数据结构之队列(c语言版)
队列(Queue):在逻辑上是一种线性存储结构。它有以下几个特点:
1、队列中数据是按照"先进先出(FIFO, First-In-First-Out)"方式进出队列的。
2、 队列只允许在"队首"进行删除操作,而在"队尾"进行插入操作。
队列通常包括的两种操作:入队列 和 出队列。
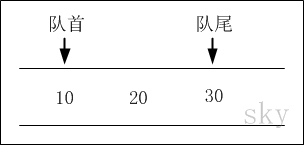
队列的种类也很多,单向队列,双向队列,循环队列。
底层可以由数组(顺序表),链表实现。
一、数组队列
基于数组实现的单向队列:
#include<stdio.h>
#include<stdlib.h>
static int *queue=NULL;//指向队列
static int count=0;//元素数量
static int MAXSIZE=20;//队列容量
//创建队列
int *create_queue(){
queue=(int *)malloc(MAXSIZE*sizeof(int));//分配空间
if(!queue){
printf("queue error!");
exit(0);
}
return queue;//返回队列
}
//判空
int Empty(){
if(count==0){
return 1;
}
return 0;
}
//判满
int full(){
if(MAXSIZE==count){
return 1;
}
return 0;
}
//入队
void enQueue(int e){
if(full()){
printf("队列已满,不允许入队\n");
exit(0);
}
queue[count++]=e;
printf("enQueue successful!!\n");
}
//出队
int deQueue(){
if(Empty()){
printf("空队,无法出队\n");
exit(0);
}
int n=queue[0];
for(int i=0;i<count;i++){
queue[i]=queue[i+1];
}
count--;
return n;
}
//遍历
void display(){
printf("队列有%d个元素\n",count);
for(int i=0;i<count;i++){
printf("The data is %d\n",queue[i]);
}
printf("遍历结束\n");
}
//销毁队列
void destory(){
if(queue){
free(queue);
}
printf("destory!!!");
}
主函数:
int main(){
int *p=create_queue();
enQueue(2);
enQueue(8);
enQueue(108);
enQueue(99);
enQueue(9);
enQueue(4);
display();
deQueue();
deQueue();
display();
destory();
}
输出:
enQueue successful!!
enQueue successful!!
enQueue successful!!
enQueue successful!!
enQueue successful!!
enQueue successful!!
队列有6个元素
The data is 2
The data is 8
The data is 108
The data is 99
The data is 9
The data is 4
遍历结束
队列有4个元素
The data is 108
The data is 99
The data is 9
The data is 4
遍历结束
destory!!!
二、链表队列
基于链表实现的单向队列
#include<stdio.h>
#include<stdlib.h>
typedef struct queue
{
int data;
struct queue *next;
}Queue;
static int count=0;//数量
static Queue *head=NULL;//队首
static Queue *tail=NULL;//队尾
//节点初始化
Queue *init(){
Queue *p=(Queue *)malloc(sizeof(Queue));
return p;
}
//创建队列
Queue *create_queue(){
head=init();
tail=head;
if(!head){
printf("queue error!");
exit(0);
}
count++;
return head;
}
//判空
int Empty(){
if(count<=1){
return 1;
}
return 0;
}
//入队
void enQueue(int e){
Queue *p=init();
p->data=e;
tail->next=p;
tail=p;
count++;//数量增加
printf("enQueue!\n");
}
//出队
int deQueue(){
if(Empty()){
printf("queue Null");
exit(0);
}
Queue *p=head->next;
int n=p->data;
head->next=p->next;
free(p);
count--;
printf("dequeue!!\n");
return n;
}
//遍历
void display(){
if(Empty()){
printf("Null queue");
exit(0);
}
printf("队列中有%d个元素\n",count);
Queue *p=head->next;
for(int i=1;i<count;i++){
printf("The data is %d\n",p->data);
p=p->next;
}
printf("display close!\n");
}
主函数:
int main(){
Queue *p=create_queue();
enQueue(2);
enQueue(8);
enQueue(108);
enQueue(99);
enQueue(9);
enQueue(4);
display();
deQueue();
deQueue();
display();
}
输出:
enQueue!
enQueue!
enQueue!
enQueue!
enQueue!
enQueue!
队列中有7个元素
The data is 2
The data is 8
The data is 108
The data is 99
The data is 9
The data is 4
display close!
dequeue!!
dequeue!!
队列中有5个元素
The data is 108
The data is 99
The data is 9
The data is 4
display close!