import React, { Component } from 'react'
import styled from 'styled-components'
import moment from 'moment'
import { TRAINING_RECORD_COURSE_TYPE } from '../../common/const'
export default class RecordCalendar extends Component {
static propTypes = {
}
static defaultProps = {
}
state = {
monthList: [
{
year: 2023,
month: '01',
},
{
year: 2023,
month: '02',
}
],
dataList: [
{
"trainingDate": "2023-02-01",
"typeList": [
1,
6
]
},
{
"trainingDate": "2023-02-03",
"typeList": [
6
]
},
{
"trainingDate": "2023-02-04",
"typeList": [
6
]
},
{
"trainingDate": "2023-02-05",
"typeList": [
1,
2,
3,
4,
5,
6
]
},
{
"trainingDate": "2023-02-07",
"typeList": [
4,
5
]
},
{
"trainingDate": "2023-02-08",
"typeList": [
6
]
},
{
"trainingDate": "2023-02-10",
"typeList": [
1,
6
]
},
{
"trainingDate": "2023-02-13",
"typeList": [
2
]
},
{
"trainingDate": "2023-02-16",
"typeList": [
1
]
},
{
"trainingDate": "2023-02-28",
"typeList": [
3
]
}
],
weekdays: ["Su", "Mo", "Tu", "We", "Th", "Fr", "Sa"],
calendarData: [],
}
componentDidMount() {
this.initModalData()
this.getMonthDays();
}
initModalData = () => {
}
getMonthDays = () => {
const year = this.state.monthList[1].year;
const month = this.state.monthList[1].month;
const currentMonthFirstDay = `${year}-${month}-01`
console.log('currentMonth: ', currentMonthFirstDay);
const currentWeekday = moment(currentMonthFirstDay).format('E');
console.log('currentWeekday: ', currentWeekday);
let currentMonthDays = moment(currentMonthFirstDay).daysInMonth();
console.log('currentMonthDays: ', currentMonthDays);
const currentMonthEndtDay = `${year}-${month}-${currentMonthDays}`
console.log('currentMonthEndtDay: ', currentMonthEndtDay);
const list = [];
let fillDaysBeforeFirstDay = 0;
if (currentWeekday !== 7) {
fillDaysBeforeFirstDay = currentWeekday;
for (let i = 0; i < fillDaysBeforeFirstDay; i++) {
list.push({
isShow: false,
})
}
}
console.log('fillDaysBeforeFirstDay: ', fillDaysBeforeFirstDay);
for (let i = 1; i <= 6 * 7 - fillDaysBeforeFirstDay; i++) {
if (i > currentMonthDays) {
if ( i > 35 - fillDaysBeforeFirstDay) {
continue;
} else {
list.push({
isShow: false,
})
continue;
}
}
const day = i.toString().padStart(2, '0')
const item = this.state.dataList.find((v) => v.trainingDate === `${year}-${month}-${day}`);
const type = item ? item.typeList : null;
let typeColors = null;
if (type && type.length) {
typeColors = type.map(t => TRAINING_RECORD_COURSE_TYPE.find((v) => v.value === t).color);
}
list.push({
year,
month,
day: i,
type,
typeColors,
})
}
console.log(list);
this.setState({calendarData: list})
}
createImg = () => {
}
render() {
return (
<ExportContainer>
<div onClick={() => this.createImg}>生成图片</div>
<CalendarContainer>
<div className='calendar-row'>
{
this.state.weekdays.map((v) => {
return <div className='calendar-row-item calendar-row-item-header' key={v}>{v}</div>
})
}
</div>
<div className='calendar-row'>
{
this.state.calendarData.map((v, index) => {
return <div className='calendar-row-item' key={index}>
{v.day}
<div className={ v.typeColors && v.typeColors.length > 3 ? 'types' : 'types-center'}>
{
v.typeColors && v.typeColors.map((color, idx) => {
return <div key={idx} style={{ background: color }} className='type-color'></div>
})
}
</div>
</div>
})
}
</div>
</CalendarContainer>
</ExportContainer>
)
}
}
const ExportContainer = styled.div`
// min-width: 1154px;
// min-height: 940px;
min-width: 540px;
width: 540px;
min-height: 240px;
background: #FFBC0D;
padding: 49px;
`
const CalendarContainer = styled.div`
width: 317px;
height: 285px;
background: #FFF;
border-radius: 16px;
margin: 6px;
.calendar-row {
display: flex;
width: 267px;
flex-wrap: wrap;
margin: auto;
}
.calendar-row-item-header {
align-items: center;
color: #9A9A9A;
font-weight: 400 !important;
}
.calendar-row-item {
height: 44px;
flex-basis: 14%;
display: flex;
justify-content: center;
font-weight: 600;
position: relative;
}
.types {
position: absolute;
display: flex;
width: 24px;
top: 20px;
flex-wrap: wrap;
margin-left: 2px;
flex-wrap: wrap;
}
.types-center {
position: absolute;
display: flex;
top: 20px;
justify-content: center;
}
.type-color {
min-width: 5px;
min-height: 5px;
border-radius: 5px;
margin-right: 2px;
margin-bottom: 2px;
}
.ant-picker-calendar-header {
display: none;
}
`
效果如图:
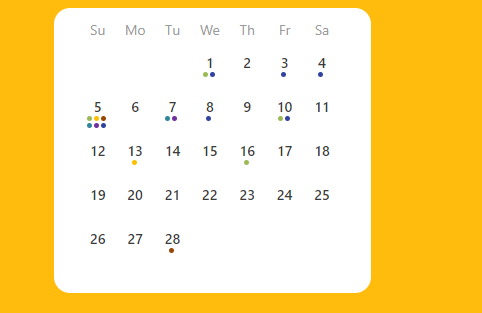