import React, { useEffect, useRef } from 'react';
const ExposedElement: React.FC = () => {
const targetRef = useRef<HTMLDivElement>(null);
useEffect(() => {
const observer = new IntersectionObserver(
([entry]) => {
if (entry.isIntersecting) {
console.log('Element is exposed:', entry.target);
// 在这里执行曝光后的逻辑
}
},
{ threshold: 0 } // 出现就算曝光
);
if (targetRef.current) {
observer.observe(targetRef.current);
}
return () => {
if (targetRef.current) {
observer.unobserve(targetRef.current);
}
};
}, []);
return (
<div>
<div style={{ height: '1500px' }}>
<div
ref={targetRef}
style={{ height: '300px', backgroundColor: 'yellow' }}
>
Target Element
</div>
</div>
</div>
);
};
export default ExposedElement;
检测元素是否在视野内React
最新推荐文章于 2024-05-12 23:38:06 发布
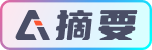