JS自定义倒计时代码
资源下载:https://download.csdn.net/download/weixin_43501359/14988671
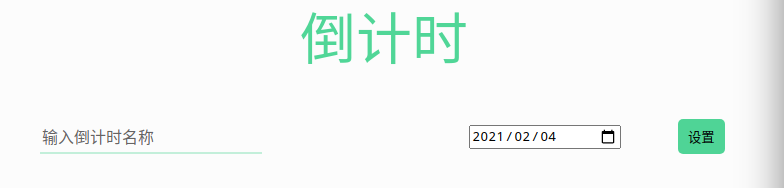
index.html
<!DOCTYPE html>
<html lang="en" >
<head>
<meta charset="UTF-8">
<title>JS自定义倒计时代码</title>
<link rel="stylesheet" href="css/style.css">
</head>
<body>
<main>
<h1 class="head"><span id="msg">倒计时</span></h1>
<h1 id="error"></h1>
<form id="countdown-form">
<div class="name">
<input type="text" name="eventName" id="eventName" />
<label for="eventName" class="label">
<span>输入倒计时名称</span>
</label>
</div>
<input type="date" name="endDate" id="end" />
<button>设置</button>
</form>
<section class="result" style="display: none;">
<p class="days">00<span>天</span></p>
<p class="hours">00 <span>时</span></p>
<p class="minutes">00 <span>分</span></p>
<p class="seconds">00 <span>秒</span></p>
</section>
<button id="cancel" style="display: none;">取消倒计时</button>
</main>
<script src="js/script.js"></script>
</body>
</html>
script.js
var cdown= {
head: document.querySelector(".head"),
message: document.querySelector("#msg"),
form: document.querySelector("#countdown-form"),
input: document.querySelector("#eventName"),
label: document.querySelector(".label>span"),
name: document.querySelector(".name #eventName"),
dayDOM: document.querySelector(".days"),
hourDOM: document.querySelector(".hours"),
minuteDOM: document.querySelector(".minutes"),
secondDOM: document.querySelector(".seconds"),
dateDom: document.querySelector("#end"),
cancelDom: document.querySelector("#cancel"),
errorDom:document.querySelector("#error"),
targetDate: 0,
timer: null,
// 开启倒计时
opencountdown: function(targetDate){
cdown.timer = setInterval(function(){
if (targetDate) {
cdown.startCountdown(targetDate);
} else {
cdown.controlError();
}
}, 1000)
},
// 更新时间显示
updateDom:function ({ seconds, minutes, hours, days }){
// Update the dom with the right values
cdown.dayDOM.innerHTML = `${days} <span>天</span>`;
cdown.secondDOM.innerHTML = `${seconds} <span>秒</span>`;
cdown.minuteDOM.innerHTML = `${minutes} <span>分</span>`;
cdown.hourDOM.innerHTML = `${hours} <span>时</span>`;
},
// 错误
controlError:function(){
cdown.errorDom.textContent = '你设在的日期已过去';
cdown.errorDom.classList.add("error");
cdown.cancel();
cdown.errorDom.style.display='';
},
// 倒计时
startCountdown: function(date){
const actualDate = new Date(date);
const dateInMilliseconds = actualDate.getTime();
const now = new Date();
const nowInMilliseconds = now.getTime();
const remainingTime = dateInMilliseconds - nowInMilliseconds;
// 数据计算
const days = Math.floor(remainingTime / (1000 * 60 * 60 * 24));
const hours = Math.floor((remainingTime % (1000 * 60 * 60 * 24)) / (1000 * 60 * 60));
const minutes = Math.floor((remainingTime % (1000 * 60 * 60)) / (1000 * 60));
const seconds = Math.floor((remainingTime % (1000 * 60)) / 1000);
// remainingTime:剩余时间
if (remainingTime <= 0) {
cdown.controlError();
clearInterval(cdown.timer);
return ;
}
cdown.message.classList.remove("error");
cdown.updateDom({ seconds, minutes, hours, days });
},
// 表单提交事件
formSubmit: function(e){
e.preventDefault();
// 获取倒计时标题
cdown.message.textContent = cdown.name.value;
cdown.name.value = "";
cdown.name.focus();
// 获取目标时间
cdown.targetDate = cdown.dateDom.value;
// 创建定时器
cdown.opencountdown(cdown.targetDate)
// 显示效果
cdown.label.classList.remove("span-up");
cdown.form.style.display='none';
cdown.cancelDom.style.display='';
cdown.errorDom.style.display='none';
document.querySelector(".result").style.display='';
},
// 表单点击事件
inputClick: function(e){
// 如果点击的是输入框,label添加样式
if (e.target === cdown.input) {
cdown.label.classList.add("span-up");
} else if (cdown.input.value) {
return;
} else {
cdown.label.classList.remove("span-up");
}
},
// 取消倒计时
cancel: function(e){
cdown.cancelDom.style.display='none';
document.querySelector(".result").style.display='none';
cdown.form.style.display='';
cdown.message.textContent='倒计时';
clearInterval(cdown.timer);
}
}
// 绑定事件
cdown.form.addEventListener("submit",cdown.formSubmit);
cdown.form.addEventListener("click",cdown.inputClick);
cdown.cancelDom.addEventListener("click",cdown.cancel);
style.css
*,
*::before,
*::after {
box-sizing: border-box;
padding: 0;
margin: 0;
}
:root {
--color-main: #333;
--color-head: rgb(73, 73, 73);
}
body {
box-sizing: border-box;
padding: 0;
margin: 0;
font-size: 16px;
font-family: "Nunito", sans-serif;
color: var(--color-main);
background-color: rgba(0, 0, 0, 0.01);
}
main {
display: flex;
gap: 2rem;
flex-direction: column;
margin: 0 auto;
width: 40vw;
height: 100vh;
font-size: min(2vw, 1rem);
align-items: center;
justify-content: center;
text-align: center;
}
main h1 {
font-size: 3.5em;
color: var(--color-head);
}
.error {
--color-head: rgba(128, 15, 15, 0.659);
}
.result {
display: flex;
gap: 20px;
font-size: 3em;
}
.result p {
display: flex;
flex-direction: column;
align-items: center;
}
.result p span {
font-size: 0.3em;
color: rgb(102, 102, 102);
}
/* form input {
padding: 10px 15px;
border-radius: 5px;
border: 0;
outline: none;
border-bottom: 2px solid rgba(0, 0, 0, 0.1);
} */
form {
display: flex;
align-items: center;
justify-content: space-around;
position: relative;
padding: 10px 15px;
width: 100%;
/* background-color: green; */
}
form .name {
height: 100%;
width: 50%;
}
form .name input {
position: absolute;
bottom: 10px;
left: 40px;
height: 100%;
padding: 10px 5px 0px;
border: 0;
border-bottom: 2px solid rgba(31, 202, 122, 0.26);
background-color: transparent;
transition: all 0.2s ease-out;
font-size: 1.09em;
color: #333;
}
form .name input:focus {
outline: none;
border-bottom: 2px solid rgba(31, 202, 122, 0.781);
}
.span-up {
transform: translate(-15px, -18px) scale(0.75);
color: rgba(102, 100, 100, 0.61);
}
form .name label {
position: absolute;
bottom: 10px;
left: 35px;
color: rgb(102, 100, 100);
font-size: 1em;
z-index: 3;
background-color: transparent;
height: 100%;
width: 30%;
border: 0;
}
form .name label span {
position: absolute;
bottom: 6px;
left: 7px;
transition: transform 0.3s ease-out, color 0.3s linear 0.25s;
}
.head span {
color: rgba(31, 202, 122, 0.781);
font-weight: 300;
}
button {
background-color: rgba(31, 202, 122, 0.781);
padding: 8px 10px;
border: 0;
border-radius: 5px;
}