1. 验证二叉树的前序序列化
One way to serialize a binary tree is to use preorder traversal. When we encounter a non-null node, we record the node’s value. If it is a null node, we record using a sentinel value such as '#'
.
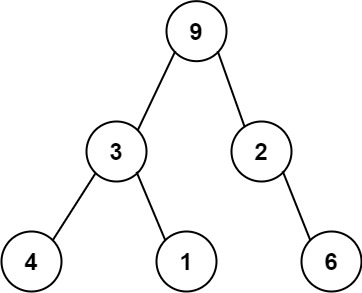
For example, the above binary tree can be serialized to the string "9,3,4,#,#,1,#,#,2,#,6,#,#"
, where '#'
represents a null node.
Given a string of comma-separated values preorder
, return true
if it is a correct preorder traversal serialization of a binary tree.
It is guaranteed that each comma-separated value in the string must be either an integer or a character '#'
representing null pointer.
You may assume that the input format is always valid.
- For example, it could never contain two consecutive commas, such as
"1,,3"
.
Note: You are not allowed to reconstruct the tree.
Example 1
Input: preorder = "9,3,4,#,#,1,#,#,2,#,6,#,#"
Output: true
Example 2
Input: preorder = "1,#"
Output: false
Constraints:
1 <= preorder.length <= 10^4
preorder
consist of integers in the range[0, 100]
and'#'
separated by commas','
.
代码
class Solution {
public:
bool isValidSerialization(string preorder) {
stringstream ss(preorder);
string tmp;
int in = -1, out = 0;
while (getline(ss, tmp, ',')) {
++in;
if (out < in) return false;
if (tmp[0] != '#') out += 2;
}
return in == out;
}
};
2. 二叉树的序列化与反序列化
Serialization is the process of converting a data structure or object into a sequence of bits so that it can be stored in a file or memory buffer, or transmitted across a network connection link to be reconstructed later in the same or another computer environment.
Design an algorithm to serialize and deserialize a binary tree. There is no restriction on how your serialization/deserialization algorithm should work. You just need to ensure that a binary tree can be serialized to a string and this string can be deserialized to the original tree structure.
Clarification: The input/output format is the same as how LeetCode serializes a binary tree. You do not necessarily need to follow this format, so please be creative and come up with different approaches yourself.
Example 1
Input: root = [1,2,3,null,null,4,5]
Output: [1,2,3,null,null,4,5]
Example 2
Input: root = []
Output: []
Constraints:
- The number of nodes in the tree is in the range
[0, 10^4]
. -1000 <= Node.val <= 1000
代码 [DFS]
class Codec {
public:
// Encodes a tree to a single string.
string serialize(TreeNode *root) {
stack < TreeNode * > st;
st.push(root);
string s;
while (!st.empty()) {
TreeNode *cur = st.top();
st.pop();
if (cur == nullptr) {
s += "#,";
} else {
s += to_string(cur->val) + ",";
st.push(cur->right);
st.push(cur->left);
}
}
s.pop_back();
return s;
}
TreeNode *dfs(stringstream &ss) {
string tmp;
getline(ss, tmp, ',');
if (tmp[0] == '#') return nullptr;
auto node = new TreeNode(stoi(tmp));
node->left = dfs(ss);
node->right = dfs(ss);
return node;
}
// Decodes your encoded data to tree.
TreeNode *deserialize(string data_str) {
stringstream ss(data_str);
return dfs(ss);
}
};
3. 兼具大小写的最好英文字母
Given a string of English letters s
, return the greatest English letter which occurs as both a lowercase and uppercase letter in s
. The returned letter should be in uppercase. If no such letter exists, return an empty string.
An English letter b
is greater than another letter a
if b
appears after a
in the English alphabet.
Example 1
Input: s = "lEeTcOdE"
Output: "E"
Explanation:
The letter 'E' is the only letter to appear in both lower and upper case.
Example 2
Input: s = "arRAzFif"
Output: "R"
Explanation:
The letter 'R' is the greatest letter to appear in both lower and upper case.
Note that 'A' and 'F' also appear in both lower and upper case, but 'R' is greater than 'F' or 'A'.
Constraints:
1 <= s.length <= 1000
s
consists of lowercase and uppercase English letters.
代码 [位运算]
class Solution {
public:
string greatestLetter(string s) {
int lo = 0;
for (auto ch:s) {
if (islower(ch)) lo |= 1 << (ch - 'a');
}
char ans = 0x40;
for (auto ch:s) {
if (isupper(ch) && (lo >> (ch - 'A')) & 1 && ch > ans) ans = ch;
}
return ans == 0x40 ? "" : string(1, ans);
}
};
4. 个位数字为 K 的整数之和
Given two integers num
and k
, consider a set of positive integers with the following properties:
- The units digit of each integer is
k
. - The sum of the integers is
num
.
Return the minimum possible size of such a set, or -1
if no such set exists.
Note:
- The set can contain multiple instances of the same integer, and the sum of an empty set is considered
0
. - The units digit of a number is the rightmost digit of the number.
Example 1
输入:num = 58, k = 9
输出:2
解释:
多重集 [9,49] 满足题目条件,和为 58 且每个整数的个位数字是 9 。
另一个满足条件的多重集是 [19,39] 。
可以证明 2 是满足题目条件的多重集的最小长度。
Example 2
输入:num = 37, k = 2
输出:-1
解释:个位数字为 2 的整数无法相加得到 37 。
Constraints:
0 <= num <= 3000
0 <= k <= 9
代码
class Solution {
public:
int minimumNumbers(int num, int k) {
if (num == 0) return 0;
if ((num & 1) && !(k & 1)) return -1;
int cnt[10]{0}, acc = 0, ans = 0, target = num % 10; // cnt 可用位运算
while (acc <= num) {
acc += k;
if (cnt[acc % 10] == 1) return -1;
++ans;
cnt[acc % 10] = 1;
if (acc % 10 == target) return ans;
}
return -1;
}
};
5. 小于等于 K 的最长二进制子序列
给你一个二进制字符串 s
和一个正整数 k
。
请你返回 s
的 最长 子序列,且该子序列对应的 二进制 数字小于等于 k
。
注意:
- 子序列可以有 前导 0 。
- 空字符串视为
0
。 - 子序列 是指从一个字符串中删除零个或者多个字符后,不改变顺序得到的剩余字符序列。
Example 1
输入:s = "1001010", k = 5
输出:5
解释:s 中小于等于 5 的最长子序列是 "00010" ,对应的十进制数字是 2 。
注意 "00100" 和 "00101" 也是可行的最长子序列,十进制分别对应 4 和 5 。
最长子序列的长度为 5 ,所以返回 5 。
Example 2
输入:s = "00101001", k = 1
输出:6
解释:"000001" 是 s 中小于等于 1 的最长子序列,对应的十进制数字是 1 。
最长子序列的长度为 6 ,所以返回 6 。
Constraints:
1 <= s.length <= 1000
s[i]
要么是'0'
,要么是'1'
。1 <= k <= 10^9
代码
又是灵神
的题解,哭了。
class Solution:
def longestSubsequence(self, s: str, k: int) -> int:
n, m = len(s), k.bit_length()
if n < m: return n
ans = m if int(s[n - m:], 2) <= k else m - 1
return ans + s.count('0', 0, n - m)
6. 卖木头块
You are given two integers m
and n
that represent the height and width of a rectangular piece of wood. You are also given a 2D integer array prices
, where prices[i] = [hi, wi, pricei]
indicates you can sell a rectangular piece of wood of height hi
and width wi
for pricei
dollars.
To cut a piece of wood, you must make a vertical or horizontal cut across the entire height or width of the piece to split it into two smaller pieces. After cutting a piece of wood into some number of smaller pieces, you can sell pieces according to prices
. You may sell multiple pieces of the same shape, and you do not have to sell all the shapes. The grain of the wood makes a difference, so you cannot rotate a piece to swap its height and width.
Return the maximum money you can earn after cutting an m x n
piece of wood.
Note that you can cut the piece of wood as many times as you want.
Example 1
Input: m = 3, n = 5, prices = [[1,4,2],[2,2,7],[2,1,3]]
Output: 19
Explanation: The diagram above shows a possible scenario. It consists of:
- 2 pieces of wood shaped 2 x 2, selling for a price of 2 * 7 = 14.
- 1 piece of wood shaped 2 x 1, selling for a price of 1 * 3 = 3.
- 1 piece of wood shaped 1 x 4, selling for a price of 1 * 2 = 2.
This obtains a total of 14 + 3 + 2 = 19 money earned.
It can be shown that 19 is the maximum amount of money that can be earned.
Example 2
Input: m = 4, n = 6, prices = [[3,2,10],[1,4,2],[4,1,3]]
Output: 32
Explanation: The diagram above shows a possible scenario. It consists of:
- 3 pieces of wood shaped 3 x 2, selling for a price of 3 * 10 = 30.
- 1 piece of wood shaped 1 x 4, selling for a price of 1 * 2 = 2.
This obtains a total of 30 + 2 = 32 money earned.
It can be shown that 32 is the maximum amount of money that can be earned.
Notice that we cannot rotate the 1 x 4 piece of wood to obtain a 4 x 1 piece of wood.
Constraints:
1 <= m, n <= 200
1 <= prices.length <= 2 * 10^4
prices[i].length == 3
1 <= hi <= m
1 <= wi <= n
1 <= pricei <= 10^6
- All the shapes of wood
(hi, wi)
are pairwise distinct.
代码