链接
12. 整数转罗马数字
代码
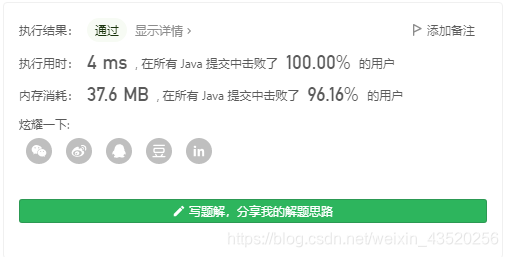
class Solution {
public String intToRoman(int num)
{
int[] values = new int[]{1000, 900, 500, 400, 100, 90, 50, 40, 10, 9, 5, 4, 1};
String[] reps = new String[]{"M", "CM", "D", "CD", "C", "XC", "L", "XL", "X", "IX", "V", "IV", "I"};
StringBuilder ret = new StringBuilder();
for (int i = 0; i < 13; i++)
{
while (num >= values[i])
{
num -= values[i];
ret.append(reps[i]);
}
}
return ret.toString();
}
}
链接
13. 罗马数字转整数
代码
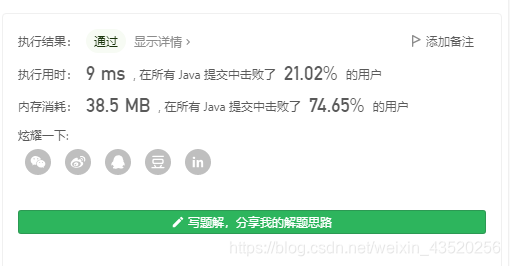
class Solution {
public int romanToInt(String s)
{
if (s == null)
{
return 0;
}
Map<Character, Integer> map = new TreeMap<>();
map.put('I', 1);
map.put('V', 5);
map.put('X', 10);
map.put('L', 50);
map.put('C', 100);
map.put('D', 500);
map.put('M', 1000);
int count = 0;
char[] chars = s.toCharArray();
for (int i = 0; i < chars.length; i++)
{
if (i == chars.length - 1)
{
count += map.get(chars[i]);
} else
{
if (map.get(chars[i]) >= map.get(chars[i + 1]))
{
count += map.get(chars[i]);
} else
{
count -= map.get(chars[i]);
}
}
}
return count;
}
}