如果想进行springboot的开发,需要使用Maven或其他管理工具来完成这一过程。
springboot运行以web项目为主
开发项目只需按照官方文档来 ↵
↵
如果想进行springboot的开发,需要使用Maven或其他想募管理工具来完成这一过程
springboot运行以web项目
开发项目的具体模板依赖可以参考官方文档 https://start.spring.io/
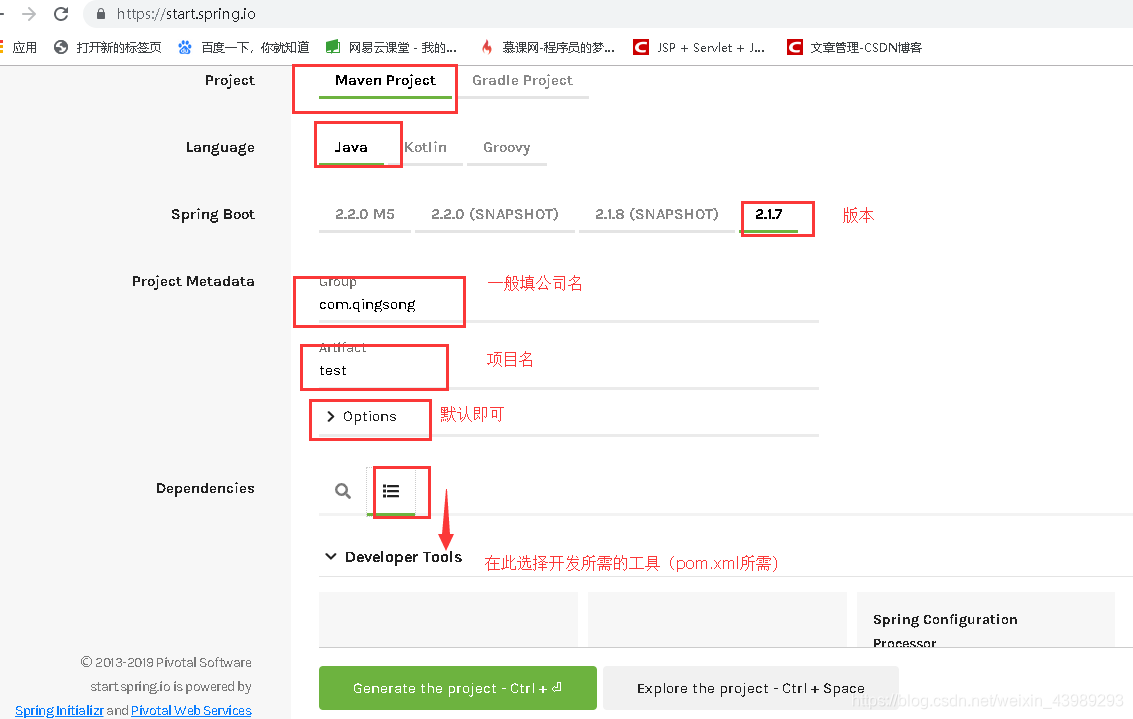
explore the project后选择本地路径,解压该文件到eclipse工作路径,在eclipse中导入该项目即可创建初步的maven工程。
本文参考此视频编写 : https://www.bilibili.com/video/av61303794
步骤:
1.创建一个maven工程
2.导入依赖springboot相关的依赖
<parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>2.1.7.RELEASE</version> <relativePath/> <!-- lookup parent from repository --> </parent> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> |
3.编写一个主程序,启动springboot应用
import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; /** * * @author taqsbj * @SpringBootApplication标注一个主程序,说明这是一个springboot应用 * */ @SpringBootApplication public class App { public static void main( String[] args ) { //将spring应用启动 SpringApplication.run(App.class, args); } } |
4.编写所需的相关的Controller等
,如:
import org.springframework.stereotype.Controller; import org.springframework.ui.Model; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.ResponseBody; @Controller public class UserController { @RequestMapping("/hello") @ResponseBody public String hello() { return "hello,world"; } @RequestMapping("/index") public String index(Model model) { model.addAttribute("name", "sheryll"); model.addAttribute("age", 20); model.addAttribute("info", "我是一个爱学习小淘气"); return "index"; } } |
5.测试程序
直接运行main方法(启动)
localhost://8080/hello(访问hello)
locallhost://8080/index(访问index)
6.运行主程序测试
导入<build>插件
<!-- 插件,此插件可以将应用打包成一个可执行的jar包 --> <build> <plugins> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> </plugin> </plugins> </build> |
将这个应用导成jar包后,可在命令窗口使用 java -jar 命令运行。
完整的pom.xml配置文件如下:
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com</groupId> <artifactId>SpringBoot-1</artifactId> <version>0.0.1-SNAPSHOT</version> <name>SpringBoot-1</name> <url>http://maven.apache.org</url> <properties> <jdk.version>1.8</jdk.version> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> </properties> <!--Springboot相关jar包版本--> <packaging>jar</packaging> <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>2.1.7.RELEASE</version> </parent> <!-- 相关jar包 --> <dependencies> <!-- Springboot核心jar包 --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter</artifactId> </dependency> <!-- web开发包:包含Tomcat和Springmvc --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <!-- thymeleaf --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-thymeleaf</artifactId> </dependency> <!-- jdbc链接容器 --> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> </dependency> <!-- Junit测试jar包 --> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <scope>test</scope> </dependency> <!-- springboot热部署(凡是文件修改保存后,自动重启) --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-devtools</artifactId> <optional>true</optional> <scope>runtime</scope> </dependency> <!-- jpa(已包含hibernate) --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-data-jpa</artifactId> </dependency> </dependencies> <!-- 插件,此插件可以将应用打包成一个可执行的jar包 --> <build> <plugins> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> <configuration> <fork>true</fork> <addResources>true</addResources> </configuration> </plugin> </plugins> </build> </project> |
注意:版本号要与eclipse的设置一致
更复杂的工程:
创建resources文件夹(src/main/java/resources)在该路径下创建新的文件命名为application.yml进行服务配置
如下:
server: port: 80 session-timeout: 30 tomcat.max-threads: 0 tomcat.uri-encoding: UTF-8 #spring相关配置 spring: thymeleaf: prefix: classpath:/templates/ suffix: .html mode: HTML5 encoding: UTF-8 content-type: text/html cache: false #配置数据库相关信息 datasource: url: jdbc:mysql://localhost:端口号/数据库名 driver-class-name: com.mysql.jdbc.Driver username: root password: 数据库密码 initial-size: 10 max-active: 20 max-idle: 8 min-idle: 8 #配置jpa(hibernate) jpa: database: mysql show-sql: true hibernate: ddl-auto: update naming: physical-strategy: org.springframework.boot.orm.jpa.hibernate.SpringPhysicalNamingStrategy database-platform: org.hibernate.dialect.MySQL5InnoDBDialect |
在mysql中创建的数据库要与之对应 保持一致
利用bean实现与前台页面的交互
利用servlet获取数据(包括接口和实现(接口的)类)
新建controller类
新建index.html前台页面展示获取到的后台数据
注意:在前端显示时通常需要用到thymeleaf(相当于c标签)
需要:
在pom.xml中添加thymeleaf配置
在resources文件夹下分别创建templates和static文件夹,分别存放html文件和css、js文件