor"]
(default: 'white') The background color.
【5】edgecolor: color, default: rcParams["figure.edgecolor"]
(default: 'white') The border color.
【6】frameonbool: default: True
If False, suppress drawing the figure frame.
【7】FigureClass: subclass of Figure
Optionally use a custom Figure instance.
【8】clear: bool, default: False
If True and the figure already exists, then it is cleared.
【9】constrained_layout: bool, default:
rcParams["figure.constrained_layout.use"]
(default: False)
If True use constrained layout to adjust
positioning of plot elements. Like tight_layout,
but designed to be more flexible. See
Constrained Layout Guide for examples. (Note:
does not work with add_subplot or subplot2grid.)
【10】**kwargs : optional
See Figure for other possible arguments.
【11】返回:
The Figure instance returned will also be passed to
new_figure_manager in the backends, which allows to hook
custom Figure classes into the pyplot interface. Additional
kwargs will be passed to the Figure init function.
2.2.2 title() 创建图像标题
(1)作用:设置轴的标题。设置三个可用轴标题之一。可用标题位于中心轴上方,与左边缘齐平,与右边缘齐平。
plt.title(label, fontdict=None, loc=None, pad=None, *, y=None, **kwargs)
(2)参数:
【1】label: str
Text to use for the title
【2】fontdict: dict
A dictionary controlling the appearance of the title
text, the default fontdict is:
{'fontsize': rcParams['axes.titlesize'],
'fontweight': rcParams['axes.titleweight'],
'color': rcParams['axes.titlecolor'],
'verticalalignment': 'baseline',
'horizontalalignment': loc}
【3】loc: {'center', 'left', 'right'}, default:
rcParams["axes.titlelocation"] (default: 'center')
Which title to set.
【4】y: float, default: rcParams["axes.titley"] (default: None)
Vertical Axes loation for the title (1.0 is the top). If None
(the default), y is determined automatically to avoid decorators
on the Axes.
【5】pad: float, default: rcParams["axes.titlepad"] (default: 6.0)
The offset of the title from the top of the Axes, in points.
【6】**kwargs: Text properties
Other keyword arguments are text properties, see Text for
a list of valid text properties.
【7】返回:
The matplotlib text instance representing the title
2.2.3 figure()和title()放在一起使用
from matplotlib import pyplot as plt
f1 = plt.figure()
plt.title("figure1",loc='left',y=1,pad=1.0)
2.2.4 坐标轴操作
2.2.4.1 xticks()和yticks() 设置x、y轴的刻度
(1) 函数原型:
plt.xticks(ticks, [labels], **kwargs)
(2)参数:
(1)ticks:数组类型,用于设置X轴刻度间隔
(2)[labels]:数组类型,用于设置每个间隔的显示标签
(3)**kwargs:用于设置标签字体倾斜度和颜色等外观属性。
(3)实例代码:
import numpy as np
import matplotlib.pyplot as plt
import calendar
x = range(1,13,1)
y = range(1,13,1)
plt.plot(x,y)
# 参数x空值X轴的间隔,第二个参数控制每个间隔显示的文本,后面两个参数控制标签的颜色和旋转角度
plt.xticks(x, calendar.month_name[1:13],color='blue',rotation=-45)
#plt.xticks(range(1,13,2))
plt.show()
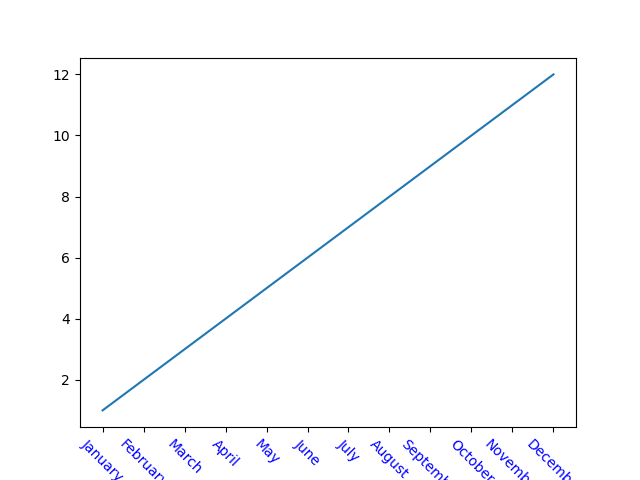 函数同xticks()
2.2.4.2 xlim() 和 ylim() 设置坐标轴范围
(1) 函数原型:
plt.xlim(xmin, xmax)
(2)参数:
(1)xmin:x轴上的最小值
(2)xmax:x轴上的最大值
(3)实例代码:
from matplotlib import pyplot as plt
plt.title("figure1",loc='left',y=1,pad=1.0)
plt.xlim(0,5)
plt.ylim(0,5)
f1 = plt.figure()
注:ylim() 函数同xlim()
2.2.4.3 xlabel() 和 ylabel() 设置坐标轴标签
(1) 函数原型:
plt.xlabel(string)
(2)参数:
(1)string:标签文本内容
(3)实例代码:
from matplotlib import pyplot as plt
plt.title("figure1",loc='left',y=1,pad=1.0)
plt.xlabel('x轴', fontproperties='SimHei', fontsize=20)
plt.ylabel('y轴', fontproperties='SimHei', fontsize=20)
f1 = plt.figure()
2.2.4.4 plt.gca()获取图像的坐标对象
2.2.4.4.1 plt.gca().spines[]. 设置图像的4条边框线
2.2.4.4.2 plt.gca().xaxis. 设置图像的坐标轴
2.2.4.4.3 实现代码
from matplotlib import pyplot as plt
# 创建标题,设置位置、偏移量等
plt.title("figure1", loc='left', y=1, pad=1.0)
# 设置想x、y轴的标签,字体、位置、字体大小
plt.xlabel('x轴', fontproperties='SimHei', loc='right', fontsize=20)
plt.ylabel('y轴', fontproperties='SimHei', loc='top', fontsize=20)
#设置x、y轴的刻度
plt.xticks(range(-5,6,1))
plt.yticks(range(-5,6,1))
# 获取当前图像的坐标对象
# ax = plt.gca()
# 将x轴固定到顶部边框线
# ax.xaxis.set_ticks_position('top')
# 将right、top边框线的颜色设置为none
plt.gca().spines['right'].set_color('none')
plt.gca().spines['top'].set_color('none')
# 将x、y轴分别固定在bottom、left边框线上
plt.gca().xaxis.set_ticks_position('bottom')
plt.gca().yaxis.set_ticks_position('left')
# 将bottom、left边框线按数值移动到0
plt.gca().spines['bottom'].set_position(('data', 0))
plt.gca().spines['left'].set_position(('data', 0))
# 创建图像
f1 = plt.figure()
# 展示图像
plt.show()
2.2.5 plot()绘制图像
(1) 函数原型:
plt.plot(x, y, ls='-', lw=2, label='xxx', color='g' )
(2)参数:
(1)x: x轴上的值
(2)y: y轴上的值
(3)ls:线条风格 (linestyle)
(4)lw:线条宽度 (linewidth)
(5)label:标签文本
(3)实例代码:
import numpy as np
import matplotlib.pyplot as plt
# 创建数值序列
x = np.linspace(0.5, 10, 1000)
y = np.cos(x)
# 绘图
plt.plot(x, y, ls='-', lw=2.5, label='cosine', color='blue')
# 增加图例
plt.legend()
# 设置坐标轴标签
plt.xlabel('x',loc='right')
plt.ylabel('cos(x)',loc='center')
plt.show()
2.2.6 savefig() 保存图像
(1) 函数原型:
plt.savefig(fname, dpi=None, facecolor=’w’, edgecolor=’w’,
orientation=’portrait’, papertype=None, format=None, transparent=False,
bbox_inches=None, pad_inches=0.1, frameon=None, metadata=None)
(2)参数:
(1)fname: (字符串或者仿路径或仿文件)如果格式已经设置,这将决定输出的格式并将文件按fname来保存。如果格式没有设置,在fname有扩展名的情况下推断按此保存,没有扩展名将按照默认格式存储为“png”格式,并将适当的扩展名添加在fname后面。
(2)dpi: 分辨率,每英寸的点数
(3) facecolor(颜色或“auto”,默认值是“auto”):图形表面颜色。如果是“auto”,使用当前图形的表面颜色。
(4) edgecolor(颜色或“auto”,默认值:“auto”):图形边缘颜色。如果是“auto”,使用当前图形的边缘颜色。
(5)orientation – {‘landscape,’ ‘portrait’}: 目前只有后端支持。.
(6) format(字符串):文件格式,比如“png”,“pdf”,“svg”等,未设置的行为将被记录在fname中。
(7)papertype: papertypes可以设置为“a0到a10”, “executive,” “b0 to b10”, “letter,” “legal,” “ledger.”
(8)bbox_inches: 只有图形给定部分会被保存。设置为“tight”用以恰当的匹配所保存的图形。
(9)pad_inches: (默认: 0.1)所保存图形周围的填充量。
(10) transparent: 用于将图片背景设置为透明。图形也会是透明,除非通过关键字参数指定了表面颜色和/或边缘颜色。
(3)实例代码:
import numpy as np
import matplotlib.pyplot as plt
# 创建数值序列
x = np.linspace(0.5, 10, 1000)
y = np.cos(x)
# 绘图
plt.plot(x, y, ls='-', lw=2.5, label='cosine', color='blue')
# 增加图例
plt.legend()
# 设置坐标轴标签
plt.xlabel('x',loc='right')
plt.ylabel('cos(x)',loc='center')
plt.savefig("./cos(x).png")
plt.show()
2.2.7 图像坐标轴显示中文问题
(1) 解决方法:
import matplotlib.pyplot as plt
plt.rcParams['font.sans-serif']=['SimHei'] #用来正常显示中文标签
plt.rcParams['axes.unicode_minus']=False #用来正常显示负号
(2)实例代码:
from matplotlib import pyplot as plt
plt.rcParams['font.sans-serif']=['SimHei']
plt.figure()
plt.title("气温表")
x = [i for i in range(1,13,1)]
y = [25,23,20,23,21,26,28,29,30,25,27,26]
plt.plot(x,y)
_xtick_labels = ["3月{}日".format(i) for i in x]
plt.xticks(x, _xtick_labels,color='blue',rotation=-45)
plt.show()