学习《极客时间、spring全家桶学习记录贴》
Spring application context介绍:
Spring applicationcontext包含了业务pojo对象,还有配置的信息,Spring applicationcontext管理了所有的组件的生命周期,比如Inventoryservice、Productservice等组件。spring依赖注入也是通过上下文的实现的,在受托管的组件中,声明A组件依赖B组件,然后spring容器就会把依赖注入进去。
Spring应用上下文常用的接口
BeanFactory
DefaultListableBeanFactory
ApplicationContext的实现类:
ClassPathxmlApplicationContext
FileSystemXmlApplicationContext
AnnotationConfigApplicationContext
一般我们使用ApplicationContext接口及其实现,BeanFactory是最近本的应用上下文,springapplication context扩展了BeanFactory。开发过程中不建议直接使用BeanFactory。
web上下文层次:
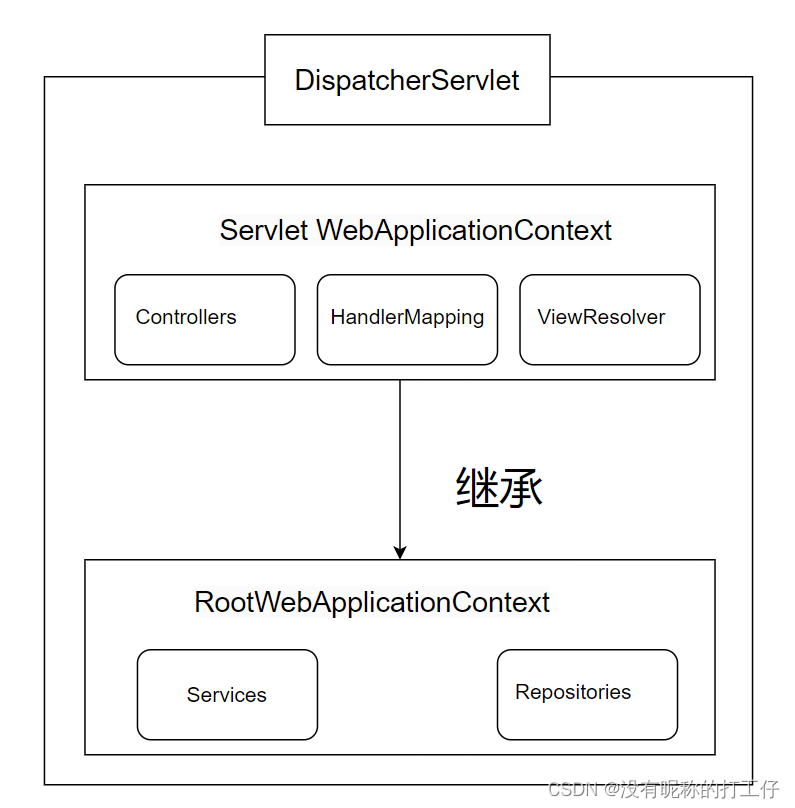
当需要在Servlet中寻找某个Bean时,如果找不到,sping会到Root中去寻找。
通过Aop增强类方法的方式理解应用上下文
/**
* 在方法结束打印信息
*/
@Aspect
@Slf4j
public class FooAspect {
@AfterReturning("bean(testBean*)")
public void printAfter() {
log.info("after hello()");
}
}
/**
* 打印信息,知道哪个上下文做了增强
*/
@Data
@NoArgsConstructor
@AllArgsConstructor
@Slf4j
public class TestBean {
private String context;
public void hello() {
log.info("hello " + context);
}
}
/**
* implements ApplicationRunner 重写run方法
* @param args
* @throws Exception
*/
@Override
public void run(ApplicationArguments args) throws Exception {
//注解方式的上下文
ApplicationContext fatherContext = new AnnotationConfigApplicationContext(FatherConfig.class);
//声明fatherContext与childrenContext两个上下文是集成关系
ClassPathXmlApplicationContext childrenContext = new ClassPathXmlApplicationContext(
new String[] {"applicationContext.xml"}, fatherContext);
TestBean bean = fatherContext.getBean("testBeanA", TestBean.class);
bean.hello();
log.info("=============");
TestBean bean1 = childrenContext.getBean("testBeanA", TestBean.class);
bean1.hello();
TestBean bean2 = childrenContext.getBean("testBeanB", TestBean.class);
bean2.hello();
}
第一种情况:Father和children上下文中都对testBeanA做了AOP增强
@Configuration
@EnableAspectJAutoProxy
public class FatherConfig {
/**
* 声明AOPAspect
* @return
*/
@Bean
public FatherAspect fatherAspect() {
return new FatherAspect();
}
@Bean
public TestBean testBeanA() {
return new TestBean("Father");
}
@Bean
public TestBean testBeanB() {
return new TestBean("Father");
}
}
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:aop="http://www.springframework.org/schema/aop"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/aop
http://www.springframework.org/schema/aop/spring-aop.xsd">
<aop:aspectj-autoproxy/>
<bean id="testBeanA" class="com.springwork.high.context.TestBean">
<constructor-arg name="context" value="children" />
</bean>
<!-- <bean id="fatherAspect" class="com.springwork.high.context.FatherAspect" />-->
</beans>
结果:Father上下文中的了testBeanA、testBeanB都得到AOP增强,同时children可以得到Father上下文中testBeanA的增强方法
23:09:56.847 [main] INFO com.springwork.high.HighApplication - Started HighApplication in 2.261 seconds (JVM running for 3.135)
23:09:56.919 [main] INFO com.springwork.high.context.TestBean - hello Father
23:09:56.920 [main] INFO com.springwork.high.context.FatherAspect - after hello()
23:09:56.920 [main] INFO com.springwork.high.HighApplication - =============
23:09:56.920 [main] INFO com.springwork.high.context.TestBean - hello children
23:09:56.920 [main] INFO com.springwork.high.context.FatherAspect - after hello()
23:09:56.920 [main] INFO com.springwork.high.context.TestBean - hello Father
23:09:56.920 [main] INFO com.springwork.high.context.FatherAspect - after hello()
23:09:56.921 [main] INFO com.springwork.high.HighApplication -
第二种情况:Father上下文中做了AOP增强,children上下文中没有AOP增强
/**
* @author high
* @version 1.0.0
* @date 2023/3/26 21:45
* @EnableAspectJAutoProxy 开启AspectJAutoProxy支持
*/
@Configuration
@EnableAspectJAutoProxy
public class FatherConfig {
/**
* 声明AOPAspect
* @return
*/
@Bean
public FatherAspect fatherAspect() {
return new FatherAspect();
}
@Bean
public TestBean testBeanA() {
return new TestBean("Father");
}
@Bean
public TestBean testBeanB() {
return new TestBean("Father");
}
}
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:aop="http://www.springframework.org/schema/aop"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/aop
http://www.springframework.org/schema/aop/spring-aop.xsd">
<!-- <aop:aspectj-autoproxy/>-->
<bean id="testBeanA" class="com.springwork.high.context.TestBean">
<constructor-arg name="context" value="children" />
</bean>
<!-- <bean id="fatherAspect" class="com.springwork.high.context.FatherAspect" />-->
</beans>
结果:代表children的testBeanA没有被增强,发现children可以得到Father上下文中testBeanB增强
22:55:05.895 [main] INFO com.springwork.high.context.TestBean - hello Father
22:55:05.896 [main] INFO com.springwork.high.context.FatherAspect - after hello()
22:55:05.896 [main] INFO com.springwork.high.HighApplication - =============
22:55:05.896 [main] INFO com.springwork.high.context.TestBean - hello children
22:55:05.896 [main] INFO com.springwork.high.context.TestBean - hello Father
22:55:05.896 [main] INFO com.springwork.high.context.FatherAspect - after hello()
22:55:05.897 [main] INFO com.springwork.high.HighApplication -
第三种情况:Father上下文中没有AOP增强,children上下文中AOP增强了
@Configuration
@EnableAspectJAutoProxy
public class FatherConfig {
/**
* 声明AOPAspect
* @return
*/
// @Bean
// public FatherAspect fatherAspect() {
// return new FatherAspect();
// }
@Bean
public TestBean testBeanA() {
return new TestBean("Father");
}
@Bean
public TestBean testBeanB() {
return new TestBean("Father");
}
}
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:aop="http://www.springframework.org/schema/aop"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/aop
http://www.springframework.org/schema/aop/spring-aop.xsd">
<aop:aspectj-autoproxy/>
<bean id="testBeanA" class="com.springwork.high.context.TestBean">
<constructor-arg name="context" value="children" />
</bean>
<bean id="fatherAspect" class="com.springwork.high.context.FatherAspect" />
</beans>
结果:Father上下文中的没有testBeanA、testBeanB没有AOP增强,children上下文中testBeanA得到AOP增强了
23:02:16.950 [main] INFO com.springwork.high.HighApplication - Started HighApplication in 2.175 seconds (JVM running for 3.014)
23:02:17.070 [main] INFO com.springwork.high.context.TestBean - hello Father
23:02:17.070 [main] INFO com.springwork.high.HighApplication - =============
23:02:17.075 [main] INFO com.springwork.high.context.TestBean - hello children
23:02:17.076 [main] INFO com.springwork.high.context.FatherAspect - after hello()
23:02:17.076 [main] INFO com.springwork.high.context.TestBean - hello Father
23:02:17.077 [main] INFO com.springwork.high.HighApplication -
总结:
Father上下文中方法得到AOP增强后,children上下文可以在Father上下文中获取到增强后的方法;
children上下文中方法得到AOP增强后,Father上下文不能够在children上下文获取到增强后的方法;