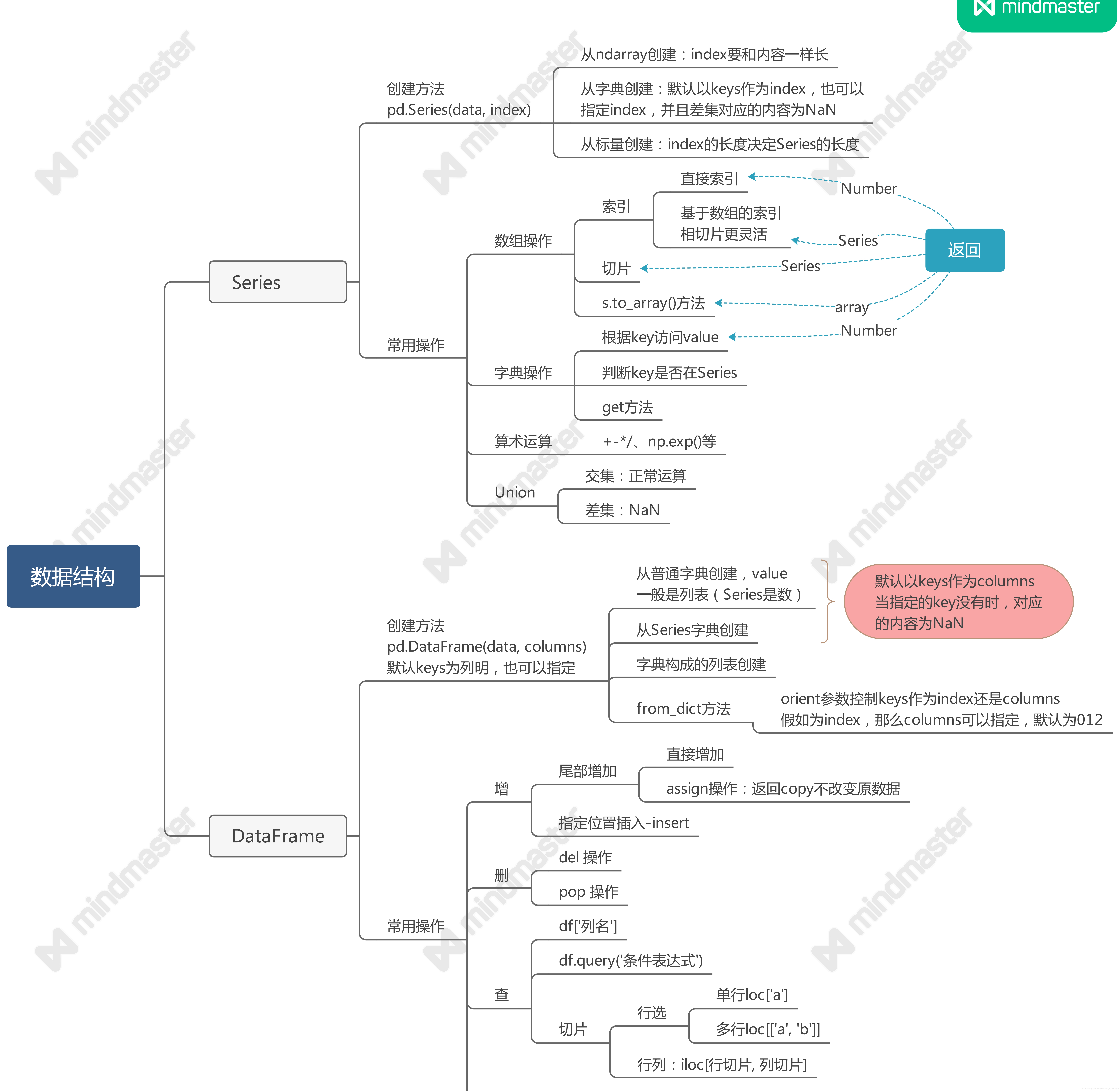
import numpy as np
import pandas as pd
Series类似于一维数组,不过添加了索引index
1、从ndarray创建
s = pd.Series(data=np.random.randint(0,5,size=3), index=['a', 'b', 'c'], dtype=int)
s
a 1
b 1
c 3
dtype: int32
2、从字典创建
data_dict = {'world':2, 'hello':1, }
s2 = pd.Series(data_dict)
s2
world 2
hello 1
dtype: int64
s2 = pd.Series(data_dict, index=['a', 'hello', 'world'])
s2
a NaN
hello 1.0
world 2.0
dtype: float64
3、从标量创建
s3 = pd.Series(5, index=['a', 'b', 'c'])
s3
a 5
b 5
c 5
dtype: int64
4、像数组一样操作
s[0]
1
s[0:3]
a 1
b 3
b 0
dtype: int32
s[[2,0]]
b 0
a 1
dtype: int32
np.exp(s)
a 2.718282
b 20.085537
b 1.000000
dtype: float64
s.array
<PandasArray>
[1, 3, 0]
Length: 3, dtype: int32
s.to_numpy()
array([1, 3, 0])
5、像字典一样操作
s2
a NaN
hello 1.0
world 2.0
dtype: float64
s2['hello']
1.0
for i in s2.index:
print(s2[i])
nan
1.0
2.0
'hello' in s2
True
s2.get('haha', np.asarray(0))
array(0)
6、np方法
s + s
a 2
b 2
c 6
dtype: int32
s
a 1
b 1
c 3
dtype: int32
s[1:] + s[:-1]
a NaN
b 2.0
c NaN
dtype: float64
(s[1:] + s[:-1]).dropna()
b 2.0
dtype: float64
DataFrame:可以理解为sql中的table,或者Series构成的字典,可以从以下创建
1、一维数组、列表、Series
2、二维列表
从字典创建
d = {"name":['jack', 'Tom'], "Age":[19, 20]}
df1 = pd.DataFrame(d)
df1
df1.Age
0 19
1 20
Name: Age, dtype: int64
从Serise字典创建
d2 = {'name':pd.Series(data=['jack', 'Tom'], index=['a', 'b']),
'Age':pd.Series(data=[19, 16], index=['a', 'b'])}
df2 = pd.DataFrame(d2)
df2
当索引index不一样的时候,会返回union
d3 = {'name':pd.Series(data=['jack', 'Tom'], index=['a', 'b']),
'Age':pd.Series(data=[19, 16])}
df3 = pd.DataFrame(d3)
df3
| name | Age |
---|
a | jack | NaN |
---|
b | Tom | NaN |
---|
0 | NaN | 19.0 |
---|
1 | NaN | 16.0 |
---|
pd.DataFrame(d2, index=['a', 'd'], columns=['name', 'M/F'])
从字典构成的列表创建
data = [{'a':1, 'b':2}, {'a':10, 'b':15, 'c':20}]
pd.DataFrame(data)
pd.DataFrame(data, columns=['a', 'b'])
一般而言字典的key是作为columns,不过调用pd.DataFrame.from_dict(),并且将orient参数设置为’index’可以把key设置为索引
默认情况下,orient等于columns
pd.DataFrame.from_dict({'a':[1,2,3], 'b':[4,5,6]}, orient='index', columns=['first', 'second', 'third'])
pd.DataFrame.from_dict({'a':[1,2,3], 'b':[4,5,6]})
columns的增删改查
df4=pd.DataFrame.from_dict({'a':[1,2,3], 'b':[4,5,6]}, orient='index', columns=['first', 'second', 'third'])
df4
查
df4['first']
a 1
b 4
Name: first, dtype: int64
df4.query('first > 2').query('second < 15')
改
df4['third'] = df4['first'] * df4['second']
df4
df[1,2] = 2
| first | second | third |
---|
a | 1 | 2 | 2 |
---|
b | 4 | 5 | 20 |
---|
增
df4['flag'] = df4['first']>1
df4
| first | second | third | flag |
---|
a | 1 | 2 | 2 | False |
---|
b | 4 | 5 | 20 | True |
---|
df4['forth'] = 4
df4
| first | second | third | flag | forth |
---|
a | 1 | 2 | 2 | False | 4 |
---|
b | 4 | 5 | 20 | True | 4 |
---|
df4.insert(0, 'haha', df4['first'])
df4
| haha | first | second | third | flag | forth |
---|
a | 1 | 1 | 2 | 2 | False | 4 |
---|
b | 4 | 4 | 5 | 20 | True | 4 |
---|
del df4['haha']
del df4['flag']
df4
| first | second | third | forth |
---|
a | 1 | 2 | 2 | 4 |
---|
b | 4 | 5 | 20 | 4 |
---|
assign用来新增column
df4.assign(ratio=lambda x: x['first'] / x['second'])
| first | second | third | forth | ratio |
---|
a | 1 | 2 | 2 | 4 | 0.5 |
---|
b | 4 | 5 | 20 | 4 | 0.8 |
---|
df4
| first | second | third | forth |
---|
a | 1 | 2 | 2 | 4 |
---|
b | 4 | 5 | 20 | 4 |
---|
df4.assign(sum2=df4['first'] + df4['second'])
| first | second | third | forth | sum2 |
---|
a | 1 | 2 | 2 | 4 | 3 |
---|
b | 4 | 5 | 20 | 4 | 9 |
---|
删
del df4['forth']
df4
| first | second | third | flag |
---|
a | 1 | 2 | 2 | False |
---|
b | 4 | 5 | 20 | True |
---|
df4.pop('forth')
a 4
b 4
Name: forth, dtype: int64
df4
df4.loc['b'].to_numpy()
array([4, 5, 6], dtype=int64)
df4.iloc[:2, :]
DataFrame算术运算
df4+1
df4.sub(df4['first'], axis=0)
np.sqrt(df4)
| first | second | third |
---|
a | 1.0 | 1.414214 | 1.732051 |
---|
b | 2.0 | 2.236068 | 2.449490 |
---|
获取表格信息
df4.info()
<class 'pandas.core.frame.DataFrame'>
Index: 2 entries, a to b
Data columns (total 3 columns):
# Column Non-Null Count Dtype
--- ------ -------------- -----
0 first 2 non-null int64
1 second 2 non-null int64
2 third 2 non-null int64
dtypes: int64(3)
memory usage: 144.0+ bytes
print(df4.to_string())
first second third
a 1 2 3
b 4 5 6
用访问属性的方式访问列
df6 = pd.DataFrame({'foo1': np.random.randn(5),'foo2': np.random.randn(5)})
df6
| foo1 | foo2 |
---|
0 | -0.663151 | -1.037235 |
---|
1 | 0.492857 | -0.012955 |
---|
2 | 0.775553 | 0.736301 |
---|
3 | 0.533148 | -2.451446 |
---|
4 | -0.748240 | -0.465478 |
---|
df6.foo1
0 -0.663151
1 0.492857
2 0.775553
3 0.533148
4 -0.748240
Name: foo1, dtype: float64