package sortTest;
import java.util.Comparator;
/**
* The {@code Selection} class provides static methods for sorting an
* array using <em>selection sort</em>.
* This implementation makes ~ ½ <em>n</em><sup>2</sup> compares to sort
* any array of length <em>n</em>, so it is not suitable for sorting large arrays.
* It performs exactly <em>n</em> exchanges.
* <p>
* This sorting algorithm is not stable. It uses Θ(1) extra memory
* (not including the input array).
* <p>
* For additional documentation, see
* <a href="https://algs4.cs.princeton.edu/21elementary">Section 2.1</a>
* of <i>Algorithms, 4th Edition</i> by Robert Sedgewick and Kevin Wayne.
*
* @author Robert Sedgewick
* @author Kevin Wayne
*/
public class Selection {
// This class should not be instantiated.
private Selection() { }
/**
* Rearranges the array in ascending order, using the natural order. 使用自然顺序,按升序重新排列数组。
* @param a the array to be sorted
*/
public static void sort(Comparable[] a) {
int n = a.length;
for (int i = 0; i < n; i++) { //表示总共比较的趟数
int min = i; //取最小基准点,让其它元素和其比较
for (int j = i+1; j < n; j++) { //找到基准点后的第一个元素,从这个元素开始往后依次和基准点对应的值比较
if (less(a[j], a[min])) //如果在序列中找到比基准元素对应值小,执行
min = j; //改变最小基准标记点给小的元素
}
exch(a, i, min); //交换a[i]和a[min]两个元素
assert isSorted(a, 0, i); //判断目前排好的序列是否正确
}
assert isSorted(a); //排序完后判断是否排序成功
}
/**
* Rearranges the array in ascending order, using a comparator. 升序
* @param a the array
* @param comparator the comparator specifying the order
*/
public static void sort(Object[] a, Comparator comparator) {
int n = a.length;
for (int i = 0; i < n; i++) { //表示总共比较的趟数
int min = i; //取最小基准点,让其它元素和其比较
for (int j = i+1; j < n; j++) { //找到基准点后的第一个元素,从这个元素开始往后依次和基准点对应的值比较
if (less(comparator, a[j], a[min])) //如果在序列中找到比基准元素对应值小,执行
min = j; //改变最小基准标记点给小的元素
}
exch(a, i, min); //交换a[i]和a[min]两个元素
assert isSorted(a, comparator, 0, i); //判断目前排好的序列是否正确
}
assert isSorted(a, comparator); //排序完后判断是否排序成功
}
/***************************************************************************
* Helper sorting functions.
***************************************************************************/
// is v < w ? 比较大小
private static boolean less(Comparable v, Comparable w) {
return v.compareTo(w) < 0;
}
// is v < w ? 比较大小
private static boolean less(Comparator comparator, Object v, Object w) {
return comparator.compare(v, w) < 0;
}
// exchange a[i] and a[j] 交换
private static void exch(Object[] a, int i, int j) {
Object swap = a[i];
a[i] = a[j];
a[j] = swap;
}
/***************************************************************************
* Check if array is sorted - useful for debugging.
***************************************************************************/
// is the array a[] sorted? 判断数组是否排好序
private static boolean isSorted(Comparable[] a) {
return isSorted(a, 0, a.length - 1);
}
// is the array sorted from a[lo] to a[hi] 判断数组是否按照升序排列
private static boolean isSorted(Comparable[] a, int lo, int hi) {
for (int i = lo + 1; i <= hi; i++)
if (less(a[i], a[i-1])) return false; //如果数组后一个数比前一个数小,则不是标准的升序排列,返回false
return true;
}
// is the array a[] sorted? 判断数组是否排好序
private static boolean isSorted(Object[] a, Comparator comparator) {
return isSorted(a, comparator, 0, a.length - 1);
}
// is the array sorted from a[lo] to a[hi]
private static boolean isSorted(Object[] a, Comparator comparator, int lo, int hi) {
for (int i = lo + 1; i <= hi; i++)
if (less(comparator, a[i], a[i-1])) return false; //如果数组后一个数比前一个数小,则不是标准的升序排列,返回false
return true;
}
// print array to standard output 输出序列的所有值
private static void show(Comparable[] a) {
for (int i = 0; i < a.length; i++) {
StdOut.println(a[i]);
}
}
/**
* Reads in a sequence of strings from standard input; selection sorts them;
* and prints them to standard output in ascending order.
*
* @param args the command-line arguments
*/
public static void main(String[] args) {
String[] a = StdIn.readAllStrings();
Selection.sort(a);
show(a);
}
}
选择排序
最新推荐文章于 2024-10-13 00:00:12 发布
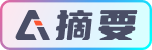