package com.pes.common.utils;
import java.text.ParseException;
import java.text.ParsePosition;
import java.text.SimpleDateFormat;
import java.util.Arrays;
import java.util.Calendar;
import java.util.Date;
import java.util.GregorianCalendar;
import java.util.TimeZone;
/**
* <p>日期工具类</p>
*/
public class DateKit {
/**定义常量**/
private static final String DATE_FULL_STR = "yyyy-MM-dd HH:mm:ss";
private static final Long DEFAULT_TIME_VALUE = 24*60*60*1000L;
public DateKit() {
}
/**
* <p>获得当前时间<日期格式>
* @return yyyy-MM-dd HH:mm:ss
*/
public static Date getCurrDateTime() {
return new Date(System.currentTimeMillis());
}
/**
* 取得当前系统时间<字符格式>
* @return yyyy-MM-dd HH:mm:ss
*/
public static String getCurrTime() {
Date date_time = new Date();
return FormatDate(date_time, "yyyy-MM-dd HH:mm:ss");
}
/**
* 取得当前系统日期<字符格式>
* @return yyyy-MM-dd
*/
public static String getCurrDate() {
Date date_time = new Date();
return FormatDate(date_time, "yyyy-MM-dd");
}
/**
* 取得日期的年份
* @param date 日期
* @return yyyy 年份字符串
*/
public static String getYear(Date date) {
return FormatDate(date, "yyyy");
}
/**
* 取得日期的月份
* @param date 日期
* @return mm 月份字符串
*/
public static String getMonth(Date date) {
return FormatDate(date, "MM");
}
/**
* 取得日期的天份
* @param date 日期
* @return dd 天字符串
*/
public static String getDay(Date date) {
return FormatDate(date, "dd");
}
/**
* 取得日期的小时
* @param date 日期
* @return hh 小时字符串
*/
public static String getHour(Date date) {
return FormatDate(date, "HH");
}
/**
* 取得日期的分钟
* @param date 时间
* @return mm 分钟字符串
*/
public static String getMinute(Date date) {
return FormatDate(date, "mm");
}
/**
* 取得时间的秒
* @param date 时间
* @return ss 秒字符串
*/
public static String getSecond(Date date) {
return FormatDate(date, "ss");
}
/**
* 使用预设格式提取字符串日期
* @param strDate 日期字符串
* @return
*/
public static Date parse(String strDate) {
return parse(strDate,DATE_FULL_STR);
}
/**
* 使用用户格式提取字符串日期
* @param strDate 日期字符串
* @param pattern 日期格式
* @return
*/
public static Date parse(String strDate, String pattern) {
SimpleDateFormat df = new SimpleDateFormat(pattern);
try {
strDate = df.format(strDate);
return df.parse(strDate);
} catch (ParseException e) {
return null;
}
}
/**
* 把yyyyMMdd 转成 yyyy-MM-dd 格式
* @param date
* @return
*/
public static String formatDate(String date){
SimpleDateFormat sdf1 = new SimpleDateFormat("yyyyMMdd");
SimpleDateFormat sdf2 = new SimpleDateFormat("yyyy-MM-dd");
String format = "";
try {
format = sdf2.format(sdf1.parse(date));
} catch (ParseException e) {
e.printStackTrace();
}
return format;
}
/**
* 两个时间比较
* @param date
* @return
*/
public static int compareDateWithNow(Date date1){
Date date2 = new Date();
int rnum =date1.compareTo(date2);
return rnum;
}
/**
* 两个时间比较(时间戳比较)
* @param date
* @return
*/
public static int compareDateWithNow(long date1){
long date2 = dateToUnixTimestamp();
if(date1>date2){
return 1;
}else if(date1<date2){
return -1;
}else{
return 0;
}
}
/**
* 获取系统当前时间
* @return
*/
public static String getNowTime(String type) {
SimpleDateFormat df = new SimpleDateFormat(type);
return df.format(new Date());
}
/**
* 将指定的日期转换成Unix时间戳
* @param String date 需要转换的日期 yyyy-MM-dd HH:mm:ss
* @return long 时间戳
*/
public static long dateToUnixTimestamp(String date) {
long timestamp = 0;
try {
timestamp = new SimpleDateFormat(DATE_FULL_STR).parse(date).getTime();
} catch (ParseException e) {
e.printStackTrace();
}
return timestamp;
}
/**
* 将指定的日期转换成Unix时间戳
* @param String date 需要转换的日期 yyyy-MM-dd
* @return long 时间戳
*/
public static long dateToUnixTimestamp(String date, String dateFormat) {
long timestamp = 0;
try {
timestamp = new SimpleDateFormat(dateFormat).parse(date).getTime();
} catch (ParseException e) {
e.printStackTrace();
}
return timestamp;
}
/**
* 将当前日期转换成Unix时间戳
* @return long 时间戳
*/
public static long dateToUnixTimestamp() {
long timestamp = new Date().getTime();
return timestamp;
}
/**
* 将Unix时间戳转换成日期
* @param long timestamp 时间戳
* @return String 日期字符串
*/
public static String unixTimestampToDate(long timestamp) {
SimpleDateFormat sd = new SimpleDateFormat(DATE_FULL_STR);
sd.setTimeZone(TimeZone.getTimeZone("GMT+8"));
return sd.format(new Date(timestamp));
}
/**
* 日期转化为字符串
* @param date 时间
* @return yyyy-MM-dd HH:mm:ss 格式化的时间字符串
*/
public static String dateToString(Date date) {
if (date == null)
return "";
return FormatDate(date, "yyyy-MM-dd HH:mm:ss");
}
/**
* 日期转化为字符串
* @param date 时间
* @return yyyy-MM-dd 格式化的时间字符串
*/
public static String dateToStringShort(Date date) {
if (date == null)
return "";
return FormatDate(date, "yyyy-MM-dd");
}
/**
* 字符串转换为日期
* @param dateString yyyy-MM-dd HH:mm:ss
* @return 日期
*/
public static Date stringToDate(String dateString) {
String sf = "yyyy-MM-dd HH:mm:ss";
Date dt = stringToDate(dateString, sf);
return dt;
}
/**
* 字符串转换为日期
* @param dateString yyyy-MM-dd
* @return 日期
*/
public static Date stringToDateShort(String dateString) {
String sf = "yyyy-MM-dd";
Date dt = stringToDate(dateString, sf);
return dt;
}
/**
* 主要在日期区间查询中使用 例:查询区间2011-10-09 - 2011-10-09
* 为了也可以查询出2011-10-09 00:00:00 - 2011-10-09 23:59:59 间的数据
* 字符串转换字符串
*
* @param dateString yyyy-MM-dd
* @return yyyy-MM-dd HH:mm:ss
*/
public static String stringToString(String dateString) {
return dateString + " 23:59:59";
}
/**
* 对日期进行格式化
* @param date 日期
* @param sf 日期格式
* @return 字符串
*/
public static String FormatDate(Date date, String sf) {
if (date == null)
return "";
SimpleDateFormat dateformat = new SimpleDateFormat(sf);
return dateformat.format(date);
}
/** 字符串转换为日期
* @param dateString 日期格式字符串
* @param sf 日期格式化定义
* @return 转换后的日期
*/
public static Date stringToDate(String dateString, String sf) {
ParsePosition pos = new ParsePosition(0);
SimpleDateFormat sdf = new SimpleDateFormat(sf);
Date dt = sdf.parse(dateString, pos);
return dt;
}
/**
* 计算两个日期差(毫秒)
* @param date1 时间1
* @param date2 时间2
* @return 相差毫秒数
*/
public static long diffTwoDate(Date date1, Date date2) {
long l1 = date1.getTime();
long l2 = date2.getTime();
return (l1 - l2);
}
/**
* 计算两个时间差(毫秒)
* @param date1 时间1
* @param date2 时间2
* @return 相差毫秒数
*/
public static long diffTwoStringDate(String dateString1, String dateString2) {
long diff = 0;
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
try {
Date d1 = sdf.parse(dateString1);
Date d2 = sdf.parse(dateString2);
diff = d1.getTime()-d2.getTime();
} catch (Exception e) {
e.printStackTrace();
}
return diff;
}
/**
* 计算两个日期差(天)
* @param date1 时间1
* @param date2 时间2
* @return 相差天数
*/
public static int diffTwoDateDay(Date date1, Date date2) {
long l1 = date1.getTime();
long l2 = date2.getTime();
int diff = Integer.parseInt("" + (l1 - l2) / 3600 / 24 / 1000);
return diff;
}
/**
*
* @param currentTime 计算的日期
* @param type 偏移的类别
* @param iQuantity 偏移数量
* @return 偏移后的时间串
*/
public static String getDateChangeTime(String currentTime, String type, int iQuantity) {
Date curr = stringToDate(currentTime);
curr = getDateChangeTime(curr, type, iQuantity);
return dateToString(curr);
}
/**
*
* @param currentTime 计算的日期
* @param type 偏移的类别
* @param iQuantity 偏移数量
* @return 偏移后的时间
*/
public static Date getDateChangeTime(Date currentTime, String type, int iQuantity) {
int year = Integer.parseInt(FormatDate(currentTime, "yyyy"));
int month = Integer.parseInt(FormatDate(currentTime, "MM"));
// 月份修正
month = month - 1;
int day = Integer.parseInt(FormatDate(currentTime, "dd"));
int hour = Integer.parseInt(FormatDate(currentTime, "HH"));
int mi = Integer.parseInt(FormatDate(currentTime, "mm"));
int ss = Integer.parseInt(FormatDate(currentTime, "ss"));
GregorianCalendar gc = new GregorianCalendar(year, month, day, hour, mi, ss);
// 月份修正
// gc.add(GregorianCalendar.MONTH, -1);
if (type.equalsIgnoreCase("y")) {
gc.add(GregorianCalendar.YEAR, iQuantity);
} else if (type.equalsIgnoreCase("m")) {
gc.add(GregorianCalendar.MONTH, iQuantity);
} else if (type.equalsIgnoreCase("d")) {
gc.add(GregorianCalendar.DATE, iQuantity);
} else if (type.equalsIgnoreCase("h")) {
gc.add(GregorianCalendar.HOUR, iQuantity);
} else if (type.equalsIgnoreCase("mi")) {
gc.add(GregorianCalendar.MINUTE, iQuantity);
} else if (type.equalsIgnoreCase("s")) {
gc.add(GregorianCalendar.SECOND, iQuantity);
}
return gc.getTime();
}
/**
*根据年、月取得月末的日期
* @param year 年
* @parm month 月
* @return time 返回日期格式"yyyy-mm-dd"
*/
public static String getTime(String year, String month) {
String time = "";
int len = 31;
int iYear = Integer.parseInt(year);
int iMonth = Integer.parseInt(month);
if (iMonth == 4 || iMonth == 6 || iMonth == 9 || iMonth == 11)
len = 30;
if (iMonth == 2) {
len = 28;
if ((iYear % 4 == 0 && iYear % 100 == 0 && iYear % 400 == 0) || (iYear % 4 == 0 && iYear % 100 != 0)) {
len = 29;
}
}
time = year + "-" + month + "-" + String.valueOf(len);
return time;
}
/**
* 取月初
* @param date
* @return
*/
public static Date getMonthBegin(Date date) {
String newDateStr = FormatDate(date, "yyyy-MM") + "-01";
// FormatDate(date, "yyyy-MM-dd");
return stringToDateShort(newDateStr);
}
/**
* 取月末时间
* @param date 日期
* @return date
*/
public static Date getMonthEnd(Date date) {
int year = Integer.parseInt(FormatDate(date, "yyyy"));
int month = Integer.parseInt(FormatDate(date, "MM"));
int day = Integer.parseInt(FormatDate(date, "dd"));
GregorianCalendar calendar = new GregorianCalendar(year, month - 1, day, 0, 0, 0);
int monthLength = calendar.getActualMaximum(Calendar.DAY_OF_MONTH);
String newDateStr = FormatDate(date, "yyyy") + "-" + FormatDate(date, "MM") + "-";
if (monthLength < 10)
newDateStr += "0" + monthLength;
else
newDateStr += "" + monthLength;
return stringToDateShort(newDateStr);
}
/**
* 取季初
* @param date
* @return
*/
public static Date getSeasonBegin(Date date) {
int month = Integer.parseInt(FormatDate(date, "MM"));
String newDateStr = FormatDate(date, "yyyy") + "-";
if (month >= 1 && month <= 3) {
newDateStr += "01-01";
} else if (month >= 4 && month <= 6) {
newDateStr += "04-01";
} else if (month >= 7 && month <= 9) {
newDateStr += "07-01";
} else if (month >= 10 && month <= 12) {
newDateStr += "10-01";
}
return stringToDateShort(newDateStr);
}
/**
* 取半年初
* @param date
* @return
*/
public static Date getHalfYearBegin(Date date) {
int month = Integer.parseInt(FormatDate(date, "MM"));
String newDateStr = FormatDate(date, "yyyy") + "-";
if (month <= 6) {
newDateStr += "01-01";
} else {
newDateStr += "07-01";
}
return stringToDateShort(newDateStr);
}
/**
* 取旬初
* @param date
* @return
*/
public static Date getPeriodBegin(Date date) {
int days = Integer.parseInt(FormatDate(date, "dd"));
String newDateStr = FormatDate(date, "yyyy-MM") + "-";
if (days <= 10) {
newDateStr += "01";
} else if (days <= 20) {
newDateStr += "11";
} else {
newDateStr += "21";
}
return stringToDateShort(newDateStr);
}
/**
* 取周初
* @param date
* @return
*/
public static Date getWeekBegin(Date date) {
int year = Integer.parseInt(FormatDate(date, "yyyy"));
int month = Integer.parseInt(FormatDate(date, "MM"));
// 月份修正
month = month - 1;
int day = Integer.parseInt(FormatDate(date, "dd"));
GregorianCalendar gc = new GregorianCalendar(year, month, day);
int week = gc.get(GregorianCalendar.DAY_OF_WEEK) - 1;
if (week == 0) {
week = 7;
}
gc.add(GregorianCalendar.DATE, 0 - week + 1);
return gc.getTime();
}
/**
* 取周末
* @param date
* @return
*/
public static Date getWeekEnd(Date date) {
int year = Integer.parseInt(FormatDate(date, "yyyy"));
int month = Integer.parseInt(FormatDate(date, "MM"));
// 月份修正
month = month - 1;
int day = Integer.parseInt(FormatDate(date, "dd"));
GregorianCalendar gc = new GregorianCalendar(year, month, day);
int week = gc.get(GregorianCalendar.DAY_OF_WEEK) - 1;
if (week == 0) {
week = 7;
}
gc.add(GregorianCalendar.DATE, 7 - week);
return gc.getTime();
}
/**
* 取旬末
* @param date
* @return
*/
public static Date getPeriodEnd(Date date) {
int days = Integer.parseInt(FormatDate(date, "dd"));
String newDateStr = FormatDate(date, "yyyy-MM") + "-";
if (days <= 10) {
newDateStr += "10";
} else if (days <= 20) {
newDateStr += "20";
} else {
newDateStr = FormatDate(getMonthEnd(date), "yyyy-MM-dd");
}
return stringToDateShort(newDateStr);
}
/**
* 取半年末
* @param date
* @return
*/
public static Date getHalfYearEnd(Date date) {
int month = Integer.parseInt(FormatDate(date, "MM"));
String newDateStr = FormatDate(date, "yyyy") + "-";
if (month <= 6) {
newDateStr += "06-30";
} else {
newDateStr += "12-31";
}
return stringToDateShort(newDateStr);
}
/**
* 取季度末时间
* @param date 日期
* @return date
*/
public static Date getSeasonEnd(Date date) {
int month = Integer.parseInt(FormatDate(date, "MM"));
String newDateStr = FormatDate(date, "yyyy") + "-";
if (month >= 1 && month <= 3) {
newDateStr += "03-31";
} else if (month >= 4 && month <= 6) {
newDateStr += "06-30";
} else if (month >= 7 && month <= 9) {
newDateStr += "09-30";
} else if (month >= 10 && month <= 12) {
newDateStr += "12-31";
}
return stringToDateShort(newDateStr);
}
/**
* 取得年初
* @param date
* @return
*/
public static Date getYearBegin(Date date) {
String newDateStr = FormatDate(date, "yyyy") + "-01-01";
return stringToDateShort(newDateStr);
}
/**
* 是否为年末
* @param date 时间
* @return
*/
public static Date getYearEnd(Date date) {
String newDateStr = FormatDate(date, "yyyy") + "-12-31";
return stringToDateShort(newDateStr);
}
/**
* 是否为旬末
* @param date 时间
* @return 是或否
*/
public static boolean IsXperiodEnd(Date date) {
boolean flag = false;
String day = getDay(date);
if (day.equalsIgnoreCase("10")) {
flag = true;
} else if (day.equalsIgnoreCase("20")) {
flag = true;
}
return flag;
}
/**
* 获取一个月后的同一天的String类型日期
* @return String
*/
public static String getDateAfterAMonth(String date) {
Date iDate = stringToDateShort(date);
Calendar calendar = Calendar.getInstance();
calendar.setTime(iDate);
calendar.add(Calendar.MONTH, 1);
SimpleDateFormat df = new SimpleDateFormat("yyyy-MM-dd");
String s = df.format(calendar.getTime());
return s;
}
/**
* 比较日期大小
* @author gaofeng
* @param dateString1
* @param dateString2
* @return rslt
*/
public static int compareDateString(String dateString1, String dateString2) {
int rslt = 0;
java.util.Date date1 = stringToDate(dateString1, "yyyy-MM-dd");
java.util.Date date2 = stringToDate(dateString2, "yyyy-MM-dd");
int intdate1 = Integer.parseInt(FormatDate(date1, "yyyyMMdd"));
int intdate2 = Integer.parseInt(FormatDate(date2, "yyyyMMdd"));
if (intdate1 > intdate2) {
rslt = 1;
} else if (intdate1 < intdate2) {
rslt = -1;
} else {
rslt = 0;
}
return rslt;
}
/**
* 比较时间大小
* @author gaofeng
* @param dateString1
* @param dateString2
* @return rslt
*/
public static int compareTimeString(String dateString1, String dateString2) {
int rslt = 0;
SimpleDateFormat sdf =new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
Calendar c1 = Calendar.getInstance();
Calendar c2 = Calendar.getInstance();
try{
c1.setTime(sdf.parse(dateString1));
c2.setTime(sdf.parse(dateString2));
}catch(ParseException e){
e.printStackTrace();
}
if(c1.compareTo(c2)>0){
rslt = 1;
} else if(c1.compareTo(c2)<0){
rslt = -1;
} else{
rslt = 0;
}
return rslt;
}
/**
* 比较日期大小
* @author gaofeng
* @param dateString1
* @param dateString2
* @return
*/
public static int compareDate(Date date1, Date date2) {
int rslt = 0;
int intdate1 = Integer.parseInt(FormatDate(date1, "yyyyMMdd"));
int intdate2 = Integer.parseInt(FormatDate(date2, "yyyyMMdd"));
if (intdate1 > intdate2) {
rslt = 1;
} else if (intdate1 < intdate2) {
rslt = -1;
} else {
rslt = 0;
}
return rslt;
}
/**
* 判断日期是否在指定的范围日期内
* @param startDate
* @param endDate
* @return
*/
public static boolean inDate(String startDate,String endDate ){
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
boolean succ = false;
try {
Date start = sdf.parse(startDate);
Date end = sdf.parse(endDate);
Date curr = DateKit.getCurrDateTime();
if (start.after(curr) && end.before(curr)) {
succ = true;
}
} catch (Exception e) {
succ = false;
}
return succ;
}
/**
* 获得当前是否在指定的日期范围内
* @param weekDate
* @return
*/
public static boolean inWeek(String... weekDate) {
Calendar cal = Calendar.getInstance();
String w = String.valueOf(cal.get(Calendar.DAY_OF_WEEK));
if (Arrays.asList(weekDate).contains(w)) {
return true;
}
return false;
}
/**
* 获得当前日期是否在指定的月份范围内
* @param monthDate
* @return
*/
public static boolean inMonth(String monthDate) {
Calendar cal = Calendar.getInstance();
String w = String.valueOf(cal.get(Calendar.DAY_OF_MONTH));
if (Arrays.asList(monthDate).contains(w)) {
return true;
}
return false;
}
/**
* 获得指定时间后毫秒数的日期
* @param date
* @param millSecond
* @return
*/
public static String getDateAddMillSecond(Date date,int millSecond){
Calendar cal = Calendar.getInstance();
if (null != date) {
cal.setTime(date);
}
cal.add(Calendar.MILLISECOND, millSecond);
return DateKit.dateToString(cal.getTime());
}
/**
* 获得到指定日期剩余秒数
* @param date
* @return
*/
public static Long remainSeconds(String date){
Date d = DateKit.stringToDate(date);
Calendar currDate = Calendar.getInstance();
currDate.setTime(d);
return currDate.getTimeInMillis();
}
/**
* 获得到指定日期剩余(秒)数
* @param date 结束日期
* @return
*/
public static int getSecondTime(String date){
long time = 0;
Calendar currDate = Calendar.getInstance();
Calendar cal = Calendar.getInstance();
if (null != date) {
SimpleDateFormat sf = new SimpleDateFormat("yyyy-MM-dd");
try {
cal.setTime(sf.parse(date));
cal.set(Calendar.HOUR_OF_DAY, 24);
cal.set(Calendar.MINUTE, 0);
cal.set(Calendar.SECOND, 0);
cal.set(Calendar.MILLISECOND, 0);
} catch (ParseException e) {}
}
time = (cal.getTimeInMillis()-currDate.getTimeInMillis())/1000;
return (int) time;
}
public static int getSecondTime(Date date){
Calendar currDate = Calendar.getInstance();
Calendar cal = Calendar.getInstance();
cal.setTime(date);
cal.set(Calendar.HOUR_OF_DAY, 24);
cal.set(Calendar.MINUTE, 0);
cal.set(Calendar.SECOND, 0);
cal.set(Calendar.MILLISECOND, 0);
return (int) ((cal.getTimeInMillis()-currDate.getTimeInMillis())/1000);
}
public static Date getTimesnight(){
Calendar cal = Calendar.getInstance();
cal.set(Calendar.HOUR_OF_DAY, 24);
cal.set(Calendar.MINUTE, 0);
cal.set(Calendar.SECOND, 0);
cal.set(Calendar.MILLISECOND, 0);
return cal.getTime();
}
/**
* 获得今天星期几
* @return 1:星期日
* 2:星期一
* 3:星期二
* 4:星期三
*/
public static String getCurrWeek() {
Calendar cal = Calendar.getInstance();
return String.valueOf(cal.get(Calendar.DAY_OF_WEEK));
}
/**
* 获得当前日期
* @return yyyyMMdd
*/
public static String getCurrDateFormat(){
Calendar cal = Calendar.getInstance();
SimpleDateFormat sdf = new SimpleDateFormat("yyyyMMdd");
return sdf.format(cal.getTime());
}
/**
* 获得当前小时
* @return
*/
public static String getCurrHour(){
Calendar cal = Calendar.getInstance();
return FormatDate(cal.getTime(), "HH");
}
/**
* 比较两个日期大小
* @param date1
* @param date2
* @return <pre>
*-1: date1小于date2
* 0: date1等于date2
* 1: date1大于date2
* </pre>
*/
public static int compareBetweenDate(Date date1, Date date2) {
Calendar first = Calendar.getInstance();
first.setTime(date1);
Calendar second = Calendar.getInstance();
second.setTime(date2);
return first.getTimeInMillis() - second.getTimeInMillis() < 0 ? -1 : first.getTimeInMillis() - second.getTimeInMillis() == 0 ? 0 : 1;
}
/**
* 是否显示倒计时
* @param date 活动日期
* @param days 活动日期前几天
* @return
*/
public static Boolean isShowCountDown(Date date,int days){
Long value = days * DEFAULT_TIME_VALUE;
Calendar currDay = Calendar.getInstance();
Calendar actyDay = Calendar.getInstance();
actyDay.setTime(date);
actyDay.set(Calendar.DATE, actyDay.get(Calendar.DATE)-days);
if ((actyDay.getTimeInMillis() - currDay.getTimeInMillis()) < value) {
return true;
}
return false;
}
private static int getDifferDate(int week){
Calendar currDay = Calendar.getInstance();
int dayofWeek = currDay.get(Calendar.DAY_OF_WEEK);
if (dayofWeek == week) {
return 0;
}else{
return week-dayofWeek;
}
}
/**
* 获得到下周的日期
* @param week 1 周日 2 周一 3 周二 4 周三 5 周四 6 周五 7 周六
* @return
*/
public static Date getNextWeek(int week){
int mondayPlus = getDifferDate(week);
Calendar currDate = new GregorianCalendar();
currDate.add(Calendar.DATE, mondayPlus+7);
return currDate.getTime();
}
/**
* 获得到本周的日期
* @param week 1 周日 2 周一 3 周二 4 周三 5 周四 6 周五 7 周六
* @return
*/
public static Date getCurrWeek(int week){
int mondayPlus = getDifferDate(week);
Calendar currDate = new GregorianCalendar();
currDate.add(Calendar.DATE, mondayPlus);
return currDate.getTime();
}
/**
* 判断日期是否在范围内
* @param begin 起始日期
* @param date 比对日期
* @param end 结束日期
* @return Boolean true在,false不在。另外begin=date,date=end时认为在范围内
*/
public static Boolean dateIn(Date begin, Date date, Date end){
Boolean flag = true;
if(compareBetweenDate(begin, date) == 1 || compareBetweenDate(date, end) == 1){
flag = false;
}
return flag;
}
public static Boolean timeIn(String begin, String compare, String end){
Boolean flag = true;
String left[] = begin.split(":");
String comp[] = compare.split(":");
String right[] = end.split(":");
int leftLimit = Integer.parseInt(left[0]+left[1]+left[2]);
int compareOne = Integer.parseInt(comp[0]+comp[1]+comp[2]);
int rightLimit = Integer.parseInt(right[0]+right[1]+right[2]);
if(leftLimit <= compareOne && compareOne < rightLimit){
flag = false;
}
return flag;
}
/**
*
* @param begin 起始日期
* @param end 截止日期
* @param day 星期几("01"星期日,"02"星期一,"03"星期二,"04"星期三,"05"星期四,"06"星期五,"07"星期六)
* @return
*/
public static int countWeeks(Date begin,Date end,String day){
int count = 0;
long beginTime = begin.getTime();
long endTime = end.getTime();
count += (endTime - beginTime)/(24*3600000) + 1;
count = count/7;
//以上:从起始日期到截止日期中有多少个整周
return count;
}
public static void main(String[] args) {
// System.out.println(getSecondTime(getWeekEnd(new Date())));
// System.out.println(getCurrWeek(4));
//System.out.println(isShowCountDown(getNextWeek(3), 2));
//System.out.println(getSecondTime(getNextWeek(3)));
String s = "0305|123456|MS0000001|684058450231|20160930|20170920|201609291212";
System.out.println(s.substring(59, 112));
}
}
时间日期类
最新推荐文章于 2024-05-19 14:55:00 发布
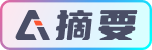