1. geojson配置后效果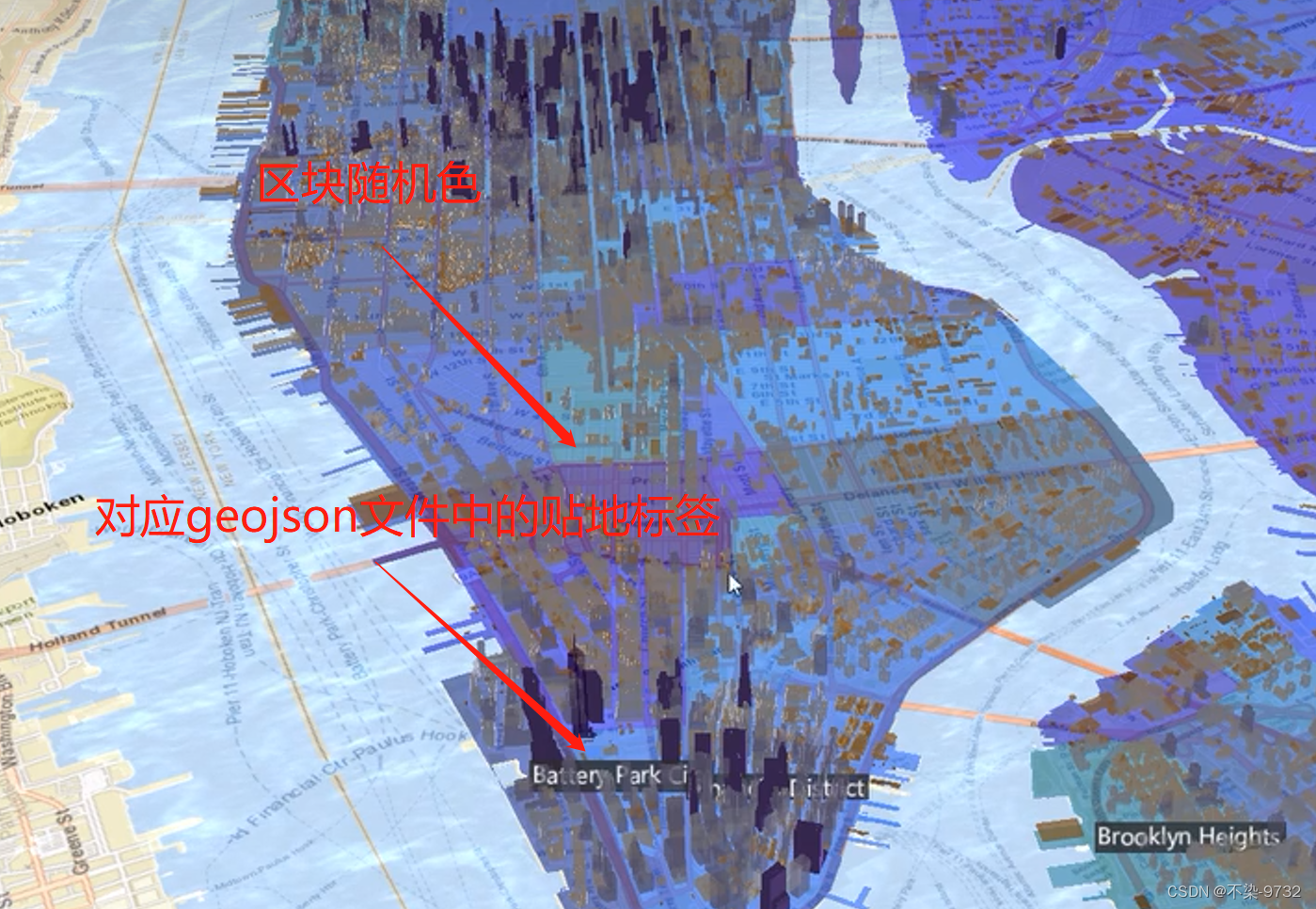
1. 数据文件加载的时候会遇到的情况:
① Blocked script execution in ‘about:blank’ because the document’s frame is sandboxed and the ‘allow-scripts’ permission is not set.
上面这个问题是由于cesium的沙盒配置不兼容导致的,解决方案:
方案1 配置沙盒中的属性
var iframe = document.getElementsByClassName("cesium-infoBox-iframe")[0];
iframe.setAttribute(
"sandbox",
"allow-same-origin allow-scripts allow-popups allow-forms"
);
iframe.setAttribute("src", ""); //必须设置src为空 否则不会生效
方案2 关闭试图中的沙盒
let viewer = new Cesium.Viewer("cesiumContainer", {
//cesium的查看器的基本属性
baseLayerPicker: true, //配置图层底图的图标
// imageryProvider:custom ,//使用上面自己配置的底图
terrainProvider: Cesium.createWorldTerrain({
//设置cesium的世界地形
requestVertexNormals: true, //地形的开启
requestWaterMask: true, //水面效果的开启
infoBox:false, 在这里关闭沙盒
}),
});
② GET http://127.0.0.1:5173/assets/simplestyles.geojson 404 (Not Found)
无法找到自己的文件 ,解决方案:将自己的geojson文件移动到public下
2. 加载自己的geojson并且配置加载完成后的地形区块完整功能块 带标签属性设置
// geojson文件加载
let neighborHoodsPromise = Cesium.GeoJsonDataSource.load(
"./assets/sampleNeighborhoods.geojson"
);
console.log("读取到的文件数据是:", neighborHoodsPromise);
let neighborhoods;
neighborHoodsPromise
.then((dataSource) => {
console.log(".then的结果是:", dataSource);
// 将数据放置到查看器中
neighborhoods = dataSource.entities;
let neighborhoodsEntities = dataSource.entities.values;
for (let i = 0; i < neighborhoodsEntities.length; i++) {
let entity = neighborhoodsEntities[i];
console.log("得到的对象是entity:", entity);
if (Cesium.defined(entity.polygon)) {
//判断当前的对象是否存在
entity.name = entity.properties.neighborhood;
// 多边形材质的设定
entity.polygon.material = Cesium.Color.fromRandom({
//设置随机颜色
red: 0.1,
maximumGreen: 0.5,
minimumBlue: 0.5,
alpha: 0.6, //透明度
});
// 多边形地形着色的操作
entity.polygon.classificationType = Cesium.ClassificationType.TERRAIN;
//获取具体的时间和位置信息
let polyPositions = entity.polygon.hierarchy.getValue(
Cesium.JulianDate.now()
).positions;
//设置中心点 贴到地形的表面
let polyCenter =
Cesium.BoundingSphere.fromPoints(polyPositions).center;
polyCenter =
Cesium.Ellipsoid.WGS84.scaleToGeocentricSurface(polyCenter);
entity.position = polyCenter;
//生成标签
entity.label = {
text: entity.name,
showBackground: true, //显示背景颜色
scale: 0.6, //设置缩放比例
horizontalOrigin: Cesium.HorizontalOrigin.center, //显示的位置
verticalOrigin: Cesium.VerticalOrigin.BOTTOM, //垂直原点贴近地表
distanceDisplayCondition: new Cesium.DistanceDisplayCondition(
10,
8000
), //设置展示范围 高度在10-8000的时候显示标签
disableDepthTestDistance: 100, //太远的时候标签不能点
};
}
}
})
.catch((e) => {});
3. 单页面完整代码
<template>
<div id="cesiumContainer"></div>
</template>
<script setup>
import * as Cesium from "cesium";
import { onMounted } from "vue";
onMounted(() => {
// 配置cesium中的相关属性
// let custom = new Cesium.ArcGisMapServerImageryProvider({
// url: "//servicse.arcgisonline.com/ArcGIS/rest/services/World_Street_Map/MapServer",
// });
let viewer = new Cesium.Viewer("cesiumContainer", {
//cesium的查看器的基本属性
baseLayerPicker: true, //配置图层底图的图标
// imageryProvider:custom ,//使用上面自己配置的底图
terrainProvider: Cesium.createWorldTerrain({
//设置cesium的世界地形
requestVertexNormals: true, //地形的开启
requestWaterMask: true, //水面效果的开启
// infoBox:false,
}),
});
// 设置默认相机视角
// viewer.camera.setView({
// destination: Cesium.Cartesian3.fromDegrees(113.318977, 23.114155, 2000),//广州坐标
// // 方向,俯视和仰视的视角
// orientation: {
// heading: Cesium.Math.toRadians(90), //坐标系旋转90度
// pitch: Cesium.Math.toRadians(-45), //设置俯仰角度为-45度
// },
// });
// 设置默认相机视角
viewer.camera.setView({
destination: new Cesium.Cartesian3(1332761, -4662399, 4137888), //纽约的地理坐标
orientation: {
heading: 0.6,
pitch: -0.66,
// pitch: Cesium.Math.toRadians(-90), //设置俯仰角度为-45度
},
});
let city = viewer.scene.primitives.add(
new Cesium.Cesium3DTileset({ url: Cesium.IonResource.fromAssetId(75343) })
);
// 定义3d样式
let heightStyle = new Cesium.Cesium3DTileStyle({
color: {
// 条件判断建筑物的颜色
conditions: [
["${Height} >= 300", "rgba(45,0,75,0.5)"],
["${Height}>=200", "rgb(102,71,151)"],
["${Height}>=100", "rgba(170,162,204,0.5)"],
["${Height}>=50", "rgb(224,226,238)"],
["${Height}>=25", "rgb(252,230,200)"],
["${Height}>=10", "rgba(248,176,87,0.5)"],
["${Height}>=5", "rgb(198,106,11)"],
["true", "rgb(127,59,8)"],
],
},
});
city.style = heightStyle;
// viewer.dataSources.add(
// Cesium.GeoJsonDataSource.load("./assets/sampleNeighborhoods.geojson")
// );
// geojson文件加载
let neighborHoodsPromise = Cesium.GeoJsonDataSource.load(
// "../public/mock/sampleNeighborhoods.geojson"
"./assets/simplestyles.geojson"
);
let neighborhoods;
// var iframe = document.getElementsByClassName("cesium-infoBox-iframe")[0];
// iframe.setAttribute(
// "sandbox",
// "allow-same-origin allow-scripts allow-popups allow-forms"
// );
var iframe = document.getElementsByClassName("cesium-infoBox-iframe")[0];
iframe.setAttribute(
"sandbox",
"allow-same-origin allow-scripts allow-popups allow-forms"
);
iframe.setAttribute("src", ""); //必须设置src为空 否则不会生效
console.log("读取到的文件数据是:", neighborHoodsPromise);
neighborHoodsPromise
.then((dataSource) => {
console.log(".then的结果是:", dataSource);
// 将数据放置到查看器中
neighborhoods = dataSource.entities;
let neighborhoodsEntities = dataSource.entities.values;
for (let i = 0; i < neighborhoodsEntities.length; i++) {
let entity = neighborhoodsEntities[i];
console.log("得到的对象是entity:", entity);
if (Cesium.defined(entity.polygon)) {
//判断当前的对象是否存在
entity.name = entity.properties.neighborhood;
// 多边形材质的设定
entity.polygon.material = Cesium.Color.fromRandom({
//设置随机颜色
red: 0.1,
maximumGreen: 0.5,
minimumBlue: 0.5,
alpha: 0.6, //透明度
});
// 多边形地形着色的操作
entity.polygon.classificationType = Cesium.ClassificationType.TERRAIN;
//获取具体的时间和位置信息
let polyPositions = entity.polygon.hierarchy.getValue(
Cesium.JulianDate.now()
).positions;
//设置中心点 贴到地形的表面
let polyCenter =
Cesium.BoundingSphere.fromPoints(polyPositions).center;
polyCenter =
Cesium.Ellipsoid.WGS84.scaleToGeocentricSurface(polyCenter);
entity.position = polyCenter;
//生成标签
entity.label = {
text: entity.name,
showBackground: true, //显示背景颜色
scale: 0.6, //设置缩放比例
horizontalOrigin: Cesium.HorizontalOrigin.center, //显示的位置
verticalOrigin: Cesium.VerticalOrigin.BOTTOM, //垂直原点贴近地表
distanceDisplayCondition: new Cesium.DistanceDisplayCondition(
10,
8000
), //设置展示范围 高度在10-8000的时候显示标签
disableDepthTestDistance: 100, //太远的时候标签不能点
};
}
}
})
.catch((e) => {});
});
</script>
<style scoped>
#cesiumContainer {
width: 100% !important;
height: 100% !important;
margin: 0 !important;
padding: 0 !important;
overflow: hidden;
position: absolute;
}
</style>